Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / WebControls / RadioButtonList.cs / 4 / RadioButtonList.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Globalization; using System.Web; using System.Web.UI; using System.Security.Permissions; ////// [ ValidationProperty("SelectedItem"), SupportsEventValidation, ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class RadioButtonList : ListControl, IRepeatInfoUser, INamingContainer, IPostBackDataHandler #if ORCAS , IItemPaginationInfo, IPaginationContainer #endif { RadioButton _controlToRepeat; private bool _cachedIsEnabled; private bool _cachedRegisterEnabled; private int _offset; #if ORCAS private int _itemCount; #endif public RadioButtonList() { _offset = 0; #if ORCAS _itemCount = -1; #endif } ///Generates a single-selection radio button group and /// defines its properties. ////// CellPadding property. /// The padding between each item. /// [ WebCategory("Layout"), DefaultValue(-1), WebSysDescription(SR.RadioButtonList_CellPadding) ] public virtual int CellPadding { get { if (ControlStyleCreated == false) { return -1; } return ((TableStyle)ControlStyle).CellPadding; } set { ((TableStyle)ControlStyle).CellPadding = value; } } ////// CellSpacing property. /// The spacing between each item. /// [ WebCategory("Layout"), DefaultValue(-1), WebSysDescription(SR.RadioButtonList_CellSpacing) ] public virtual int CellSpacing { get { if (ControlStyleCreated == false) { return -1; } return ((TableStyle)ControlStyle).CellSpacing; } set { ((TableStyle)ControlStyle).CellSpacing = value; } } private RadioButton ControlToRepeat { get { if (_controlToRepeat != null) return _controlToRepeat; _controlToRepeat = new RadioButton(); _controlToRepeat.EnableViewState = false; Controls.Add(_controlToRepeat); // A // Apply properties that are the same for each radio button _controlToRepeat.AutoPostBack = AutoPostBack; _controlToRepeat.CausesValidation = CausesValidation; _controlToRepeat.ValidationGroup = ValidationGroup; return _controlToRepeat; } } ////// Indicates the column count of radio buttons /// within the group. /// [ WebCategory("Layout"), DefaultValue(0), WebSysDescription(SR.RadioButtonList_RepeatColumns) ] public virtual int RepeatColumns { get { object o = ViewState["RepeatColumns"]; return((o == null) ? 0 : (int)o); } set { if (value < 0) { throw new ArgumentOutOfRangeException("value"); } ViewState["RepeatColumns"] = value; } } ////// [ WebCategory("Layout"), DefaultValue(RepeatDirection.Vertical), WebSysDescription(SR.Item_RepeatDirection) ] public virtual RepeatDirection RepeatDirection { get { object o = ViewState["RepeatDirection"]; return((o == null) ? RepeatDirection.Vertical : (RepeatDirection)o); } set { if (value < RepeatDirection.Horizontal || value > RepeatDirection.Vertical) { throw new ArgumentOutOfRangeException("value"); } ViewState["RepeatDirection"] = value; } } ///Gets or sets the direction of flow of /// the radio buttons within the group. ////// [ WebCategory("Layout"), DefaultValue(RepeatLayout.Table), WebSysDescription(SR.WebControl_RepeatLayout) ] public virtual RepeatLayout RepeatLayout { get { object o = ViewState["RepeatLayout"]; return((o == null) ? RepeatLayout.Table : (RepeatLayout)o); } set { if (value < RepeatLayout.Table || value > RepeatLayout.Flow) { throw new ArgumentOutOfRangeException("value"); } ViewState["RepeatLayout"] = value; } } ///Indicates the layout of radio buttons within the /// group. ////// [ WebCategory("Appearance"), DefaultValue(TextAlign.Right), WebSysDescription(SR.WebControl_TextAlign) ] public virtual TextAlign TextAlign { get { object align = ViewState["TextAlign"]; return((align == null) ? TextAlign.Right : (TextAlign)align); } set { if (value < TextAlign.Left || value > TextAlign.Right) { throw new ArgumentOutOfRangeException("value"); } ViewState["TextAlign"] = value; } } ////// Indicates the label text alignment for the radio buttons within the group. ////// /// protected override Style CreateControlStyle() { return new TableStyle(ViewState); } ////// /// protected override Control FindControl(string id, int pathOffset) { return this; } #if ORCAS #region IItemPaginationInfo implementation int IItemPaginationInfo.FirstVisibleItemIndex { get { return this.FirstVisibleItemIndex; } } protected int FirstVisibleItemIndex { get { return _offset; } } ///Catches auto postback from a ///in the list. /// int IItemPaginationInfo.ItemCount { get { return ItemCount; } } ////// The number of items held by the container for pagination calculations. /// ////// protected virtual int ItemCount { get { EnsureDataBound(); return Items.Count; } } ////// The number of items held by the container for pagination calculations. /// ////// int IItemPaginationInfo.ItemWeight { get { return ItemWeight; } } ////// The weight for pagination calculations for each item of the container. /// Returns a constant value of 100 for RadioButtonList. /// ////// protected virtual int ItemWeight { get { return 100; } } int IItemPaginationInfo.VisibleItemCount { get { return this.VisibleItemCount; } } protected int VisibleItemCount { get { if (_itemCount >= 0) { return _itemCount; } else { return ItemCount; } } } ////// The weight for pagination calculations for each item of the container. /// Returns a constant value of 100 for RadioButtonList. /// ////// void IItemPaginationInfo.SetVisibleItems(int firstItem, int itemCount) { SetVisibleItems(firstItem, itemCount); } ////// Indicates which child items of the container are visible on the current page. /// ////// protected virtual void SetVisibleItems(int firstItem, int itemCount) { _offset = firstItem; _itemCount = itemCount; } #endregion #endif ////// Indicates which child items of the container are visible on the current page. /// ////// /// bool IPostBackDataHandler.LoadPostData(String postDataKey, NameValueCollection postCollection) { return LoadPostData(postDataKey, postCollection); } ///Loads the posted content of the list control if it is different from the last /// posting. ////// /// protected virtual bool LoadPostData(String postDataKey, NameValueCollection postCollection) { // When a RadioButtonList is disabled, then there is no postback data for it. // Since RadioButtonList doesn't call RegisterRequiresPostBack, this method will // never be called, so we don't need to worry about ignoring empty postback data. string post = postCollection[postDataKey]; int currentSelectedIndex = SelectedIndex; EnsureDataBound(); int n = Items.Count; for (int i=0; i < n; i++) { if (post == Items[i].Value && Items[i].Enabled) { ValidateEvent(postDataKey, post); if (i != currentSelectedIndex) { SetPostDataSelection(i); return true; } return false; } } return false; } ///Loads the posted content of the list control if it is different from the last /// posting. ////// /// void IPostBackDataHandler.RaisePostDataChangedEvent() { RaisePostDataChangedEvent(); } ///Invokes the OnSelectedIndexChanged /// method whenever posted data for the ////// control has changed. /// /// protected virtual void RaisePostDataChangedEvent() { if (AutoPostBack && Page != null && !Page.IsPostBackEventControlRegistered) { // Page.AutoPostBackControl = this; if (CausesValidation) { Page.Validate(ValidationGroup); } } OnSelectedIndexChanged(EventArgs.Empty); } protected internal override void Render(HtmlTextWriter writer) { #if ORCAS if (VisibleItemCount == 0) { return; } #else if (Items.Count == 0 && !EnableLegacyRendering) { return; } #endif RepeatInfo repeatInfo = new RepeatInfo(); Style style = (ControlStyleCreated ? ControlStyle : null); short tabIndex = TabIndex; bool undirtyTabIndex = false; // TabIndex here is special... it needs to be applied to the individual // radiobuttons and not the outer control itself // cache away the TabIndex property state ControlToRepeat.TabIndex = tabIndex; if (tabIndex != 0) { if (ViewState.IsItemDirty("TabIndex") == false) { undirtyTabIndex = true; } TabIndex = 0; } repeatInfo.RepeatColumns = RepeatColumns; repeatInfo.RepeatDirection = RepeatDirection; // If the device does not support tables, use the flow layout to render if (!DesignMode && !Context.Request.Browser.Tables) { repeatInfo.RepeatLayout = RepeatLayout.Flow; } else { repeatInfo.RepeatLayout = RepeatLayout; } if (repeatInfo.RepeatLayout == RepeatLayout.Flow) { repeatInfo.EnableLegacyRendering = EnableLegacyRendering; } repeatInfo.RenderRepeater(writer, (IRepeatInfoUser)this, style, this); if (Page != null) { Page.ClientScript.RegisterForEventValidation(UniqueID); } // restore the state of the TabIndex property if (tabIndex != 0) { TabIndex = tabIndex; } if (undirtyTabIndex) { ViewState.SetItemDirty("TabIndex", false); } } ///Invokes the OnSelectedIndexChanged /// method whenever posted data for the ////// control has changed. /// /// bool IRepeatInfoUser.HasFooter { get { return HasFooter; } } ////// /// protected virtual bool HasFooter { get { return false; } } ////// /// bool IRepeatInfoUser.HasHeader { get { return HasHeader; } } ////// /// protected virtual bool HasHeader { get { return false; } } ////// /// bool IRepeatInfoUser.HasSeparators { get { return HasSeparators; } } ////// /// protected virtual bool HasSeparators { get { return false; } } ////// /// int IRepeatInfoUser.RepeatedItemCount { get { return RepeatedItemCount; } } ////// /// protected virtual int RepeatedItemCount { get { #if ORCAS // If the whole control is contained on a single page, // the count is the number of items. if (VirtualStartPage == VirtualEndPage || _itemCount == -1) { return Items.Count; } return _itemCount; #else return (Items != null) ? Items.Count : 0; #endif } } ////// /// Style IRepeatInfoUser.GetItemStyle(ListItemType itemType, int repeatIndex) { return GetItemStyle(itemType, repeatIndex); } ////// /// protected virtual Style GetItemStyle(ListItemType itemType, int repeatIndex) { return null; } ////// /// Called by the RepeatInfo helper to render each item /// void IRepeatInfoUser.RenderItem(ListItemType itemType, int repeatIndex, RepeatInfo repeatInfo, HtmlTextWriter writer) { RenderItem(itemType, repeatIndex, repeatInfo, writer); } ////// /// Called by the RepeatInfo helper to render each item /// protected virtual void RenderItem(ListItemType itemType, int repeatIndex, RepeatInfo repeatInfo, HtmlTextWriter writer) { if (repeatIndex == 0) { _cachedIsEnabled = IsEnabled; _cachedRegisterEnabled = (Page != null) && (SaveSelectedIndicesViewState == false); } RadioButton controlToRepeat = ControlToRepeat; // Apply properties of the list items int repeatIndexOffset = repeatIndex + _offset; ListItem item = Items[repeatIndexOffset]; // controlToRepeat.Attributes.Clear(); if (item.HasAttributes) { foreach (string key in item.Attributes.Keys) { controlToRepeat.Attributes[key] = item.Attributes[key]; } } controlToRepeat.ID = repeatIndexOffset.ToString(NumberFormatInfo.InvariantInfo); controlToRepeat.Text = item.Text; controlToRepeat.Attributes["value"] = item.Value; controlToRepeat.Checked = item.Selected; controlToRepeat.Enabled = _cachedIsEnabled && item.Enabled; controlToRepeat.TextAlign = TextAlign; controlToRepeat.RenderControl(writer); if (controlToRepeat.Enabled && _cachedRegisterEnabled && Page != null) { // Store a client-side array of enabled control, so we can re-enable them on // postback (in case they are disabled client-side) // Postback is needed when SelectedIndices is not saved in view state Page.RegisterEnabledControl(controlToRepeat); } } #if ORCAS ///[ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public virtual int MaximumWeight { get { if (Page != null && Page.Form != null) { return Page.Form.MaximumWeight; } return 0; } } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
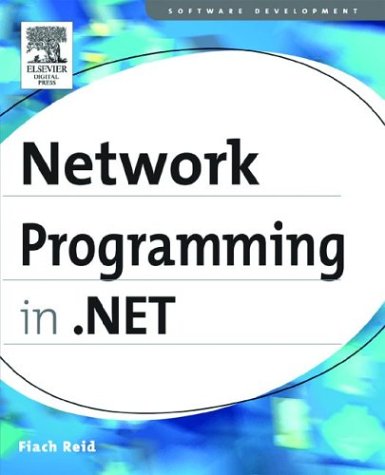
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Constants.cs
- ElementMarkupObject.cs
- DataObjectMethodAttribute.cs
- Rule.cs
- MergeEnumerator.cs
- TreeSet.cs
- SiteOfOriginPart.cs
- DataSourceControl.cs
- ReferencedType.cs
- BypassElementCollection.cs
- CultureMapper.cs
- UserControl.cs
- LinkArea.cs
- BindingsCollection.cs
- MergeLocalizationDirectives.cs
- NullRuntimeConfig.cs
- RootCodeDomSerializer.cs
- StylusCaptureWithinProperty.cs
- DocumentViewerBaseAutomationPeer.cs
- SqlStatistics.cs
- Types.cs
- ReferenceEqualityComparer.cs
- XmlDocument.cs
- SQLSingleStorage.cs
- _Connection.cs
- CodeValidator.cs
- UnknownBitmapDecoder.cs
- ETagAttribute.cs
- TemplatedMailWebEventProvider.cs
- XamlParser.cs
- TransactedReceiveScope.cs
- Hashtable.cs
- XmlNodeList.cs
- ApplicationDirectoryMembershipCondition.cs
- OpenTypeLayout.cs
- RectAnimationUsingKeyFrames.cs
- LinearGradientBrush.cs
- CrossSiteScriptingValidation.cs
- PersistChildrenAttribute.cs
- TextModifier.cs
- FileNameEditor.cs
- GCHandleCookieTable.cs
- OracleParameter.cs
- PrintController.cs
- PopupEventArgs.cs
- BitmapEffectDrawing.cs
- UserControlCodeDomTreeGenerator.cs
- StylusCaptureWithinProperty.cs
- ChannelBinding.cs
- LinqDataSourceDisposeEventArgs.cs
- InvokePatternIdentifiers.cs
- MatrixUtil.cs
- GridPatternIdentifiers.cs
- ExclusiveHandle.cs
- ServicesUtilities.cs
- CodeAttachEventStatement.cs
- NonSerializedAttribute.cs
- FormViewInsertEventArgs.cs
- ConnectionManagementElement.cs
- VisualStyleTypesAndProperties.cs
- CompilerLocalReference.cs
- WindowsMenu.cs
- ThreadSafeList.cs
- BoundingRectTracker.cs
- ExtendLockCommand.cs
- InstanceDataCollectionCollection.cs
- DBNull.cs
- WebPartConnectVerb.cs
- UIElementPropertyUndoUnit.cs
- HtmlElementCollection.cs
- WebPartCollection.cs
- Privilege.cs
- ToolBarOverflowPanel.cs
- ListControl.cs
- Knowncolors.cs
- RtfControls.cs
- SchemaNames.cs
- BitmapScalingModeValidation.cs
- MachineKeyConverter.cs
- ParameterBuilder.cs
- AnonymousIdentificationSection.cs
- Type.cs
- PropertyValueUIItem.cs
- RawStylusInput.cs
- Itemizer.cs
- CharAnimationUsingKeyFrames.cs
- XNodeSchemaApplier.cs
- Themes.cs
- OdbcPermission.cs
- __ConsoleStream.cs
- DesignerRegionMouseEventArgs.cs
- TraceListeners.cs
- EventEntry.cs
- PartialList.cs
- DataControlFieldHeaderCell.cs
- ImportDesigner.xaml.cs
- SqlLiftIndependentRowExpressions.cs
- ListSortDescriptionCollection.cs
- SamlEvidence.cs
- DependencyObjectProvider.cs