Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / RemoteCryptoRsaServiceProvider.cs / 1 / RemoteCryptoRsaServiceProvider.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.IO; using System.Security; using System.Security.Cryptography; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // Encrypt request for RpcCrypto // internal sealed class RemoteCryptoRsaServiceProvider : RSA, ICspAsymmetricAlgorithm { const int CRYPT_OAEP = 0x40; X509CertificateTokenFactoryCredential m_credential; RpcCryptoContext m_context; CspKeyContainerInfo m_cspInfo; UIAgentRequest m_request; object m_sync = new object(); bool m_disposed = false; public RemoteCryptoRsaServiceProvider( X509CertificateTokenFactoryCredential credential, UIAgentRequest request ) { m_credential = credential; m_context = new RpcCryptoContext( m_credential.PortName, m_credential.ContextKey ); CspParameters p = new CspParameters(); p.KeyContainerName = "RpcCrypto"; p.ProviderName = "RpcCrypto"; p.ProviderType = 0; p.KeyNumber = (int)KeyNumber.Exchange; m_cspInfo = new CspKeyContainerInfo( p ); m_request = request; } // // Summary: // returns the KeyInfo for the current keys. // CspKeyContainerInfo ICspAsymmetricAlgorithm.CspKeyContainerInfo { get { return m_cspInfo; } } // // Summary: // Exports the public key info to a CspBlob // // Arguments: // includePrivateParameters: must be false // // Returns: // the new CspBlob // byte[] ICspAsymmetricAlgorithm.ExportCspBlob(bool includePrivateParameters) { ICspAsymmetricAlgorithm alg = m_credential.Certificate.PublicKey.Key as ICspAsymmetricAlgorithm; if( null == alg ) { throw IDT.ThrowHelperError( new NotSupportedException() ); } if( includePrivateParameters ) { throw IDT.ThrowHelperError( new NotSupportedException() ); } return alg.ExportCspBlob( false ); } // // Summary: // Import is not supported. // void ICspAsymmetricAlgorithm.ImportCspBlob(byte[] rawData) { throw IDT.ThrowHelperError( new NotSupportedException() ); } // // Summary: // Returns the KeyExchangeAlg // public override string KeyExchangeAlgorithm { get { if( null != m_credential && null != m_credential.Certificate && null != m_credential.Certificate.PublicKey && null != m_credential.Certificate.PublicKey.Key ) { return m_credential.Certificate.PublicKey.Key.KeyExchangeAlgorithm; } return null; } } // // Summary: // Returns the SignatureAlgorithm // public override string SignatureAlgorithm { get { if( null != m_credential && null != m_credential.Certificate && null != m_credential.Certificate.PublicKey && null != m_credential.Certificate.PublicKey.Key ) { return m_credential.Certificate.PublicKey.Key.SignatureAlgorithm; } return null; } } // // Summary: // Returns the key size. // public override int KeySize { get { if( null != m_credential && null != m_credential.Certificate && null != m_credential.Certificate.PublicKey && null != m_credential.Certificate.PublicKey.Key ) { return m_credential.Certificate.PublicKey.Key.KeySize; } return 0; } set { throw IDT.ThrowHelperError( new NotSupportedException() ); } } // // Summary: // Returns the legal key sizes. // public override KeySizes[] LegalKeySizes { get { return new KeySizes[] { new KeySizes(KeySize, KeySize, 0) }; } } // // Summary: // Signs the provided hash // // Arguments: // hashAlg: the ALG_ID of the hash // hash: the hash to verify. // // Returns: // the new signature. // public byte[] SignHash( int hashAlg, byte[] hash ) { ThrowIfRemoteProcessDied(); RemoteCryptoSignHashRequest req = new RemoteCryptoSignHashRequest( GetContext(), hashAlg, 0, hash ); req.Process(); byte[] signature = req.GetSignature(); ThrowIfRemoteProcessDied(); return signature; } // // Summary: // Decrypts the specified // // Arguments: // rgb: data to decrypt // // Returns: // returns the clear text value. // public override byte[] DecryptValue(byte[] rgb) { ThrowIfRemoteProcessDied(); byte[] localBuffer = new byte[ rgb.Length ]; Array.Copy( rgb, 0, localBuffer, 0, rgb.Length ); RemoteCryptoDecryptRequest req = new RemoteCryptoDecryptRequest( GetContext(), 0, true, localBuffer, 0, localBuffer.Length, 0, null ); req.Process(); byte[] returnbuffer = new byte[ req.Length ]; Array.Copy( req.GetBuffer(), req.Index, returnbuffer, 0, req.Length ); ThrowIfRemoteProcessDied(); return returnbuffer; } // // Summary: // Encrypts the specified // // Arguments: // rgb: data to encrypt // // Returns: // returns the encrypted text value. // public override byte[] EncryptValue(byte[] rgb) { return ((RSA)m_credential.Certificate.PublicKey.Key).EncryptValue(rgb); } // // Summary: // Exports the public key info as RSAParameters // // Arguments: // includePrivateParameters: must be false; // // Returns: // The RSAParameters for the public key. // public override RSAParameters ExportParameters(bool includePrivateParameters) { if( includePrivateParameters || null == m_credential.Certificate.PublicKey ) { throw IDT.ThrowHelperError( new CryptographicException( SR.GetString( SR.ServiceCanNotExportCertIdentityPrivateKey ) ) ); } if( m_credential.Certificate.PublicKey.Key is RSA ) { return ((RSA)m_credential.Certificate.PublicKey.Key).ExportParameters(false); } throw IDT.ThrowHelperError( new CryptographicException( SR.GetString( SR.ServiceCanNotExportCertIdentityPrivateKey ) ) ); } // // Summary: // // public override void ImportParameters(RSAParameters parameters) { throw IDT.ThrowHelperError( new NotSupportedException() ); } protected override void Dispose( bool disposing ) { if ( m_disposed ) { return; } lock( m_sync ) { if( m_disposed ) { return; } try { if( disposing ) { // // Dispose managed resources // } // // Dispose unmanaged resources // // // Tear down the context. // (( IDisposable )m_context).Dispose(); m_disposed = true; } finally { ; } } } RpcCryptoContext GetContext() { if( !m_context.IsOpen ) { m_context.Open(); } return m_context; } private void ThrowIfRemoteProcessDied() { // // If the UIAgent has died then we no longer can be certain who we are talking to. Crypto operations // should no longer be trusted. Before the UIAgent has died we were given the random name of then endpoint // by the UIAgent so we can be certain that we are talking directly to the right process. // if( !m_request.ParentRequest.UIAgentActive ) { throw IDT.ThrowHelperError( new CryptographicException( SR.GetString( SR.RemoteCryptoSessionUnavailable ) ) ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
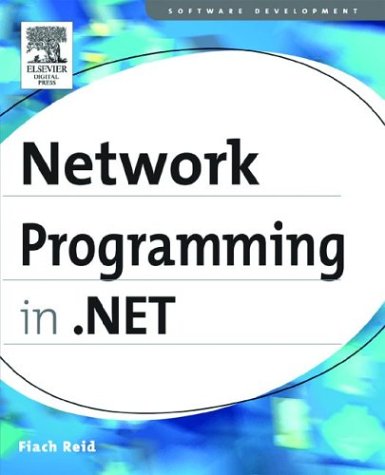
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PresentationSource.cs
- BitmapData.cs
- XmlSerializerNamespaces.cs
- Trigger.cs
- SizeAnimation.cs
- Compiler.cs
- FastPropertyAccessor.cs
- NamespaceExpr.cs
- StringAttributeCollection.cs
- FixedBufferAttribute.cs
- FormViewInsertedEventArgs.cs
- ValidationEventArgs.cs
- TargetParameterCountException.cs
- MultiByteCodec.cs
- Point.cs
- IisTraceListener.cs
- Char.cs
- Point3DCollectionConverter.cs
- UseManagedPresentationBindingElement.cs
- SystemIcmpV4Statistics.cs
- _SSPIWrapper.cs
- CssStyleCollection.cs
- sqlstateclientmanager.cs
- CqlBlock.cs
- Event.cs
- DetectEofStream.cs
- RegexWorker.cs
- ModelPropertyImpl.cs
- FrameworkPropertyMetadata.cs
- InputLangChangeRequestEvent.cs
- AsyncOperationLifetimeManager.cs
- TypeUtils.cs
- SettingsPropertyCollection.cs
- UseManagedPresentationBindingElement.cs
- AnimationClock.cs
- SamlSubject.cs
- TrustLevel.cs
- OuterGlowBitmapEffect.cs
- PeerValidationBehavior.cs
- MemberInfoSerializationHolder.cs
- StorageFunctionMapping.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- NetworkInformationException.cs
- MasterPageCodeDomTreeGenerator.cs
- ThreadStateException.cs
- BasicViewGenerator.cs
- TextEndOfSegment.cs
- OleStrCAMarshaler.cs
- FlowDocument.cs
- HttpCapabilitiesBase.cs
- InternalConfigEventArgs.cs
- SmtpClient.cs
- TreeView.cs
- messageonlyhwndwrapper.cs
- webeventbuffer.cs
- InputReport.cs
- CmsInterop.cs
- ButtonBase.cs
- CodeTypeDeclaration.cs
- SimpleBitVector32.cs
- XmlNamespaceMappingCollection.cs
- DbgUtil.cs
- TraceInternal.cs
- EmbossBitmapEffect.cs
- Win32MouseDevice.cs
- SQLMembershipProvider.cs
- SpotLight.cs
- ToolTipService.cs
- _RegBlobWebProxyDataBuilder.cs
- DataGridState.cs
- DocumentViewerHelper.cs
- TreeNodeSelectionProcessor.cs
- SharedPersonalizationStateInfo.cs
- DetailsViewRowCollection.cs
- SolidColorBrush.cs
- HtmlTitle.cs
- SecurityAppliedMessage.cs
- SignedXml.cs
- MarshalByRefObject.cs
- AsmxEndpointPickerExtension.cs
- UIElementAutomationPeer.cs
- MsmqActivation.cs
- mediaclock.cs
- SizeAnimationUsingKeyFrames.cs
- IssuedTokenClientBehaviorsElement.cs
- PathGradientBrush.cs
- SqlCacheDependencyDatabaseCollection.cs
- SpecularMaterial.cs
- Pair.cs
- WebMessageBodyStyleHelper.cs
- XhtmlBasicFormAdapter.cs
- DriveNotFoundException.cs
- ViewStateModeByIdAttribute.cs
- ManagedWndProcTracker.cs
- TypeConverter.cs
- LogSwitch.cs
- StackBuilderSink.cs
- AffineTransform3D.cs
- SetMemberBinder.cs
- SelectionItemPattern.cs