Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Net / System / Net / NetworkInformation / PhysicalAddress.cs / 1305376 / PhysicalAddress.cs
using System; using System.Text; namespace System.Net.NetworkInformation { public class PhysicalAddress { byte[] address = null; bool changed = true; int hash = 0; // FxCop: if this class is ever made mutable (like, given any non-readonly fields), // the readonly should be removed from the None decoration. public static readonly PhysicalAddress None = new PhysicalAddress(new byte[0]); // constructors public PhysicalAddress(byte[] address){ this.address = address; } public override int GetHashCode() { if (changed) { changed = false; hash = 0; int i; int size = address.Length & ~3; for (i = 0; i < size; i += 4) { hash ^= (int)address[i] | ((int)address[i+1] << 8) | ((int)address[i+2] << 16) | ((int)address[i+3] << 24); } if ((address.Length & 3) != 0) { int remnant = 0; int shift = 0; for (; i < address.Length; ++i) { remnant |= ((int)address[i]) << shift; shift += 8; } hash ^= remnant; } } return hash; } public override bool Equals(object comparand) { PhysicalAddress address = comparand as PhysicalAddress; if (address == null) return false; if (this.address.Length != address.address.Length) { return false; } for (int i = 0; i < address.address.Length; i++) { if(this.address[i] != address.address[i]) return false; } return true; } public override string ToString(){ StringBuilder addressString = new StringBuilder(); foreach (byte value in address ) { int tmp = (value >> 4) & 0x0F; for (int i = 0; i<2; i++) { if (tmp < 0x0A ) { addressString.Append((char)(tmp+0x30)); } else{ addressString.Append((char)(tmp+0x37)); } tmp = ((int)value & 0x0F); } } return addressString.ToString(); } public byte[] GetAddressBytes(){ byte[] tmp = new byte[address.Length]; Buffer.BlockCopy(address,0,tmp,0,address.Length); return tmp; } public static PhysicalAddress Parse(string address) { int validCount = 0; bool hasDashes = false; byte[] buffer = null; if(address == null) { return PhysicalAddress.None; } //has dashes? if (address.IndexOf('-') >= 0 ){ hasDashes = true; buffer = new byte[(address.Length+1)/3]; } else{ if(address.Length % 2 > 0){ //should be even throw new FormatException(SR.GetString(SR.net_bad_mac_address)); } buffer = new byte[address.Length/2]; } int j = 0; for (int i = 0; i < address.Length; i++ ) { int value = (int)address[i]; if (value >= 0x30 && value <=0x39){ value -= 0x30; } else if (value >= 0x41 && value <= 0x46) { value -= 0x37; } else if (value == (int)'-'){ if (validCount == 2) { validCount = 0; continue; } else{ throw new FormatException(SR.GetString(SR.net_bad_mac_address)); } } else{ throw new FormatException(SR.GetString(SR.net_bad_mac_address)); } //we had too many characters after the last dash if(hasDashes && validCount >= 2){ throw new FormatException(SR.GetString(SR.net_bad_mac_address)); } if (validCount%2 == 0) { buffer[j] = (byte) (value << 4); } else{ buffer[j++] |= (byte) value; } validCount++; } //we too few characters after the last dash if(validCount < 2){ throw new FormatException(SR.GetString(SR.net_bad_mac_address)); } return new PhysicalAddress(buffer); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Text; namespace System.Net.NetworkInformation { public class PhysicalAddress { byte[] address = null; bool changed = true; int hash = 0; // FxCop: if this class is ever made mutable (like, given any non-readonly fields), // the readonly should be removed from the None decoration. public static readonly PhysicalAddress None = new PhysicalAddress(new byte[0]); // constructors public PhysicalAddress(byte[] address){ this.address = address; } public override int GetHashCode() { if (changed) { changed = false; hash = 0; int i; int size = address.Length & ~3; for (i = 0; i < size; i += 4) { hash ^= (int)address[i] | ((int)address[i+1] << 8) | ((int)address[i+2] << 16) | ((int)address[i+3] << 24); } if ((address.Length & 3) != 0) { int remnant = 0; int shift = 0; for (; i < address.Length; ++i) { remnant |= ((int)address[i]) << shift; shift += 8; } hash ^= remnant; } } return hash; } public override bool Equals(object comparand) { PhysicalAddress address = comparand as PhysicalAddress; if (address == null) return false; if (this.address.Length != address.address.Length) { return false; } for (int i = 0; i < address.address.Length; i++) { if(this.address[i] != address.address[i]) return false; } return true; } public override string ToString(){ StringBuilder addressString = new StringBuilder(); foreach (byte value in address ) { int tmp = (value >> 4) & 0x0F; for (int i = 0; i<2; i++) { if (tmp < 0x0A ) { addressString.Append((char)(tmp+0x30)); } else{ addressString.Append((char)(tmp+0x37)); } tmp = ((int)value & 0x0F); } } return addressString.ToString(); } public byte[] GetAddressBytes(){ byte[] tmp = new byte[address.Length]; Buffer.BlockCopy(address,0,tmp,0,address.Length); return tmp; } public static PhysicalAddress Parse(string address) { int validCount = 0; bool hasDashes = false; byte[] buffer = null; if(address == null) { return PhysicalAddress.None; } //has dashes? if (address.IndexOf('-') >= 0 ){ hasDashes = true; buffer = new byte[(address.Length+1)/3]; } else{ if(address.Length % 2 > 0){ //should be even throw new FormatException(SR.GetString(SR.net_bad_mac_address)); } buffer = new byte[address.Length/2]; } int j = 0; for (int i = 0; i < address.Length; i++ ) { int value = (int)address[i]; if (value >= 0x30 && value <=0x39){ value -= 0x30; } else if (value >= 0x41 && value <= 0x46) { value -= 0x37; } else if (value == (int)'-'){ if (validCount == 2) { validCount = 0; continue; } else{ throw new FormatException(SR.GetString(SR.net_bad_mac_address)); } } else{ throw new FormatException(SR.GetString(SR.net_bad_mac_address)); } //we had too many characters after the last dash if(hasDashes && validCount >= 2){ throw new FormatException(SR.GetString(SR.net_bad_mac_address)); } if (validCount%2 == 0) { buffer[j] = (byte) (value << 4); } else{ buffer[j++] |= (byte) value; } validCount++; } //we too few characters after the last dash if(validCount < 2){ throw new FormatException(SR.GetString(SR.net_bad_mac_address)); } return new PhysicalAddress(buffer); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
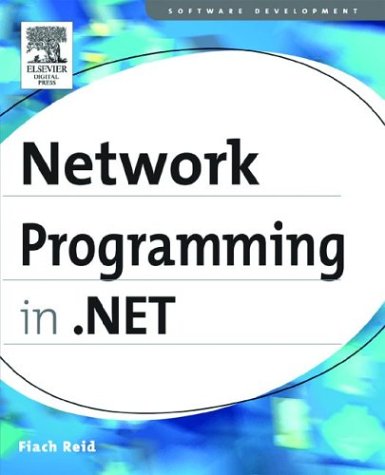
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Random.cs
- SQLBytes.cs
- externdll.cs
- HistoryEventArgs.cs
- WebResourceAttribute.cs
- SoapReflectionImporter.cs
- KerberosRequestorSecurityTokenAuthenticator.cs
- Simplifier.cs
- RectangleHotSpot.cs
- ScopeElement.cs
- PointCollectionValueSerializer.cs
- UriExt.cs
- LinkButton.cs
- ItemContainerGenerator.cs
- Hashtable.cs
- ClientBuildManagerCallback.cs
- NamespaceEmitter.cs
- Mouse.cs
- HitTestFilterBehavior.cs
- ObjectPropertyMapping.cs
- DecoderFallbackWithFailureFlag.cs
- BaseValidator.cs
- DynamicActionMessageFilter.cs
- PersonalizationProvider.cs
- AlignmentXValidation.cs
- StopStoryboard.cs
- AsyncPostBackErrorEventArgs.cs
- TypedTableGenerator.cs
- ADMembershipProvider.cs
- TimeStampChecker.cs
- CodeObject.cs
- ZoneMembershipCondition.cs
- XmlText.cs
- DistinctQueryOperator.cs
- PriorityItem.cs
- NativeMethods.cs
- RestHandlerFactory.cs
- ExceptionHandler.cs
- CompiledIdentityConstraint.cs
- JsonQueryStringConverter.cs
- GlyphCollection.cs
- InputReport.cs
- XmlWellformedWriter.cs
- MemberRelationshipService.cs
- ConfigurationErrorsException.cs
- ColumnMapProcessor.cs
- QuestionEventArgs.cs
- MostlySingletonList.cs
- SharedUtils.cs
- CodeTypeDelegate.cs
- SmiMetaDataProperty.cs
- SamlAttributeStatement.cs
- SerializationUtilities.cs
- InputReport.cs
- VectorAnimationBase.cs
- BitmapSource.cs
- InstanceCollisionException.cs
- BaseInfoTable.cs
- DelayedRegex.cs
- Style.cs
- PartialCachingControl.cs
- IdleTimeoutMonitor.cs
- RepeatBehaviorConverter.cs
- Module.cs
- ScriptControl.cs
- FormViewModeEventArgs.cs
- FileChangesMonitor.cs
- SourceChangedEventArgs.cs
- _IPv4Address.cs
- ClientUrlResolverWrapper.cs
- SapiRecoContext.cs
- FormView.cs
- GridViewSortEventArgs.cs
- ConfigurationManagerHelperFactory.cs
- XmlElementAttribute.cs
- WSSecurityPolicy.cs
- SystemIPv6InterfaceProperties.cs
- CultureSpecificStringDictionary.cs
- HttpContext.cs
- ObjectCache.cs
- SettingsProviderCollection.cs
- PerformanceCounterPermissionAttribute.cs
- ZoneIdentityPermission.cs
- ContourSegment.cs
- XPathNodeInfoAtom.cs
- ServiceModelConfigurationSectionGroup.cs
- PngBitmapEncoder.cs
- LambdaCompiler.Logical.cs
- XPathCompileException.cs
- BitmapVisualManager.cs
- UserControlFileEditor.cs
- OdbcDataAdapter.cs
- BooleanAnimationUsingKeyFrames.cs
- ByteStreamGeometryContext.cs
- WorkflowOwnerAsyncResult.cs
- ThreadExceptionDialog.cs
- HttpCacheParams.cs
- XmlParser.cs
- SendKeys.cs
- SinglePageViewer.cs