Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Documents / TextEditorTables.cs / 1 / TextEditorTables.cs
//---------------------------------------------------------------------------- // // File: TextEditorTables.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: A Component of TextEditor supporting Table editing commands // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using MS.Internal; using System.Globalization; using System.Threading; using System.ComponentModel; using System.Text; using System.Collections; // ArrayList using System.Runtime.InteropServices; using System.Windows.Threading; using System.Windows.Input; using System.Windows.Controls; // ScrollChangedEventArgs using System.Windows.Controls.Primitives; // CharacterCasing, TextBoxBase using System.Windows.Media; using System.Windows.Markup; using MS.Utility; using MS.Win32; using MS.Internal.Documents; using MS.Internal.Commands; // CommandHelpers ////// Text editing service for controls. /// internal static class TextEditorTables { //----------------------------------------------------- // // Class Internal Methods // //----------------------------------------------------- #region Class Internal Methods // Registers all text editing command handlers for a given control type internal static void _RegisterClassHandlers(Type controlType, bool registerEventListeners) { var onTableCommand = new ExecutedRoutedEventHandler(OnTableCommand); var onQueryStatusNYI = new CanExecuteRoutedEventHandler(OnQueryStatusNYI); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.InsertTable , onTableCommand, onQueryStatusNYI, SRID.KeyInsertTable, SRID.KeyInsertTableDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.InsertRows , onTableCommand, onQueryStatusNYI, SRID.KeyInsertRows, SRID.KeyInsertRowsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.InsertColumns , onTableCommand, onQueryStatusNYI, SRID.KeyInsertColumns, SRID.KeyInsertColumnsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.DeleteRows , onTableCommand, onQueryStatusNYI, SRID.KeyDeleteRows, SRID.KeyDeleteRowsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.DeleteColumns , onTableCommand, onQueryStatusNYI, SRID.KeyDeleteColumns, SRID.KeyDeleteColumnsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.MergeCells , onTableCommand, onQueryStatusNYI, SRID.KeyMergeCells, SRID.KeyMergeCellsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.SplitCell , onTableCommand, onQueryStatusNYI, SRID.KeySplitCell, SRID.KeySplitCellDisplayString); } #endregion Class Internal Methods //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods private static void OnTableCommand(object target, ExecutedRoutedEventArgs args) { TextEditor This = TextEditor._GetTextEditor(target); if (This == null || !This._IsEnabled || This.IsReadOnly || !This.AcceptsRichContent || !(This.Selection is TextSelection)) { return; } TextEditorTyping._FlushPendingInputItems(This); // Forget previously suggested horizontal position TextEditorSelection._ClearSuggestedX(This); // Execute the command if (args.Command == EditingCommands.InsertTable) { ((TextSelection)This.Selection).InsertTable(/*rowCount:*/4, /*columnCount:*/4); } else if (args.Command == EditingCommands.InsertRows) { ((TextSelection)This.Selection).InsertRows(+1); } else if (args.Command == EditingCommands.InsertColumns) { ((TextSelection)This.Selection).InsertColumns(+1); } else if (args.Command == EditingCommands.DeleteRows) { ((TextSelection)This.Selection).DeleteRows(); } else if (args.Command == EditingCommands.DeleteColumns) { ((TextSelection)This.Selection).DeleteColumns(); } else if (args.Command == EditingCommands.MergeCells) { ((TextSelection)This.Selection).MergeCells(); } else if (args.Command == EditingCommands.SplitCell) { ((TextSelection)This.Selection).SplitCell(1000, 1000); // Split all ways to possible maximum } } // ---------------------------------------------------------- // // Misceleneous Commands // // ---------------------------------------------------------- #region Misceleneous Commands ////// StartInputCorrection command QueryStatus handler /// private static void OnQueryStatusNYI(object target, CanExecuteRoutedEventArgs args) { TextEditor This = TextEditor._GetTextEditor(target); if (This == null) { return; } args.CanExecute = true; } #endregion Misceleneous Commands #endregion Private methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: TextEditorTables.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: A Component of TextEditor supporting Table editing commands // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using MS.Internal; using System.Globalization; using System.Threading; using System.ComponentModel; using System.Text; using System.Collections; // ArrayList using System.Runtime.InteropServices; using System.Windows.Threading; using System.Windows.Input; using System.Windows.Controls; // ScrollChangedEventArgs using System.Windows.Controls.Primitives; // CharacterCasing, TextBoxBase using System.Windows.Media; using System.Windows.Markup; using MS.Utility; using MS.Win32; using MS.Internal.Documents; using MS.Internal.Commands; // CommandHelpers ////// Text editing service for controls. /// internal static class TextEditorTables { //----------------------------------------------------- // // Class Internal Methods // //----------------------------------------------------- #region Class Internal Methods // Registers all text editing command handlers for a given control type internal static void _RegisterClassHandlers(Type controlType, bool registerEventListeners) { var onTableCommand = new ExecutedRoutedEventHandler(OnTableCommand); var onQueryStatusNYI = new CanExecuteRoutedEventHandler(OnQueryStatusNYI); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.InsertTable , onTableCommand, onQueryStatusNYI, SRID.KeyInsertTable, SRID.KeyInsertTableDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.InsertRows , onTableCommand, onQueryStatusNYI, SRID.KeyInsertRows, SRID.KeyInsertRowsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.InsertColumns , onTableCommand, onQueryStatusNYI, SRID.KeyInsertColumns, SRID.KeyInsertColumnsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.DeleteRows , onTableCommand, onQueryStatusNYI, SRID.KeyDeleteRows, SRID.KeyDeleteRowsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.DeleteColumns , onTableCommand, onQueryStatusNYI, SRID.KeyDeleteColumns, SRID.KeyDeleteColumnsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.MergeCells , onTableCommand, onQueryStatusNYI, SRID.KeyMergeCells, SRID.KeyMergeCellsDisplayString); CommandHelpers.RegisterCommandHandler(controlType, EditingCommands.SplitCell , onTableCommand, onQueryStatusNYI, SRID.KeySplitCell, SRID.KeySplitCellDisplayString); } #endregion Class Internal Methods //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods private static void OnTableCommand(object target, ExecutedRoutedEventArgs args) { TextEditor This = TextEditor._GetTextEditor(target); if (This == null || !This._IsEnabled || This.IsReadOnly || !This.AcceptsRichContent || !(This.Selection is TextSelection)) { return; } TextEditorTyping._FlushPendingInputItems(This); // Forget previously suggested horizontal position TextEditorSelection._ClearSuggestedX(This); // Execute the command if (args.Command == EditingCommands.InsertTable) { ((TextSelection)This.Selection).InsertTable(/*rowCount:*/4, /*columnCount:*/4); } else if (args.Command == EditingCommands.InsertRows) { ((TextSelection)This.Selection).InsertRows(+1); } else if (args.Command == EditingCommands.InsertColumns) { ((TextSelection)This.Selection).InsertColumns(+1); } else if (args.Command == EditingCommands.DeleteRows) { ((TextSelection)This.Selection).DeleteRows(); } else if (args.Command == EditingCommands.DeleteColumns) { ((TextSelection)This.Selection).DeleteColumns(); } else if (args.Command == EditingCommands.MergeCells) { ((TextSelection)This.Selection).MergeCells(); } else if (args.Command == EditingCommands.SplitCell) { ((TextSelection)This.Selection).SplitCell(1000, 1000); // Split all ways to possible maximum } } // ---------------------------------------------------------- // // Misceleneous Commands // // ---------------------------------------------------------- #region Misceleneous Commands ////// StartInputCorrection command QueryStatus handler /// private static void OnQueryStatusNYI(object target, CanExecuteRoutedEventArgs args) { TextEditor This = TextEditor._GetTextEditor(target); if (This == null) { return; } args.CanExecute = true; } #endregion Misceleneous Commands #endregion Private methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
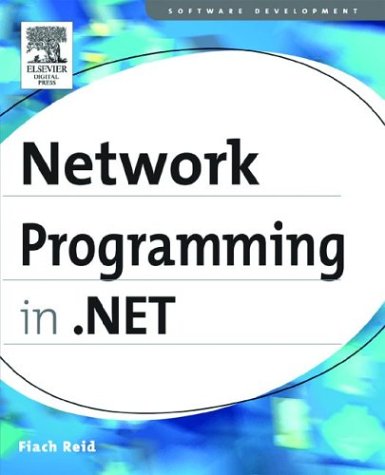
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PolicyUnit.cs
- UnicodeEncoding.cs
- DynamicMetaObjectBinder.cs
- UxThemeWrapper.cs
- Parser.cs
- ProxyWebPart.cs
- InvalidDataException.cs
- SmiContext.cs
- ComboBox.cs
- UnsafeNativeMethodsTablet.cs
- FigureParagraph.cs
- XmlSchemaSimpleContentExtension.cs
- BamlRecordHelper.cs
- XMLSyntaxException.cs
- EncryptedPackageFilter.cs
- XsdCachingReader.cs
- DesignerPainter.cs
- DecoderExceptionFallback.cs
- DependencyObjectPropertyDescriptor.cs
- AutoGeneratedField.cs
- NativeActivityAbortContext.cs
- ipaddressinformationcollection.cs
- DiscoveryReferences.cs
- ParseChildrenAsPropertiesAttribute.cs
- KerberosRequestorSecurityToken.cs
- TableAutomationPeer.cs
- DiscoveryUtility.cs
- DesignerDataView.cs
- ResizingMessageFilter.cs
- CorrelationRequestContext.cs
- DbParameterHelper.cs
- CryptoKeySecurity.cs
- CheckBox.cs
- DataListItemCollection.cs
- ImageKeyConverter.cs
- BaseCodePageEncoding.cs
- IPAddressCollection.cs
- WindowsBrush.cs
- WebPartHeaderCloseVerb.cs
- TabPanel.cs
- HttpCacheVaryByContentEncodings.cs
- XPathNodeList.cs
- MarshalByValueComponent.cs
- DataServiceResponse.cs
- SapiRecognizer.cs
- CfgArc.cs
- InternalCache.cs
- ZipFileInfo.cs
- TextEndOfParagraph.cs
- ValueUtilsSmi.cs
- ChtmlCalendarAdapter.cs
- HtmlLink.cs
- SQLInt32Storage.cs
- EdmScalarPropertyAttribute.cs
- ImmutablePropertyDescriptorGridEntry.cs
- DataGridViewTextBoxColumn.cs
- CodeBinaryOperatorExpression.cs
- ConditionalExpression.cs
- ClientTargetCollection.cs
- HostProtectionPermission.cs
- ImplicitInputBrush.cs
- WhitespaceSignificantCollectionAttribute.cs
- ProtocolElementCollection.cs
- VirtualizedContainerService.cs
- TransactionValidationBehavior.cs
- Track.cs
- EmptyQuery.cs
- WebPartMinimizeVerb.cs
- SpecialFolderEnumConverter.cs
- DataServiceConfiguration.cs
- MsmqOutputChannel.cs
- ToolbarAUtomationPeer.cs
- CodeIdentifiers.cs
- RuleInfoComparer.cs
- BooleanFacetDescriptionElement.cs
- EventInfo.cs
- ScaleTransform.cs
- FillRuleValidation.cs
- Type.cs
- FileDialog.cs
- CommandBindingCollection.cs
- DefaultAutoFieldGenerator.cs
- PerformanceCounter.cs
- LinqDataSourceView.cs
- Types.cs
- Label.cs
- SmtpSection.cs
- CapabilitiesPattern.cs
- ContentWrapperAttribute.cs
- MailMessage.cs
- ContainerVisual.cs
- SiteOfOriginContainer.cs
- RestHandler.cs
- StateMachineWorkflowInstance.cs
- SqlUnionizer.cs
- ToolZone.cs
- ColorBlend.cs
- VBIdentifierName.cs
- InfiniteTimeSpanConverter.cs
- EventLogReader.cs