Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / MS / Internal / Shaping / UshortList2.cs / 1305600 / UshortList2.cs
//------------------------------------------------------------------------ // // Microsoft Avalon // Copyright (C) Microsoft Corporation, 2002 // // File: List2.cs // // Contents: Internal growable list with sublisting // // Created: 11-04-2002 Worachai Chaoweeraprasit (wchao) // 16-07-2002 sergeym - major rewrite. // //----------------------------------------------------------------------- using System.Windows; using System; using System.Collections; using System.Diagnostics; using MS.Internal; using System.Security; using System.Security.Permissions; namespace MS.Internal.Shaping { ////// Growable ushort array. /// Only current sublist is visible for client. Sublists should be processed /// in sequence from start to end. /// internal class UshortList { internal UshortList(int capacity, int leap) { Invariant.Assert(capacity >= 0 && leap >= 0, "Invalid parameter"); _storage = new UshortArray(capacity, leap); } internal UshortList(ushort[] array) { Invariant.Assert(array != null, "Invalid parameter"); _storage = new UshortArray(array); } internal UshortList(CheckedUShortPointer unsafeArray, int arrayLength) { _storage = new UnsafeUshortArray(unsafeArray, arrayLength); _length = arrayLength; } ////// Critical: This code accepts pointers and manipulates /// them without validation.Critical for set only /// TreatAsSafe: The method does proper bound check. /// public ushort this[int index] { [SecurityCritical,SecurityTreatAsSafe] get { Invariant.Assert(index >= 0 && index < _length, "Index out of range"); return _storage[_index + index]; } [SecurityCritical,SecurityTreatAsSafe] set { Invariant.Assert(index >= 0 && index < _length, "Index out of range"); _storage[_index + index] = value; } } ////// Length of current sublist /// ////// Critical: This code accepts pointers and manipulates /// them without validation, critical only for set /// public int Length { get { return _length; } [SecurityCritical] set { _length = value; } } ////// Offset inside whole storage /// public int Offset { get { return _index; } } ////// Reset processing sequence to the start of the storage /// public void SetRange(int index, int length) { Invariant.Assert(length >= 0 && (index + length) <= _storage.Length, "List out of storage"); _index = index; _length = length; } ////// Insert elements to the current run. Elements are not intialized. /// /// Position /// Number of elements public void Insert(int index, int count) { Invariant.Assert(index <= _length && index >= 0, "Index out of range"); Invariant.Assert(count > 0, "Invalid argument"); _storage.Insert(_index + index, count, _index + _length); _length += count; } ////// Remove elements from the current run. /// /// Position /// Number of elements public void Remove(int index, int count) { Invariant.Assert(index < _length && index >= 0, "Index out of range"); Invariant.Assert(count > 0 && (index + count) <= _length, "Invalid argument"); _storage.Remove(_index + index, count, _index + _length); _length -= count; } public ushort[] ToArray() { return _storage.ToArray(); } public ushort[] GetCopy() { return _storage.GetSubsetCopy(_index,_length); } private UshortBuffer _storage; private int _index; private int _length; } ////// Abstract ushort buffer /// internal abstract class UshortBuffer { protected int _leap; public abstract ushort this[int index] { get; set; } public abstract int Length { get; } public virtual ushort[] ToArray() { Debug.Assert(false, "Not supported"); return null; } public virtual ushort[] GetSubsetCopy(int index, int count) { Debug.Assert(false, "Not supported"); return null; } public virtual void Insert(int index, int count, int length) { Debug.Assert(false, "Not supported"); } public virtual void Remove(int index, int count, int length) { Debug.Assert(false, "Not supported"); } } ////// Ushort buffer implemented as managed ushort array /// internal class UshortArray : UshortBuffer { private ushort[] _array; internal UshortArray(ushort[] array) { _array = array; } internal UshortArray(int capacity, int leap) { _array = new ushort[capacity]; _leap = leap; } public override ushort this[int index] { get { return _array[index]; } set { _array[index] = value; } } public override int Length { get { return _array.Length; } } public override ushort[] ToArray() { return _array; } public override ushort[] GetSubsetCopy(int index, int count) { ushort[] subsetArray = new ushort[count]; //Move elements Buffer.BlockCopy( _array, index * sizeof(ushort), subsetArray, 0, ((index + count) <= _array.Length ? count : _array.Length) * sizeof(ushort) ); return subsetArray; } public override void Insert(int index, int count, int length) { int newLength = length + count; if (newLength > _array.Length) { Invariant.Assert(_leap > 0, "Growing an ungrowable list!"); //increase storage by integral number of _leaps. int extra = newLength - _array.Length; int newArraySize = _array.Length + ((extra - 1) / _leap + 1) * _leap; // get a new buffer ushort[] newArray = new ushort[newArraySize]; //Move elements Buffer.BlockCopy(_array, 0, newArray, 0, index * sizeof(ushort)); if (index < length) { Buffer.BlockCopy( _array, index * sizeof(ushort), newArray, (index + count) * sizeof(ushort), (length - index) * sizeof(ushort) ); } _array = newArray; } else { if (index < length) { Buffer.BlockCopy( _array, index * sizeof(ushort), _array, (index + count) * sizeof(ushort), (length - index) * sizeof(ushort) ); } } } public override void Remove(int index, int count, int length) { Buffer.BlockCopy( _array, (index + count) * sizeof(ushort), _array, (index) * sizeof(ushort), (length - index - count) * sizeof(ushort) ); } } ////// Ushort buffer implemented as unmanaged ushort array /// internal unsafe class UnsafeUshortArray : UshortBuffer { ////// Critical:Holds reference to a pointer /// [SecurityCritical] private ushort* _array; ////// Critical:Can be used to cause a buffer overrun /// private SecurityCriticalDataForSet_arrayLength; /// /// Critical: This code probes into checked pointer. /// Safe : The pointer is validated at probing. /// [SecurityCritical, SecurityTreatAsSafe] internal UnsafeUshortArray(CheckedUShortPointer array, int arrayLength) { _array = array.Probe(0, arrayLength); _arrayLength.Value = arrayLength; } ////// Critical: This code accepts pointers and manipulates /// them without validation.Critical for set only /// Safe : Setter does propery bound check. /// public override ushort this[int index] { [SecurityCritical,SecurityTreatAsSafe] get { Invariant.Assert(index >= 0 && index < _arrayLength.Value); return _array[index]; } [SecurityCritical, SecurityTreatAsSafe] set { Invariant.Assert(index >= 0 && index < _arrayLength.Value); _array[index] = value; } } public override int Length { get { return _arrayLength.Value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------ // // Microsoft Avalon // Copyright (C) Microsoft Corporation, 2002 // // File: List2.cs // // Contents: Internal growable list with sublisting // // Created: 11-04-2002 Worachai Chaoweeraprasit (wchao) // 16-07-2002 sergeym - major rewrite. // //----------------------------------------------------------------------- using System.Windows; using System; using System.Collections; using System.Diagnostics; using MS.Internal; using System.Security; using System.Security.Permissions; namespace MS.Internal.Shaping { ////// Growable ushort array. /// Only current sublist is visible for client. Sublists should be processed /// in sequence from start to end. /// internal class UshortList { internal UshortList(int capacity, int leap) { Invariant.Assert(capacity >= 0 && leap >= 0, "Invalid parameter"); _storage = new UshortArray(capacity, leap); } internal UshortList(ushort[] array) { Invariant.Assert(array != null, "Invalid parameter"); _storage = new UshortArray(array); } internal UshortList(CheckedUShortPointer unsafeArray, int arrayLength) { _storage = new UnsafeUshortArray(unsafeArray, arrayLength); _length = arrayLength; } ////// Critical: This code accepts pointers and manipulates /// them without validation.Critical for set only /// TreatAsSafe: The method does proper bound check. /// public ushort this[int index] { [SecurityCritical,SecurityTreatAsSafe] get { Invariant.Assert(index >= 0 && index < _length, "Index out of range"); return _storage[_index + index]; } [SecurityCritical,SecurityTreatAsSafe] set { Invariant.Assert(index >= 0 && index < _length, "Index out of range"); _storage[_index + index] = value; } } ////// Length of current sublist /// ////// Critical: This code accepts pointers and manipulates /// them without validation, critical only for set /// public int Length { get { return _length; } [SecurityCritical] set { _length = value; } } ////// Offset inside whole storage /// public int Offset { get { return _index; } } ////// Reset processing sequence to the start of the storage /// public void SetRange(int index, int length) { Invariant.Assert(length >= 0 && (index + length) <= _storage.Length, "List out of storage"); _index = index; _length = length; } ////// Insert elements to the current run. Elements are not intialized. /// /// Position /// Number of elements public void Insert(int index, int count) { Invariant.Assert(index <= _length && index >= 0, "Index out of range"); Invariant.Assert(count > 0, "Invalid argument"); _storage.Insert(_index + index, count, _index + _length); _length += count; } ////// Remove elements from the current run. /// /// Position /// Number of elements public void Remove(int index, int count) { Invariant.Assert(index < _length && index >= 0, "Index out of range"); Invariant.Assert(count > 0 && (index + count) <= _length, "Invalid argument"); _storage.Remove(_index + index, count, _index + _length); _length -= count; } public ushort[] ToArray() { return _storage.ToArray(); } public ushort[] GetCopy() { return _storage.GetSubsetCopy(_index,_length); } private UshortBuffer _storage; private int _index; private int _length; } ////// Abstract ushort buffer /// internal abstract class UshortBuffer { protected int _leap; public abstract ushort this[int index] { get; set; } public abstract int Length { get; } public virtual ushort[] ToArray() { Debug.Assert(false, "Not supported"); return null; } public virtual ushort[] GetSubsetCopy(int index, int count) { Debug.Assert(false, "Not supported"); return null; } public virtual void Insert(int index, int count, int length) { Debug.Assert(false, "Not supported"); } public virtual void Remove(int index, int count, int length) { Debug.Assert(false, "Not supported"); } } ////// Ushort buffer implemented as managed ushort array /// internal class UshortArray : UshortBuffer { private ushort[] _array; internal UshortArray(ushort[] array) { _array = array; } internal UshortArray(int capacity, int leap) { _array = new ushort[capacity]; _leap = leap; } public override ushort this[int index] { get { return _array[index]; } set { _array[index] = value; } } public override int Length { get { return _array.Length; } } public override ushort[] ToArray() { return _array; } public override ushort[] GetSubsetCopy(int index, int count) { ushort[] subsetArray = new ushort[count]; //Move elements Buffer.BlockCopy( _array, index * sizeof(ushort), subsetArray, 0, ((index + count) <= _array.Length ? count : _array.Length) * sizeof(ushort) ); return subsetArray; } public override void Insert(int index, int count, int length) { int newLength = length + count; if (newLength > _array.Length) { Invariant.Assert(_leap > 0, "Growing an ungrowable list!"); //increase storage by integral number of _leaps. int extra = newLength - _array.Length; int newArraySize = _array.Length + ((extra - 1) / _leap + 1) * _leap; // get a new buffer ushort[] newArray = new ushort[newArraySize]; //Move elements Buffer.BlockCopy(_array, 0, newArray, 0, index * sizeof(ushort)); if (index < length) { Buffer.BlockCopy( _array, index * sizeof(ushort), newArray, (index + count) * sizeof(ushort), (length - index) * sizeof(ushort) ); } _array = newArray; } else { if (index < length) { Buffer.BlockCopy( _array, index * sizeof(ushort), _array, (index + count) * sizeof(ushort), (length - index) * sizeof(ushort) ); } } } public override void Remove(int index, int count, int length) { Buffer.BlockCopy( _array, (index + count) * sizeof(ushort), _array, (index) * sizeof(ushort), (length - index - count) * sizeof(ushort) ); } } ////// Ushort buffer implemented as unmanaged ushort array /// internal unsafe class UnsafeUshortArray : UshortBuffer { ////// Critical:Holds reference to a pointer /// [SecurityCritical] private ushort* _array; ////// Critical:Can be used to cause a buffer overrun /// private SecurityCriticalDataForSet_arrayLength; /// /// Critical: This code probes into checked pointer. /// Safe : The pointer is validated at probing. /// [SecurityCritical, SecurityTreatAsSafe] internal UnsafeUshortArray(CheckedUShortPointer array, int arrayLength) { _array = array.Probe(0, arrayLength); _arrayLength.Value = arrayLength; } ////// Critical: This code accepts pointers and manipulates /// them without validation.Critical for set only /// Safe : Setter does propery bound check. /// public override ushort this[int index] { [SecurityCritical,SecurityTreatAsSafe] get { Invariant.Assert(index >= 0 && index < _arrayLength.Value); return _array[index]; } [SecurityCritical, SecurityTreatAsSafe] set { Invariant.Assert(index >= 0 && index < _arrayLength.Value); _array[index] = value; } } public override int Length { get { return _arrayLength.Value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
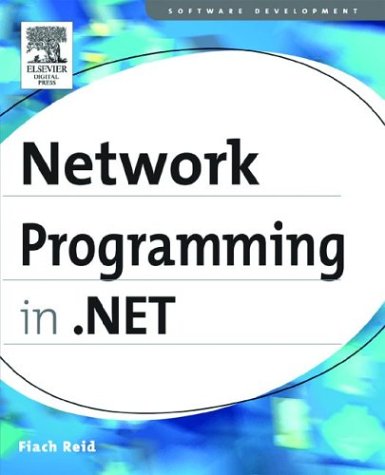
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FrameworkContentElement.cs
- HtmlMeta.cs
- SafeViewOfFileHandle.cs
- UInt32Storage.cs
- Transform3D.cs
- BevelBitmapEffect.cs
- ExportOptions.cs
- XNameTypeConverter.cs
- ExtensionFile.cs
- CollectionContainer.cs
- Util.cs
- NameSpaceExtractor.cs
- CompiledELinqQueryState.cs
- ViewUtilities.cs
- ResourceDefaultValueAttribute.cs
- SoapSchemaMember.cs
- ExceptionUtil.cs
- FormattedText.cs
- MasterPageBuildProvider.cs
- DesignerFrame.cs
- UrlMappingsSection.cs
- AttachedAnnotation.cs
- HMACRIPEMD160.cs
- Table.cs
- DefaultPropertyAttribute.cs
- OSFeature.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- UrlMappingsSection.cs
- CompensationParticipant.cs
- SystemBrushes.cs
- ThumbAutomationPeer.cs
- HtmlTextArea.cs
- SqlDataSourceConfigureFilterForm.cs
- SqlVersion.cs
- ExternalFile.cs
- FixedPageAutomationPeer.cs
- ExpressionHelper.cs
- XmlSchemaSet.cs
- MonitorWrapper.cs
- PenContexts.cs
- BuildProvider.cs
- UniqueID.cs
- PointLightBase.cs
- CmsInterop.cs
- DataListItemCollection.cs
- KnownTypesProvider.cs
- XPathNavigator.cs
- AlternateView.cs
- PropertyEmitterBase.cs
- EdmType.cs
- DynamicQueryableWrapper.cs
- LabelLiteral.cs
- WebZoneDesigner.cs
- RenderDataDrawingContext.cs
- OpenTypeCommon.cs
- ChannelPool.cs
- StateElement.cs
- DataRowComparer.cs
- DrawListViewItemEventArgs.cs
- datacache.cs
- KeyboardEventArgs.cs
- BackgroundFormatInfo.cs
- ClientReliableChannelBinder.cs
- PriorityBindingExpression.cs
- QilChoice.cs
- SizeChangedEventArgs.cs
- MetafileEditor.cs
- MetaModel.cs
- ThrowHelper.cs
- TextFormatterImp.cs
- ConnectionManagementElement.cs
- Rule.cs
- WebEventCodes.cs
- XmlSchemaSimpleContentRestriction.cs
- ListDictionaryInternal.cs
- MetadataUtil.cs
- TcpClientSocketManager.cs
- objectquery_tresulttype.cs
- Region.cs
- NullableLongMinMaxAggregationOperator.cs
- OperationResponse.cs
- HttpApplicationStateBase.cs
- MetadataCache.cs
- CodeParameterDeclarationExpression.cs
- EntityException.cs
- FixedDocument.cs
- CodeAssignStatement.cs
- KnownColorTable.cs
- ExpressionBuilder.cs
- Schema.cs
- AutomationEvent.cs
- WhiteSpaceTrimStringConverter.cs
- Label.cs
- Vector3DAnimationUsingKeyFrames.cs
- JsonWriterDelegator.cs
- TextFindEngine.cs
- EndpointDiscoveryBehavior.cs
- BufferedReadStream.cs
- ObjectListCommand.cs
- Win32.cs