Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / MS / Internal / TextFormatting / TextProperties.cs / 1 / TextProperties.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2001 // // File: TextProperties.cs // // Contents: Properties of text, text line and paragraph // // Created: 2-25-2003 Worachai Chaoweeraprasit (wchao) // //----------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Globalization; using System.Diagnostics; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; using System.Windows.Media.TextFormatting; using MS.Internal; using MS.Internal.Shaping; namespace MS.Internal.TextFormatting { ////// Internal paragraph properties wrapper /// internal sealed class ParaProp { ////// Constructing paragraph properties /// /// Text formatter /// paragraph properties /// produce optimal break internal ParaProp( TextFormatterImp formatter, TextParagraphProperties paragraphProperties, bool optimalBreak ) { _paragraphProperties = paragraphProperties; _emSize = formatter.RealToIdeal(paragraphProperties.DefaultTextRunProperties.FontRenderingEmSize); _indent = formatter.RealToIdeal(paragraphProperties.Indent); _paragraphIndent = formatter.RealToIdeal(paragraphProperties.ParagraphIndent); _height = formatter.RealToIdeal(paragraphProperties.LineHeight); if (_paragraphProperties.FlowDirection == FlowDirection.RightToLeft) { _statusFlags |= StatusFlags.Rtl; } if (optimalBreak) { _statusFlags |= StatusFlags.OptimalBreak; } } [Flags] private enum StatusFlags { Rtl = 0x00000001, // right-to-left reading OptimalBreak = 0x00000002, // produce optimal break } internal bool RightToLeft { get { return (_statusFlags & StatusFlags.Rtl) != 0; } } internal bool OptimalBreak { get { return (_statusFlags & StatusFlags.OptimalBreak) != 0; } } internal bool FirstLineInParagraph { get { return _paragraphProperties.FirstLineInParagraph; } } internal bool AlwaysCollapsible { get { return _paragraphProperties.AlwaysCollapsible; } } internal int Indent { get { return _indent; } } internal int ParagraphIndent { get { return _paragraphIndent; } } internal double DefaultIncrementalTab { get { return _paragraphProperties.DefaultIncrementalTab; } } internal IListTabs { get { return _paragraphProperties.Tabs; } } internal TextAlignment Align { get { return _paragraphProperties.TextAlignment; } } internal bool Justify { get { return _paragraphProperties.TextAlignment == TextAlignment.Justify; } } internal bool EmergencyWrap { get { return _paragraphProperties.TextWrapping == TextWrapping.Wrap; } } internal bool Wrap { get { return _paragraphProperties.TextWrapping == TextWrapping.WrapWithOverflow || EmergencyWrap; } } internal Typeface DefaultTypeface { get { return _paragraphProperties.DefaultTextRunProperties.Typeface; } } internal int EmSize { get { return _emSize; } } internal int LineHeight { get { return _height; } } internal TextMarkerProperties TextMarkerProperties { get { return _paragraphProperties.TextMarkerProperties; } } internal TextLexicalService Hyphenator { get { return _paragraphProperties.Hyphenator; } } internal TextDecorationCollection TextDecorations { get { return _paragraphProperties.TextDecorations; } } internal Brush DefaultTextDecorationsBrush { get { return _paragraphProperties.DefaultTextRunProperties.ForegroundBrush; } } private StatusFlags _statusFlags; private TextParagraphProperties _paragraphProperties; private int _emSize; private int _indent; private int _paragraphIndent; private int _height; } /// /// State of textrun started when it's fetched /// internal sealed class TextRunInfo { private CharacterBufferRange _charBufferRange; private int _textRunLength; private int _offsetToFirstCp; private TextRun _textRun; private Plsrun _plsrun; private CultureInfo _digitCulture; private ushort _charFlags; private ushort _runFlags; private TextModifierScope _modifierScope; private TextRunProperties _properties; ////// Constructing a textrun info /// /// characte buffer range for the run /// textrun length /// character offset to run first cp /// text run /// the internal LS run type /// character attribute flags /// digit culture for the run /// contextual number substitution for the run /// if true, indicates a text run in a symbol (i.e., non-Unicode) font /// The current TextModifier scope for this TextRunInfo internal TextRunInfo( CharacterBufferRange charBufferRange, int textRunLength, int offsetToFirstCp, TextRun textRun, Plsrun lsRunType, ushort charFlags, CultureInfo digitCulture, bool contextualSubstitution, bool symbolTypeface, TextModifierScope modifierScope ) { _charBufferRange = charBufferRange; _textRunLength = textRunLength; _offsetToFirstCp = offsetToFirstCp; _textRun = textRun; _plsrun = lsRunType; _charFlags = charFlags; _digitCulture = digitCulture; _runFlags = 0; _modifierScope = modifierScope; if (contextualSubstitution) { _runFlags |= (ushort)RunFlags.ContextualSubstitution; } if (symbolTypeface) { _runFlags |= (ushort)RunFlags.IsSymbol; } } ////// Text run /// internal TextRun TextRun { get { return _textRun; } } ////// The final TextRunProperties of the TextRun /// internal TextRunProperties Properties { get { // The non-null value is cached if (_properties == null) { if (_modifierScope != null) { _properties = _modifierScope.ModifyProperties(_textRun.Properties); } else { _properties = _textRun.Properties; } } return _properties; } } ////// character buffer /// internal CharacterBuffer CharacterBuffer { get { return _charBufferRange.CharacterBuffer; } } ////// Character offset to run first character in the buffer /// internal int OffsetToFirstChar { get { return _charBufferRange.OffsetToFirstChar; } } ////// Character offset to run first cp from line start /// internal int OffsetToFirstCp { get { return _offsetToFirstCp; } } ////// String length /// internal int StringLength { get { return _charBufferRange.Length; } set { _charBufferRange = new CharacterBufferRange(_charBufferRange.CharacterBufferReference, value); } } ////// Run length /// internal int Length { get { return _textRunLength; } set { _textRunLength = value; } } ////// State of character in the run /// internal ushort CharacterAttributeFlags { get { return _charFlags; } set { _charFlags = value; } } ////// Digit culture for the run. /// internal CultureInfo DigitCulture { get { return _digitCulture; } } ////// Specifies whether the run requires contextual number substitution. /// internal bool ContextualSubstitution { get { return (_runFlags & (ushort)RunFlags.ContextualSubstitution) != 0; } } ////// Specifies whether the run is in a non-Unicode font, such as Symbol or Wingdings. /// ////// Non-Unicode runs require special handling because code points do not have their /// standard Unicode meanings. /// internal bool IsSymbol { get { return (_runFlags & (ushort)RunFlags.IsSymbol) != 0; } } ////// Plsrun type /// internal Plsrun Plsrun { get { return _plsrun; } } ////// Is run an end of line? /// internal bool IsEndOfLine { get { return _textRun is TextEndOfLine; } } ////// The modification scope of this run /// internal TextModifierScope TextModifierScope { get { return _modifierScope; } } ////// Get rough width of the run /// internal int GetRoughWidth(double realToIdeal) { TextRunProperties properties = _textRun.Properties; if (properties != null) { // estimate rough width of each character in a run being 75% of Em. return (int)Math.Round(properties.FontRenderingEmSize * 0.75 * _textRunLength * realToIdeal); } return 0; } ////// Map TextRun type to known plsrun type /// internal static Plsrun GetRunType(TextRun textRun) { if (textRun is ITextSymbols || textRun is TextShapeableSymbols) return Plsrun.Text; if (textRun is TextEmbeddedObject) return Plsrun.InlineObject; if (textRun is TextEndOfParagraph) return Plsrun.ParaBreak; if (textRun is TextEndOfLine) return Plsrun.LineBreak; // Other text run type are all considered hidden by LS return Plsrun.Hidden; } [Flags] private enum RunFlags { ContextualSubstitution = 0x0001, IsSymbol = 0x0002, } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2001 // // File: TextProperties.cs // // Contents: Properties of text, text line and paragraph // // Created: 2-25-2003 Worachai Chaoweeraprasit (wchao) // //----------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Globalization; using System.Diagnostics; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; using System.Windows.Media.TextFormatting; using MS.Internal; using MS.Internal.Shaping; namespace MS.Internal.TextFormatting { ////// Internal paragraph properties wrapper /// internal sealed class ParaProp { ////// Constructing paragraph properties /// /// Text formatter /// paragraph properties /// produce optimal break internal ParaProp( TextFormatterImp formatter, TextParagraphProperties paragraphProperties, bool optimalBreak ) { _paragraphProperties = paragraphProperties; _emSize = formatter.RealToIdeal(paragraphProperties.DefaultTextRunProperties.FontRenderingEmSize); _indent = formatter.RealToIdeal(paragraphProperties.Indent); _paragraphIndent = formatter.RealToIdeal(paragraphProperties.ParagraphIndent); _height = formatter.RealToIdeal(paragraphProperties.LineHeight); if (_paragraphProperties.FlowDirection == FlowDirection.RightToLeft) { _statusFlags |= StatusFlags.Rtl; } if (optimalBreak) { _statusFlags |= StatusFlags.OptimalBreak; } } [Flags] private enum StatusFlags { Rtl = 0x00000001, // right-to-left reading OptimalBreak = 0x00000002, // produce optimal break } internal bool RightToLeft { get { return (_statusFlags & StatusFlags.Rtl) != 0; } } internal bool OptimalBreak { get { return (_statusFlags & StatusFlags.OptimalBreak) != 0; } } internal bool FirstLineInParagraph { get { return _paragraphProperties.FirstLineInParagraph; } } internal bool AlwaysCollapsible { get { return _paragraphProperties.AlwaysCollapsible; } } internal int Indent { get { return _indent; } } internal int ParagraphIndent { get { return _paragraphIndent; } } internal double DefaultIncrementalTab { get { return _paragraphProperties.DefaultIncrementalTab; } } internal IListTabs { get { return _paragraphProperties.Tabs; } } internal TextAlignment Align { get { return _paragraphProperties.TextAlignment; } } internal bool Justify { get { return _paragraphProperties.TextAlignment == TextAlignment.Justify; } } internal bool EmergencyWrap { get { return _paragraphProperties.TextWrapping == TextWrapping.Wrap; } } internal bool Wrap { get { return _paragraphProperties.TextWrapping == TextWrapping.WrapWithOverflow || EmergencyWrap; } } internal Typeface DefaultTypeface { get { return _paragraphProperties.DefaultTextRunProperties.Typeface; } } internal int EmSize { get { return _emSize; } } internal int LineHeight { get { return _height; } } internal TextMarkerProperties TextMarkerProperties { get { return _paragraphProperties.TextMarkerProperties; } } internal TextLexicalService Hyphenator { get { return _paragraphProperties.Hyphenator; } } internal TextDecorationCollection TextDecorations { get { return _paragraphProperties.TextDecorations; } } internal Brush DefaultTextDecorationsBrush { get { return _paragraphProperties.DefaultTextRunProperties.ForegroundBrush; } } private StatusFlags _statusFlags; private TextParagraphProperties _paragraphProperties; private int _emSize; private int _indent; private int _paragraphIndent; private int _height; } /// /// State of textrun started when it's fetched /// internal sealed class TextRunInfo { private CharacterBufferRange _charBufferRange; private int _textRunLength; private int _offsetToFirstCp; private TextRun _textRun; private Plsrun _plsrun; private CultureInfo _digitCulture; private ushort _charFlags; private ushort _runFlags; private TextModifierScope _modifierScope; private TextRunProperties _properties; ////// Constructing a textrun info /// /// characte buffer range for the run /// textrun length /// character offset to run first cp /// text run /// the internal LS run type /// character attribute flags /// digit culture for the run /// contextual number substitution for the run /// if true, indicates a text run in a symbol (i.e., non-Unicode) font /// The current TextModifier scope for this TextRunInfo internal TextRunInfo( CharacterBufferRange charBufferRange, int textRunLength, int offsetToFirstCp, TextRun textRun, Plsrun lsRunType, ushort charFlags, CultureInfo digitCulture, bool contextualSubstitution, bool symbolTypeface, TextModifierScope modifierScope ) { _charBufferRange = charBufferRange; _textRunLength = textRunLength; _offsetToFirstCp = offsetToFirstCp; _textRun = textRun; _plsrun = lsRunType; _charFlags = charFlags; _digitCulture = digitCulture; _runFlags = 0; _modifierScope = modifierScope; if (contextualSubstitution) { _runFlags |= (ushort)RunFlags.ContextualSubstitution; } if (symbolTypeface) { _runFlags |= (ushort)RunFlags.IsSymbol; } } ////// Text run /// internal TextRun TextRun { get { return _textRun; } } ////// The final TextRunProperties of the TextRun /// internal TextRunProperties Properties { get { // The non-null value is cached if (_properties == null) { if (_modifierScope != null) { _properties = _modifierScope.ModifyProperties(_textRun.Properties); } else { _properties = _textRun.Properties; } } return _properties; } } ////// character buffer /// internal CharacterBuffer CharacterBuffer { get { return _charBufferRange.CharacterBuffer; } } ////// Character offset to run first character in the buffer /// internal int OffsetToFirstChar { get { return _charBufferRange.OffsetToFirstChar; } } ////// Character offset to run first cp from line start /// internal int OffsetToFirstCp { get { return _offsetToFirstCp; } } ////// String length /// internal int StringLength { get { return _charBufferRange.Length; } set { _charBufferRange = new CharacterBufferRange(_charBufferRange.CharacterBufferReference, value); } } ////// Run length /// internal int Length { get { return _textRunLength; } set { _textRunLength = value; } } ////// State of character in the run /// internal ushort CharacterAttributeFlags { get { return _charFlags; } set { _charFlags = value; } } ////// Digit culture for the run. /// internal CultureInfo DigitCulture { get { return _digitCulture; } } ////// Specifies whether the run requires contextual number substitution. /// internal bool ContextualSubstitution { get { return (_runFlags & (ushort)RunFlags.ContextualSubstitution) != 0; } } ////// Specifies whether the run is in a non-Unicode font, such as Symbol or Wingdings. /// ////// Non-Unicode runs require special handling because code points do not have their /// standard Unicode meanings. /// internal bool IsSymbol { get { return (_runFlags & (ushort)RunFlags.IsSymbol) != 0; } } ////// Plsrun type /// internal Plsrun Plsrun { get { return _plsrun; } } ////// Is run an end of line? /// internal bool IsEndOfLine { get { return _textRun is TextEndOfLine; } } ////// The modification scope of this run /// internal TextModifierScope TextModifierScope { get { return _modifierScope; } } ////// Get rough width of the run /// internal int GetRoughWidth(double realToIdeal) { TextRunProperties properties = _textRun.Properties; if (properties != null) { // estimate rough width of each character in a run being 75% of Em. return (int)Math.Round(properties.FontRenderingEmSize * 0.75 * _textRunLength * realToIdeal); } return 0; } ////// Map TextRun type to known plsrun type /// internal static Plsrun GetRunType(TextRun textRun) { if (textRun is ITextSymbols || textRun is TextShapeableSymbols) return Plsrun.Text; if (textRun is TextEmbeddedObject) return Plsrun.InlineObject; if (textRun is TextEndOfParagraph) return Plsrun.ParaBreak; if (textRun is TextEndOfLine) return Plsrun.LineBreak; // Other text run type are all considered hidden by LS return Plsrun.Hidden; } [Flags] private enum RunFlags { ContextualSubstitution = 0x0001, IsSymbol = 0x0002, } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
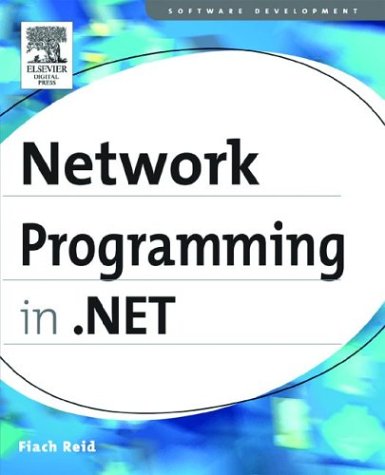
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SingleObjectCollection.cs
- GlyphTypeface.cs
- CalendarButton.cs
- ModuleElement.cs
- Content.cs
- TextEvent.cs
- basevalidator.cs
- WorkflowServiceHostFactory.cs
- TraceXPathNavigator.cs
- DNS.cs
- ClientSettingsStore.cs
- ECDiffieHellmanCngPublicKey.cs
- InheritablePropertyChangeInfo.cs
- ApplicationManager.cs
- GridViewCancelEditEventArgs.cs
- XsdBuilder.cs
- StringCollectionEditor.cs
- ContainerFilterService.cs
- EntityDataSourceWrapper.cs
- XmlDocument.cs
- InvokeMethod.cs
- AnonymousIdentificationModule.cs
- basevalidator.cs
- DataControlButton.cs
- XmlSchemaType.cs
- Size3D.cs
- AssemblyCollection.cs
- LinqDataSourceStatusEventArgs.cs
- Comparer.cs
- TableLayoutStyle.cs
- EncoderBestFitFallback.cs
- PlainXmlDeserializer.cs
- SvcMapFile.cs
- ErrorRuntimeConfig.cs
- OleDbSchemaGuid.cs
- CompoundFileIOPermission.cs
- KoreanLunisolarCalendar.cs
- FigureParagraph.cs
- OutOfProcStateClientManager.cs
- SqlClientWrapperSmiStream.cs
- TransportConfigurationTypeElement.cs
- TableRow.cs
- ErrorEventArgs.cs
- BufferModesCollection.cs
- Visitor.cs
- Rect.cs
- Constraint.cs
- WindowPattern.cs
- NoClickablePointException.cs
- Membership.cs
- SwitchLevelAttribute.cs
- EncoderBestFitFallback.cs
- Adorner.cs
- Brush.cs
- ClickablePoint.cs
- XPathDocumentBuilder.cs
- XmlComment.cs
- EncoderNLS.cs
- DataColumnMappingCollection.cs
- DataTablePropertyDescriptor.cs
- CommandField.cs
- WorkflowApplicationUnloadedException.cs
- AdornerPresentationContext.cs
- SubstitutionList.cs
- QueuedDeliveryRequirementsMode.cs
- HttpApplicationStateWrapper.cs
- DataList.cs
- TransactionManager.cs
- PasswordDeriveBytes.cs
- CharacterBufferReference.cs
- ActivityMarkupSerializationProvider.cs
- Match.cs
- HttpBufferlessInputStream.cs
- BulletChrome.cs
- WebPartManagerDesigner.cs
- ReadOnlyDataSourceView.cs
- DateRangeEvent.cs
- OdbcInfoMessageEvent.cs
- NotifyInputEventArgs.cs
- BackgroundFormatInfo.cs
- CapabilitiesRule.cs
- NamespaceMapping.cs
- RequestCacheManager.cs
- HtmlEncodedRawTextWriter.cs
- GlyphShapingProperties.cs
- SelectorAutomationPeer.cs
- Rect3DConverter.cs
- PageThemeCodeDomTreeGenerator.cs
- ValueProviderWrapper.cs
- FloaterParaClient.cs
- TextCollapsingProperties.cs
- TreeViewItemAutomationPeer.cs
- TdsParser.cs
- NoResizeSelectionBorderGlyph.cs
- _Win32.cs
- ValueQuery.cs
- SendActivityValidator.cs
- CodeNamespaceCollection.cs
- ConfigXmlWhitespace.cs
- CodeIndexerExpression.cs