Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Security / Cryptography / SignatureDescription.cs / 1305376 / SignatureDescription.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // // // SignatureDescription.cs // namespace System.Security.Cryptography { using System.Security.Util; using System.Diagnostics.Contracts; [System.Runtime.InteropServices.ComVisible(true)] public class SignatureDescription { private String _strKey; private String _strDigest; private String _strFormatter; private String _strDeformatter; // // public constructors // public SignatureDescription() { } public SignatureDescription(SecurityElement el) { if (el == null) throw new ArgumentNullException("el"); Contract.EndContractBlock(); _strKey = el.SearchForTextOfTag("Key"); _strDigest = el.SearchForTextOfTag("Digest"); _strFormatter = el.SearchForTextOfTag("Formatter"); _strDeformatter = el.SearchForTextOfTag("Deformatter"); } // // property methods // public String KeyAlgorithm { get { return _strKey; } set { _strKey = value; } } public String DigestAlgorithm { get { return _strDigest; } set { _strDigest = value; } } public String FormatterAlgorithm { get { return _strFormatter; } set { _strFormatter = value; } } public String DeformatterAlgorithm { get {return _strDeformatter; } set {_strDeformatter = value; } } // // public methods // [System.Security.SecuritySafeCritical] // auto-generated public virtual AsymmetricSignatureDeformatter CreateDeformatter(AsymmetricAlgorithm key) { AsymmetricSignatureDeformatter item; item = (AsymmetricSignatureDeformatter) CryptoConfig.CreateFromName(_strDeformatter); item.SetKey(key); return item; } [System.Security.SecuritySafeCritical] // auto-generated public virtual AsymmetricSignatureFormatter CreateFormatter(AsymmetricAlgorithm key) { AsymmetricSignatureFormatter item; item = (AsymmetricSignatureFormatter) CryptoConfig.CreateFromName(_strFormatter); item.SetKey(key); return item; } [System.Security.SecuritySafeCritical] // auto-generated public virtual HashAlgorithm CreateDigest() { return (HashAlgorithm) CryptoConfig.CreateFromName(_strDigest); } } internal class RSAPKCS1SHA1SignatureDescription : SignatureDescription { public RSAPKCS1SHA1SignatureDescription() { KeyAlgorithm = "System.Security.Cryptography.RSACryptoServiceProvider"; DigestAlgorithm = "System.Security.Cryptography.SHA1CryptoServiceProvider"; FormatterAlgorithm = "System.Security.Cryptography.RSAPKCS1SignatureFormatter"; DeformatterAlgorithm = "System.Security.Cryptography.RSAPKCS1SignatureDeformatter"; } public override AsymmetricSignatureDeformatter CreateDeformatter(AsymmetricAlgorithm key) { AsymmetricSignatureDeformatter item = (AsymmetricSignatureDeformatter) CryptoConfig.CreateFromName(DeformatterAlgorithm); item.SetKey(key); item.SetHashAlgorithm("SHA1"); return item; } } internal class DSASignatureDescription : SignatureDescription { public DSASignatureDescription() { KeyAlgorithm = "System.Security.Cryptography.DSACryptoServiceProvider"; DigestAlgorithm = "System.Security.Cryptography.SHA1CryptoServiceProvider"; FormatterAlgorithm = "System.Security.Cryptography.DSASignatureFormatter"; DeformatterAlgorithm = "System.Security.Cryptography.DSASignatureDeformatter"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // // // SignatureDescription.cs // namespace System.Security.Cryptography { using System.Security.Util; using System.Diagnostics.Contracts; [System.Runtime.InteropServices.ComVisible(true)] public class SignatureDescription { private String _strKey; private String _strDigest; private String _strFormatter; private String _strDeformatter; // // public constructors // public SignatureDescription() { } public SignatureDescription(SecurityElement el) { if (el == null) throw new ArgumentNullException("el"); Contract.EndContractBlock(); _strKey = el.SearchForTextOfTag("Key"); _strDigest = el.SearchForTextOfTag("Digest"); _strFormatter = el.SearchForTextOfTag("Formatter"); _strDeformatter = el.SearchForTextOfTag("Deformatter"); } // // property methods // public String KeyAlgorithm { get { return _strKey; } set { _strKey = value; } } public String DigestAlgorithm { get { return _strDigest; } set { _strDigest = value; } } public String FormatterAlgorithm { get { return _strFormatter; } set { _strFormatter = value; } } public String DeformatterAlgorithm { get {return _strDeformatter; } set {_strDeformatter = value; } } // // public methods // [System.Security.SecuritySafeCritical] // auto-generated public virtual AsymmetricSignatureDeformatter CreateDeformatter(AsymmetricAlgorithm key) { AsymmetricSignatureDeformatter item; item = (AsymmetricSignatureDeformatter) CryptoConfig.CreateFromName(_strDeformatter); item.SetKey(key); return item; } [System.Security.SecuritySafeCritical] // auto-generated public virtual AsymmetricSignatureFormatter CreateFormatter(AsymmetricAlgorithm key) { AsymmetricSignatureFormatter item; item = (AsymmetricSignatureFormatter) CryptoConfig.CreateFromName(_strFormatter); item.SetKey(key); return item; } [System.Security.SecuritySafeCritical] // auto-generated public virtual HashAlgorithm CreateDigest() { return (HashAlgorithm) CryptoConfig.CreateFromName(_strDigest); } } internal class RSAPKCS1SHA1SignatureDescription : SignatureDescription { public RSAPKCS1SHA1SignatureDescription() { KeyAlgorithm = "System.Security.Cryptography.RSACryptoServiceProvider"; DigestAlgorithm = "System.Security.Cryptography.SHA1CryptoServiceProvider"; FormatterAlgorithm = "System.Security.Cryptography.RSAPKCS1SignatureFormatter"; DeformatterAlgorithm = "System.Security.Cryptography.RSAPKCS1SignatureDeformatter"; } public override AsymmetricSignatureDeformatter CreateDeformatter(AsymmetricAlgorithm key) { AsymmetricSignatureDeformatter item = (AsymmetricSignatureDeformatter) CryptoConfig.CreateFromName(DeformatterAlgorithm); item.SetKey(key); item.SetHashAlgorithm("SHA1"); return item; } } internal class DSASignatureDescription : SignatureDescription { public DSASignatureDescription() { KeyAlgorithm = "System.Security.Cryptography.DSACryptoServiceProvider"; DigestAlgorithm = "System.Security.Cryptography.SHA1CryptoServiceProvider"; FormatterAlgorithm = "System.Security.Cryptography.DSASignatureFormatter"; DeformatterAlgorithm = "System.Security.Cryptography.DSASignatureDeformatter"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
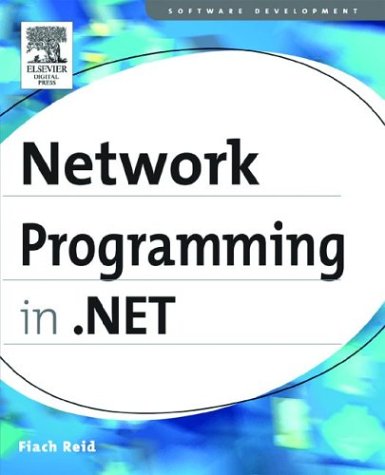
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RelationshipEndMember.cs
- ResourceReader.cs
- OpenTypeLayoutCache.cs
- ToolStripSettings.cs
- ValidatorCompatibilityHelper.cs
- EventListenerClientSide.cs
- SqlBinder.cs
- HwndProxyElementProvider.cs
- PtsHost.cs
- SqlConnectionPoolProviderInfo.cs
- SamlAssertion.cs
- NumericUpDown.cs
- CodeTypeParameter.cs
- SqlDataSourceConfigureFilterForm.cs
- SoapAttributeAttribute.cs
- X509UI.cs
- BooleanStorage.cs
- HttpHandlersInstallComponent.cs
- ProcessModuleCollection.cs
- SafeCoTaskMem.cs
- XmlUtilWriter.cs
- DeclarationUpdate.cs
- DataColumnMapping.cs
- XmlHierarchicalDataSourceView.cs
- TabItemAutomationPeer.cs
- ArgIterator.cs
- RegexFCD.cs
- ElementHostAutomationPeer.cs
- TrustSection.cs
- RegexRunner.cs
- RequiredAttributeAttribute.cs
- DropSource.cs
- Stopwatch.cs
- TraceXPathNavigator.cs
- ExtensionFile.cs
- MetafileHeaderWmf.cs
- Parser.cs
- MetadataProperty.cs
- XmlNamespaceManager.cs
- Delegate.cs
- ButtonChrome.cs
- DataGridViewButtonColumn.cs
- GradientStop.cs
- ListBindingHelper.cs
- SimpleWebHandlerParser.cs
- Win32KeyboardDevice.cs
- DiscriminatorMap.cs
- OpenTypeCommon.cs
- PropertyPathWorker.cs
- ResourceDescriptionAttribute.cs
- DatatypeImplementation.cs
- CdpEqualityComparer.cs
- TypeDescriptionProviderAttribute.cs
- TileModeValidation.cs
- EventDescriptorCollection.cs
- BulletChrome.cs
- BitmapEffect.cs
- DictionaryGlobals.cs
- CurrencyWrapper.cs
- PerformanceCounterPermissionAttribute.cs
- OdbcHandle.cs
- QuaternionAnimationBase.cs
- ResourceDisplayNameAttribute.cs
- HashCodeCombiner.cs
- dataobject.cs
- QilReference.cs
- PasswordBoxAutomationPeer.cs
- EntityViewGenerationConstants.cs
- LinqDataSourceContextEventArgs.cs
- ResourceContainer.cs
- ExpressionList.cs
- ISAPIRuntime.cs
- _SslState.cs
- EnumerableCollectionView.cs
- AppDomainUnloadedException.cs
- ConnectionStringsExpressionBuilder.cs
- TextElementAutomationPeer.cs
- CaseCqlBlock.cs
- PagedControl.cs
- UniqueTransportManagerRegistration.cs
- ContractComponent.cs
- CodeVariableReferenceExpression.cs
- XmlDataSourceView.cs
- BrushValueSerializer.cs
- Vector3DCollectionConverter.cs
- Rectangle.cs
- StylusEventArgs.cs
- CreateUserWizard.cs
- LogicalExpressionTypeConverter.cs
- BufferedReceiveManager.cs
- _NestedSingleAsyncResult.cs
- COM2Properties.cs
- TakeQueryOptionExpression.cs
- handlecollector.cs
- ModelTreeEnumerator.cs
- KoreanCalendar.cs
- ThreadExceptionEvent.cs
- ChangePassword.cs
- OdbcError.cs
- XmlSchemaCompilationSettings.cs