Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / WinForms / Managed / System / WinForms / CheckBox.cs / 1 / CheckBox.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Runtime.Serialization.Formatters; using System.Runtime.Remoting; using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.Security.Permissions; using System.Windows.Forms.ButtonInternal; using System.ComponentModel; using System.ComponentModel.Design; using System.Windows.Forms.Layout; using System.Drawing; using System.Windows.Forms.Internal; using System.Drawing.Drawing2D; using Microsoft.Win32; using System.Globalization; ////// /// [ ComVisible(true), ClassInterface(ClassInterfaceType.AutoDispatch), DefaultProperty("Checked"), DefaultEvent("CheckedChanged"), DefaultBindingProperty("CheckState"), ToolboxItem("System.Windows.Forms.Design.AutoSizeToolboxItem," + AssemblyRef.SystemDesign), SRDescription(SR.DescriptionCheckBox) ] public class CheckBox : ButtonBase { private static readonly object EVENT_CHECKEDCHANGED = new object(); private static readonly object EVENT_CHECKSTATECHANGED = new object(); private static readonly object EVENT_APPEARANCECHANGED = new object(); static readonly ContentAlignment anyRight = ContentAlignment.TopRight | ContentAlignment.MiddleRight | ContentAlignment.BottomRight; private bool autoCheck; private bool threeState; private bool accObjDoDefaultAction = false; private ContentAlignment checkAlign = ContentAlignment.MiddleLeft; private CheckState checkState; private Appearance appearance; ///Represents a Windows /// check box. ////// /// public CheckBox() : base() { // Checkboxes shouldn't respond to right clicks, so we need to do all our own click logic SetStyle(ControlStyles.StandardClick | ControlStyles.StandardDoubleClick, false); SetAutoSizeMode(AutoSizeMode.GrowAndShrink); autoCheck = true; TextAlign = ContentAlignment.MiddleLeft; } private bool AccObjDoDefaultAction { get { return this.accObjDoDefaultAction; } set { this.accObjDoDefaultAction = value; } } ////// Initializes a new instance of the ///class. /// /// /// [ DefaultValue(Appearance.Normal), Localizable(true), SRCategory(SR.CatAppearance), SRDescription(SR.CheckBoxAppearanceDescr) ] public Appearance Appearance { get { return appearance; } set { //valid values are 0x0 to 0x1 if (!ClientUtils.IsEnumValid(value, (int)value, (int)Appearance.Normal, (int)Appearance.Button)){ throw new InvalidEnumArgumentException("value", (int)value, typeof(Appearance)); } if (appearance != value) { using (LayoutTransaction.CreateTransactionIf(AutoSize, this.ParentInternal, this, PropertyNames.Appearance)) { appearance = value; if (OwnerDraw) { Refresh(); } else { UpdateStyles(); } OnAppearanceChanged(EventArgs.Empty); } } } } ///Gets /// or sets the value that determines the appearance of a /// check box control. ////// /// [SRCategory(SR.CatPropertyChanged), SRDescription(SR.CheckBoxOnAppearanceChangedDescr)] public event EventHandler AppearanceChanged { add { Events.AddHandler(EVENT_APPEARANCECHANGED, value); } remove { Events.RemoveHandler(EVENT_APPEARANCECHANGED, value); } } ///[To be supplied.] ////// /// [ DefaultValue(true), SRCategory(SR.CatBehavior), SRDescription(SR.CheckBoxAutoCheckDescr) ] public bool AutoCheck { get { return autoCheck; } set { autoCheck = value; } } ///Gets or sets a value indicating whether the ///or /// value and the check box's appearance are automatically /// changed when it is clicked. /// /// [ Bindable(true), Localizable(true), SRCategory(SR.CatAppearance), DefaultValue(ContentAlignment.MiddleLeft), SRDescription(SR.CheckBoxCheckAlignDescr) ] public ContentAlignment CheckAlign { get { return checkAlign; } set { if (!WindowsFormsUtils.EnumValidator.IsValidContentAlignment(value)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(ContentAlignment)); } if (checkAlign != value) { checkAlign = value; LayoutTransaction.DoLayoutIf(AutoSize, ParentInternal, this, PropertyNames.CheckAlign); if (OwnerDraw) { Invalidate(); } else { UpdateStyles(); } } } } ////// Gets or sets /// the horizontal and vertical alignment of a check box on a check box /// control. /// /// ////// /// [ Bindable(true), SettingsBindable(true), DefaultValue(false), SRCategory(SR.CatAppearance), RefreshProperties(RefreshProperties.All), SRDescription(SR.CheckBoxCheckedDescr) ] public bool Checked { get { return checkState != CheckState.Unchecked; } set { if (value != Checked) { CheckState = value ? CheckState.Checked : CheckState.Unchecked; } } } ////// Gets /// or sets a value indicating whether the /// check box /// is checked. /// ////// /// [ Bindable(true), SRCategory(SR.CatAppearance), DefaultValue(CheckState.Unchecked), RefreshProperties(RefreshProperties.All), SRDescription(SR.CheckBoxCheckStateDescr) ] public CheckState CheckState { get { return checkState; } set { // valid values are 0-2 inclusive. if (!ClientUtils.IsEnumValid(value, (int)value, (int)CheckState.Unchecked, (int)CheckState.Indeterminate)){ throw new InvalidEnumArgumentException("value", (int)value, typeof(CheckState)); } if (checkState != value) { bool oldChecked = Checked; checkState = value; if (IsHandleCreated) { SendMessage(NativeMethods.BM_SETCHECK, (int)checkState, 0); } if (oldChecked != Checked) { OnCheckedChanged(EventArgs.Empty); } OnCheckStateChanged(EventArgs.Empty); } } } ///Gets /// or sets a value indicating whether the check box is checked. ////// [Browsable(false), EditorBrowsable(EditorBrowsableState.Never)] public new event EventHandler DoubleClick { add { base.DoubleClick += value; } remove { base.DoubleClick -= value; } } /// /// [Browsable(false), EditorBrowsable(EditorBrowsableState.Never)] public new event MouseEventHandler MouseDoubleClick { add { base.MouseDoubleClick += value; } remove { base.MouseDoubleClick -= value; } } /// /// /// /// protected override CreateParams CreateParams { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] get { CreateParams cp = base.CreateParams; cp.ClassName = "BUTTON"; if (OwnerDraw) { cp.Style |= NativeMethods.BS_OWNERDRAW; } else { cp.Style |= NativeMethods.BS_3STATE; if (Appearance == Appearance.Button) { cp.Style |= NativeMethods.BS_PUSHLIKE; } // Determine the alignment of the check box // ContentAlignment align = RtlTranslateContent(CheckAlign); if ((int)(align & anyRight) != 0) { cp.Style |= NativeMethods.BS_RIGHTBUTTON; } } return cp; } } ////// Gets the information used to create the handle for the /// ////// control. /// /// /// Deriving classes can override this to configure a default size for their control. /// This is more efficient than setting the size in the control's constructor. /// protected override Size DefaultSize { get { return new Size(104, 24); } } internal override Size GetPreferredSizeCore(Size proposedConstraints) { if (Appearance == Appearance.Button) { ButtonStandardAdapter adapter = new ButtonStandardAdapter(this); return adapter.GetPreferredSizeCore(proposedConstraints); } if(FlatStyle != FlatStyle.System) { return base.GetPreferredSizeCore(proposedConstraints); } Size textSize = TextRenderer.MeasureText(this.Text, this.Font); Size size = SizeFromClientSize(textSize); size.Width += 25; size.Height += 5; return size + Padding.Size; } ////// /// /// internal override Rectangle OverChangeRectangle { get { if (Appearance == Appearance.Button) { return base.OverChangeRectangle; } else { if (FlatStyle == FlatStyle.Standard) { // this Rectangle will cause no Invalidation // can't use Rectangle.Empty because it will cause Invalidate(ClientRectangle) return new Rectangle(-1, -1, 1, 1); } else { // Popup mouseover rectangle is actually bigger than GetCheckmarkRectangle return Adapter.CommonLayout().Layout().checkBounds; } } } } ////// /// /// internal override Rectangle DownChangeRectangle { get { if (Appearance == Appearance.Button || FlatStyle == FlatStyle.System) { return base.DownChangeRectangle; } else { // Popup mouseover rectangle is actually bigger than GetCheckmarkRectangle() return Adapter.CommonLayout().Layout().checkBounds; } } } ////// /// /// [ Localizable(true), DefaultValue(ContentAlignment.MiddleLeft) ] public override ContentAlignment TextAlign { get { return base.TextAlign; } set { base.TextAlign = value; } } ////// Gets or sets a value indicating the alignment of the /// text on the checkbox control. /// /// ////// /// [ DefaultValue(false), SRCategory(SR.CatBehavior), SRDescription(SR.CheckBoxThreeStateDescr) ] public bool ThreeState { get { return threeState; } set { threeState = value; } } ///Gets or sets a value indicating /// whether the check box will allow three check states rather than two. ////// /// [SRDescription(SR.CheckBoxOnCheckedChangedDescr)] public event EventHandler CheckedChanged { add { Events.AddHandler(EVENT_CHECKEDCHANGED, value); } remove { Events.RemoveHandler(EVENT_CHECKEDCHANGED, value); } } ///Occurs when the /// value of the ////// property changes. /// /// [SRDescription(SR.CheckBoxOnCheckStateChangedDescr)] public event EventHandler CheckStateChanged { add { Events.AddHandler(EVENT_CHECKSTATECHANGED, value); } remove { Events.RemoveHandler(EVENT_CHECKSTATECHANGED, value); } } ///Occurs when the /// value of the ////// property changes. /// /// /// protected override AccessibleObject CreateAccessibilityInstance() { return new CheckBoxAccessibleObject(this); } ////// Constructs the new instance of the accessibility object for this control. Subclasses /// should not call base.CreateAccessibilityObject. /// ////// /// protected virtual void OnAppearanceChanged(EventArgs e) { EventHandler eh = Events[EVENT_APPEARANCECHANGED] as EventHandler; if (eh != null) { eh(this, e); } } ///[To be supplied.] ////// /// protected virtual void OnCheckedChanged(EventArgs e) { // accessibility stuff if (this.FlatStyle == FlatStyle.System) { AccessibilityNotifyClients(AccessibleEvents.SystemCaptureStart, -1); } AccessibilityNotifyClients(AccessibleEvents.StateChange, -1); AccessibilityNotifyClients(AccessibleEvents.NameChange, -1); if (this.FlatStyle == FlatStyle.System) { AccessibilityNotifyClients(AccessibleEvents.SystemCaptureEnd, -1); } EventHandler handler = (EventHandler)Events[EVENT_CHECKEDCHANGED]; if (handler != null) handler(this,e); } ///Raises the ////// event. /// /// protected virtual void OnCheckStateChanged(EventArgs e) { if (OwnerDraw) { Refresh(); } EventHandler handler = (EventHandler)Events[EVENT_CHECKSTATECHANGED]; if (handler != null) handler(this,e); } ///Raises the ///event. /// /// /// protected override void OnClick(EventArgs e) { if (autoCheck) { switch (CheckState) { case CheckState.Unchecked: CheckState = CheckState.Checked; break; case CheckState.Checked: if (threeState) { CheckState = CheckState.Indeterminate; // If the check box is clicked as a result of AccObj::DoDefaultAction // then the native check box does not fire OBJ_STATE_CHANGE event when going to Indeterminate state. // So the WinForms layer fires the OBJ_STATE_CHANGE event. // vsw 543351. if (this.AccObjDoDefaultAction) { AccessibilityNotifyClients(AccessibleEvents.StateChange, -1); } } else { CheckState = CheckState.Unchecked; } break; default: CheckState = CheckState.Unchecked; break; } } base.OnClick(e); } ////// Fires the event indicating that the control has been clicked. /// Inheriting controls should use this in favour of actually listening to /// the event, but should not forget to call base.onClicked() to /// ensure that the event is still fired for external listeners. /// /// ////// /// We override this to ensure that the control's click values are set up /// correctly. /// ///protected override void OnHandleCreated(EventArgs e) { base.OnHandleCreated(e); // Since this is a protected override... // this can be directly called in by a overriden class.. // and the Handle need not be created... // So Check for the handle if (IsHandleCreated) { SendMessage(NativeMethods.BM_SETCHECK, (int)checkState, 0); } } /// /// We override this to ensure that press '+' or '=' checks the box, /// while pressing '-' unchecks the box /// ///protected override void OnKeyDown(KeyEventArgs e) { //this fixes bug 235019, but it will be a breaking change from Everett //see the comments attached to bug 235019 /* if (Enabled) { if (e.KeyCode == Keys.Oemplus || e.KeyCode == Keys.Add) { CheckState = CheckState.Checked; } if (e.KeyCode == Keys.OemMinus || e.KeyCode == Keys.Subtract) { CheckState = CheckState.Unchecked; } } */ base.OnKeyDown(e); } /// /// /// /// protected override void OnMouseUp(MouseEventArgs mevent) { if (mevent.Button == MouseButtons.Left && MouseIsPressed) { // It's best not to have the mouse captured while running Click events if (base.MouseIsDown) { Point pt = PointToScreen(new Point(mevent.X, mevent.Y)); if (UnsafeNativeMethods.WindowFromPoint(pt.X, pt.Y) == Handle) { //Paint in raised state... ResetFlagsandPaint(); if (!ValidationCancelled) { if (this.Capture) { OnClick(mevent); } OnMouseClick(mevent); } } } } base.OnMouseUp(mevent); } internal override ButtonBaseAdapter CreateFlatAdapter() { return new CheckBoxFlatAdapter(this); } internal override ButtonBaseAdapter CreatePopupAdapter() { return new CheckBoxPopupAdapter(this); } internal override ButtonBaseAdapter CreateStandardAdapter() { return new CheckBoxStandardAdapter(this); } ////// Raises the ///event. /// /// /// /// Overridden to handle mnemonics properly. /// ///[UIPermission(SecurityAction.LinkDemand, Window=UIPermissionWindow.AllWindows)] protected internal override bool ProcessMnemonic(char charCode) { if (UseMnemonic && IsMnemonic(charCode, Text) && CanSelect) { if (FocusInternal()) { //Paint in raised state... // ResetFlagsandPaint(); if (!ValidationCancelled) { OnClick(EventArgs.Empty); } } return true; } return false; } /// /// /// Provides some interesting information for the CheckBox control in /// String form. /// ///public override string ToString() { string s = base.ToString(); // C#R cpb: 14744 ([....]) We shouldn't need to convert the enum to int -- EE M10 workitem. int checkState = (int)CheckState; return s + ", CheckState: " + checkState.ToString(CultureInfo.InvariantCulture); } /// /// /// /// [System.Runtime.InteropServices.ComVisible(true)] public class CheckBoxAccessibleObject : ButtonBaseAccessibleObject { ////// /// public CheckBoxAccessibleObject(Control owner) : base(owner) { } ///[To be supplied.] ////// /// public override string DefaultAction { get { string defaultAction = Owner.AccessibleDefaultActionDescription; if (defaultAction != null) { return defaultAction; } if (((CheckBox)Owner).Checked) { return SR.GetString(SR.AccessibleActionUncheck); } else { return SR.GetString(SR.AccessibleActionCheck); } } } ///[To be supplied.] ////// /// public override AccessibleRole Role { get { AccessibleRole role = Owner.AccessibleRole; if (role != AccessibleRole.Default) { return role; } return AccessibleRole.CheckButton; } } ///[To be supplied.] ////// /// public override AccessibleStates State { get { switch (((CheckBox)Owner).CheckState) { case CheckState.Checked: return AccessibleStates.Checked | base.State; case CheckState.Indeterminate: return AccessibleStates.Indeterminate | base.State; } return base.State; } } ///[To be supplied.] ////// /// [SecurityPermission(SecurityAction.Demand, Flags = SecurityPermissionFlag.UnmanagedCode)] public override void DoDefaultAction() { CheckBox cb = this.Owner as CheckBox; if (cb != null) { cb.AccObjDoDefaultAction = true; } try { base.DoDefaultAction(); } finally { if (cb != null) { cb.AccObjDoDefaultAction = false; } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Runtime.Serialization.Formatters; using System.Runtime.Remoting; using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.Security.Permissions; using System.Windows.Forms.ButtonInternal; using System.ComponentModel; using System.ComponentModel.Design; using System.Windows.Forms.Layout; using System.Drawing; using System.Windows.Forms.Internal; using System.Drawing.Drawing2D; using Microsoft.Win32; using System.Globalization; ////// /// [ ComVisible(true), ClassInterface(ClassInterfaceType.AutoDispatch), DefaultProperty("Checked"), DefaultEvent("CheckedChanged"), DefaultBindingProperty("CheckState"), ToolboxItem("System.Windows.Forms.Design.AutoSizeToolboxItem," + AssemblyRef.SystemDesign), SRDescription(SR.DescriptionCheckBox) ] public class CheckBox : ButtonBase { private static readonly object EVENT_CHECKEDCHANGED = new object(); private static readonly object EVENT_CHECKSTATECHANGED = new object(); private static readonly object EVENT_APPEARANCECHANGED = new object(); static readonly ContentAlignment anyRight = ContentAlignment.TopRight | ContentAlignment.MiddleRight | ContentAlignment.BottomRight; private bool autoCheck; private bool threeState; private bool accObjDoDefaultAction = false; private ContentAlignment checkAlign = ContentAlignment.MiddleLeft; private CheckState checkState; private Appearance appearance; ///Represents a Windows /// check box. ////// /// public CheckBox() : base() { // Checkboxes shouldn't respond to right clicks, so we need to do all our own click logic SetStyle(ControlStyles.StandardClick | ControlStyles.StandardDoubleClick, false); SetAutoSizeMode(AutoSizeMode.GrowAndShrink); autoCheck = true; TextAlign = ContentAlignment.MiddleLeft; } private bool AccObjDoDefaultAction { get { return this.accObjDoDefaultAction; } set { this.accObjDoDefaultAction = value; } } ////// Initializes a new instance of the ///class. /// /// /// [ DefaultValue(Appearance.Normal), Localizable(true), SRCategory(SR.CatAppearance), SRDescription(SR.CheckBoxAppearanceDescr) ] public Appearance Appearance { get { return appearance; } set { //valid values are 0x0 to 0x1 if (!ClientUtils.IsEnumValid(value, (int)value, (int)Appearance.Normal, (int)Appearance.Button)){ throw new InvalidEnumArgumentException("value", (int)value, typeof(Appearance)); } if (appearance != value) { using (LayoutTransaction.CreateTransactionIf(AutoSize, this.ParentInternal, this, PropertyNames.Appearance)) { appearance = value; if (OwnerDraw) { Refresh(); } else { UpdateStyles(); } OnAppearanceChanged(EventArgs.Empty); } } } } ///Gets /// or sets the value that determines the appearance of a /// check box control. ////// /// [SRCategory(SR.CatPropertyChanged), SRDescription(SR.CheckBoxOnAppearanceChangedDescr)] public event EventHandler AppearanceChanged { add { Events.AddHandler(EVENT_APPEARANCECHANGED, value); } remove { Events.RemoveHandler(EVENT_APPEARANCECHANGED, value); } } ///[To be supplied.] ////// /// [ DefaultValue(true), SRCategory(SR.CatBehavior), SRDescription(SR.CheckBoxAutoCheckDescr) ] public bool AutoCheck { get { return autoCheck; } set { autoCheck = value; } } ///Gets or sets a value indicating whether the ///or /// value and the check box's appearance are automatically /// changed when it is clicked. /// /// [ Bindable(true), Localizable(true), SRCategory(SR.CatAppearance), DefaultValue(ContentAlignment.MiddleLeft), SRDescription(SR.CheckBoxCheckAlignDescr) ] public ContentAlignment CheckAlign { get { return checkAlign; } set { if (!WindowsFormsUtils.EnumValidator.IsValidContentAlignment(value)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(ContentAlignment)); } if (checkAlign != value) { checkAlign = value; LayoutTransaction.DoLayoutIf(AutoSize, ParentInternal, this, PropertyNames.CheckAlign); if (OwnerDraw) { Invalidate(); } else { UpdateStyles(); } } } } ////// Gets or sets /// the horizontal and vertical alignment of a check box on a check box /// control. /// /// ////// /// [ Bindable(true), SettingsBindable(true), DefaultValue(false), SRCategory(SR.CatAppearance), RefreshProperties(RefreshProperties.All), SRDescription(SR.CheckBoxCheckedDescr) ] public bool Checked { get { return checkState != CheckState.Unchecked; } set { if (value != Checked) { CheckState = value ? CheckState.Checked : CheckState.Unchecked; } } } ////// Gets /// or sets a value indicating whether the /// check box /// is checked. /// ////// /// [ Bindable(true), SRCategory(SR.CatAppearance), DefaultValue(CheckState.Unchecked), RefreshProperties(RefreshProperties.All), SRDescription(SR.CheckBoxCheckStateDescr) ] public CheckState CheckState { get { return checkState; } set { // valid values are 0-2 inclusive. if (!ClientUtils.IsEnumValid(value, (int)value, (int)CheckState.Unchecked, (int)CheckState.Indeterminate)){ throw new InvalidEnumArgumentException("value", (int)value, typeof(CheckState)); } if (checkState != value) { bool oldChecked = Checked; checkState = value; if (IsHandleCreated) { SendMessage(NativeMethods.BM_SETCHECK, (int)checkState, 0); } if (oldChecked != Checked) { OnCheckedChanged(EventArgs.Empty); } OnCheckStateChanged(EventArgs.Empty); } } } ///Gets /// or sets a value indicating whether the check box is checked. ////// [Browsable(false), EditorBrowsable(EditorBrowsableState.Never)] public new event EventHandler DoubleClick { add { base.DoubleClick += value; } remove { base.DoubleClick -= value; } } /// /// [Browsable(false), EditorBrowsable(EditorBrowsableState.Never)] public new event MouseEventHandler MouseDoubleClick { add { base.MouseDoubleClick += value; } remove { base.MouseDoubleClick -= value; } } /// /// /// /// protected override CreateParams CreateParams { [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] get { CreateParams cp = base.CreateParams; cp.ClassName = "BUTTON"; if (OwnerDraw) { cp.Style |= NativeMethods.BS_OWNERDRAW; } else { cp.Style |= NativeMethods.BS_3STATE; if (Appearance == Appearance.Button) { cp.Style |= NativeMethods.BS_PUSHLIKE; } // Determine the alignment of the check box // ContentAlignment align = RtlTranslateContent(CheckAlign); if ((int)(align & anyRight) != 0) { cp.Style |= NativeMethods.BS_RIGHTBUTTON; } } return cp; } } ////// Gets the information used to create the handle for the /// ////// control. /// /// /// Deriving classes can override this to configure a default size for their control. /// This is more efficient than setting the size in the control's constructor. /// protected override Size DefaultSize { get { return new Size(104, 24); } } internal override Size GetPreferredSizeCore(Size proposedConstraints) { if (Appearance == Appearance.Button) { ButtonStandardAdapter adapter = new ButtonStandardAdapter(this); return adapter.GetPreferredSizeCore(proposedConstraints); } if(FlatStyle != FlatStyle.System) { return base.GetPreferredSizeCore(proposedConstraints); } Size textSize = TextRenderer.MeasureText(this.Text, this.Font); Size size = SizeFromClientSize(textSize); size.Width += 25; size.Height += 5; return size + Padding.Size; } ////// /// /// internal override Rectangle OverChangeRectangle { get { if (Appearance == Appearance.Button) { return base.OverChangeRectangle; } else { if (FlatStyle == FlatStyle.Standard) { // this Rectangle will cause no Invalidation // can't use Rectangle.Empty because it will cause Invalidate(ClientRectangle) return new Rectangle(-1, -1, 1, 1); } else { // Popup mouseover rectangle is actually bigger than GetCheckmarkRectangle return Adapter.CommonLayout().Layout().checkBounds; } } } } ////// /// /// internal override Rectangle DownChangeRectangle { get { if (Appearance == Appearance.Button || FlatStyle == FlatStyle.System) { return base.DownChangeRectangle; } else { // Popup mouseover rectangle is actually bigger than GetCheckmarkRectangle() return Adapter.CommonLayout().Layout().checkBounds; } } } ////// /// /// [ Localizable(true), DefaultValue(ContentAlignment.MiddleLeft) ] public override ContentAlignment TextAlign { get { return base.TextAlign; } set { base.TextAlign = value; } } ////// Gets or sets a value indicating the alignment of the /// text on the checkbox control. /// /// ////// /// [ DefaultValue(false), SRCategory(SR.CatBehavior), SRDescription(SR.CheckBoxThreeStateDescr) ] public bool ThreeState { get { return threeState; } set { threeState = value; } } ///Gets or sets a value indicating /// whether the check box will allow three check states rather than two. ////// /// [SRDescription(SR.CheckBoxOnCheckedChangedDescr)] public event EventHandler CheckedChanged { add { Events.AddHandler(EVENT_CHECKEDCHANGED, value); } remove { Events.RemoveHandler(EVENT_CHECKEDCHANGED, value); } } ///Occurs when the /// value of the ////// property changes. /// /// [SRDescription(SR.CheckBoxOnCheckStateChangedDescr)] public event EventHandler CheckStateChanged { add { Events.AddHandler(EVENT_CHECKSTATECHANGED, value); } remove { Events.RemoveHandler(EVENT_CHECKSTATECHANGED, value); } } ///Occurs when the /// value of the ////// property changes. /// /// /// protected override AccessibleObject CreateAccessibilityInstance() { return new CheckBoxAccessibleObject(this); } ////// Constructs the new instance of the accessibility object for this control. Subclasses /// should not call base.CreateAccessibilityObject. /// ////// /// protected virtual void OnAppearanceChanged(EventArgs e) { EventHandler eh = Events[EVENT_APPEARANCECHANGED] as EventHandler; if (eh != null) { eh(this, e); } } ///[To be supplied.] ////// /// protected virtual void OnCheckedChanged(EventArgs e) { // accessibility stuff if (this.FlatStyle == FlatStyle.System) { AccessibilityNotifyClients(AccessibleEvents.SystemCaptureStart, -1); } AccessibilityNotifyClients(AccessibleEvents.StateChange, -1); AccessibilityNotifyClients(AccessibleEvents.NameChange, -1); if (this.FlatStyle == FlatStyle.System) { AccessibilityNotifyClients(AccessibleEvents.SystemCaptureEnd, -1); } EventHandler handler = (EventHandler)Events[EVENT_CHECKEDCHANGED]; if (handler != null) handler(this,e); } ///Raises the ////// event. /// /// protected virtual void OnCheckStateChanged(EventArgs e) { if (OwnerDraw) { Refresh(); } EventHandler handler = (EventHandler)Events[EVENT_CHECKSTATECHANGED]; if (handler != null) handler(this,e); } ///Raises the ///event. /// /// /// protected override void OnClick(EventArgs e) { if (autoCheck) { switch (CheckState) { case CheckState.Unchecked: CheckState = CheckState.Checked; break; case CheckState.Checked: if (threeState) { CheckState = CheckState.Indeterminate; // If the check box is clicked as a result of AccObj::DoDefaultAction // then the native check box does not fire OBJ_STATE_CHANGE event when going to Indeterminate state. // So the WinForms layer fires the OBJ_STATE_CHANGE event. // vsw 543351. if (this.AccObjDoDefaultAction) { AccessibilityNotifyClients(AccessibleEvents.StateChange, -1); } } else { CheckState = CheckState.Unchecked; } break; default: CheckState = CheckState.Unchecked; break; } } base.OnClick(e); } ////// Fires the event indicating that the control has been clicked. /// Inheriting controls should use this in favour of actually listening to /// the event, but should not forget to call base.onClicked() to /// ensure that the event is still fired for external listeners. /// /// ////// /// We override this to ensure that the control's click values are set up /// correctly. /// ///protected override void OnHandleCreated(EventArgs e) { base.OnHandleCreated(e); // Since this is a protected override... // this can be directly called in by a overriden class.. // and the Handle need not be created... // So Check for the handle if (IsHandleCreated) { SendMessage(NativeMethods.BM_SETCHECK, (int)checkState, 0); } } /// /// We override this to ensure that press '+' or '=' checks the box, /// while pressing '-' unchecks the box /// ///protected override void OnKeyDown(KeyEventArgs e) { //this fixes bug 235019, but it will be a breaking change from Everett //see the comments attached to bug 235019 /* if (Enabled) { if (e.KeyCode == Keys.Oemplus || e.KeyCode == Keys.Add) { CheckState = CheckState.Checked; } if (e.KeyCode == Keys.OemMinus || e.KeyCode == Keys.Subtract) { CheckState = CheckState.Unchecked; } } */ base.OnKeyDown(e); } /// /// /// /// protected override void OnMouseUp(MouseEventArgs mevent) { if (mevent.Button == MouseButtons.Left && MouseIsPressed) { // It's best not to have the mouse captured while running Click events if (base.MouseIsDown) { Point pt = PointToScreen(new Point(mevent.X, mevent.Y)); if (UnsafeNativeMethods.WindowFromPoint(pt.X, pt.Y) == Handle) { //Paint in raised state... ResetFlagsandPaint(); if (!ValidationCancelled) { if (this.Capture) { OnClick(mevent); } OnMouseClick(mevent); } } } } base.OnMouseUp(mevent); } internal override ButtonBaseAdapter CreateFlatAdapter() { return new CheckBoxFlatAdapter(this); } internal override ButtonBaseAdapter CreatePopupAdapter() { return new CheckBoxPopupAdapter(this); } internal override ButtonBaseAdapter CreateStandardAdapter() { return new CheckBoxStandardAdapter(this); } ////// Raises the ///event. /// /// /// /// Overridden to handle mnemonics properly. /// ///[UIPermission(SecurityAction.LinkDemand, Window=UIPermissionWindow.AllWindows)] protected internal override bool ProcessMnemonic(char charCode) { if (UseMnemonic && IsMnemonic(charCode, Text) && CanSelect) { if (FocusInternal()) { //Paint in raised state... // ResetFlagsandPaint(); if (!ValidationCancelled) { OnClick(EventArgs.Empty); } } return true; } return false; } /// /// /// Provides some interesting information for the CheckBox control in /// String form. /// ///public override string ToString() { string s = base.ToString(); // C#R cpb: 14744 ([....]) We shouldn't need to convert the enum to int -- EE M10 workitem. int checkState = (int)CheckState; return s + ", CheckState: " + checkState.ToString(CultureInfo.InvariantCulture); } /// /// /// /// [System.Runtime.InteropServices.ComVisible(true)] public class CheckBoxAccessibleObject : ButtonBaseAccessibleObject { ////// /// public CheckBoxAccessibleObject(Control owner) : base(owner) { } ///[To be supplied.] ////// /// public override string DefaultAction { get { string defaultAction = Owner.AccessibleDefaultActionDescription; if (defaultAction != null) { return defaultAction; } if (((CheckBox)Owner).Checked) { return SR.GetString(SR.AccessibleActionUncheck); } else { return SR.GetString(SR.AccessibleActionCheck); } } } ///[To be supplied.] ////// /// public override AccessibleRole Role { get { AccessibleRole role = Owner.AccessibleRole; if (role != AccessibleRole.Default) { return role; } return AccessibleRole.CheckButton; } } ///[To be supplied.] ////// /// public override AccessibleStates State { get { switch (((CheckBox)Owner).CheckState) { case CheckState.Checked: return AccessibleStates.Checked | base.State; case CheckState.Indeterminate: return AccessibleStates.Indeterminate | base.State; } return base.State; } } ///[To be supplied.] ////// /// [SecurityPermission(SecurityAction.Demand, Flags = SecurityPermissionFlag.UnmanagedCode)] public override void DoDefaultAction() { CheckBox cb = this.Owner as CheckBox; if (cb != null) { cb.AccObjDoDefaultAction = true; } try { base.DoDefaultAction(); } finally { if (cb != null) { cb.AccObjDoDefaultAction = false; } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
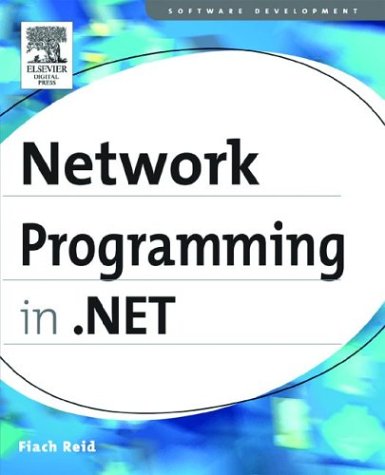
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ChangeBlockUndoRecord.cs
- EntityDataSourceContextCreatingEventArgs.cs
- GridViewSelectEventArgs.cs
- DebugTraceHelper.cs
- TextEditorLists.cs
- DataKeyArray.cs
- TimelineGroup.cs
- SiteMapProvider.cs
- QilUnary.cs
- NetSectionGroup.cs
- BooleanExpr.cs
- DataSpaceManager.cs
- MenuItemCollection.cs
- AccessDataSource.cs
- Site.cs
- SmtpSection.cs
- XmlILStorageConverter.cs
- PointUtil.cs
- Stream.cs
- VisualStyleRenderer.cs
- VectorCollectionConverter.cs
- SelectionChangedEventArgs.cs
- TrustManager.cs
- ResourcesBuildProvider.cs
- DrawListViewSubItemEventArgs.cs
- Receive.cs
- GeometryGroup.cs
- MeasurementDCInfo.cs
- ToolBarButtonClickEvent.cs
- FlowchartDesignerCommands.cs
- VariableQuery.cs
- SrgsRulesCollection.cs
- AssemblyResolver.cs
- BufferBuilder.cs
- UserThread.cs
- TableLayoutSettings.cs
- JavaScriptObjectDeserializer.cs
- HtmlElementCollection.cs
- ReadOnlyHierarchicalDataSourceView.cs
- Semaphore.cs
- SessionStateSection.cs
- WorkflowPrinting.cs
- unsafenativemethodstextservices.cs
- InputLanguageSource.cs
- PagesChangedEventArgs.cs
- AssemblyCollection.cs
- ImageButton.cs
- ArrayHelper.cs
- RoutedCommand.cs
- XmlDataImplementation.cs
- XhtmlBasicTextViewAdapter.cs
- SchemaMerger.cs
- ControlCollection.cs
- UIElementParagraph.cs
- StickyNoteHelper.cs
- MaskInputRejectedEventArgs.cs
- ComponentCollection.cs
- SurrogateChar.cs
- PngBitmapDecoder.cs
- PersonalizablePropertyEntry.cs
- securitymgrsite.cs
- AddInEnvironment.cs
- MLangCodePageEncoding.cs
- ObjectDataProvider.cs
- NamespaceList.cs
- ObjectSecurity.cs
- ProgressBarHighlightConverter.cs
- IdentifierElement.cs
- DependencyPropertyAttribute.cs
- DocumentGrid.cs
- Rect.cs
- ConfigurationElementCollection.cs
- ScriptingRoleServiceSection.cs
- ArgumentOutOfRangeException.cs
- ApplicationHost.cs
- InputLanguageCollection.cs
- WebEventCodes.cs
- StronglyTypedResourceBuilder.cs
- EncodingDataItem.cs
- ArithmeticException.cs
- SerializerProvider.cs
- MapPathBasedVirtualPathProvider.cs
- CfgParser.cs
- ValueProviderWrapper.cs
- SelectionListDesigner.cs
- shaperfactoryquerycacheentry.cs
- WindowsComboBox.cs
- SqlParameter.cs
- MonthCalendar.cs
- HtmlInputSubmit.cs
- XPathScanner.cs
- EdmType.cs
- DispatcherSynchronizationContext.cs
- ObjectAnimationBase.cs
- Parser.cs
- RtfFormatStack.cs
- AccessDataSourceView.cs
- DelimitedListTraceListener.cs
- TextChange.cs
- SqlBuffer.cs