Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Common / DbProviderManifest.cs / 1 / DbProviderManifest.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Collections.ObjectModel; using System.Data.Metadata.Edm; using System.Xml; namespace System.Data.Common { ////// Metadata Interface for all CLR types types /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public abstract class DbProviderManifest { ////// Constructor /// protected DbProviderManifest() { } ///Value to pass to GetInformation to get the StoreSchemaDefinition public static readonly string StoreSchemaDefinition = "StoreSchemaDefinition"; ///Value to pass to GetInformation to get the StoreSchemaMapping public static readonly string StoreSchemaMapping = "StoreSchemaMapping"; ///Value to pass to GetInformation to get the ConceptualSchemaDefinition public static readonly string ConceptualSchemaDefinition = "ConceptualSchemaDefinition"; // System Facet Info ////// Name of the MaxLength Facet /// internal const string MaxLengthFacetName = "MaxLength"; ////// Name of the Unicode Facet /// internal const string UnicodeFacetName = "Unicode"; ////// Name of the FixedLength Facet /// internal const string FixedLengthFacetName = "FixedLength"; ////// Name of the Precision Facet /// internal const string PrecisionFacetName = "Precision"; ////// Name of the Scale Facet /// internal const string ScaleFacetName = "Scale"; ////// Name of the Nullable Facet /// internal const string NullableFacetName = "Nullable"; ////// Name of the DefaultValue Facet /// internal const string DefaultValueFacetName = "DefaultValue"; ////// Name of the Collation Facet /// internal const string CollationFacetName = "Collation"; ////// Returns the namespace used by this provider manifest /// public abstract string NamespaceName {get;} ////// Return the set of types supported by the store /// ///A collection of primitive types public abstract System.Collections.ObjectModel.ReadOnlyCollectionGetStoreTypes(); /// /// Returns all the edm functions supported by the provider manifest. /// ///A collection of edm functions. public abstract System.Collections.ObjectModel.ReadOnlyCollectionGetStoreFunctions(); /// /// Returns all the FacetDescriptions for a particular type /// /// the type to return FacetDescriptions for ///The FacetDescriptions for the type given [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "edm")] public abstract System.Collections.ObjectModel.ReadOnlyCollectionGetFacetDescriptions(EdmType edmType); /// /// This method allows a provider writer to take a type and a set of facets /// and reason about what the best mapped equivalent type in EDM would be. /// /// A TypeUsage encapsulating a store type and a set of facets ///A TypeUsage encapsulating an EDM type and a set of facets [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] public abstract TypeUsage GetEdmType(TypeUsage storeType); ////// This method allows a provider writer to take a type and a set of facets /// and reason about what the best mapped equivalent type in the store would be. /// /// A TypeUsage encapsulating an EDM type and a set of facets ///A TypeUsage encapsulating a store type and a set of facets [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "edm")] public abstract TypeUsage GetStoreType(TypeUsage edmType); ////// Providers should override this to return information specific to their provider. /// /// This method should never return null. /// /// The name of the information to be retrieved. ///An XmlReader at the begining of the information requested. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] protected abstract XmlReader GetDbInformation(string informationType); ////// Gets framework and provider specific information /// /// This method should never return null. /// /// The name of the information to be retrieved. ///An XmlReader at the begining of the information requested. public XmlReader GetInformation(string informationType) { XmlReader reader = null; try { reader = GetDbInformation(informationType); } catch (Exception e) { // we should not be wrapping all exceptions if (EntityUtil.IsCatchableExceptionType(e)) { // we don't want folks to have to know all the various types of exceptions that can // occur, so we just rethrow a ProviderIncompatibleException and make whatever we caught // the inner exception of it. throw EntityUtil.ProviderIncompatible( System.Data.Entity.Strings.EntityClient_FailedToGetInformation(informationType), e); } throw; } if (reader == null) { // if the provider returned null for the conceptual schema definition, return the default one if (informationType == ConceptualSchemaDefinition) { return DbProviderServices.GetConceptualSchemaDescription(); } throw EntityUtil.ProviderIncompatible(System.Data.Entity.Strings.ProviderReturnedNullForGetDbInformation(informationType)); } return reader; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Collections.ObjectModel; using System.Data.Metadata.Edm; using System.Xml; namespace System.Data.Common { ////// Metadata Interface for all CLR types types /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public abstract class DbProviderManifest { ////// Constructor /// protected DbProviderManifest() { } ///Value to pass to GetInformation to get the StoreSchemaDefinition public static readonly string StoreSchemaDefinition = "StoreSchemaDefinition"; ///Value to pass to GetInformation to get the StoreSchemaMapping public static readonly string StoreSchemaMapping = "StoreSchemaMapping"; ///Value to pass to GetInformation to get the ConceptualSchemaDefinition public static readonly string ConceptualSchemaDefinition = "ConceptualSchemaDefinition"; // System Facet Info ////// Name of the MaxLength Facet /// internal const string MaxLengthFacetName = "MaxLength"; ////// Name of the Unicode Facet /// internal const string UnicodeFacetName = "Unicode"; ////// Name of the FixedLength Facet /// internal const string FixedLengthFacetName = "FixedLength"; ////// Name of the Precision Facet /// internal const string PrecisionFacetName = "Precision"; ////// Name of the Scale Facet /// internal const string ScaleFacetName = "Scale"; ////// Name of the Nullable Facet /// internal const string NullableFacetName = "Nullable"; ////// Name of the DefaultValue Facet /// internal const string DefaultValueFacetName = "DefaultValue"; ////// Name of the Collation Facet /// internal const string CollationFacetName = "Collation"; ////// Returns the namespace used by this provider manifest /// public abstract string NamespaceName {get;} ////// Return the set of types supported by the store /// ///A collection of primitive types public abstract System.Collections.ObjectModel.ReadOnlyCollectionGetStoreTypes(); /// /// Returns all the edm functions supported by the provider manifest. /// ///A collection of edm functions. public abstract System.Collections.ObjectModel.ReadOnlyCollectionGetStoreFunctions(); /// /// Returns all the FacetDescriptions for a particular type /// /// the type to return FacetDescriptions for ///The FacetDescriptions for the type given [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "edm")] public abstract System.Collections.ObjectModel.ReadOnlyCollectionGetFacetDescriptions(EdmType edmType); /// /// This method allows a provider writer to take a type and a set of facets /// and reason about what the best mapped equivalent type in EDM would be. /// /// A TypeUsage encapsulating a store type and a set of facets ///A TypeUsage encapsulating an EDM type and a set of facets [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] public abstract TypeUsage GetEdmType(TypeUsage storeType); ////// This method allows a provider writer to take a type and a set of facets /// and reason about what the best mapped equivalent type in the store would be. /// /// A TypeUsage encapsulating an EDM type and a set of facets ///A TypeUsage encapsulating a store type and a set of facets [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "edm")] public abstract TypeUsage GetStoreType(TypeUsage edmType); ////// Providers should override this to return information specific to their provider. /// /// This method should never return null. /// /// The name of the information to be retrieved. ///An XmlReader at the begining of the information requested. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] protected abstract XmlReader GetDbInformation(string informationType); ////// Gets framework and provider specific information /// /// This method should never return null. /// /// The name of the information to be retrieved. ///An XmlReader at the begining of the information requested. public XmlReader GetInformation(string informationType) { XmlReader reader = null; try { reader = GetDbInformation(informationType); } catch (Exception e) { // we should not be wrapping all exceptions if (EntityUtil.IsCatchableExceptionType(e)) { // we don't want folks to have to know all the various types of exceptions that can // occur, so we just rethrow a ProviderIncompatibleException and make whatever we caught // the inner exception of it. throw EntityUtil.ProviderIncompatible( System.Data.Entity.Strings.EntityClient_FailedToGetInformation(informationType), e); } throw; } if (reader == null) { // if the provider returned null for the conceptual schema definition, return the default one if (informationType == ConceptualSchemaDefinition) { return DbProviderServices.GetConceptualSchemaDescription(); } throw EntityUtil.ProviderIncompatible(System.Data.Entity.Strings.ProviderReturnedNullForGetDbInformation(informationType)); } return reader; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
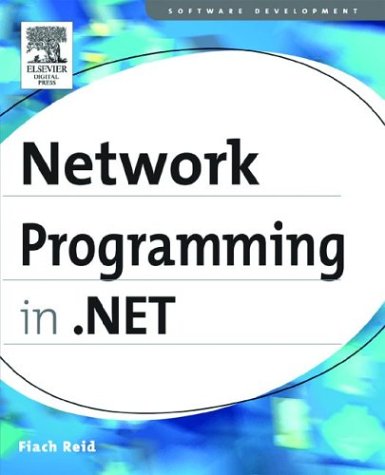
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OdbcCommand.cs
- Menu.cs
- XmlQueryStaticData.cs
- BamlLocalizableResource.cs
- UserUseLicenseDictionaryLoader.cs
- EditorBrowsableAttribute.cs
- SmtpAuthenticationManager.cs
- ViewBox.cs
- AuthorizationSection.cs
- ReferencedAssembly.cs
- XmlCountingReader.cs
- BackgroundWorker.cs
- PngBitmapEncoder.cs
- MetadataItemCollectionFactory.cs
- PermissionListSet.cs
- TextCompositionManager.cs
- TdsEnums.cs
- TextInfo.cs
- Stroke.cs
- TypeConverterBase.cs
- MachineKeySection.cs
- SqlDependencyListener.cs
- FunctionImportElement.cs
- DelegateTypeInfo.cs
- BuildManager.cs
- OpenFileDialog.cs
- ZeroOpNode.cs
- MessageSecurityException.cs
- SchemaSetCompiler.cs
- ModelItemExtensions.cs
- XPathParser.cs
- InteropBitmapSource.cs
- ConstraintConverter.cs
- DSASignatureDeformatter.cs
- RootBuilder.cs
- DataContractSerializerSection.cs
- SerialPinChanges.cs
- PartitionedStreamMerger.cs
- TimeZoneNotFoundException.cs
- TheQuery.cs
- DateTimeConstantAttribute.cs
- DeferredSelectedIndexReference.cs
- EncoderBestFitFallback.cs
- GlobalAllocSafeHandle.cs
- DictionaryItemsCollection.cs
- WarningException.cs
- ConnectionPoint.cs
- RsaSecurityKey.cs
- ApplicationException.cs
- PerformanceCounter.cs
- NonClientArea.cs
- MobileListItemCollection.cs
- PartialCachingControl.cs
- SafeUserTokenHandle.cs
- RNGCryptoServiceProvider.cs
- DataGridViewEditingControlShowingEventArgs.cs
- PageThemeCodeDomTreeGenerator.cs
- WindowPatternIdentifiers.cs
- GraphicsState.cs
- UdpMessageProperty.cs
- SignatureDescription.cs
- StreamWriter.cs
- SubqueryRules.cs
- ZipFileInfo.cs
- StateMachineTimers.cs
- FunctionImportElement.cs
- MenuItemAutomationPeer.cs
- BStrWrapper.cs
- PropertyKey.cs
- PhonemeEventArgs.cs
- StringReader.cs
- EncryptedKey.cs
- Timer.cs
- ServiceAuthorizationManager.cs
- TagPrefixAttribute.cs
- StringUtil.cs
- Frame.cs
- PersonalizablePropertyEntry.cs
- AccessDataSourceView.cs
- UnmanagedBitmapWrapper.cs
- TreeNodeMouseHoverEvent.cs
- LocationUpdates.cs
- Sentence.cs
- CrossSiteScriptingValidation.cs
- TemplateParser.cs
- RuleElement.cs
- WindowsTitleBar.cs
- CollectionViewGroupRoot.cs
- MediaSystem.cs
- HotSpot.cs
- WeakReference.cs
- UndoUnit.cs
- IndentedWriter.cs
- Version.cs
- InternalConfigEventArgs.cs
- ResourceDictionary.cs
- KeyTime.cs
- KnowledgeBase.cs
- DataGridViewTopLeftHeaderCell.cs
- Pkcs9Attribute.cs