Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / Printing / PrintControllerWithStatusDialog.cs / 1 / PrintControllerWithStatusDialog.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using System.Threading; using System.Drawing; using Microsoft.Win32; using System.ComponentModel; using System.Drawing.Printing; using System.Security; using System.Security.Permissions; ////// /// public class PrintControllerWithStatusDialog : PrintController { private PrintController underlyingController; private PrintDocument document; private BackgroundThread backgroundThread; private int pageNumber; private string dialogTitle; ///[To be supplied.] ////// /// public PrintControllerWithStatusDialog(PrintController underlyingController) : this(underlyingController, SR.GetString(SR.PrintControllerWithStatusDialog_DialogTitlePrint)) { } ///[To be supplied.] ////// /// public PrintControllerWithStatusDialog(PrintController underlyingController, string dialogTitle) { this.underlyingController = underlyingController; this.dialogTitle = dialogTitle; } ///[To be supplied.] ////// /// public override bool IsPreview { get { if (underlyingController != null) { return underlyingController.IsPreview; } return false; } } ////// This is new public property which notifies if this controller is used for PrintPreview.. so get the underlying Controller /// and return its IsPreview Property. /// ////// /// /// public override void OnStartPrint(PrintDocument document, PrintEventArgs e) { base.OnStartPrint(document, e); this.document = document; pageNumber = 1; if (SystemInformation.UserInteractive) { backgroundThread = new BackgroundThread(this); // starts running & shows dialog automatically } // OnStartPrint does the security check... lots of // extra setup to make sure that we tear down // correctly... // try { underlyingController.OnStartPrint(document, e); } catch { if (backgroundThread != null) { backgroundThread.Stop(); } throw; } finally { if (backgroundThread != null && backgroundThread.canceled) { e.Cancel = true; } } } ////// Implements StartPrint by delegating to the underlying controller. /// ////// /// /// public override Graphics OnStartPage(PrintDocument document, PrintPageEventArgs e) { base.OnStartPage(document, e); if (backgroundThread != null) { backgroundThread.UpdateLabel(); } Graphics result = underlyingController.OnStartPage(document, e); if (backgroundThread != null && backgroundThread.canceled){ e.Cancel = true; } return result; } ////// Implements StartPage by delegating to the underlying controller. /// ////// /// /// public override void OnEndPage(PrintDocument document, PrintPageEventArgs e) { underlyingController.OnEndPage(document, e); if (backgroundThread != null && backgroundThread.canceled) { e.Cancel = true; } pageNumber++; base.OnEndPage(document, e); } ////// Implements EndPage by delegating to the underlying controller. /// ////// /// /// public override void OnEndPrint(PrintDocument document, PrintEventArgs e) { underlyingController.OnEndPrint(document, e); if (backgroundThread != null && backgroundThread.canceled) { e.Cancel = true; } if (backgroundThread != null) { backgroundThread.Stop(); } base.OnEndPrint(document, e); } private class BackgroundThread { private PrintControllerWithStatusDialog parent; private StatusDialog dialog; private Thread thread; internal bool canceled = false; private bool alreadyStopped = false; // Called from any thread internal BackgroundThread(PrintControllerWithStatusDialog parent) { this.parent = parent; // Calling Application.DoEvents() from within a paint event causes all sorts of problems, // so we need to put the dialog on its own thread. thread = new Thread(new ThreadStart(Run)); thread.SetApartmentState(ApartmentState.STA); thread.Start(); } // on correct thread [ UIPermission(SecurityAction.Assert, Window=UIPermissionWindow.AllWindows), SecurityPermission(SecurityAction.Assert, Flags=SecurityPermissionFlag.UnmanagedCode), ] private void Run() { // SECREVIEW : need all permissions to make the window not get adorned... // try { lock (this) { if (alreadyStopped) { return; } dialog = new StatusDialog(this, parent.dialogTitle); ThreadUnsafeUpdateLabel(); dialog.Visible = true; } if (!alreadyStopped) { Application.Run(dialog); } } finally { lock (this) { if (dialog != null) { dialog.Dispose(); dialog = null; } } } } // Called from any thread internal void Stop() { lock (this) { if (dialog != null && dialog.IsHandleCreated) { dialog.BeginInvoke(new MethodInvoker(dialog.Close)); return; } alreadyStopped = true; } } // on correct thread private void ThreadUnsafeUpdateLabel() { // "page {0} of {1}" dialog.label1.Text = SR.GetString(SR.PrintControllerWithStatusDialog_NowPrinting, parent.pageNumber, parent.document.DocumentName); } // Called from any thread internal void UpdateLabel() { if (dialog != null && dialog.IsHandleCreated) { dialog.BeginInvoke(new MethodInvoker(ThreadUnsafeUpdateLabel)); // Don't wait for a response } } } private class StatusDialog : Form { internal Label label1; private Button button1; private BackgroundThread backgroundThread; internal StatusDialog(BackgroundThread backgroundThread, string dialogTitle) { InitializeComponent(); this.backgroundThread = backgroundThread; this.Text = dialogTitle; this.MinimumSize = Size; } ////// Implements EndPrint by delegating to the underlying controller. /// ////// Tells whether the current resources for this dll have been /// localized for a RTL language. /// private static bool IsRTLResources { get { return SR.GetString(SR.RTL) != "RTL_False"; } } private void InitializeComponent() { if (IsRTLResources) { this.RightToLeft = RightToLeft.Yes; } this.label1 = new Label(); this.button1 = new Button(); label1.Location = new Point(8, 16); label1.TextAlign = ContentAlignment.MiddleCenter; label1.Size = new Size(240, 64); label1.TabIndex = 1; label1.Anchor = AnchorStyles.Top | AnchorStyles.Left | AnchorStyles.Right; button1.Size = new Size(75, 23); button1.TabIndex = 0; button1.Text = SR.GetString(SR.PrintControllerWithStatusDialog_Cancel); button1.Location = new Point(88, 88); button1.Anchor = AnchorStyles.Bottom; button1.Click += new EventHandler(button1_Click); this.AutoScaleDimensions = new Size(6, 13); this.AutoScaleMode = AutoScaleMode.Font; this.MaximizeBox = false; this.ControlBox = false; this.MinimizeBox = false; this.ClientSize = new Size(256, 122); this.CancelButton = button1; this.SizeGripStyle = System.Windows.Forms.SizeGripStyle.Hide; this.Controls.Add(label1); this.Controls.Add(button1); } private void button1_Click(object sender, System.EventArgs e) { button1.Enabled = false; label1.Text = SR.GetString(SR.PrintControllerWithStatusDialog_Canceling); backgroundThread.canceled = true; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
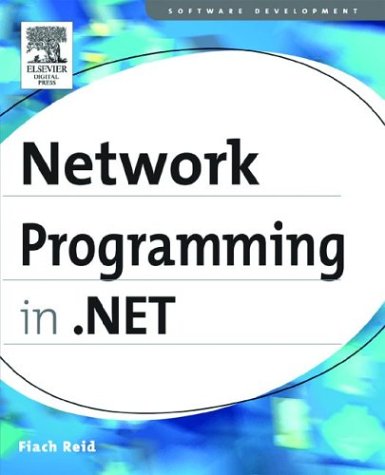
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CharUnicodeInfo.cs
- SByteConverter.cs
- HTMLTextWriter.cs
- BezierSegment.cs
- PropertyInfo.cs
- DelegateHelpers.Generated.cs
- PolygonHotSpot.cs
- RegexParser.cs
- XmlTextReaderImpl.cs
- ConfigXmlComment.cs
- DataGridViewImageCell.cs
- MouseDevice.cs
- configsystem.cs
- SqlVisitor.cs
- DataGridViewCellPaintingEventArgs.cs
- XmlSchemaAnnotation.cs
- QueryCreatedEventArgs.cs
- TextBoxDesigner.cs
- EntryWrittenEventArgs.cs
- DrawListViewSubItemEventArgs.cs
- AssemblyInfo.cs
- DbParameterCollectionHelper.cs
- PageContentCollection.cs
- NumberSubstitution.cs
- TemplatePagerField.cs
- EntityClassGenerator.cs
- Rule.cs
- TreeViewBindingsEditorForm.cs
- HelpProvider.cs
- _NtlmClient.cs
- SystemDropShadowChrome.cs
- XmlDataSourceView.cs
- HebrewNumber.cs
- EasingKeyFrames.cs
- SpeechUI.cs
- ReflectTypeDescriptionProvider.cs
- DeflateEmulationStream.cs
- CapacityStreamGeometryContext.cs
- Helper.cs
- RequestReplyCorrelator.cs
- PageTheme.cs
- XmlSchemaSimpleContent.cs
- XmlUrlResolver.cs
- CodeTypeReference.cs
- ReplyChannelAcceptor.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- ManipulationStartedEventArgs.cs
- TimeoutException.cs
- GAC.cs
- DecimalConverter.cs
- ClockController.cs
- Int16Converter.cs
- SoapTransportImporter.cs
- WebPartVerbCollection.cs
- FormsIdentity.cs
- ColorMatrix.cs
- UdpTransportBindingElement.cs
- Cursor.cs
- DataServiceCollectionOfT.cs
- TdsValueSetter.cs
- RowToFieldTransformer.cs
- ConfigurationCollectionAttribute.cs
- Process.cs
- HashHelper.cs
- WindowExtensionMethods.cs
- GridToolTip.cs
- BuildProviderAppliesToAttribute.cs
- ToolboxItem.cs
- SizeAnimationClockResource.cs
- EdmError.cs
- WindowsBrush.cs
- FlowPanelDesigner.cs
- PropVariant.cs
- NavigationPropertyEmitter.cs
- XmlSchemaSubstitutionGroup.cs
- GroupQuery.cs
- Scripts.cs
- DataFormats.cs
- DataSourceProvider.cs
- SerializableAttribute.cs
- XhtmlBasicTextBoxAdapter.cs
- DirectoryObjectSecurity.cs
- SerializationEventsCache.cs
- GradientStop.cs
- DefaultSerializationProviderAttribute.cs
- ClientSettingsStore.cs
- NextPreviousPagerField.cs
- NonDualMessageSecurityOverHttpElement.cs
- ContextMenu.cs
- BindingWorker.cs
- nulltextcontainer.cs
- Properties.cs
- EditorZone.cs
- Tablet.cs
- Missing.cs
- NativeMethods.cs
- Module.cs
- PromptBuilder.cs
- XmlEncoding.cs
- HGlobalSafeHandle.cs