Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / SolidColorBrush.cs / 1305600 / SolidColorBrush.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: SolidColorBrush.cs // // Description: This file contains the implementation of SolidColorBrush. // The SolidColorBrush is the simplest of the Brushes. consisting // as it does of just a color. // // History: // 04/28/2003 : [....] - Created it. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.PresentationCore; using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Reflection; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; using System.Windows.Markup; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// SolidColorBrush /// The SolidColorBrush is the simplest of the Brushes. It can be used to /// fill an area with a solid color, which can be animate. /// public sealed partial class SolidColorBrush : Brush { #region Constructors ////// Default constructor for SolidColorBrush. /// public SolidColorBrush() { } ////// SolidColorBrush - The constructor accepts the color of the brush /// /// The color value. public SolidColorBrush(Color color) { Color = color; } #endregion Constructors #region Serialization // This enum is used to identify brush types for deserialization in the // ConvertCustomBinaryToObject method. If we support more types of brushes, // then we may have to expose this publically and add more enum values. internal enum SerializationBrushType : byte { Unknown = 0, KnownSolidColor = 1, OtherColor = 2, } ////// Serialize this object using the passed writer in compact BAML binary format. /// ////// This is called ONLY from the Parser and is not a general public method. /// ////// Thrown if "writer" is null. /// [FriendAccessAllowed] // Built into Core, also used by Framework. internal static bool SerializeOn(BinaryWriter writer, string stringValue) { // ********* VERY IMPORTANT NOTE ***************** // If this method is changed, then XamlBrushSerilaizer.SerializeOn() needs // to be correspondingly changed as well. That code is linked into PBT.dll // and duplicates the code below to avoid pulling in SCB & base classes as well. // ********* VERY IMPORTANT NOTE ***************** if (writer == null) { throw new ArgumentNullException("writer"); } KnownColor knownColor = KnownColors.ColorStringToKnownColor(stringValue); if (knownColor != KnownColor.UnknownColor) { // Serialize values of the type "Red", "Blue" and other names writer.Write((byte)SerializationBrushType.KnownSolidColor); writer.Write((uint)knownColor); return true; } else { // Serialize values of the type "#F00", "#0000FF" and other hex color values. // We don't have a good way to check if this is valid without running the // converter at this point, so just store the string if it has at least a // minimum length of 4. stringValue = stringValue.Trim(); if (stringValue.Length > 3) { writer.Write((byte)SerializationBrushType.OtherColor); writer.Write(stringValue); return true; } } return false; } ////// Deserialize this object using the passed reader. Throw an exception if /// the format is not a solid color brush. /// ////// This is called ONLY from the Parser and is not a general public method. /// ////// Thrown if "reader" is null. /// public static object DeserializeFrom(BinaryReader reader) { if (reader == null) { throw new ArgumentNullException("reader"); } return DeserializeFrom(reader, null); } internal static object DeserializeFrom(BinaryReader reader, ITypeDescriptorContext context) { SerializationBrushType brushType = (SerializationBrushType)reader.ReadByte(); if (brushType == SerializationBrushType.KnownSolidColor) { uint knownColorUint = reader.ReadUInt32(); return KnownColors.SolidColorBrushFromUint(knownColorUint); } else if (brushType == SerializationBrushType.OtherColor) { string colorValue = reader.ReadString(); BrushConverter converter = new BrushConverter(); return converter.ConvertFromInvariantString(context, colorValue); } else { throw new Exception(SR.Get(SRID.BrushUnknownBamlType)); } } #endregion Serialization #region ToString ////// CanSerializeToString - an internal helper method which determines whether this object /// can fully serialize to a string with no data loss. /// ////// bool - true if full fidelity serialization is possible, false if not. /// internal override bool CanSerializeToString() { if (HasAnimatedProperties || HasAnyExpression() || !Transform.IsIdentity || !DoubleUtil.AreClose(Opacity, Brush.c_Opacity)) { return false; } return true; } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal override string ConvertToString(string format, IFormatProvider provider) { return Color.ConvertToString(format, provider); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: SolidColorBrush.cs // // Description: This file contains the implementation of SolidColorBrush. // The SolidColorBrush is the simplest of the Brushes. consisting // as it does of just a color. // // History: // 04/28/2003 : [....] - Created it. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.PresentationCore; using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Reflection; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; using System.Windows.Markup; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// SolidColorBrush /// The SolidColorBrush is the simplest of the Brushes. It can be used to /// fill an area with a solid color, which can be animate. /// public sealed partial class SolidColorBrush : Brush { #region Constructors ////// Default constructor for SolidColorBrush. /// public SolidColorBrush() { } ////// SolidColorBrush - The constructor accepts the color of the brush /// /// The color value. public SolidColorBrush(Color color) { Color = color; } #endregion Constructors #region Serialization // This enum is used to identify brush types for deserialization in the // ConvertCustomBinaryToObject method. If we support more types of brushes, // then we may have to expose this publically and add more enum values. internal enum SerializationBrushType : byte { Unknown = 0, KnownSolidColor = 1, OtherColor = 2, } ////// Serialize this object using the passed writer in compact BAML binary format. /// ////// This is called ONLY from the Parser and is not a general public method. /// ////// Thrown if "writer" is null. /// [FriendAccessAllowed] // Built into Core, also used by Framework. internal static bool SerializeOn(BinaryWriter writer, string stringValue) { // ********* VERY IMPORTANT NOTE ***************** // If this method is changed, then XamlBrushSerilaizer.SerializeOn() needs // to be correspondingly changed as well. That code is linked into PBT.dll // and duplicates the code below to avoid pulling in SCB & base classes as well. // ********* VERY IMPORTANT NOTE ***************** if (writer == null) { throw new ArgumentNullException("writer"); } KnownColor knownColor = KnownColors.ColorStringToKnownColor(stringValue); if (knownColor != KnownColor.UnknownColor) { // Serialize values of the type "Red", "Blue" and other names writer.Write((byte)SerializationBrushType.KnownSolidColor); writer.Write((uint)knownColor); return true; } else { // Serialize values of the type "#F00", "#0000FF" and other hex color values. // We don't have a good way to check if this is valid without running the // converter at this point, so just store the string if it has at least a // minimum length of 4. stringValue = stringValue.Trim(); if (stringValue.Length > 3) { writer.Write((byte)SerializationBrushType.OtherColor); writer.Write(stringValue); return true; } } return false; } ////// Deserialize this object using the passed reader. Throw an exception if /// the format is not a solid color brush. /// ////// This is called ONLY from the Parser and is not a general public method. /// ////// Thrown if "reader" is null. /// public static object DeserializeFrom(BinaryReader reader) { if (reader == null) { throw new ArgumentNullException("reader"); } return DeserializeFrom(reader, null); } internal static object DeserializeFrom(BinaryReader reader, ITypeDescriptorContext context) { SerializationBrushType brushType = (SerializationBrushType)reader.ReadByte(); if (brushType == SerializationBrushType.KnownSolidColor) { uint knownColorUint = reader.ReadUInt32(); return KnownColors.SolidColorBrushFromUint(knownColorUint); } else if (brushType == SerializationBrushType.OtherColor) { string colorValue = reader.ReadString(); BrushConverter converter = new BrushConverter(); return converter.ConvertFromInvariantString(context, colorValue); } else { throw new Exception(SR.Get(SRID.BrushUnknownBamlType)); } } #endregion Serialization #region ToString ////// CanSerializeToString - an internal helper method which determines whether this object /// can fully serialize to a string with no data loss. /// ////// bool - true if full fidelity serialization is possible, false if not. /// internal override bool CanSerializeToString() { if (HasAnimatedProperties || HasAnyExpression() || !Transform.IsIdentity || !DoubleUtil.AreClose(Opacity, Brush.c_Opacity)) { return false; } return true; } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal override string ConvertToString(string format, IFormatProvider provider) { return Color.ConvertToString(format, provider); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
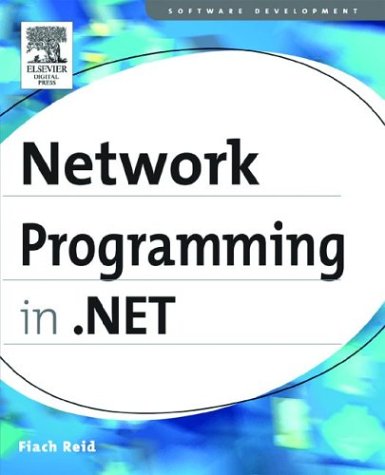
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NativeMethodsOther.cs
- Boolean.cs
- PropertyToken.cs
- TextTrailingCharacterEllipsis.cs
- ChtmlTextWriter.cs
- SendActivityValidator.cs
- TextProviderWrapper.cs
- PerfCounterSection.cs
- Block.cs
- AssociationSet.cs
- AttachmentService.cs
- CreateSequence.cs
- LocalizationComments.cs
- EncoderNLS.cs
- ObjectParameterCollection.cs
- DataGridViewRowConverter.cs
- ConfigDefinitionUpdates.cs
- StandardCommands.cs
- COAUTHIDENTITY.cs
- SBCSCodePageEncoding.cs
- SizeValueSerializer.cs
- EndpointInstanceProvider.cs
- XmlParserContext.cs
- DataContractSet.cs
- MetafileHeaderWmf.cs
- SerializableAttribute.cs
- ListViewGroup.cs
- LineGeometry.cs
- ColorContext.cs
- TrackBarRenderer.cs
- DefaultAutoFieldGenerator.cs
- QuotaThrottle.cs
- UniqueSet.cs
- BaseCodeDomTreeGenerator.cs
- UInt16Storage.cs
- MatrixValueSerializer.cs
- ElementMarkupObject.cs
- RegexTree.cs
- PartialToken.cs
- MenuTracker.cs
- IChannel.cs
- AsymmetricSignatureFormatter.cs
- ActivityPreviewDesigner.cs
- HTTPNotFoundHandler.cs
- EmptyEnumerable.cs
- CodeFieldReferenceExpression.cs
- ApplicationServiceHelper.cs
- SerialStream.cs
- NullRuntimeConfig.cs
- RsaKeyIdentifierClause.cs
- X500Name.cs
- OleDbFactory.cs
- GridViewDeleteEventArgs.cs
- PresentationAppDomainManager.cs
- DES.cs
- DataGridTemplateColumn.cs
- COM2ComponentEditor.cs
- StringValueSerializer.cs
- DesignerVerbCollection.cs
- Binding.cs
- Transform3D.cs
- TraceSource.cs
- CodeDesigner.cs
- HyperLinkColumn.cs
- UnsafeNativeMethods.cs
- EmptyEnumerator.cs
- COM2PropertyDescriptor.cs
- AutomationPattern.cs
- FilterQuery.cs
- DoubleAnimationClockResource.cs
- MultipartIdentifier.cs
- ObjectResult.cs
- CompiledQuery.cs
- PathGeometry.cs
- XmlComplianceUtil.cs
- CodeGeneratorOptions.cs
- SocketElement.cs
- ScaleTransform.cs
- FrameworkContentElement.cs
- ProviderConnectionPointCollection.cs
- XmlWhitespace.cs
- SafeTokenHandle.cs
- ServiceNameElement.cs
- SessionStateContainer.cs
- IgnoreDataMemberAttribute.cs
- Tracking.cs
- EditorBrowsableAttribute.cs
- MobileControl.cs
- RequestCachingSection.cs
- CategoryNameCollection.cs
- DodSequenceMerge.cs
- CaretElement.cs
- TabletDeviceInfo.cs
- PrinterResolution.cs
- WebScriptMetadataMessage.cs
- Pair.cs
- SystemIcmpV6Statistics.cs
- Interfaces.cs
- StaticTextPointer.cs
- ArithmeticLiteral.cs