Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Sys / System / Configuration / SettingsAttributes.cs / 1 / SettingsAttributes.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System; ////// Indicates that a setting is to be stored on a per-application basis. /// [AttributeUsage(AttributeTargets.Property)] public sealed class ApplicationScopedSettingAttribute : SettingAttribute { } ////// Indicates to the provider what default value to use for this setting when no stored value /// is found. The value should be encoded into a string and is interpreted based on the SerializeAs /// value for this setting. For example, if SerializeAs is Xml, the default value will be /// "stringified" Xml. /// [AttributeUsage(AttributeTargets.Property)] public sealed class DefaultSettingValueAttribute : Attribute { private readonly string _value; ////// Constructor takes the default value as string. /// public DefaultSettingValueAttribute(string value) { _value = value; } ////// Default value. /// public string Value { get { return _value; } } } ////// Indicates that the provider should disable any logic that gets invoked when an application /// upgrade is detected. /// [AttributeUsage(AttributeTargets.Property)] public sealed class NoSettingsVersionUpgradeAttribute : Attribute { } ////// Use this attribute to mark properties on a settings class that are to be treated /// as settings. ApplicationSettingsBase will ignore all properties not marked with /// this or a derived attribute. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1813:AvoidUnsealedAttributes")] [AttributeUsage(AttributeTargets.Property)] public class SettingAttribute : Attribute { } ////// Description for a particular setting. /// [AttributeUsage(AttributeTargets.Property)] public sealed class SettingsDescriptionAttribute : Attribute { private readonly string _desc; ////// Constructor takes the description string. /// public SettingsDescriptionAttribute(string description) { _desc = description; } ////// Description string. /// public string Description { get { return _desc; } } } ////// Description for a particular settings group. /// [AttributeUsage(AttributeTargets.Class)] public sealed class SettingsGroupDescriptionAttribute : Attribute { private readonly string _desc; ////// Constructor takes the description string. /// public SettingsGroupDescriptionAttribute(string description) { _desc = description; } ////// Description string. /// public string Description { get { return _desc; } } } ////// Name of a particular settings group. /// [AttributeUsage(AttributeTargets.Class)] public sealed class SettingsGroupNameAttribute : Attribute { private readonly string _groupName; ////// Constructor takes the group name. /// public SettingsGroupNameAttribute(string groupName) { _groupName = groupName; } ////// Name of the settings group. /// public string GroupName { get { return _groupName; } } } ////// Indicates the SettingsManageability for a group of/individual setting. /// [AttributeUsage(AttributeTargets.Class | AttributeTargets.Property)] public sealed class SettingsManageabilityAttribute : Attribute { private readonly SettingsManageability _manageability; ////// Constructor takes a SettingsManageability enum value. /// public SettingsManageabilityAttribute(SettingsManageability manageability) { _manageability = manageability; } ////// SettingsManageability value to use /// public SettingsManageability Manageability { get { return _manageability; } } } ////// Indicates the provider associated with a group of/individual setting. /// [AttributeUsage(AttributeTargets.Class | AttributeTargets.Property)] public sealed class SettingsProviderAttribute : Attribute { private readonly string _providerTypeName; ////// Constructor takes the provider's assembly qualified type name. /// public SettingsProviderAttribute(string providerTypeName) { _providerTypeName = providerTypeName; } ////// Constructor takes the provider's type. /// public SettingsProviderAttribute(Type providerType) { if (providerType != null) { _providerTypeName = providerType.AssemblyQualifiedName; } } ////// Type name of the provider /// public string ProviderTypeName { get { return _providerTypeName; } } } ////// Indicates the SettingsSerializeAs for a group of/individual setting. /// [AttributeUsage(AttributeTargets.Class | AttributeTargets.Property)] public sealed class SettingsSerializeAsAttribute : Attribute { private readonly SettingsSerializeAs _serializeAs; ////// Constructor takes a SettingsSerializeAs enum value. /// public SettingsSerializeAsAttribute(SettingsSerializeAs serializeAs) { _serializeAs = serializeAs; } ////// SettingsSerializeAs value to use /// public SettingsSerializeAs SerializeAs { get { return _serializeAs; } } } ////// Indicates the SpecialSetting for a group of/individual setting. /// [AttributeUsage(AttributeTargets.Class | AttributeTargets.Property)] public sealed class SpecialSettingAttribute : Attribute { private readonly SpecialSetting _specialSetting; ////// Constructor takes a SpecialSetting enum value. /// public SpecialSettingAttribute(SpecialSetting specialSetting) { _specialSetting = specialSetting; } ////// SpecialSetting value to use /// public SpecialSetting SpecialSetting { get { return _specialSetting; } } } ////// Indicates that a setting is to be stored on a per-user basis. /// [AttributeUsage(AttributeTargets.Property)] public sealed class UserScopedSettingAttribute : SettingAttribute { } public enum SettingsManageability { Roaming = 0 } ////// Indicates settings that are to be treated "specially". /// public enum SpecialSetting { ConnectionString = 0, WebServiceUrl = 1 } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System; ////// Indicates that a setting is to be stored on a per-application basis. /// [AttributeUsage(AttributeTargets.Property)] public sealed class ApplicationScopedSettingAttribute : SettingAttribute { } ////// Indicates to the provider what default value to use for this setting when no stored value /// is found. The value should be encoded into a string and is interpreted based on the SerializeAs /// value for this setting. For example, if SerializeAs is Xml, the default value will be /// "stringified" Xml. /// [AttributeUsage(AttributeTargets.Property)] public sealed class DefaultSettingValueAttribute : Attribute { private readonly string _value; ////// Constructor takes the default value as string. /// public DefaultSettingValueAttribute(string value) { _value = value; } ////// Default value. /// public string Value { get { return _value; } } } ////// Indicates that the provider should disable any logic that gets invoked when an application /// upgrade is detected. /// [AttributeUsage(AttributeTargets.Property)] public sealed class NoSettingsVersionUpgradeAttribute : Attribute { } ////// Use this attribute to mark properties on a settings class that are to be treated /// as settings. ApplicationSettingsBase will ignore all properties not marked with /// this or a derived attribute. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1813:AvoidUnsealedAttributes")] [AttributeUsage(AttributeTargets.Property)] public class SettingAttribute : Attribute { } ////// Description for a particular setting. /// [AttributeUsage(AttributeTargets.Property)] public sealed class SettingsDescriptionAttribute : Attribute { private readonly string _desc; ////// Constructor takes the description string. /// public SettingsDescriptionAttribute(string description) { _desc = description; } ////// Description string. /// public string Description { get { return _desc; } } } ////// Description for a particular settings group. /// [AttributeUsage(AttributeTargets.Class)] public sealed class SettingsGroupDescriptionAttribute : Attribute { private readonly string _desc; ////// Constructor takes the description string. /// public SettingsGroupDescriptionAttribute(string description) { _desc = description; } ////// Description string. /// public string Description { get { return _desc; } } } ////// Name of a particular settings group. /// [AttributeUsage(AttributeTargets.Class)] public sealed class SettingsGroupNameAttribute : Attribute { private readonly string _groupName; ////// Constructor takes the group name. /// public SettingsGroupNameAttribute(string groupName) { _groupName = groupName; } ////// Name of the settings group. /// public string GroupName { get { return _groupName; } } } ////// Indicates the SettingsManageability for a group of/individual setting. /// [AttributeUsage(AttributeTargets.Class | AttributeTargets.Property)] public sealed class SettingsManageabilityAttribute : Attribute { private readonly SettingsManageability _manageability; ////// Constructor takes a SettingsManageability enum value. /// public SettingsManageabilityAttribute(SettingsManageability manageability) { _manageability = manageability; } ////// SettingsManageability value to use /// public SettingsManageability Manageability { get { return _manageability; } } } ////// Indicates the provider associated with a group of/individual setting. /// [AttributeUsage(AttributeTargets.Class | AttributeTargets.Property)] public sealed class SettingsProviderAttribute : Attribute { private readonly string _providerTypeName; ////// Constructor takes the provider's assembly qualified type name. /// public SettingsProviderAttribute(string providerTypeName) { _providerTypeName = providerTypeName; } ////// Constructor takes the provider's type. /// public SettingsProviderAttribute(Type providerType) { if (providerType != null) { _providerTypeName = providerType.AssemblyQualifiedName; } } ////// Type name of the provider /// public string ProviderTypeName { get { return _providerTypeName; } } } ////// Indicates the SettingsSerializeAs for a group of/individual setting. /// [AttributeUsage(AttributeTargets.Class | AttributeTargets.Property)] public sealed class SettingsSerializeAsAttribute : Attribute { private readonly SettingsSerializeAs _serializeAs; ////// Constructor takes a SettingsSerializeAs enum value. /// public SettingsSerializeAsAttribute(SettingsSerializeAs serializeAs) { _serializeAs = serializeAs; } ////// SettingsSerializeAs value to use /// public SettingsSerializeAs SerializeAs { get { return _serializeAs; } } } ////// Indicates the SpecialSetting for a group of/individual setting. /// [AttributeUsage(AttributeTargets.Class | AttributeTargets.Property)] public sealed class SpecialSettingAttribute : Attribute { private readonly SpecialSetting _specialSetting; ////// Constructor takes a SpecialSetting enum value. /// public SpecialSettingAttribute(SpecialSetting specialSetting) { _specialSetting = specialSetting; } ////// SpecialSetting value to use /// public SpecialSetting SpecialSetting { get { return _specialSetting; } } } ////// Indicates that a setting is to be stored on a per-user basis. /// [AttributeUsage(AttributeTargets.Property)] public sealed class UserScopedSettingAttribute : SettingAttribute { } public enum SettingsManageability { Roaming = 0 } ////// Indicates settings that are to be treated "specially". /// public enum SpecialSetting { ConnectionString = 0, WebServiceUrl = 1 } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
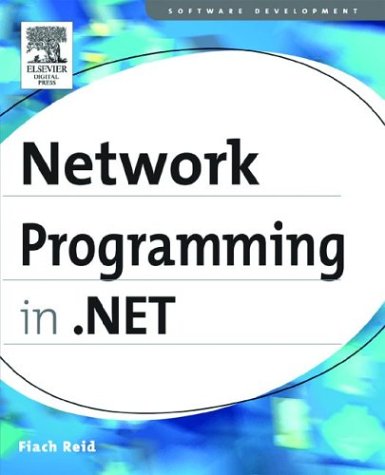
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- __ConsoleStream.cs
- ScriptRegistrationManager.cs
- RelationshipEndCollection.cs
- DataServiceCollectionOfT.cs
- HttpModuleCollection.cs
- Parsers.cs
- EllipseGeometry.cs
- Lease.cs
- DataGridViewCheckBoxColumn.cs
- PropertyMapper.cs
- CompilationRelaxations.cs
- TdsParserStateObject.cs
- MetabaseServerConfig.cs
- FileSystemInfo.cs
- WCFServiceClientProxyGenerator.cs
- SmiConnection.cs
- FileCodeGroup.cs
- TraceUtility.cs
- PackageDigitalSignature.cs
- CdpEqualityComparer.cs
- JsonFormatWriterGenerator.cs
- FormsAuthenticationUserCollection.cs
- AsyncOperationLifetimeManager.cs
- UnsafeNativeMethods.cs
- HandlerBase.cs
- CompilationRelaxations.cs
- DrawingImage.cs
- NonSerializedAttribute.cs
- ErrorWrapper.cs
- MyContact.cs
- FrameworkPropertyMetadata.cs
- TextFindEngine.cs
- IdentityManager.cs
- XmlAnyAttributeAttribute.cs
- DesignerCategoryAttribute.cs
- TextRangeEditLists.cs
- CodeStatementCollection.cs
- Configuration.cs
- KeyPressEvent.cs
- DiscoveryExceptionDictionary.cs
- DnsPermission.cs
- SessionParameter.cs
- EventLogInternal.cs
- SiteMapNode.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- ImagingCache.cs
- BindingSource.cs
- BufferedGraphics.cs
- CodeExpressionCollection.cs
- TextEditorParagraphs.cs
- MDIControlStrip.cs
- SettingsContext.cs
- FontEmbeddingManager.cs
- Message.cs
- MulticastNotSupportedException.cs
- DataGridViewButtonColumn.cs
- VectorKeyFrameCollection.cs
- SourceLocationProvider.cs
- Root.cs
- Calendar.cs
- DateTimeFormat.cs
- MulticastNotSupportedException.cs
- LeafCellTreeNode.cs
- LazyTextWriterCreator.cs
- SystemInfo.cs
- DataObjectFieldAttribute.cs
- FileDialog.cs
- PackWebRequest.cs
- HttpHandlerActionCollection.cs
- DispatcherEventArgs.cs
- DateTimeConstantAttribute.cs
- CompressEmulationStream.cs
- NavigationProperty.cs
- PackWebResponse.cs
- BamlTreeMap.cs
- InboundActivityHelper.cs
- Attributes.cs
- SHA1CryptoServiceProvider.cs
- IntSecurity.cs
- ClientSettingsProvider.cs
- xmlsaver.cs
- LeftCellWrapper.cs
- BaseValidator.cs
- XPathSelectionIterator.cs
- GridViewCellAutomationPeer.cs
- ThousandthOfEmRealPoints.cs
- SqlClientPermission.cs
- SqlDataSourceCache.cs
- SmtpAuthenticationManager.cs
- MenuItem.cs
- ChooseAction.cs
- CustomAttribute.cs
- QualificationDataAttribute.cs
- CodeBinaryOperatorExpression.cs
- UndoManager.cs
- ProtocolElement.cs
- PropertyPathWorker.cs
- RequestChannel.cs
- XmlTypeAttribute.cs
- RootBrowserWindowProxy.cs