Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / Protocol / PerformanceCounters.cs / 1 / PerformanceCounters.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // This file contains wrappers for all WS-AT performance counters using System; using System.ServiceModel.Channels; using System.ComponentModel; using System.Diagnostics; using Microsoft.Transactions.Wsat.Messaging; using DiagnosticUtility = Microsoft.Transactions.Bridge.DiagnosticUtility; namespace Microsoft.Transactions.Wsat.Protocol { class PerformanceCounterWrapper { PerformanceCounter counter; public PerformanceCounterWrapper(string counterName) { string categoryName = PerformanceCounterStrings.MSDTC_BRIDGE.TransactionBridgeV1PerfCounters; Exception exception; try { // We reset each performance counter on startup. If we don't do this, our // performance counters aren't reset properly when shutdown is not graceful // // This is related to the following Whidbey DCR: // BugID: 30673 PerformanceCounter: // Allocated memory for the counter's instances are not deallocated and this leads to OOM condition. this.counter = new PerformanceCounter (categoryName, counterName, string.Empty, false); this.counter.RemoveInstance(); this.counter = new PerformanceCounter (categoryName, counterName, string.Empty, false); exception = null; } catch (InvalidOperationException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); exception = e; } catch (Win32Exception e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Warning); exception = e; } if (exception != null) { if (DebugTrace.Error) { DebugTrace.Trace ( TraceLevel.Error, "Unable to initialize performance counter {0}: {1}", counterName, exception ); } PerformanceCounterInitializationFailureRecord.TraceAndLog( PluggableProtocol10.ProtocolGuid, // the counter used by WSAT1.0 is also used by WSAT1.1; for internal compatibility, we trace only the 1.0 guid though counterName, exception ); } } public long Increment() { if (this.counter != null) return this.counter.Increment(); return 0; } public long IncrementBy (long value) { if (this.counter != null) return this.counter.IncrementBy (value); return 0; } } class PerformanceCounterHolder { PerformanceCounterWrapper messageSendFailureCountPerInterval = new PerformanceCounterWrapper (PerformanceCounterStrings.MSDTC_BRIDGE.MessageSendFailureCountPerInterval); public PerformanceCounterWrapper MessageSendFailureCountPerInterval { get { return this.messageSendFailureCountPerInterval; } } PerformanceCounterWrapper prepareRetryCountPerInterval = new PerformanceCounterWrapper(PerformanceCounterStrings.MSDTC_BRIDGE.PrepareRetryCountPerInterval); public PerformanceCounterWrapper PrepareRetryCountPerInterval { get { return this.prepareRetryCountPerInterval; } } PerformanceCounterWrapper commitRetryCountPerInterval = new PerformanceCounterWrapper(PerformanceCounterStrings.MSDTC_BRIDGE.CommitRetryCountPerInterval); public PerformanceCounterWrapper CommitRetryCountPerInterval { get { return this.commitRetryCountPerInterval; } } PerformanceCounterWrapper preparedRetryCountPerInterval = new PerformanceCounterWrapper(PerformanceCounterStrings.MSDTC_BRIDGE.PreparedRetryCountPerInterval); public PerformanceCounterWrapper PreparedRetryCountPerInterval { get { return this.preparedRetryCountPerInterval; } } PerformanceCounterWrapper replayRetryCountPerInterval = new PerformanceCounterWrapper(PerformanceCounterStrings.MSDTC_BRIDGE.ReplayRetryCountPerInterval); public PerformanceCounterWrapper ReplayRetryCountPerInterval { get { return this.replayRetryCountPerInterval; } } PerformanceCounterWrapper faultsReceivedCountPerInterval = new PerformanceCounterWrapper(PerformanceCounterStrings.MSDTC_BRIDGE.FaultsReceivedCountPerInterval); public PerformanceCounterWrapper FaultsReceivedCountPerInterval { get { return this.faultsReceivedCountPerInterval; } } PerformanceCounterWrapper faultsSentCountPerInterval = new PerformanceCounterWrapper(PerformanceCounterStrings.MSDTC_BRIDGE.FaultsSentCountPerInterval); public PerformanceCounterWrapper FaultsSentCountPerInterval { get { return this.faultsSentCountPerInterval; } } PerformanceCounterWrapper averageParticipantPrepareResponseTime = new PerformanceCounterWrapper(PerformanceCounterStrings.MSDTC_BRIDGE.AverageParticipantPrepareResponseTime); public PerformanceCounterWrapper AverageParticipantPrepareResponseTime { get { return this.averageParticipantPrepareResponseTime; } } PerformanceCounterWrapper averageParticipantPrepareResponseTimeBase = new PerformanceCounterWrapper(PerformanceCounterStrings.MSDTC_BRIDGE.AverageParticipantPrepareResponseTimeBase); public PerformanceCounterWrapper AverageParticipantPrepareResponseTimeBase { get { return this.averageParticipantPrepareResponseTimeBase; } } PerformanceCounterWrapper averageParticipantCommitResponseTime = new PerformanceCounterWrapper(PerformanceCounterStrings.MSDTC_BRIDGE.AverageParticipantCommitResponseTime); public PerformanceCounterWrapper AverageParticipantCommitResponseTime { get { return this.averageParticipantCommitResponseTime; } } PerformanceCounterWrapper averageParticipantCommitResponseTimeBase = new PerformanceCounterWrapper(PerformanceCounterStrings.MSDTC_BRIDGE.AverageParticipantCommitResponseTimeBase); public PerformanceCounterWrapper AverageParticipantCommitResponseTimeBase { get { return this.averageParticipantCommitResponseTimeBase; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
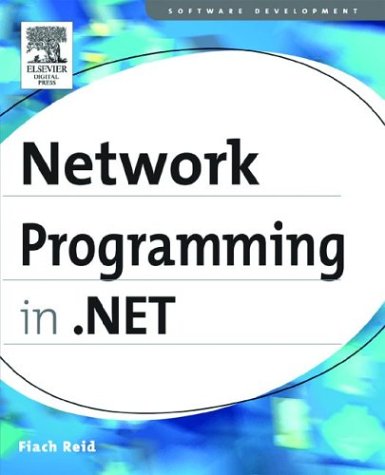
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IUnknownConstantAttribute.cs
- CommandHelper.cs
- DrawTreeNodeEventArgs.cs
- regiisutil.cs
- CFStream.cs
- IConvertible.cs
- OperandQuery.cs
- ConstraintConverter.cs
- FacetDescription.cs
- MethodToken.cs
- XmlWrappingWriter.cs
- StringDictionaryEditor.cs
- ToolStripOverflowButton.cs
- ListChangedEventArgs.cs
- DSASignatureFormatter.cs
- TextEditorThreadLocalStore.cs
- OpacityConverter.cs
- FontFamily.cs
- FixedPageProcessor.cs
- SettingsPropertyIsReadOnlyException.cs
- MenuAutomationPeer.cs
- QueryTask.cs
- FormViewInsertedEventArgs.cs
- DoubleAnimationBase.cs
- Block.cs
- Zone.cs
- SafeWaitHandle.cs
- DescendantBaseQuery.cs
- DesignTimeVisibleAttribute.cs
- ClientSettingsSection.cs
- ClientTargetSection.cs
- RtfNavigator.cs
- ExceptionValidationRule.cs
- SslStream.cs
- TypeLoadException.cs
- Window.cs
- DataBindingCollectionConverter.cs
- TraceContextRecord.cs
- IndependentAnimationStorage.cs
- UserControlFileEditor.cs
- GenericTypeParameterBuilder.cs
- DrawToolTipEventArgs.cs
- MulticastNotSupportedException.cs
- RuleProcessor.cs
- TableLayoutPanelBehavior.cs
- TreeNodeStyleCollection.cs
- BitmapEffectState.cs
- OracleLob.cs
- ColumnMapVisitor.cs
- InvalidWMPVersionException.cs
- RenderDataDrawingContext.cs
- SoapAttributeAttribute.cs
- SafeRightsManagementSessionHandle.cs
- HttpContext.cs
- oledbmetadatacollectionnames.cs
- PageOrientation.cs
- GestureRecognitionResult.cs
- FocusManager.cs
- HtmlElementCollection.cs
- CodeTypeMemberCollection.cs
- ListMarkerSourceInfo.cs
- DataViewManager.cs
- BindingList.cs
- SystemInformation.cs
- SynchronizationScope.cs
- SerializationAttributes.cs
- DataGridTextBox.cs
- ChannelServices.cs
- AncillaryOps.cs
- ConsoleKeyInfo.cs
- EnumCodeDomSerializer.cs
- UnmanagedMemoryStream.cs
- CommandConverter.cs
- CodeTypeDeclaration.cs
- MaterialGroup.cs
- Mutex.cs
- EntityContainerEntitySet.cs
- Assembly.cs
- PhysicalAddress.cs
- StateItem.cs
- SqlTransaction.cs
- SoundPlayerAction.cs
- ObjectParameter.cs
- HttpListenerPrefixCollection.cs
- EndPoint.cs
- Help.cs
- WebZone.cs
- XmlComplianceUtil.cs
- InputLanguageSource.cs
- BezierSegment.cs
- WorkflowServiceInstance.cs
- RelationshipFixer.cs
- DataGridItemCollection.cs
- OverrideMode.cs
- AutoGeneratedFieldProperties.cs
- MenuCommands.cs
- TdsParser.cs
- DataSourceHelper.cs
- BulletedList.cs
- BitmapFrameDecode.cs