Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Common / Utils / Boolean / Vertex.cs / 1 / Vertex.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; namespace System.Data.Common.Utils.Boolean { using System.Diagnostics; using System.Globalization; ////// A node in a Reduced Ordered Boolean Decision Diagram. Reads as: /// /// if 'Variable' then 'Then' else 'Else' /// /// Invariant: the Then and Else children must refer to 'deeper' variables, /// or variables with a higher value. Otherwise, the graph is not 'Ordered'. /// All creation of vertices is mediated by the Solver class which ensures /// each vertex is unique. Otherwise, the graph is not 'Reduced'. /// sealed class Vertex : IEquatable{ /// /// Initializes a sink BDD node (zero or one) /// private Vertex() { this.Variable = int.MaxValue; this.Children = new Vertex[] { }; } internal Vertex(int variable, Vertex[] children) { EntityUtil.BoolExprAssert(variable < int.MaxValue, "exceeded number of supported variables"); AssertConstructorArgumentsValid(variable, children); this.Variable = variable; this.Children = children; } [Conditional("DEBUG")] private static void AssertConstructorArgumentsValid(int variable, Vertex[] children) { Debug.Assert(null != children, "internal vertices must define children"); Debug.Assert(2 <= children.Length, "internal vertices must have at least two children"); Debug.Assert(0 < variable, "internal vertices must have 0 < variable"); foreach (Vertex child in children) { Debug.Assert(variable < child.Variable, "children must have greater variable"); } } ////// Sink node representing the Boolean function '1' (true) /// internal static readonly Vertex One = new Vertex(); ////// Sink node representing the Boolean function '0' (false) /// internal static readonly Vertex Zero = new Vertex(); ////// Gets the variable tested by this vertex. If this is a sink node, returns /// int.MaxValue since there is no variable to test (and since this is a leaf, /// this non-existent variable is 'deeper' than any existing variable; the /// variable value is larger than any real variable) /// internal readonly int Variable; ////// Note: do not modify elements. /// Gets the result when Variable evaluates to true. If this is a sink node, /// returns null. /// internal readonly Vertex[] Children; ////// Returns true if this is '1'. /// internal bool IsOne() { return object.ReferenceEquals(Vertex.One, this); } ////// Returns true if this is '0'. /// internal bool IsZero() { return object.ReferenceEquals(Vertex.Zero, this); } ////// Returns true if this is '0' or '1'. /// internal bool IsSink() { return Variable == int.MaxValue; } public bool Equals(Vertex other) { return object.ReferenceEquals(this, other); } public override bool Equals(object obj) { Debug.Fail("used typed Equals"); return base.Equals(obj); } public override int GetHashCode() { return base.GetHashCode(); } public override string ToString() { if (IsOne()) { return "_1_"; } if (IsZero()) { return "_0_"; } return String.Format(CultureInfo.InvariantCulture, "<{0}, {1}>", Variable, StringUtil.ToCommaSeparatedString(Children)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; namespace System.Data.Common.Utils.Boolean { using System.Diagnostics; using System.Globalization; ////// A node in a Reduced Ordered Boolean Decision Diagram. Reads as: /// /// if 'Variable' then 'Then' else 'Else' /// /// Invariant: the Then and Else children must refer to 'deeper' variables, /// or variables with a higher value. Otherwise, the graph is not 'Ordered'. /// All creation of vertices is mediated by the Solver class which ensures /// each vertex is unique. Otherwise, the graph is not 'Reduced'. /// sealed class Vertex : IEquatable{ /// /// Initializes a sink BDD node (zero or one) /// private Vertex() { this.Variable = int.MaxValue; this.Children = new Vertex[] { }; } internal Vertex(int variable, Vertex[] children) { EntityUtil.BoolExprAssert(variable < int.MaxValue, "exceeded number of supported variables"); AssertConstructorArgumentsValid(variable, children); this.Variable = variable; this.Children = children; } [Conditional("DEBUG")] private static void AssertConstructorArgumentsValid(int variable, Vertex[] children) { Debug.Assert(null != children, "internal vertices must define children"); Debug.Assert(2 <= children.Length, "internal vertices must have at least two children"); Debug.Assert(0 < variable, "internal vertices must have 0 < variable"); foreach (Vertex child in children) { Debug.Assert(variable < child.Variable, "children must have greater variable"); } } ////// Sink node representing the Boolean function '1' (true) /// internal static readonly Vertex One = new Vertex(); ////// Sink node representing the Boolean function '0' (false) /// internal static readonly Vertex Zero = new Vertex(); ////// Gets the variable tested by this vertex. If this is a sink node, returns /// int.MaxValue since there is no variable to test (and since this is a leaf, /// this non-existent variable is 'deeper' than any existing variable; the /// variable value is larger than any real variable) /// internal readonly int Variable; ////// Note: do not modify elements. /// Gets the result when Variable evaluates to true. If this is a sink node, /// returns null. /// internal readonly Vertex[] Children; ////// Returns true if this is '1'. /// internal bool IsOne() { return object.ReferenceEquals(Vertex.One, this); } ////// Returns true if this is '0'. /// internal bool IsZero() { return object.ReferenceEquals(Vertex.Zero, this); } ////// Returns true if this is '0' or '1'. /// internal bool IsSink() { return Variable == int.MaxValue; } public bool Equals(Vertex other) { return object.ReferenceEquals(this, other); } public override bool Equals(object obj) { Debug.Fail("used typed Equals"); return base.Equals(obj); } public override int GetHashCode() { return base.GetHashCode(); } public override string ToString() { if (IsOne()) { return "_1_"; } if (IsZero()) { return "_0_"; } return String.Format(CultureInfo.InvariantCulture, "<{0}, {1}>", Variable, StringUtil.ToCommaSeparatedString(Children)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
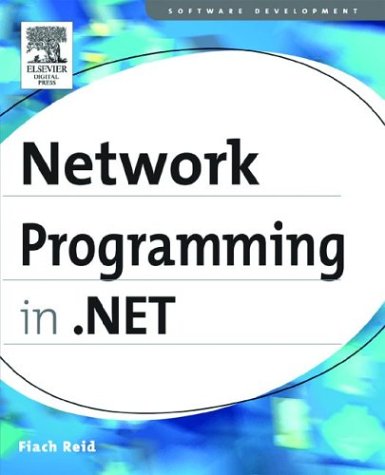
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BoundsDrawingContextWalker.cs
- StorageComplexPropertyMapping.cs
- XPathParser.cs
- SignatureToken.cs
- MissingMethodException.cs
- XPathNodePointer.cs
- AVElementHelper.cs
- RotateTransform.cs
- AssemblyAssociatedContentFileAttribute.cs
- HtmlInputText.cs
- ContourSegment.cs
- ServiceOperationParameter.cs
- X509UI.cs
- GeneralTransform.cs
- RadioButton.cs
- RSACryptoServiceProvider.cs
- RegisteredScript.cs
- DataFieldConverter.cs
- LineUtil.cs
- ListViewInsertEventArgs.cs
- RelatedPropertyManager.cs
- PixelFormats.cs
- SchemaMerger.cs
- AudioFileOut.cs
- CurrencyWrapper.cs
- CapabilitiesPattern.cs
- DESCryptoServiceProvider.cs
- FormClosedEvent.cs
- Pkcs7Signer.cs
- AssemblySettingAttributes.cs
- InputMethodStateTypeInfo.cs
- OrderToken.cs
- RootBrowserWindowAutomationPeer.cs
- CompilerScopeManager.cs
- ScrollBar.cs
- DataExpression.cs
- WindowPattern.cs
- RectangleConverter.cs
- MonitorWrapper.cs
- HtmlEmptyTagControlBuilder.cs
- X509ChainPolicy.cs
- Queue.cs
- SQLDoubleStorage.cs
- MimeTypePropertyAttribute.cs
- StorageBasedPackageProperties.cs
- XPathNavigator.cs
- WindowClosedEventArgs.cs
- HitTestWithGeometryDrawingContextWalker.cs
- RecommendedAsConfigurableAttribute.cs
- _UriSyntax.cs
- XamlSerializerUtil.cs
- TransactionContext.cs
- Cursor.cs
- Error.cs
- CoreSwitches.cs
- MethodToken.cs
- Viewport3DVisual.cs
- safelink.cs
- AutomationPatternInfo.cs
- ResourceCategoryAttribute.cs
- XamlSerializationHelper.cs
- FileDialogPermission.cs
- XPathEmptyIterator.cs
- SqlDataSourceCache.cs
- BatchParser.cs
- FixedTextBuilder.cs
- ConfigUtil.cs
- filewebresponse.cs
- SetterBaseCollection.cs
- MetadataPropertyvalue.cs
- ExpressionVisitor.cs
- EntityProviderFactory.cs
- HyperLinkStyle.cs
- cookie.cs
- __FastResourceComparer.cs
- TextRangeEditLists.cs
- EventWaitHandleSecurity.cs
- ExtentKey.cs
- ContourSegment.cs
- PenContext.cs
- AbstractSvcMapFileLoader.cs
- SeverityFilter.cs
- XmlChildEnumerator.cs
- ValueType.cs
- ChangeDirector.cs
- EmptyQuery.cs
- XmlSchemaImport.cs
- StylusPointPropertyUnit.cs
- ParserStreamGeometryContext.cs
- CompilationLock.cs
- TypedTableGenerator.cs
- DoubleCollection.cs
- SiteMapNodeItem.cs
- PeerResolverSettings.cs
- AssemblyNameProxy.cs
- PropertyHelper.cs
- BrowserCapabilitiesFactory35.cs
- PolygonHotSpot.cs
- TrustManager.cs
- DrawListViewColumnHeaderEventArgs.cs