Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / WebParts / EditorZoneDesigner.cs / 1 / EditorZoneDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design.WebControls.WebParts { using System.ComponentModel; using System.ComponentModel.Design; using System.Data; using System.Design; using System.Diagnostics; using System.Globalization; using System.IO; using System.Web.UI.Design; using System.Web.UI.Design.WebControls; using System.Web.UI.WebControls; using System.Web.UI.WebControls.WebParts; ////// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] public class EditorZoneDesigner : ToolZoneDesigner { private static DesignerAutoFormatCollection _autoFormats; private EditorZone _zone; private TemplateGroup _templateGroup; public override DesignerAutoFormatCollection AutoFormats { get { if (_autoFormats == null) { _autoFormats = CreateAutoFormats(AutoFormatSchemes.EDITORZONE_SCHEMES, delegate(DataRow schemeData) { return new EditorZoneAutoFormat(schemeData); }); } return _autoFormats; } } public override TemplateGroupCollection TemplateGroups { get { TemplateGroupCollection groups = base.TemplateGroups; if (_templateGroup == null) { _templateGroup = CreateZoneTemplateGroup(); } groups.Add(_templateGroup); return groups; } } public override string GetDesignTimeHtml() { return GetDesignTimeHtml(null); } ////// Provides the layout html for the control in the designer, including regions. /// public override string GetDesignTimeHtml(DesignerRegionCollection regions) { string designTimeHtml; try { EditorZone zone = (EditorZone)ViewControl; bool useRegions = UseRegions(regions, _zone.ZoneTemplate, zone.ZoneTemplate); // When there is an editable region, we want to use the regular control // rendering instead of the EmptyDesignTimeHtml if (zone.ZoneTemplate == null && !useRegions) { designTimeHtml = GetEmptyDesignTimeHtml(); } else { ((ICompositeControlDesignerAccessor)zone).RecreateChildControls(); if (regions != null && useRegions) { // If the tools supports editable regions, the initial rendering of the // WebParts in the Zone is thrown away by the tool anyway, so we should clear // the controls collection before rendering. If we don't clear the controls // collection and a WebPart inside the Zone throws an exception when rendering, // this would cause the whole Zone to render as an error, instead of just // the offending WebPart. This also improves perf. zone.Controls.Clear(); EditorPartEditableDesignerRegion region = new EditorPartEditableDesignerRegion(zone, TemplateDefinition); // Tells Venus that all controls inside the EditableRegion should be parented to the zone region.Properties[typeof(Control)] = zone; region.IsSingleInstanceTemplate = true; region.Description = SR.GetString(SR.ContainerControlDesigner_RegionWatermark); regions.Add(region); } designTimeHtml = base.GetDesignTimeHtml(); } // Don't substitute the placeholder if this is the AutoFormat PreviewControl if (ViewInBrowseMode && zone.ID != EditorZoneAutoFormat.PreviewControlID) { designTimeHtml = CreatePlaceHolderDesignTimeHtml(); } } catch (Exception e) { designTimeHtml = GetErrorDesignTimeHtml(e); } return designTimeHtml; } ////// /// Get the content for the specified region /// public override string GetEditableDesignerRegionContent(EditableDesignerRegion region) { Debug.Assert(region != null); // Occasionally getting NullRef here in WebMatrix. Maybe Zone is null? Debug.Assert(_zone != null); return ControlPersister.PersistTemplate(_zone.ZoneTemplate, (IDesignerHost)Component.Site.GetService(typeof(IDesignerHost))); } protected override string GetEmptyDesignTimeHtml() { return CreatePlaceHolderDesignTimeHtml(SR.GetString(SR.EditorZoneDesigner_Empty)); } public override void Initialize(IComponent component) { VerifyInitializeArgument(component, typeof(EditorZone)); base.Initialize(component); _zone = (EditorZone)component; } ////// /// Set the content for the specified region /// public override void SetEditableDesignerRegionContent(EditableDesignerRegion region, string content) { Debug.Assert(region != null); _zone.ZoneTemplate = ControlParser.ParseTemplate((IDesignerHost)Component.Site.GetService(typeof(IDesignerHost)), content); IsDirtyInternal = true; } private sealed class EditorPartEditableDesignerRegion : TemplatedEditableDesignerRegion { private EditorZone _zone; public EditorPartEditableDesignerRegion(EditorZone zone, TemplateDefinition templateDefinition) : base(templateDefinition) { _zone = zone; } public override ViewRendering GetChildViewRendering(Control control) { if (control == null) { throw new ArgumentNullException("control"); } DesignerEditorPartChrome chrome = new DesignerEditorPartChrome(_zone); return chrome.GetViewRendering(control); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
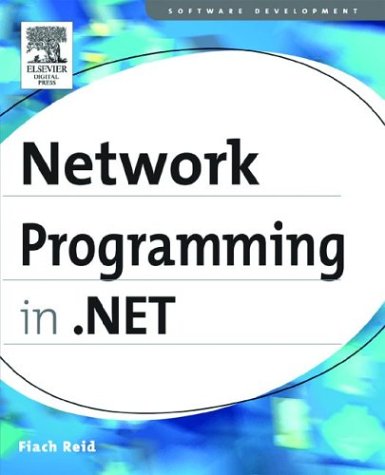
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ViewStateModeByIdAttribute.cs
- WebPartHeaderCloseVerb.cs
- GridItemCollection.cs
- ScopelessEnumAttribute.cs
- CompilationLock.cs
- ZipIOExtraFieldPaddingElement.cs
- UserControl.cs
- RunWorkerCompletedEventArgs.cs
- BaseDataListActionList.cs
- ApplicationManager.cs
- ToolBarButton.cs
- tooltip.cs
- HttpListenerContext.cs
- ArgumentNullException.cs
- TextBoxBase.cs
- ListBindingConverter.cs
- DecimalStorage.cs
- ProjectionPathSegment.cs
- PageMediaType.cs
- _emptywebproxy.cs
- Stack.cs
- codemethodreferenceexpression.cs
- BindingNavigatorDesigner.cs
- ReceiveActivityValidator.cs
- ComponentChangedEvent.cs
- DocumentCollection.cs
- MulticastOption.cs
- Adorner.cs
- CellQuery.cs
- PrivateFontCollection.cs
- SqlInternalConnectionTds.cs
- GenericsInstances.cs
- MembershipUser.cs
- PopupRoot.cs
- HtmlTable.cs
- RuleSettingsCollection.cs
- ConstructorExpr.cs
- DecoratedNameAttribute.cs
- GridItem.cs
- ResourceDescriptionAttribute.cs
- AspCompat.cs
- SqlAggregateChecker.cs
- SortKey.cs
- safelinkcollection.cs
- XmlSchemaSimpleType.cs
- LabelDesigner.cs
- HandleExceptionArgs.cs
- TraceXPathNavigator.cs
- DbConnectionPoolIdentity.cs
- TimerElapsedEvenArgs.cs
- ActivationServices.cs
- LayoutEngine.cs
- BitmapSourceSafeMILHandle.cs
- CodeSubDirectory.cs
- StdValidatorsAndConverters.cs
- CngKey.cs
- VisualStates.cs
- TextBoxBase.cs
- XslVisitor.cs
- ViewStateModeByIdAttribute.cs
- UserControlParser.cs
- Validator.cs
- NavigationEventArgs.cs
- ConstrainedDataObject.cs
- DataColumnPropertyDescriptor.cs
- TrackingMemoryStream.cs
- IOException.cs
- LayoutDump.cs
- FatalException.cs
- ResourceReader.cs
- Stroke2.cs
- AuthorizationPolicyTypeElementCollection.cs
- VisualStyleTypesAndProperties.cs
- TransactedReceiveData.cs
- SmiMetaDataProperty.cs
- DataGridViewCellStateChangedEventArgs.cs
- HttpRequest.cs
- ObjectStateEntryDbDataRecord.cs
- ThreadAbortException.cs
- EntityDataSourceSelectingEventArgs.cs
- SettingsPropertyValueCollection.cs
- AppDomain.cs
- DesignerDataStoredProcedure.cs
- Array.cs
- CompositeCollection.cs
- Char.cs
- TextAction.cs
- ListViewGroup.cs
- Drawing.cs
- WebPartPersonalization.cs
- DescendentsWalker.cs
- OdbcCommand.cs
- DecoderBestFitFallback.cs
- LabelEditEvent.cs
- ReverseInheritProperty.cs
- PanelStyle.cs
- COMException.cs
- TailPinnedEventArgs.cs
- NetworkInformationPermission.cs
- XmlSchema.cs