Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Common / Shared / DelegateTypeInfo.cs / 1305376 / DelegateTypeInfo.cs
// Copyright (c) Microsoft Corporation. All rights reserved. // // THIS CODE AND INFORMATION IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, // WHETHER EXPRESSED OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE IMPLIED // WARRANTIES OF MERCHANTABILITY AND/OR FITNESS FOR A PARTICULAR PURPOSE. // THE ENTIRE RISK OF USE OR RESULTS IN CONNECTION WITH THE USE OF THIS CODE // AND INFORMATION REMAINS WITH THE USER. /********************************************************************** * NOTE: A copy of this file exists at: WF\Activities\Common * The two files must be kept in [....]. Any change made here must also * be made to WF\Activities\Common\DelegateTypeInfo.cs *********************************************************************/ namespace System.Workflow.ComponentModel { using System; using System.CodeDom; using System.Collections; using System.Globalization; using System.ComponentModel; using System.ComponentModel.Design; using System.Reflection; using System.Diagnostics.CodeAnalysis; internal class DelegateTypeInfo { private CodeParameterDeclarationExpression[] parameters; private Type[] parameterTypes; private CodeTypeReference returnType; internal CodeParameterDeclarationExpression[] Parameters { get { return parameters; } } internal Type[] ParameterTypes { get { return parameterTypes; } } internal CodeTypeReference ReturnType { get { return returnType; } } internal DelegateTypeInfo(Type delegateClass) { Resolve(delegateClass); } [SuppressMessage("Microsoft.Globalization", "CA1307:SpecifyStringComparison", Justification = "EndsWith(\"&\") not a security issue.")] private void Resolve(Type delegateClass) { MethodInfo invokeMethod = delegateClass.GetMethod("Invoke"); if (invokeMethod == null) throw new ArgumentException("delegateClass"); Resolve(invokeMethod); } [SuppressMessage("Microsoft.Globalization", "CA1307:SpecifyStringComparison", Justification = "EndsWith(\"&\") not a security issue.")] private void Resolve(MethodInfo method) { // Here we build up an array of argument types, separated // by commas. ParameterInfo[] argTypes = method.GetParameters(); parameters = new CodeParameterDeclarationExpression[argTypes.Length]; parameterTypes = new Type[argTypes.Length]; for (int index = 0; index < argTypes.Length; index++) { string paramName = argTypes[index].Name; Type paramType = argTypes[index].ParameterType; if (paramName == null || paramName.Length == 0) paramName = "param" + index.ToString(CultureInfo.InvariantCulture); FieldDirection fieldDir = FieldDirection.In; // check for the '&' that means ref (gotta love it!) // and we need to strip that & before we continue. Ouch. if (paramType.IsByRef) { if (paramType.FullName.EndsWith("&")) { // strip the & and reload the type without it. paramType = paramType.Assembly.GetType(paramType.FullName.Substring(0, paramType.FullName.Length - 1), true); } fieldDir = FieldDirection.Ref; } if (argTypes[index].IsOut) { if (argTypes[index].IsIn) fieldDir = FieldDirection.Ref; else fieldDir = FieldDirection.Out; } parameters[index] = new CodeParameterDeclarationExpression(new CodeTypeReference(paramType), paramName); parameters[index].Direction = fieldDir; parameterTypes[index] = paramType; } this.returnType = new CodeTypeReference(method.ReturnType); } public override bool Equals(object other) { if (other == null) return false; DelegateTypeInfo dtiOther = other as DelegateTypeInfo; if (dtiOther == null) return false; if (ReturnType.BaseType != dtiOther.ReturnType.BaseType || Parameters.Length != dtiOther.Parameters.Length) return false; for (int parameter = 0; parameter < Parameters.Length; parameter++) { CodeParameterDeclarationExpression otherParam = dtiOther.Parameters[parameter]; if (otherParam.Type.BaseType != Parameters[parameter].Type.BaseType) return false; } return true; } public override int GetHashCode() { return base.GetHashCode(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. // // THIS CODE AND INFORMATION IS PROVIDED "AS IS" WITHOUT WARRANTY OF ANY KIND, // WHETHER EXPRESSED OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE IMPLIED // WARRANTIES OF MERCHANTABILITY AND/OR FITNESS FOR A PARTICULAR PURPOSE. // THE ENTIRE RISK OF USE OR RESULTS IN CONNECTION WITH THE USE OF THIS CODE // AND INFORMATION REMAINS WITH THE USER. /********************************************************************** * NOTE: A copy of this file exists at: WF\Activities\Common * The two files must be kept in [....]. Any change made here must also * be made to WF\Activities\Common\DelegateTypeInfo.cs *********************************************************************/ namespace System.Workflow.ComponentModel { using System; using System.CodeDom; using System.Collections; using System.Globalization; using System.ComponentModel; using System.ComponentModel.Design; using System.Reflection; using System.Diagnostics.CodeAnalysis; internal class DelegateTypeInfo { private CodeParameterDeclarationExpression[] parameters; private Type[] parameterTypes; private CodeTypeReference returnType; internal CodeParameterDeclarationExpression[] Parameters { get { return parameters; } } internal Type[] ParameterTypes { get { return parameterTypes; } } internal CodeTypeReference ReturnType { get { return returnType; } } internal DelegateTypeInfo(Type delegateClass) { Resolve(delegateClass); } [SuppressMessage("Microsoft.Globalization", "CA1307:SpecifyStringComparison", Justification = "EndsWith(\"&\") not a security issue.")] private void Resolve(Type delegateClass) { MethodInfo invokeMethod = delegateClass.GetMethod("Invoke"); if (invokeMethod == null) throw new ArgumentException("delegateClass"); Resolve(invokeMethod); } [SuppressMessage("Microsoft.Globalization", "CA1307:SpecifyStringComparison", Justification = "EndsWith(\"&\") not a security issue.")] private void Resolve(MethodInfo method) { // Here we build up an array of argument types, separated // by commas. ParameterInfo[] argTypes = method.GetParameters(); parameters = new CodeParameterDeclarationExpression[argTypes.Length]; parameterTypes = new Type[argTypes.Length]; for (int index = 0; index < argTypes.Length; index++) { string paramName = argTypes[index].Name; Type paramType = argTypes[index].ParameterType; if (paramName == null || paramName.Length == 0) paramName = "param" + index.ToString(CultureInfo.InvariantCulture); FieldDirection fieldDir = FieldDirection.In; // check for the '&' that means ref (gotta love it!) // and we need to strip that & before we continue. Ouch. if (paramType.IsByRef) { if (paramType.FullName.EndsWith("&")) { // strip the & and reload the type without it. paramType = paramType.Assembly.GetType(paramType.FullName.Substring(0, paramType.FullName.Length - 1), true); } fieldDir = FieldDirection.Ref; } if (argTypes[index].IsOut) { if (argTypes[index].IsIn) fieldDir = FieldDirection.Ref; else fieldDir = FieldDirection.Out; } parameters[index] = new CodeParameterDeclarationExpression(new CodeTypeReference(paramType), paramName); parameters[index].Direction = fieldDir; parameterTypes[index] = paramType; } this.returnType = new CodeTypeReference(method.ReturnType); } public override bool Equals(object other) { if (other == null) return false; DelegateTypeInfo dtiOther = other as DelegateTypeInfo; if (dtiOther == null) return false; if (ReturnType.BaseType != dtiOther.ReturnType.BaseType || Parameters.Length != dtiOther.Parameters.Length) return false; for (int parameter = 0; parameter < Parameters.Length; parameter++) { CodeParameterDeclarationExpression otherParam = dtiOther.Parameters[parameter]; if (otherParam.Type.BaseType != Parameters[parameter].Type.BaseType) return false; } return true; } public override int GetHashCode() { return base.GetHashCode(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
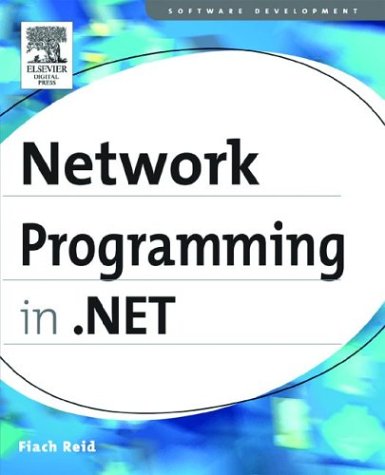
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StylusPointPropertyInfoDefaults.cs
- BamlResourceContent.cs
- HScrollProperties.cs
- MemberInfoSerializationHolder.cs
- HtmlProps.cs
- MemberPath.cs
- TextSearch.cs
- DataAdapter.cs
- TemplateField.cs
- AttachedPropertyDescriptor.cs
- FontResourceCache.cs
- TemplateBaseAction.cs
- TextOnlyOutput.cs
- UIElement.cs
- LocalizationParserHooks.cs
- XsdSchemaFileEditor.cs
- Utility.cs
- UTF32Encoding.cs
- ConfigurationManagerHelper.cs
- TraceFilter.cs
- Point3DAnimationBase.cs
- RtfControlWordInfo.cs
- ClientFactory.cs
- PoisonMessageException.cs
- Int32CAMarshaler.cs
- InputLangChangeEvent.cs
- CacheModeConverter.cs
- ObjectHelper.cs
- PlanCompiler.cs
- XsdBuilder.cs
- ConnectionManagementElement.cs
- XmlObjectSerializerContext.cs
- SHA384Managed.cs
- PersonalizationEntry.cs
- PreProcessor.cs
- WebPartMinimizeVerb.cs
- XmlDownloadManager.cs
- SafeRightsManagementQueryHandle.cs
- Trace.cs
- WindowsListViewItemCheckBox.cs
- ListItemParagraph.cs
- HScrollBar.cs
- ItemDragEvent.cs
- LicenseProviderAttribute.cs
- Button.cs
- Debug.cs
- ListenerChannelContext.cs
- XmlSchemaFacet.cs
- ButtonBaseAutomationPeer.cs
- FixedFindEngine.cs
- LazyTextWriterCreator.cs
- PermissionListSet.cs
- SamlSubjectStatement.cs
- TextBoxView.cs
- AsnEncodedData.cs
- Semaphore.cs
- RoleManagerModule.cs
- SchemaTableColumn.cs
- StackSpiller.cs
- BindingNavigator.cs
- DependencyPropertyValueSerializer.cs
- EdmProviderManifest.cs
- SettingsBase.cs
- Comparer.cs
- X509Certificate2Collection.cs
- Error.cs
- ColumnResizeUndoUnit.cs
- EncodingNLS.cs
- CompositeFontFamily.cs
- ContourSegment.cs
- BaseServiceProvider.cs
- SQLConvert.cs
- StaticTextPointer.cs
- OleDbConnectionInternal.cs
- Oci.cs
- EdmToObjectNamespaceMap.cs
- FixedSOMSemanticBox.cs
- EntityStoreSchemaFilterEntry.cs
- Point4D.cs
- FixedDocumentSequencePaginator.cs
- AsnEncodedData.cs
- AudioFileOut.cs
- DescendantOverDescendantQuery.cs
- TextEffectCollection.cs
- SafeCertificateContext.cs
- TextHidden.cs
- DesignBindingPropertyDescriptor.cs
- KeyConstraint.cs
- UInt64Storage.cs
- ServerValidateEventArgs.cs
- DataGridViewSelectedRowCollection.cs
- CollectionViewProxy.cs
- CharStorage.cs
- SemanticKeyElement.cs
- DelayedRegex.cs
- CollectionBuilder.cs
- InfoCardRSAOAEPKeyExchangeFormatter.cs
- PingOptions.cs
- ColorConvertedBitmap.cs
- DrawingCollection.cs