Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / UIAutomation / UIAutomationClient / MS / Win32 / NativeMethods.cs / 1305600 / NativeMethods.cs
//**************************************************************************** // HOW TO USE THIS FILE // // If you need access to a Win32 API that is not exposed, simply uncomment // it in one of the following files: // // NativeMethods.cs // UnsafeNativeMethods.cs // SafeNativeMethods.cs // // Only uncomment what you need to avoid code bloat. // // DO NOT adjust the visibility of anything in these files. They are marked // internal on pupose. //*************************************************************************** using System; using System.Runtime.InteropServices; namespace MS.Win32 { internal static class NativeMethods { public const int MAX_PATH = 260; // Dialog Codes internal const int WM_GETDLGCODE = 0x0087; internal const int DLGC_STATIC = 0x0100; [StructLayout(LayoutKind.Sequential)] public struct HWND { public IntPtr h; public static HWND Cast(IntPtr h) { HWND hTemp = new HWND(); hTemp.h = h; return hTemp; } public static implicit operator IntPtr(HWND h) { return h.h; } public static HWND NULL { get { HWND hTemp = new HWND(); hTemp.h = IntPtr.Zero; return hTemp; } } public static bool operator==(HWND hl, HWND hr) { return hl.h == hr.h; } public static bool operator!=(HWND hl, HWND hr) { return hl.h != hr.h; } override public bool Equals(object oCompare) { HWND hr = Cast((HWND)oCompare); return h == hr.h; } public override int GetHashCode() { return (int) h; } } [StructLayout(LayoutKind.Sequential)] public struct RECT { public int left; public int top; public int right; public int bottom; public RECT(int left, int top, int right, int bottom) { this.left = left; this.top = top; this.right = right; this.bottom = bottom; } public bool IsEmpty { get { return left >= right || top >= bottom; } } } [StructLayout(LayoutKind.Sequential)] public struct POINT { public int x; public int y; public POINT(int x, int y) { this.x = x; this.y = y; } } // WinEvent specific consts and delegates public const int EVENT_MIN = 0; public const int EVENT_MAX = 0x7FFFFFFF; public const int EVENT_SYSTEM_MENUSTART = 0x0004; public const int EVENT_SYSTEM_MENUEND = 0x0005; public const int EVENT_SYSTEM_MENUPOPUPSTART = 0x0006; public const int EVENT_SYSTEM_MENUPOPUPEND = 0x0007; public const int EVENT_SYSTEM_CAPTURESTART = 0x0008; public const int EVENT_SYSTEM_CAPTUREEND = 0x0009; public const int EVENT_SYSTEM_SWITCHSTART = 0x0014; public const int EVENT_SYSTEM_SWITCHEND = 0x0015; public const int EVENT_OBJECT_CREATE = 0x8000; public const int EVENT_OBJECT_DESTROY = 0x8001; public const int EVENT_OBJECT_SHOW = 0x8002; public const int EVENT_OBJECT_HIDE = 0x8003; public const int EVENT_OBJECT_FOCUS = 0x8005; public const int EVENT_OBJECT_STATECHANGE = 0x800A; public const int EVENT_OBJECT_LOCATIONCHANGE = 0x800B; public const int WINEVENT_OUTOFCONTEXT = 0x0000; public const int WINEVENT_SKIPOWNTHREAD = 0x0001; public const int WINEVENT_SKIPOWNPROCESS = 0x0002; public const int WINEVENT_INCONTEXT = 0x0004; // WinEvent fired when new Avalon UI is created public const int EventObjectUIFragmentCreate = 0x6FFFFFFF; // the delegate passed to USER for receiving a WinEvent public delegate void WinEventProcDef(int winEventHook, int eventId, IntPtr hwnd, int idObject, int idChild, int eventThread, uint eventTime); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //**************************************************************************** // HOW TO USE THIS FILE // // If you need access to a Win32 API that is not exposed, simply uncomment // it in one of the following files: // // NativeMethods.cs // UnsafeNativeMethods.cs // SafeNativeMethods.cs // // Only uncomment what you need to avoid code bloat. // // DO NOT adjust the visibility of anything in these files. They are marked // internal on pupose. //*************************************************************************** using System; using System.Runtime.InteropServices; namespace MS.Win32 { internal static class NativeMethods { public const int MAX_PATH = 260; // Dialog Codes internal const int WM_GETDLGCODE = 0x0087; internal const int DLGC_STATIC = 0x0100; [StructLayout(LayoutKind.Sequential)] public struct HWND { public IntPtr h; public static HWND Cast(IntPtr h) { HWND hTemp = new HWND(); hTemp.h = h; return hTemp; } public static implicit operator IntPtr(HWND h) { return h.h; } public static HWND NULL { get { HWND hTemp = new HWND(); hTemp.h = IntPtr.Zero; return hTemp; } } public static bool operator==(HWND hl, HWND hr) { return hl.h == hr.h; } public static bool operator!=(HWND hl, HWND hr) { return hl.h != hr.h; } override public bool Equals(object oCompare) { HWND hr = Cast((HWND)oCompare); return h == hr.h; } public override int GetHashCode() { return (int) h; } } [StructLayout(LayoutKind.Sequential)] public struct RECT { public int left; public int top; public int right; public int bottom; public RECT(int left, int top, int right, int bottom) { this.left = left; this.top = top; this.right = right; this.bottom = bottom; } public bool IsEmpty { get { return left >= right || top >= bottom; } } } [StructLayout(LayoutKind.Sequential)] public struct POINT { public int x; public int y; public POINT(int x, int y) { this.x = x; this.y = y; } } // WinEvent specific consts and delegates public const int EVENT_MIN = 0; public const int EVENT_MAX = 0x7FFFFFFF; public const int EVENT_SYSTEM_MENUSTART = 0x0004; public const int EVENT_SYSTEM_MENUEND = 0x0005; public const int EVENT_SYSTEM_MENUPOPUPSTART = 0x0006; public const int EVENT_SYSTEM_MENUPOPUPEND = 0x0007; public const int EVENT_SYSTEM_CAPTURESTART = 0x0008; public const int EVENT_SYSTEM_CAPTUREEND = 0x0009; public const int EVENT_SYSTEM_SWITCHSTART = 0x0014; public const int EVENT_SYSTEM_SWITCHEND = 0x0015; public const int EVENT_OBJECT_CREATE = 0x8000; public const int EVENT_OBJECT_DESTROY = 0x8001; public const int EVENT_OBJECT_SHOW = 0x8002; public const int EVENT_OBJECT_HIDE = 0x8003; public const int EVENT_OBJECT_FOCUS = 0x8005; public const int EVENT_OBJECT_STATECHANGE = 0x800A; public const int EVENT_OBJECT_LOCATIONCHANGE = 0x800B; public const int WINEVENT_OUTOFCONTEXT = 0x0000; public const int WINEVENT_SKIPOWNTHREAD = 0x0001; public const int WINEVENT_SKIPOWNPROCESS = 0x0002; public const int WINEVENT_INCONTEXT = 0x0004; // WinEvent fired when new Avalon UI is created public const int EventObjectUIFragmentCreate = 0x6FFFFFFF; // the delegate passed to USER for receiving a WinEvent public delegate void WinEventProcDef(int winEventHook, int eventId, IntPtr hwnd, int idObject, int idChild, int eventThread, uint eventTime); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
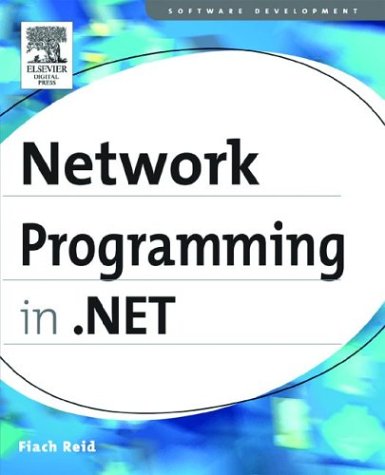
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeRegionDirective.cs
- GenericAuthenticationEventArgs.cs
- SafeFindHandle.cs
- SplitterDesigner.cs
- DataGridViewCellStateChangedEventArgs.cs
- BidirectionalDictionary.cs
- IPPacketInformation.cs
- XamlClipboardData.cs
- DateTimeFormat.cs
- CellTreeNode.cs
- ComplexTypeEmitter.cs
- DrawingVisualDrawingContext.cs
- SafePipeHandle.cs
- QueryContinueDragEvent.cs
- XpsViewerException.cs
- XsdDataContractExporter.cs
- CultureInfoConverter.cs
- WebScriptMetadataMessage.cs
- HeaderFilter.cs
- AsymmetricKeyExchangeDeformatter.cs
- WebAdminConfigurationHelper.cs
- SqlConnectionPoolProviderInfo.cs
- DataKey.cs
- JsonUriDataContract.cs
- TableLayoutPanel.cs
- ServiceNameCollection.cs
- TrackingProfile.cs
- DesignerCommandSet.cs
- LineProperties.cs
- DocumentScope.cs
- Message.cs
- PolyQuadraticBezierSegment.cs
- SqlBulkCopyColumnMappingCollection.cs
- SystemIPInterfaceProperties.cs
- ObjectConverter.cs
- XmlSchemaObject.cs
- PathFigureCollectionConverter.cs
- StructuredType.cs
- HybridCollection.cs
- ObfuscationAttribute.cs
- SymmetricKeyWrap.cs
- GeometryDrawing.cs
- documentsequencetextcontainer.cs
- StatusBarDrawItemEvent.cs
- SemaphoreFullException.cs
- AxImporter.cs
- QuotedPrintableStream.cs
- ListViewInsertEventArgs.cs
- HeaderElement.cs
- CompiledAction.cs
- DocumentViewerAutomationPeer.cs
- TransportChannelListener.cs
- SponsorHelper.cs
- TextProperties.cs
- GridPattern.cs
- PropertyToken.cs
- TransactionContext.cs
- AttachmentService.cs
- ExceptionValidationRule.cs
- Ref.cs
- GeneratedContractType.cs
- ByteConverter.cs
- OdbcParameter.cs
- ListComponentEditor.cs
- SoapTransportImporter.cs
- MediaEntryAttribute.cs
- BitmapEffect.cs
- RawStylusInputReport.cs
- Rule.cs
- GuidConverter.cs
- DataGrid.cs
- ToolStripContainer.cs
- HyperLink.cs
- BaseDataListDesigner.cs
- StoryFragments.cs
- PasswordPropertyTextAttribute.cs
- ListViewItemEventArgs.cs
- ZipIOLocalFileDataDescriptor.cs
- AspCompat.cs
- CustomCategoryAttribute.cs
- MasterPage.cs
- Wizard.cs
- OleDbInfoMessageEvent.cs
- Collection.cs
- TableAutomationPeer.cs
- TemplateInstanceAttribute.cs
- ControlParameter.cs
- SiteMap.cs
- HttpCacheVary.cs
- ConsoleKeyInfo.cs
- UnitySerializationHolder.cs
- MsmqDiagnostics.cs
- Int16KeyFrameCollection.cs
- ByteAnimation.cs
- RoutedUICommand.cs
- LinkButton.cs
- RealProxy.cs
- Int16Storage.cs
- Bold.cs
- _HTTPDateParse.cs