Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / DataOracleClient / System / Data / OracleClient / OracleParameter.cs / 1 / OracleParameter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Data.OracleClient { using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Data; using System.Data.Common; using System.Data.ProviderBase; using System.Data.SqlTypes; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; //--------------------------------------------------------------------- // OracleParameter // [ TypeConverterAttribute(typeof(System.Data.OracleClient.OracleParameter.OracleParameterConverter)) ] sealed public partial class OracleParameter : DbParameter, ICloneable, IDbDataParameter { private MetaType _metaType; // type information; only set when DbType or OracleType is set. private int _commandSetResult; // the ordinal result number for parameters in support of Command Set, 0 indicates it is not a Command Set result. private MetaType _coercedMetaType; private string _parameterName; private byte _precision; private byte _scale; private bool _hasScale; // Construct an "empty" parameter public OracleParameter() : base() {} // Construct from a parameter name and a value object public OracleParameter(string name, object value) { this.ParameterName = name; this.Value = value; } // Construct from a parameter name and a data type public OracleParameter(string name, OracleType oracleType) : this() { this.ParameterName = name; this.OracleType = oracleType; } // Construct from a parameter name, a data type and the size public OracleParameter(string name, OracleType oracleType, int size) : this() { this.ParameterName = name; this.OracleType = oracleType; this.Size = size; } // Construct from a parameter name, a data type, the size and the source column public OracleParameter( string name, OracleType oracleType, int size, string srcColumn ) : this() { this.ParameterName = name; this.OracleType = oracleType; this.Size = size; this.SourceColumn = srcColumn; } // Construct from everything but the kitchen sink public OracleParameter(string name, OracleType oracleType, int size, ParameterDirection direction, bool isNullable, byte precision, byte scale, string srcColumn, DataRowVersion srcVersion, object value) : this() { this.ParameterName = name; this.OracleType = oracleType; this.Size = size; this.Direction = direction; this.IsNullable = isNullable; PrecisionInternal = precision; ScaleInternal = scale; this.SourceColumn = srcColumn; this.SourceVersion = srcVersion; this.Value = value; } // V2.0 everything - round trip all browsable properties public OracleParameter(string name, OracleType oracleType, int size, ParameterDirection direction, string sourceColumn, DataRowVersion sourceVersion, bool sourceColumnNullMapping, object value) : this() { this.ParameterName = name; this.OracleType = oracleType; this.Size = size; this.Direction = direction; this.SourceColumn = sourceColumn; this.SourceVersion = sourceVersion; this.SourceColumnNullMapping = sourceColumnNullMapping; this.Value = value; } internal int BindSize { get { int size = GetActualSize(); // If the size of the value is huge and it's an input-only parameter, // we can try and figure out the binding size. if (short.MaxValue < size && ParameterDirection.Input == Direction) { size = ValueSize(GetCoercedValueInternal()); } return size; } } internal int CommandSetResult { get { return _commandSetResult; } set { _commandSetResult = value; } } override public DbType DbType { get { return GetMetaType().DbType; } set { if ((null == _metaType) || (_metaType.DbType != value)) { PropertyTypeChanging(); _metaType = MetaType.GetMetaTypeForType(value); } } } public override void ResetDbType() { ResetOracleType(); } [ DefaultValue(OracleType.VarChar), // MDAC 65862 ResCategoryAttribute(Res.OracleCategory_Data), RefreshProperties(RefreshProperties.All), ResDescriptionAttribute(Res.OracleParameter_OracleType), System.Data.Common.DbProviderSpecificTypePropertyAttribute(true), ] public OracleType OracleType { get { return GetMetaType().OracleType; } set { MetaType metatype = _metaType; if ((null == metatype) || (metatype.OracleType != value)) { PropertyTypeChanging(); _metaType = MetaType.GetMetaTypeForType(value); } } } public void ResetOracleType() { if (null != _metaType) { PropertyTypeChanging(); _metaType = null; } } [ ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbParameter_ParameterName), ] override public string ParameterName { // V1.2.3300, XXXParameter V1.0.3300 get { string parameterName = _parameterName; return ((null != parameterName) ? parameterName : ADP.StrEmpty); } set { if (_parameterName != value) { _parameterName = value; } } } [Browsable(false)] [EditorBrowsableAttribute(EditorBrowsableState.Never)] [Obsolete("Precision has been deprecated. Use the Math classes to explicitly set the precision of a decimal. http://go.microsoft.com/fwlink/?linkid=14202")] public Byte Precision { get { return PrecisionInternal; } set { PrecisionInternal = value; } } private byte PrecisionInternal { get { return _precision; } set { if (_precision != value) { _precision = value; } } } private bool ShouldSerializePrecision() { return (0 != _precision); } [Browsable(false)] [EditorBrowsableAttribute(EditorBrowsableState.Never)] [Obsolete("Scale has been deprecated. Use the Math classes to explicitly set the scale of a decimal. http://go.microsoft.com/fwlink/?linkid=14202")] public Byte Scale { get { return ScaleInternal; } set { ScaleInternal = value; } } private byte ScaleInternal { get { return _scale; } set { if (_scale != value || !_hasScale) { //PropertyChanging(); _scale = value; _hasScale = true; } } } private bool ShouldSerializeScale() { return _hasScale; } private static object CoerceValue(object value, MetaType destinationType) { Debug.Assert(null != destinationType, "null destinationType"); if ((null != value) && !Convert.IsDBNull(value) && (typeof(object) != destinationType.BaseType)) { Type currentType = value.GetType(); if ((currentType != destinationType.BaseType) && (currentType != destinationType.NoConvertType)) { try { if ((typeof(string) == destinationType.BaseType) && (typeof(char[]) == currentType)) { value = new string((char[])value); } else if ((DbType.Currency == destinationType.DbType) && (typeof(string) == currentType)) { value = Decimal.Parse((string)value, NumberStyles.Currency, (IFormatProvider)null); // WebData 99376 } else { value = Convert.ChangeType(value, destinationType.BaseType, (IFormatProvider)null); } } catch(Exception e) { // if (!ADP.IsCatchableExceptionType(e)) { throw; } throw ADP.ParameterConversionFailed(value, destinationType.BaseType, e); // WebData 75433 } } } return value; } object ICloneable.Clone() { return new OracleParameter(this); } private void CloneHelper(OracleParameter destination) { CloneHelperCore(destination); destination._metaType = _metaType; destination._parameterName = _parameterName; destination._precision = _precision; destination._scale = _scale; destination._hasScale = _hasScale; } internal int GetActualSize() { return ShouldSerializeSize() ? Size : ValueSize(CoercedValue); } private MetaType GetMetaType() { // if the user specifed a type, then return it's meta data return GetMetaType(Value); } internal MetaType GetMetaType(object value) { MetaType metaType = _metaType; if (null == metaType) { // if the didn't specify a type, but they specified a value, then // return the meta data for the value they specified if ((null != value) && !Convert.IsDBNull(value)) { metaType = MetaType.GetMetaTypeForObject(value); } else { // if they haven't specified anything, then return the default // meta data information metaType = MetaType.GetDefaultMetaType(); } _metaType = metaType; } return metaType; } internal object GetCoercedValueInternal() { object value = CoercedValue; // will also be set during parameter Validation if (null == value) { value = CoerceValue(this.Value, _coercedMetaType); CoercedValue = value; } return value; } private void PropertyChanging() { } private void PropertyTypeChanging() { PropertyChanging(); CoercedValue = null; } internal void SetCoercedValueInternal(object value, MetaType metaType) { _coercedMetaType = metaType; CoercedValue = CoerceValue(value, metaType); } private bool ShouldSerializeOracleType() { return (null != _metaType); } [ RefreshProperties(RefreshProperties.All), ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbParameter_Value), TypeConverterAttribute(typeof(StringConverter)), ] override public object Value { get { return _value; } set { _coercedValue = null; _value = value; } } private int ValueSize(object value) { if (value is OracleString) return ((OracleString) value).Length; if (value is string) return ((string) value).Length; if (value is char[]) return ((char[]) value).Length; if (value is OracleBinary) return ((OracleBinary) value).Length; return ValueSizeCore(value); } sealed internal class OracleParameterConverter : ExpandableObjectConverter { // converter classes should have public ctor public OracleParameterConverter() { } public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw ADP.ArgumentNull("destinationType"); } if (destinationType == typeof(InstanceDescriptor) && value is OracleParameter) { return ConvertToInstanceDescriptor(value as OracleParameter); } return base.ConvertTo(context, culture, value, destinationType); } private InstanceDescriptor ConvertToInstanceDescriptor(OracleParameter p) { // MDAC 67321 - reducing parameter generated code int flags = 0; // if part of the collection - the parametername can't be empty if (p.ShouldSerializeOracleType()) { flags |= 1; } if (p.ShouldSerializeSize()) { flags |= 2; } if (!ADP.IsEmpty(p.SourceColumn)) { flags |= 4; } if (null != p.Value) { flags |= 8; } if ((ParameterDirection.Input != p.Direction) || p.IsNullable || p.ShouldSerializePrecision() || p.ShouldSerializeScale() || (DataRowVersion.Current != p.SourceVersion)) { flags |= 16; } if (p.SourceColumnNullMapping) { flags |= 32; } Type[] ctorParams; object[] ctorValues; switch(flags) { case 0: // ParameterName case 1: // OracleType ctorParams = new Type[] { typeof(string), typeof(OracleType) }; ctorValues = new object[] { p.ParameterName, p.OracleType }; break; case 2: // Size case 3: // Size, OracleType ctorParams = new Type[] { typeof(string), typeof(OracleType), typeof(int) }; ctorValues = new object[] { p.ParameterName, p.OracleType, p.Size }; break; case 4: // SourceColumn case 5: // SourceColumn, OracleType case 6: // SourceColumn, Size case 7: // SourceColumn, Size, OracleType ctorParams = new Type[] { typeof(string), typeof(OracleType), typeof(int), typeof(string) }; ctorValues = new object[] { p.ParameterName, p.OracleType, p.Size, p.SourceColumn }; break; case 8: // Value ctorParams = new Type[] { typeof(string), typeof(object) }; ctorValues = new object[] { p.ParameterName, p.Value }; break; default: if (0 == (32 & flags)) { ctorParams = new Type[] { typeof(string), typeof(OracleType), typeof(int), typeof(ParameterDirection), typeof(bool), typeof(byte), typeof(byte), typeof(string), typeof(DataRowVersion), typeof(object) }; ctorValues = new object[] { p.ParameterName, p.OracleType, p.Size, p.Direction, p.IsNullable, p.PrecisionInternal, p.ScaleInternal, p.SourceColumn, p.SourceVersion, p.Value }; } else { ctorParams = new Type[] { typeof(string), typeof(OracleType), typeof(int), typeof(ParameterDirection), typeof(string), typeof(DataRowVersion), typeof(bool), typeof(object) }; ctorValues = new object[] { p.ParameterName, p.OracleType, p.Size, p.Direction, p.SourceColumn, p.SourceVersion, p.SourceColumnNullMapping, p.Value }; } break; } System.Reflection.ConstructorInfo ctor = typeof(OracleParameter).GetConstructor(ctorParams); return new InstanceDescriptor(ctor, ctorValues); } } } }; // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Data.OracleClient { using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Data; using System.Data.Common; using System.Data.ProviderBase; using System.Data.SqlTypes; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; //--------------------------------------------------------------------- // OracleParameter // [ TypeConverterAttribute(typeof(System.Data.OracleClient.OracleParameter.OracleParameterConverter)) ] sealed public partial class OracleParameter : DbParameter, ICloneable, IDbDataParameter { private MetaType _metaType; // type information; only set when DbType or OracleType is set. private int _commandSetResult; // the ordinal result number for parameters in support of Command Set, 0 indicates it is not a Command Set result. private MetaType _coercedMetaType; private string _parameterName; private byte _precision; private byte _scale; private bool _hasScale; // Construct an "empty" parameter public OracleParameter() : base() {} // Construct from a parameter name and a value object public OracleParameter(string name, object value) { this.ParameterName = name; this.Value = value; } // Construct from a parameter name and a data type public OracleParameter(string name, OracleType oracleType) : this() { this.ParameterName = name; this.OracleType = oracleType; } // Construct from a parameter name, a data type and the size public OracleParameter(string name, OracleType oracleType, int size) : this() { this.ParameterName = name; this.OracleType = oracleType; this.Size = size; } // Construct from a parameter name, a data type, the size and the source column public OracleParameter( string name, OracleType oracleType, int size, string srcColumn ) : this() { this.ParameterName = name; this.OracleType = oracleType; this.Size = size; this.SourceColumn = srcColumn; } // Construct from everything but the kitchen sink public OracleParameter(string name, OracleType oracleType, int size, ParameterDirection direction, bool isNullable, byte precision, byte scale, string srcColumn, DataRowVersion srcVersion, object value) : this() { this.ParameterName = name; this.OracleType = oracleType; this.Size = size; this.Direction = direction; this.IsNullable = isNullable; PrecisionInternal = precision; ScaleInternal = scale; this.SourceColumn = srcColumn; this.SourceVersion = srcVersion; this.Value = value; } // V2.0 everything - round trip all browsable properties public OracleParameter(string name, OracleType oracleType, int size, ParameterDirection direction, string sourceColumn, DataRowVersion sourceVersion, bool sourceColumnNullMapping, object value) : this() { this.ParameterName = name; this.OracleType = oracleType; this.Size = size; this.Direction = direction; this.SourceColumn = sourceColumn; this.SourceVersion = sourceVersion; this.SourceColumnNullMapping = sourceColumnNullMapping; this.Value = value; } internal int BindSize { get { int size = GetActualSize(); // If the size of the value is huge and it's an input-only parameter, // we can try and figure out the binding size. if (short.MaxValue < size && ParameterDirection.Input == Direction) { size = ValueSize(GetCoercedValueInternal()); } return size; } } internal int CommandSetResult { get { return _commandSetResult; } set { _commandSetResult = value; } } override public DbType DbType { get { return GetMetaType().DbType; } set { if ((null == _metaType) || (_metaType.DbType != value)) { PropertyTypeChanging(); _metaType = MetaType.GetMetaTypeForType(value); } } } public override void ResetDbType() { ResetOracleType(); } [ DefaultValue(OracleType.VarChar), // MDAC 65862 ResCategoryAttribute(Res.OracleCategory_Data), RefreshProperties(RefreshProperties.All), ResDescriptionAttribute(Res.OracleParameter_OracleType), System.Data.Common.DbProviderSpecificTypePropertyAttribute(true), ] public OracleType OracleType { get { return GetMetaType().OracleType; } set { MetaType metatype = _metaType; if ((null == metatype) || (metatype.OracleType != value)) { PropertyTypeChanging(); _metaType = MetaType.GetMetaTypeForType(value); } } } public void ResetOracleType() { if (null != _metaType) { PropertyTypeChanging(); _metaType = null; } } [ ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbParameter_ParameterName), ] override public string ParameterName { // V1.2.3300, XXXParameter V1.0.3300 get { string parameterName = _parameterName; return ((null != parameterName) ? parameterName : ADP.StrEmpty); } set { if (_parameterName != value) { _parameterName = value; } } } [Browsable(false)] [EditorBrowsableAttribute(EditorBrowsableState.Never)] [Obsolete("Precision has been deprecated. Use the Math classes to explicitly set the precision of a decimal. http://go.microsoft.com/fwlink/?linkid=14202")] public Byte Precision { get { return PrecisionInternal; } set { PrecisionInternal = value; } } private byte PrecisionInternal { get { return _precision; } set { if (_precision != value) { _precision = value; } } } private bool ShouldSerializePrecision() { return (0 != _precision); } [Browsable(false)] [EditorBrowsableAttribute(EditorBrowsableState.Never)] [Obsolete("Scale has been deprecated. Use the Math classes to explicitly set the scale of a decimal. http://go.microsoft.com/fwlink/?linkid=14202")] public Byte Scale { get { return ScaleInternal; } set { ScaleInternal = value; } } private byte ScaleInternal { get { return _scale; } set { if (_scale != value || !_hasScale) { //PropertyChanging(); _scale = value; _hasScale = true; } } } private bool ShouldSerializeScale() { return _hasScale; } private static object CoerceValue(object value, MetaType destinationType) { Debug.Assert(null != destinationType, "null destinationType"); if ((null != value) && !Convert.IsDBNull(value) && (typeof(object) != destinationType.BaseType)) { Type currentType = value.GetType(); if ((currentType != destinationType.BaseType) && (currentType != destinationType.NoConvertType)) { try { if ((typeof(string) == destinationType.BaseType) && (typeof(char[]) == currentType)) { value = new string((char[])value); } else if ((DbType.Currency == destinationType.DbType) && (typeof(string) == currentType)) { value = Decimal.Parse((string)value, NumberStyles.Currency, (IFormatProvider)null); // WebData 99376 } else { value = Convert.ChangeType(value, destinationType.BaseType, (IFormatProvider)null); } } catch(Exception e) { // if (!ADP.IsCatchableExceptionType(e)) { throw; } throw ADP.ParameterConversionFailed(value, destinationType.BaseType, e); // WebData 75433 } } } return value; } object ICloneable.Clone() { return new OracleParameter(this); } private void CloneHelper(OracleParameter destination) { CloneHelperCore(destination); destination._metaType = _metaType; destination._parameterName = _parameterName; destination._precision = _precision; destination._scale = _scale; destination._hasScale = _hasScale; } internal int GetActualSize() { return ShouldSerializeSize() ? Size : ValueSize(CoercedValue); } private MetaType GetMetaType() { // if the user specifed a type, then return it's meta data return GetMetaType(Value); } internal MetaType GetMetaType(object value) { MetaType metaType = _metaType; if (null == metaType) { // if the didn't specify a type, but they specified a value, then // return the meta data for the value they specified if ((null != value) && !Convert.IsDBNull(value)) { metaType = MetaType.GetMetaTypeForObject(value); } else { // if they haven't specified anything, then return the default // meta data information metaType = MetaType.GetDefaultMetaType(); } _metaType = metaType; } return metaType; } internal object GetCoercedValueInternal() { object value = CoercedValue; // will also be set during parameter Validation if (null == value) { value = CoerceValue(this.Value, _coercedMetaType); CoercedValue = value; } return value; } private void PropertyChanging() { } private void PropertyTypeChanging() { PropertyChanging(); CoercedValue = null; } internal void SetCoercedValueInternal(object value, MetaType metaType) { _coercedMetaType = metaType; CoercedValue = CoerceValue(value, metaType); } private bool ShouldSerializeOracleType() { return (null != _metaType); } [ RefreshProperties(RefreshProperties.All), ResCategoryAttribute(Res.DataCategory_Data), ResDescriptionAttribute(Res.DbParameter_Value), TypeConverterAttribute(typeof(StringConverter)), ] override public object Value { get { return _value; } set { _coercedValue = null; _value = value; } } private int ValueSize(object value) { if (value is OracleString) return ((OracleString) value).Length; if (value is string) return ((string) value).Length; if (value is char[]) return ((char[]) value).Length; if (value is OracleBinary) return ((OracleBinary) value).Length; return ValueSizeCore(value); } sealed internal class OracleParameterConverter : ExpandableObjectConverter { // converter classes should have public ctor public OracleParameterConverter() { } public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw ADP.ArgumentNull("destinationType"); } if (destinationType == typeof(InstanceDescriptor) && value is OracleParameter) { return ConvertToInstanceDescriptor(value as OracleParameter); } return base.ConvertTo(context, culture, value, destinationType); } private InstanceDescriptor ConvertToInstanceDescriptor(OracleParameter p) { // MDAC 67321 - reducing parameter generated code int flags = 0; // if part of the collection - the parametername can't be empty if (p.ShouldSerializeOracleType()) { flags |= 1; } if (p.ShouldSerializeSize()) { flags |= 2; } if (!ADP.IsEmpty(p.SourceColumn)) { flags |= 4; } if (null != p.Value) { flags |= 8; } if ((ParameterDirection.Input != p.Direction) || p.IsNullable || p.ShouldSerializePrecision() || p.ShouldSerializeScale() || (DataRowVersion.Current != p.SourceVersion)) { flags |= 16; } if (p.SourceColumnNullMapping) { flags |= 32; } Type[] ctorParams; object[] ctorValues; switch(flags) { case 0: // ParameterName case 1: // OracleType ctorParams = new Type[] { typeof(string), typeof(OracleType) }; ctorValues = new object[] { p.ParameterName, p.OracleType }; break; case 2: // Size case 3: // Size, OracleType ctorParams = new Type[] { typeof(string), typeof(OracleType), typeof(int) }; ctorValues = new object[] { p.ParameterName, p.OracleType, p.Size }; break; case 4: // SourceColumn case 5: // SourceColumn, OracleType case 6: // SourceColumn, Size case 7: // SourceColumn, Size, OracleType ctorParams = new Type[] { typeof(string), typeof(OracleType), typeof(int), typeof(string) }; ctorValues = new object[] { p.ParameterName, p.OracleType, p.Size, p.SourceColumn }; break; case 8: // Value ctorParams = new Type[] { typeof(string), typeof(object) }; ctorValues = new object[] { p.ParameterName, p.Value }; break; default: if (0 == (32 & flags)) { ctorParams = new Type[] { typeof(string), typeof(OracleType), typeof(int), typeof(ParameterDirection), typeof(bool), typeof(byte), typeof(byte), typeof(string), typeof(DataRowVersion), typeof(object) }; ctorValues = new object[] { p.ParameterName, p.OracleType, p.Size, p.Direction, p.IsNullable, p.PrecisionInternal, p.ScaleInternal, p.SourceColumn, p.SourceVersion, p.Value }; } else { ctorParams = new Type[] { typeof(string), typeof(OracleType), typeof(int), typeof(ParameterDirection), typeof(string), typeof(DataRowVersion), typeof(bool), typeof(object) }; ctorValues = new object[] { p.ParameterName, p.OracleType, p.Size, p.Direction, p.SourceColumn, p.SourceVersion, p.SourceColumnNullMapping, p.Value }; } break; } System.Reflection.ConstructorInfo ctor = typeof(OracleParameter).GetConstructor(ctorParams); return new InstanceDescriptor(ctor, ctorValues); } } } }; // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
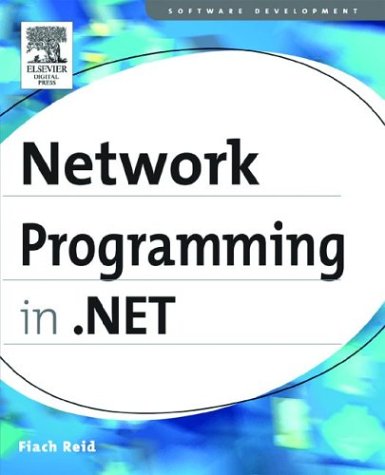
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RepeatBehavior.cs
- SqlNotificationEventArgs.cs
- HybridDictionary.cs
- SimpleExpression.cs
- ContextMenuService.cs
- StringDictionaryEditor.cs
- HeaderUtility.cs
- TextTreeNode.cs
- TablePatternIdentifiers.cs
- TemplateBindingExtension.cs
- baseaxisquery.cs
- PolygonHotSpot.cs
- ContentType.cs
- BooleanFacetDescriptionElement.cs
- MsmqQueue.cs
- TemplateNameScope.cs
- OpenTypeMethods.cs
- DataServiceKeyAttribute.cs
- GifBitmapDecoder.cs
- MailDefinition.cs
- SizeIndependentAnimationStorage.cs
- SchemaSetCompiler.cs
- SqlMethodTransformer.cs
- UnsafeNativeMethods.cs
- XmlDataDocument.cs
- SinglePageViewer.cs
- BitmapEffectrendercontext.cs
- RegistryDataKey.cs
- CmsUtils.cs
- SqlReorderer.cs
- EditBehavior.cs
- XmlSchemaSimpleType.cs
- __Filters.cs
- TemplateEditingFrame.cs
- XmlAttributeCollection.cs
- SurrogateDataContract.cs
- ResourceDictionaryCollection.cs
- BulletChrome.cs
- SqlCacheDependencyDatabase.cs
- BridgeDataRecord.cs
- WebControlsSection.cs
- InputBindingCollection.cs
- RectIndependentAnimationStorage.cs
- DrawingCollection.cs
- HitTestDrawingContextWalker.cs
- formatter.cs
- Propagator.JoinPropagator.cs
- ProfileService.cs
- ControlType.cs
- WebPartDisplayModeCollection.cs
- COM2IManagedPerPropertyBrowsingHandler.cs
- SQLInt16.cs
- StructuredProperty.cs
- StorageMappingFragment.cs
- HttpCookie.cs
- HwndSubclass.cs
- DispatcherTimer.cs
- EntitySqlQueryCacheKey.cs
- ConditionValidator.cs
- WorkItem.cs
- Errors.cs
- EntityTypeBase.cs
- ActiveXHelper.cs
- CalculatedColumn.cs
- SqlNotificationRequest.cs
- UrlSyndicationContent.cs
- DataError.cs
- CommandConverter.cs
- Vector3dCollection.cs
- SplitterDesigner.cs
- GroupPartitionExpr.cs
- ThreadExceptionDialog.cs
- MgmtConfigurationRecord.cs
- LambdaCompiler.cs
- WebBrowserProgressChangedEventHandler.cs
- GraphicsPath.cs
- SafeProcessHandle.cs
- PrivateFontCollection.cs
- SmtpCommands.cs
- CodeExporter.cs
- ByteArrayHelperWithString.cs
- Debugger.cs
- SystemIPInterfaceProperties.cs
- QueryConverter.cs
- DbMetaDataColumnNames.cs
- Exceptions.cs
- XmlAnyElementAttribute.cs
- followingquery.cs
- RadioButtonPopupAdapter.cs
- ActivityCodeGenerator.cs
- BinaryObjectReader.cs
- WorkflowMarkupSerializerMapping.cs
- TemplateControlBuildProvider.cs
- InstanceCreationEditor.cs
- InstallerTypeAttribute.cs
- WmlValidationSummaryAdapter.cs
- TextEndOfLine.cs
- RightsManagementLicense.cs
- MenuItemBinding.cs
- ShaderEffect.cs