Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / ListViewInsertionMark.cs / 1 / ListViewInsertionMark.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Drawing; using System.Runtime.InteropServices; using System.Diagnostics; namespace System.Windows.Forms { ////// /// public sealed class ListViewInsertionMark { private ListView listView; private int index = 0; private Color color = Color.Empty; private bool appearsAfterItem = false; internal ListViewInsertionMark(ListView listView) { this.listView = listView; } ////// Encapsulates insertion-mark information /// ////// /// Specifies whether the insertion mark appears /// after the item - otherwise it appears /// before the item (the default). /// /// public bool AppearsAfterItem { get { return appearsAfterItem; } set { if (appearsAfterItem != value) { appearsAfterItem = value; if (listView.IsHandleCreated) { UpdateListView(); } } } } ////// /// Returns bounds of the insertion-mark. /// /// public Rectangle Bounds { get { NativeMethods.RECT rect = new NativeMethods.RECT(); listView.SendMessage(NativeMethods.LVM_GETINSERTMARKRECT, 0, ref rect); return Rectangle.FromLTRB(rect.left, rect.top, rect.right, rect.bottom); } } ////// /// The color of the insertion-mark. /// /// public Color Color { get { if (color.IsEmpty) { color = SafeNativeMethods.ColorFromCOLORREF((int)listView.SendMessage(NativeMethods.LVM_GETINSERTMARKCOLOR, 0, 0)); } return color; } set { if (color != value) { color = value; if (listView.IsHandleCreated) { listView.SendMessage(NativeMethods.LVM_SETINSERTMARKCOLOR, 0, SafeNativeMethods.ColorToCOLORREF(color)); } } } } ////// /// Item next to which the insertion-mark appears. /// /// public int Index { get { return index; } set { if (index != value) { index = value; if (listView.IsHandleCreated) { UpdateListView(); } } } } ////// /// Performs a hit-test at the specified insertion point /// and returns the closest item. /// /// public int NearestIndex(Point pt) { NativeMethods.POINT point = new NativeMethods.POINT(); point.x = pt.X; point.y = pt.Y; NativeMethods.LVINSERTMARK lvInsertMark = new NativeMethods.LVINSERTMARK(); UnsafeNativeMethods.SendMessage(new HandleRef(listView, listView.Handle), NativeMethods.LVM_INSERTMARKHITTEST, point, lvInsertMark); return lvInsertMark.iItem; } internal void UpdateListView() { Debug.Assert(listView.IsHandleCreated, "ApplySavedState Precondition: List-view handle must be created"); NativeMethods.LVINSERTMARK lvInsertMark = new NativeMethods.LVINSERTMARK(); lvInsertMark.dwFlags = appearsAfterItem ? NativeMethods.LVIM_AFTER : 0; lvInsertMark.iItem = index; UnsafeNativeMethods.SendMessage(new HandleRef(listView, listView.Handle), NativeMethods.LVM_SETINSERTMARK, 0, lvInsertMark); if (!color.IsEmpty) { listView.SendMessage(NativeMethods.LVM_SETINSERTMARKCOLOR, 0, SafeNativeMethods.ColorToCOLORREF(color)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Drawing; using System.Runtime.InteropServices; using System.Diagnostics; namespace System.Windows.Forms { ////// /// public sealed class ListViewInsertionMark { private ListView listView; private int index = 0; private Color color = Color.Empty; private bool appearsAfterItem = false; internal ListViewInsertionMark(ListView listView) { this.listView = listView; } ////// Encapsulates insertion-mark information /// ////// /// Specifies whether the insertion mark appears /// after the item - otherwise it appears /// before the item (the default). /// /// public bool AppearsAfterItem { get { return appearsAfterItem; } set { if (appearsAfterItem != value) { appearsAfterItem = value; if (listView.IsHandleCreated) { UpdateListView(); } } } } ////// /// Returns bounds of the insertion-mark. /// /// public Rectangle Bounds { get { NativeMethods.RECT rect = new NativeMethods.RECT(); listView.SendMessage(NativeMethods.LVM_GETINSERTMARKRECT, 0, ref rect); return Rectangle.FromLTRB(rect.left, rect.top, rect.right, rect.bottom); } } ////// /// The color of the insertion-mark. /// /// public Color Color { get { if (color.IsEmpty) { color = SafeNativeMethods.ColorFromCOLORREF((int)listView.SendMessage(NativeMethods.LVM_GETINSERTMARKCOLOR, 0, 0)); } return color; } set { if (color != value) { color = value; if (listView.IsHandleCreated) { listView.SendMessage(NativeMethods.LVM_SETINSERTMARKCOLOR, 0, SafeNativeMethods.ColorToCOLORREF(color)); } } } } ////// /// Item next to which the insertion-mark appears. /// /// public int Index { get { return index; } set { if (index != value) { index = value; if (listView.IsHandleCreated) { UpdateListView(); } } } } ////// /// Performs a hit-test at the specified insertion point /// and returns the closest item. /// /// public int NearestIndex(Point pt) { NativeMethods.POINT point = new NativeMethods.POINT(); point.x = pt.X; point.y = pt.Y; NativeMethods.LVINSERTMARK lvInsertMark = new NativeMethods.LVINSERTMARK(); UnsafeNativeMethods.SendMessage(new HandleRef(listView, listView.Handle), NativeMethods.LVM_INSERTMARKHITTEST, point, lvInsertMark); return lvInsertMark.iItem; } internal void UpdateListView() { Debug.Assert(listView.IsHandleCreated, "ApplySavedState Precondition: List-view handle must be created"); NativeMethods.LVINSERTMARK lvInsertMark = new NativeMethods.LVINSERTMARK(); lvInsertMark.dwFlags = appearsAfterItem ? NativeMethods.LVIM_AFTER : 0; lvInsertMark.iItem = index; UnsafeNativeMethods.SendMessage(new HandleRef(listView, listView.Handle), NativeMethods.LVM_SETINSERTMARK, 0, lvInsertMark); if (!color.IsEmpty) { listView.SendMessage(NativeMethods.LVM_SETINSERTMARKCOLOR, 0, SafeNativeMethods.ColorToCOLORREF(color)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
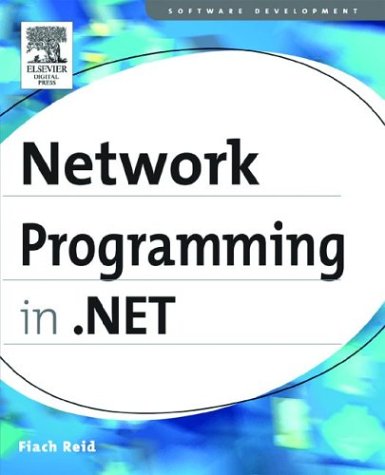
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PageRequestManager.cs
- MenuBase.cs
- DropAnimation.xaml.cs
- MemberInfoSerializationHolder.cs
- HtmlInputRadioButton.cs
- MatrixCamera.cs
- ColumnHeaderConverter.cs
- SmtpException.cs
- XmlDocumentFragment.cs
- TreeWalker.cs
- figurelength.cs
- EdgeProfileValidation.cs
- WorkflowOperationContext.cs
- Stroke2.cs
- FtpWebResponse.cs
- diagnosticsswitches.cs
- WebBrowserNavigatedEventHandler.cs
- ToolboxDataAttribute.cs
- HttpFileCollection.cs
- PlaceHolder.cs
- InvalidComObjectException.cs
- ResourceProviderFactory.cs
- Condition.cs
- IconConverter.cs
- SByte.cs
- WebPartConnectionsConnectVerb.cs
- Opcode.cs
- UpdatePanelTrigger.cs
- DataServiceQueryException.cs
- DataDocumentXPathNavigator.cs
- CharacterBuffer.cs
- FrameworkElementFactoryMarkupObject.cs
- ConfigurationErrorsException.cs
- SystemWebCachingSectionGroup.cs
- ISessionStateStore.cs
- FileDataSourceCache.cs
- ObjRef.cs
- LinearGradientBrush.cs
- JoinGraph.cs
- MultiSelectRootGridEntry.cs
- ContextMenu.cs
- NetDataContractSerializer.cs
- DispatcherHookEventArgs.cs
- CompositeFontParser.cs
- Action.cs
- Funcletizer.cs
- BitStream.cs
- Vars.cs
- Figure.cs
- DES.cs
- BaseAsyncResult.cs
- ReflectionTypeLoadException.cs
- DisableDpiAwarenessAttribute.cs
- PointLight.cs
- XmlAttributeOverrides.cs
- AttributeEmitter.cs
- InstanceCreationEditor.cs
- UrlMapping.cs
- CompareValidator.cs
- ButtonAutomationPeer.cs
- AssemblyAssociatedContentFileAttribute.cs
- HostDesigntimeLicenseContext.cs
- BrushMappingModeValidation.cs
- TriggerActionCollection.cs
- HashStream.cs
- tooltip.cs
- TemplateComponentConnector.cs
- QuaternionAnimationBase.cs
- WsdlInspector.cs
- KeyValuePairs.cs
- ButtonFieldBase.cs
- UnsafeNativeMethodsTablet.cs
- GridEntry.cs
- PathFigure.cs
- HttpMethodConstraint.cs
- ClassHandlersStore.cs
- WmlLiteralTextAdapter.cs
- DropDownList.cs
- PersonalizationEntry.cs
- Utility.cs
- UTF7Encoding.cs
- WindowsFont.cs
- CodeTypeMemberCollection.cs
- TypeSystem.cs
- TemplateXamlParser.cs
- HttpGetProtocolReflector.cs
- X509CertificateInitiatorClientCredential.cs
- KerberosSecurityTokenProvider.cs
- XmlNamespaceManager.cs
- DataKeyCollection.cs
- MethodSet.cs
- RouteItem.cs
- EntityTypeBase.cs
- HttpApplicationStateWrapper.cs
- FontSizeConverter.cs
- OdbcConnectionOpen.cs
- SizeChangedInfo.cs
- ClientBuildManagerCallback.cs
- DependencyPropertyKey.cs
- CodeEntryPointMethod.cs