Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / System / ComponentModel / SortDescription.cs / 1305600 / SortDescription.cs
//---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: Defines property and direction to sort. // // See spec at http://avalon/connecteddata/M5%20Specs/IDataCollection.mht // // History: // 06/02/2003 : [....] - Created // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Windows; // SR using MS.Internal.WindowsBase; namespace System.ComponentModel { ////// Defines a property and direction to sort a list by. /// public struct SortDescription { //----------------------------------------------------- // // Public Constructors // //----------------------------------------------------- #region Public Constructors ////// Create a sort description. /// /// Property to sort by /// Specifies the direction of sort operation ///direction is not a valid value for ListSortDirection public SortDescription(string propertyName, ListSortDirection direction) { if (direction != ListSortDirection.Ascending && direction != ListSortDirection.Descending) throw new InvalidEnumArgumentException("direction", (int)direction, typeof(ListSortDirection)); _propertyName = propertyName; _direction = direction; _sealed = false; } #endregion Public Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// Property name to sort by. /// public string PropertyName { get { return _propertyName; } set { if (_sealed) throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "SortDescription")); _propertyName = value; } } ////// Sort direction. /// public ListSortDirection Direction { get { return _direction; } set { if (_sealed) throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "SortDescription")); if (value < ListSortDirection.Ascending || value > ListSortDirection.Descending) throw new InvalidEnumArgumentException("value", (int) value, typeof(ListSortDirection)); _direction = value; } } ////// Returns true if the SortDescription is in use (sealed). /// public bool IsSealed { get { return _sealed; } } #endregion Public Properties //------------------------------------------------------ // // Public methods // //------------------------------------------------------ #region Public Methods ///Override of Object.Equals public override bool Equals(object obj) { return (obj is SortDescription) ? (this == (SortDescription)obj) : false; } ///Equality operator for SortDescription. public static bool operator==(SortDescription sd1, SortDescription sd2) { return sd1.PropertyName == sd2.PropertyName && sd1.Direction == sd2.Direction; } ///Inequality operator for SortDescription. public static bool operator!=(SortDescription sd1, SortDescription sd2) { return !(sd1 == sd2); } ///Override of Object.GetHashCode public override int GetHashCode() { int result = Direction.GetHashCode(); if (PropertyName != null) { result = unchecked(PropertyName.GetHashCode() + result); } return result; } #endregion Public Methods //----------------------------------------------------- // // Internal methods // //------------------------------------------------------ #region Internal Methods internal void Seal() { _sealed = true; } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private string _propertyName; private ListSortDirection _direction; bool _sealed; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: Defines property and direction to sort. // // See spec at http://avalon/connecteddata/M5%20Specs/IDataCollection.mht // // History: // 06/02/2003 : [....] - Created // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Windows; // SR using MS.Internal.WindowsBase; namespace System.ComponentModel { ////// Defines a property and direction to sort a list by. /// public struct SortDescription { //----------------------------------------------------- // // Public Constructors // //----------------------------------------------------- #region Public Constructors ////// Create a sort description. /// /// Property to sort by /// Specifies the direction of sort operation ///direction is not a valid value for ListSortDirection public SortDescription(string propertyName, ListSortDirection direction) { if (direction != ListSortDirection.Ascending && direction != ListSortDirection.Descending) throw new InvalidEnumArgumentException("direction", (int)direction, typeof(ListSortDirection)); _propertyName = propertyName; _direction = direction; _sealed = false; } #endregion Public Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// Property name to sort by. /// public string PropertyName { get { return _propertyName; } set { if (_sealed) throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "SortDescription")); _propertyName = value; } } ////// Sort direction. /// public ListSortDirection Direction { get { return _direction; } set { if (_sealed) throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "SortDescription")); if (value < ListSortDirection.Ascending || value > ListSortDirection.Descending) throw new InvalidEnumArgumentException("value", (int) value, typeof(ListSortDirection)); _direction = value; } } ////// Returns true if the SortDescription is in use (sealed). /// public bool IsSealed { get { return _sealed; } } #endregion Public Properties //------------------------------------------------------ // // Public methods // //------------------------------------------------------ #region Public Methods ///Override of Object.Equals public override bool Equals(object obj) { return (obj is SortDescription) ? (this == (SortDescription)obj) : false; } ///Equality operator for SortDescription. public static bool operator==(SortDescription sd1, SortDescription sd2) { return sd1.PropertyName == sd2.PropertyName && sd1.Direction == sd2.Direction; } ///Inequality operator for SortDescription. public static bool operator!=(SortDescription sd1, SortDescription sd2) { return !(sd1 == sd2); } ///Override of Object.GetHashCode public override int GetHashCode() { int result = Direction.GetHashCode(); if (PropertyName != null) { result = unchecked(PropertyName.GetHashCode() + result); } return result; } #endregion Public Methods //----------------------------------------------------- // // Internal methods // //------------------------------------------------------ #region Internal Methods internal void Seal() { _sealed = true; } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private string _propertyName; private ListSortDirection _direction; bool _sealed; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
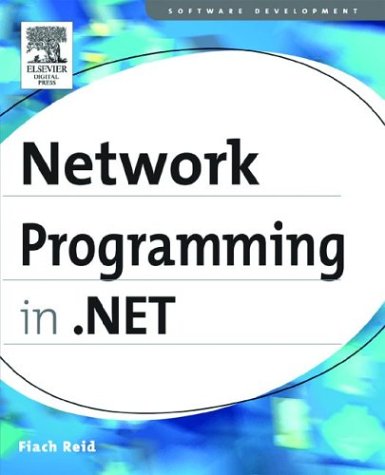
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectListFieldsPage.cs
- XmlStreamStore.cs
- Setter.cs
- StatusBarPanel.cs
- FontEmbeddingManager.cs
- RelatedPropertyManager.cs
- SmiGettersStream.cs
- StateMachine.cs
- FlowDocument.cs
- BuildDependencySet.cs
- DataGridViewRowCollection.cs
- DataTableClearEvent.cs
- QuadraticBezierSegment.cs
- WindowsIPAddress.cs
- WebPartActionVerb.cs
- SecurityUtils.cs
- PriorityQueue.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- InputScope.cs
- FaultContractAttribute.cs
- ScriptResourceHandler.cs
- XMLUtil.cs
- DataIdProcessor.cs
- MsmqChannelFactory.cs
- LoginViewDesigner.cs
- CommandManager.cs
- SspiSecurityTokenProvider.cs
- SessionStateItemCollection.cs
- TextRenderer.cs
- PositiveTimeSpanValidatorAttribute.cs
- QilGeneratorEnv.cs
- ScriptControlDescriptor.cs
- ReadOnlyObservableCollection.cs
- MemoryFailPoint.cs
- SymLanguageVendor.cs
- HttpHeaderCollection.cs
- IndentedTextWriter.cs
- SchemaCompiler.cs
- IsolatedStorageFilePermission.cs
- LinkTarget.cs
- ConnectionStringSettings.cs
- GlyphTypeface.cs
- ReadOnlyHierarchicalDataSourceView.cs
- XmlSchemaObject.cs
- NavigationCommands.cs
- MapPathBasedVirtualPathProvider.cs
- AliasExpr.cs
- X500Name.cs
- ToolStripDropTargetManager.cs
- SqlCacheDependencyDatabaseCollection.cs
- DialogResultConverter.cs
- ManagementClass.cs
- metadatamappinghashervisitor.hashsourcebuilder.cs
- RootBrowserWindowProxy.cs
- MultilineStringConverter.cs
- GlyphElement.cs
- EmptyEnumerable.cs
- EmptyEnumerable.cs
- MexHttpBindingElement.cs
- WizardPanelChangingEventArgs.cs
- EntityClassGenerator.cs
- AggregateNode.cs
- Triangle.cs
- MethodBuilder.cs
- Control.cs
- DataColumnSelectionConverter.cs
- LocalClientSecuritySettings.cs
- DataGridViewAccessibleObject.cs
- MembershipSection.cs
- Section.cs
- WinInet.cs
- SchemaEntity.cs
- SafeProcessHandle.cs
- BaseUriHelper.cs
- InputScopeManager.cs
- EarlyBoundInfo.cs
- VerificationException.cs
- TemplateInstanceAttribute.cs
- Visual.cs
- Base64Encoding.cs
- X509ChainElement.cs
- PublisherIdentityPermission.cs
- TypeToTreeConverter.cs
- HttpConfigurationContext.cs
- BooleanSwitch.cs
- ApplicationBuildProvider.cs
- FragmentQuery.cs
- CodeTryCatchFinallyStatement.cs
- TransformerInfo.cs
- XmlLanguageConverter.cs
- SafeLocalMemHandle.cs
- WasHttpModulesInstallComponent.cs
- EntityStoreSchemaGenerator.cs
- UnicastIPAddressInformationCollection.cs
- ExceptionHelpers.cs
- XdrBuilder.cs
- ScaleTransform3D.cs
- ValidatingReaderNodeData.cs
- MDIWindowDialog.cs
- OverflowException.cs