Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / Selectors / SecurityTokenSerializer.cs / 1305376 / SecurityTokenSerializer.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.IdentityModel.Selectors { using System.Xml; using System.IdentityModel.Tokens; ////// SecurityTokenSerializer is responsible for writing and reading SecurityKeyIdentifiers, SecurityKeyIdentifierClauses and SecurityTokens. /// In order to read SecurityTokens the SecurityTokenSerializer may need to resolve token references using the SecurityTokenResolvers that get passed in. /// The SecurityTokenSerializer is stateless /// Exceptions: XmlException, SecurityTokenException, NotSupportedException, InvalidOperationException, ArgumentException /// public abstract class SecurityTokenSerializer { // public methods public bool CanReadToken(XmlReader reader) { if (reader == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); } return CanReadTokenCore(reader); ; } public bool CanWriteToken(SecurityToken token) { if (token == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("token"); } return CanWriteTokenCore(token); ; } public bool CanReadKeyIdentifier(XmlReader reader) { if (reader == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); } return CanReadKeyIdentifierCore(reader); ; } public bool CanWriteKeyIdentifier(SecurityKeyIdentifier keyIdentifier) { if (keyIdentifier == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("keyIdentifier"); } return CanWriteKeyIdentifierCore(keyIdentifier); ; } public bool CanReadKeyIdentifierClause(XmlReader reader) { if (reader == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); } return CanReadKeyIdentifierClauseCore(reader); ; } public bool CanWriteKeyIdentifierClause(SecurityKeyIdentifierClause keyIdentifierClause) { if (keyIdentifierClause == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("keyIdentifierClause"); } return CanWriteKeyIdentifierClauseCore(keyIdentifierClause); ; } public SecurityToken ReadToken(XmlReader reader, SecurityTokenResolver tokenResolver) { if (reader == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); } return ReadTokenCore(reader, tokenResolver); } public void WriteToken(XmlWriter writer, SecurityToken token) { if (writer == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("writer"); } if (token == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("token"); } WriteTokenCore(writer, token); } public SecurityKeyIdentifier ReadKeyIdentifier(XmlReader reader) { if (reader == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); } return ReadKeyIdentifierCore(reader); } public void WriteKeyIdentifier(XmlWriter writer, SecurityKeyIdentifier keyIdentifier) { if (writer == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("writer"); } if (keyIdentifier == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("keyIdentifier"); } WriteKeyIdentifierCore(writer, keyIdentifier); } public SecurityKeyIdentifierClause ReadKeyIdentifierClause(XmlReader reader) { if (reader == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("reader"); } return ReadKeyIdentifierClauseCore(reader); } public void WriteKeyIdentifierClause(XmlWriter writer, SecurityKeyIdentifierClause keyIdentifierClause) { if (writer == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("writer"); } if (keyIdentifierClause == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("keyIdentifierClause"); } WriteKeyIdentifierClauseCore(writer, keyIdentifierClause); } // protected abstract methods protected abstract bool CanReadTokenCore(XmlReader reader); protected abstract bool CanWriteTokenCore(SecurityToken token); protected abstract bool CanReadKeyIdentifierCore(XmlReader reader); protected abstract bool CanWriteKeyIdentifierCore(SecurityKeyIdentifier keyIdentifier); protected abstract bool CanReadKeyIdentifierClauseCore(XmlReader reader); protected abstract bool CanWriteKeyIdentifierClauseCore(SecurityKeyIdentifierClause keyIdentifierClause); protected abstract SecurityToken ReadTokenCore(XmlReader reader, SecurityTokenResolver tokenResolver); protected abstract void WriteTokenCore(XmlWriter writer, SecurityToken token); protected abstract SecurityKeyIdentifier ReadKeyIdentifierCore(XmlReader reader); protected abstract void WriteKeyIdentifierCore(XmlWriter writer, SecurityKeyIdentifier keyIdentifier); protected abstract SecurityKeyIdentifierClause ReadKeyIdentifierClauseCore(XmlReader reader); protected abstract void WriteKeyIdentifierClauseCore(XmlWriter writer, SecurityKeyIdentifierClause keyIdentifierClause); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
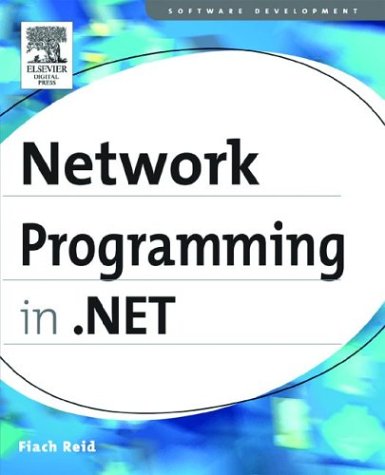
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TabControl.cs
- FileDialog.cs
- HitTestParameters.cs
- HtmlButton.cs
- TrackingServices.cs
- ComboBoxRenderer.cs
- CodeParameterDeclarationExpression.cs
- RemoteCryptoRsaServiceProvider.cs
- MaskedTextProvider.cs
- DataGridTextBoxColumn.cs
- RuntimeCompatibilityAttribute.cs
- StoreAnnotationsMap.cs
- SecuritySessionFilter.cs
- TreeNodeBinding.cs
- JsonReader.cs
- IdentityReference.cs
- PlatformNotSupportedException.cs
- TextAction.cs
- ClassDataContract.cs
- MissingManifestResourceException.cs
- CodeGotoStatement.cs
- PermissionSetTriple.cs
- DataGridViewSortCompareEventArgs.cs
- FixedBufferAttribute.cs
- CacheRequest.cs
- KnownBoxes.cs
- HtmlInputCheckBox.cs
- SafeNativeHandle.cs
- IdentityHolder.cs
- ValueUnavailableException.cs
- LingerOption.cs
- BigInt.cs
- Pair.cs
- ExpandCollapseProviderWrapper.cs
- ContextMarshalException.cs
- Animatable.cs
- UserControlCodeDomTreeGenerator.cs
- TreeNodeCollection.cs
- NativeActivityMetadata.cs
- TraceLog.cs
- WeakReadOnlyCollection.cs
- WebPartActionVerb.cs
- Utility.cs
- HttpBrowserCapabilitiesWrapper.cs
- LoginName.cs
- SubpageParaClient.cs
- DynamicILGenerator.cs
- Missing.cs
- QilUnary.cs
- QuaternionRotation3D.cs
- SamlAudienceRestrictionCondition.cs
- ContextDataSource.cs
- AbandonedMutexException.cs
- SchemaImporterExtensionElement.cs
- Animatable.cs
- SimpleApplicationHost.cs
- EventManager.cs
- PriorityItem.cs
- Enum.cs
- ListSortDescription.cs
- FilterQuery.cs
- CryptoKeySecurity.cs
- Mutex.cs
- MultipartContentParser.cs
- CodeVariableReferenceExpression.cs
- SymbolType.cs
- XsltFunctions.cs
- ISAPIRuntime.cs
- UnsafeNativeMethods.cs
- SAPIEngineTypes.cs
- FolderLevelBuildProviderAppliesToAttribute.cs
- TerminatorSinks.cs
- SystemIcmpV6Statistics.cs
- WebBrowser.cs
- SettingsAttributes.cs
- FileClassifier.cs
- ScrollItemPattern.cs
- ConditionalDesigner.cs
- Delay.cs
- MsmqChannelFactory.cs
- FamilyMapCollection.cs
- PropertyDescriptorCollection.cs
- FieldToken.cs
- ACE.cs
- ControlCachePolicy.cs
- SynchronizedDispatch.cs
- TableTextElementCollectionInternal.cs
- RSAOAEPKeyExchangeDeformatter.cs
- NullableDoubleAverageAggregationOperator.cs
- ContentPlaceHolder.cs
- ListViewHitTestInfo.cs
- Rijndael.cs
- RegistryPermission.cs
- DeclarationUpdate.cs
- ImageSource.cs
- HandledEventArgs.cs
- DesignerAdapterUtil.cs
- SharedDp.cs
- SchemaNamespaceManager.cs
- IItemProperties.cs