Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / ContextMenuService.cs / 1305600 / ContextMenuService.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Input; using MS.Internal.KnownBoxes; using System.ComponentModel; namespace System.Windows.Controls { ////// Service class that provides the system implementation for displaying ContextMenus. /// public static class ContextMenuService { #region Attached Properties ////// The DependencyProperty for the ContextMenu property. /// public static readonly DependencyProperty ContextMenuProperty = DependencyProperty.RegisterAttached( "ContextMenu", // Name typeof(ContextMenu), // Type typeof(ContextMenuService), // Owner new FrameworkPropertyMetadata((ContextMenu)null, FrameworkPropertyMetadataOptions.None)); ////// Gets the value of the ContextMenu property on the specified object. /// /// The object on which to query the ContextMenu property. ///The value of the ContextMenu property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static ContextMenu GetContextMenu(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } ContextMenu cm = (ContextMenu)element.GetValue(ContextMenuProperty); if ((cm != null) && (element.Dispatcher != cm.Dispatcher)) { throw new ArgumentException(SR.Get(SRID.ContextMenuInDifferentDispatcher)); } return cm; } ////// Sets the ContextMenu property on the specified object. /// /// The object on which to set the ContextMenu property. /// /// The value of the ContextMenu property. If the value is of type ContextMenu, then /// that is the ContextMenu that will be used (without any modification). If the value /// is of any other type, then that value will be used as the content for a ContextMenu /// provided by this service, and the other attached properties of this service /// will be used to configure the ContextMenu. /// public static void SetContextMenu(DependencyObject element, ContextMenu value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(ContextMenuProperty, value); } ////// The DependencyProperty for the HorizontalOffset property. /// public static readonly DependencyProperty HorizontalOffsetProperty = DependencyProperty.RegisterAttached("HorizontalOffset", // Name typeof(double), // Type typeof(ContextMenuService), // Owner new FrameworkPropertyMetadata(0d)); // Default Value ////// Gets the value of the HorizontalOffset property. /// /// The object on which to query the property. ///The value of the property. [TypeConverter(typeof(LengthConverter))] [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static double GetHorizontalOffset(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (double)element.GetValue(HorizontalOffsetProperty); } ////// Sets the value of the HorizontalOffset property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetHorizontalOffset(DependencyObject element, double value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(HorizontalOffsetProperty, value); } ////// The DependencyProperty for the VerticalOffset property. /// public static readonly DependencyProperty VerticalOffsetProperty = DependencyProperty.RegisterAttached("VerticalOffset", // Name typeof(double), // Type typeof(ContextMenuService), // Owner new FrameworkPropertyMetadata(0d)); // Default Value ////// Gets the value of the VerticalOffset property. /// /// The object on which to query the property. ///The value of the property. [TypeConverter(typeof(LengthConverter))] [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static double GetVerticalOffset(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (double)element.GetValue(VerticalOffsetProperty); } ////// Sets the value of the VerticalOffset property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetVerticalOffset(DependencyObject element, double value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(VerticalOffsetProperty, value); } ////// The DependencyProperty for the HasDropShadow property. /// public static readonly DependencyProperty HasDropShadowProperty = DependencyProperty.RegisterAttached("HasDropShadow", // Name typeof(bool), // Type typeof(ContextMenuService), // Owner new FrameworkPropertyMetadata(BooleanBoxes.FalseBox)); //Default Value ////// Gets the value of the HasDropShadow property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetHasDropShadow(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(HasDropShadowProperty); } ////// Sets the value of the HasDropShadow property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetHasDropShadow(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(HasDropShadowProperty, BooleanBoxes.Box(value)); } ////// The DependencyProperty for the PlacementTarget property. /// public static readonly DependencyProperty PlacementTargetProperty = DependencyProperty.RegisterAttached("PlacementTarget", // Name typeof(UIElement), // Type typeof(ContextMenuService), // Owner new FrameworkPropertyMetadata((UIElement)null)); // Default Value ////// Gets the value of the PlacementTarget property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static UIElement GetPlacementTarget(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (UIElement)element.GetValue(PlacementTargetProperty); } ////// Sets the value of the PlacementTarget property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetPlacementTarget(DependencyObject element, UIElement value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(PlacementTargetProperty, value); } ////// The DependencyProperty for the PlacementRectangle property. /// public static readonly DependencyProperty PlacementRectangleProperty = DependencyProperty.RegisterAttached("PlacementRectangle", // Name typeof(Rect), // Type typeof(ContextMenuService), // Owner new FrameworkPropertyMetadata(Rect.Empty)); // Default Value ////// Gets the value of the PlacementRectangle property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static Rect GetPlacementRectangle(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (Rect)element.GetValue(PlacementRectangleProperty); } ////// Sets the value of the PlacementRectangle property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetPlacementRectangle(DependencyObject element, Rect value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(PlacementRectangleProperty, value); } ////// The DependencyProperty for the Placement property. /// public static readonly DependencyProperty PlacementProperty = DependencyProperty.RegisterAttached("Placement", // Name typeof(PlacementMode), // Type typeof(ContextMenuService), // Owner new FrameworkPropertyMetadata(PlacementMode.MousePoint)); // Default Value ////// Gets the value of the Placement property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static PlacementMode GetPlacement(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (PlacementMode)element.GetValue(PlacementProperty); } ////// Sets the value of the Placement property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetPlacement(DependencyObject element, PlacementMode value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(PlacementProperty, value); } ////// The DependencyProperty for the ShowOnDisabled property. /// public static readonly DependencyProperty ShowOnDisabledProperty = DependencyProperty.RegisterAttached("ShowOnDisabled", // Name typeof(bool), // Type typeof(ContextMenuService), // Owner new FrameworkPropertyMetadata(BooleanBoxes.FalseBox)); // Default Value ////// Gets the value of the ShowOnDisabled property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetShowOnDisabled(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(ShowOnDisabledProperty); } ////// Sets the value of the ShowOnDisabled property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetShowOnDisabled(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(ShowOnDisabledProperty, BooleanBoxes.Box(value)); } ////// The DependencyProperty for the IsEnabled property. /// public static readonly DependencyProperty IsEnabledProperty = DependencyProperty.RegisterAttached("IsEnabled", // Name typeof(bool), // Type typeof(ContextMenuService), // Owner new FrameworkPropertyMetadata(BooleanBoxes.TrueBox)); // Default Value ////// Gets the value of the IsEnabled property. /// /// The object on which to query the property. ///The value of the property. [AttachedPropertyBrowsableForType(typeof(DependencyObject))] public static bool GetIsEnabled(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(IsEnabledProperty); } ////// Sets the value of the IsEnabled property. /// /// The object on which to set the value. /// The desired value of the property. public static void SetIsEnabled(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(IsEnabledProperty, BooleanBoxes.Box(value)); } #endregion #region Events ////// An event that fires just before a ContextMenu should be opened. /// /// To manually open and close ContextMenus, mark this event as handled. /// Otherwise, the value of the the ContextMenu property will be used /// to automatically open a ContextMenu. /// public static readonly RoutedEvent ContextMenuOpeningEvent = EventManager.RegisterRoutedEvent("ContextMenuOpening", RoutingStrategy.Bubble, typeof(ContextMenuEventHandler), typeof(ContextMenuService)); ////// An event that fires just as a ContextMenu closes. /// public static readonly RoutedEvent ContextMenuClosingEvent = EventManager.RegisterRoutedEvent("ContextMenuClosing", RoutingStrategy.Bubble, typeof(ContextMenuEventHandler), typeof(ContextMenuService)); static ContextMenuService() { EventManager.RegisterClassHandler(typeof(UIElement), ContextMenuOpeningEvent, new ContextMenuEventHandler(OnContextMenuOpening)); EventManager.RegisterClassHandler(typeof(ContentElement), ContextMenuOpeningEvent, new ContextMenuEventHandler(OnContextMenuOpening)); EventManager.RegisterClassHandler(typeof(UIElement3D), ContextMenuOpeningEvent, new ContextMenuEventHandler(OnContextMenuOpening)); } private static void OnContextMenuOpening(object sender, ContextMenuEventArgs e) { if (e.TargetElement == null) { DependencyObject o = sender as DependencyObject; if (o != null) { if (ContextMenuIsEnabled(o)) { // Store for later e.TargetElement = o; } } } } #endregion #region Implementation internal static bool ContextMenuIsEnabled(DependencyObject o) { bool contextMenuIsEnabled = false; object menu = GetContextMenu(o); if ((menu != null) && GetIsEnabled(o)) { if (PopupControlService.IsElementEnabled(o) || GetShowOnDisabled(o)) { contextMenuIsEnabled = true; } } return contextMenuIsEnabled; } #endregion } ////// The callback type for handling a ContextMenuEvent /// public delegate void ContextMenuEventHandler(object sender, ContextMenuEventArgs e); ////// The data sent on a ContextMenuEvent /// public sealed class ContextMenuEventArgs : RoutedEventArgs { internal ContextMenuEventArgs(object source, bool opening) : this(source, opening, -1.0, -1.0) { } internal ContextMenuEventArgs(object source, bool opening, double left, double top) { _left = left; _top = top; RoutedEvent =(opening ? ContextMenuService.ContextMenuOpeningEvent : ContextMenuService.ContextMenuClosingEvent); Source = source; } ////// Position (horizontal) that context menu should displayed /// public double CursorLeft { get { return _left; } } ////// Position (vertical) that context menu should displayed /// public double CursorTop { get { return _top; } } internal DependencyObject TargetElement { get { return _targetElement; } set { _targetElement = value; } } ////// Support DynamicInvoke for ContextMenuEvent /// /// /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { ContextMenuEventHandler handler = (ContextMenuEventHandler)genericHandler; handler(genericTarget, this); } private double _left; private double _top; private DependencyObject _targetElement; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
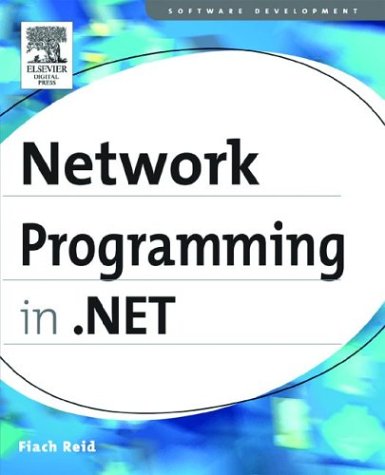
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlClientMetaDataCollectionNames.cs
- DeviceContext.cs
- BaseDataList.cs
- SqlRowUpdatingEvent.cs
- DiscriminatorMap.cs
- ExpressionBuilder.cs
- SynchronizationContextHelper.cs
- CalendarDay.cs
- WebControlsSection.cs
- HttpDictionary.cs
- CompatibleComparer.cs
- EncoderExceptionFallback.cs
- ViewManager.cs
- Journaling.cs
- WebException.cs
- TableLayoutPanelBehavior.cs
- XmlDesignerDataSourceView.cs
- AnnotationStore.cs
- HtmlTableRow.cs
- FocusWithinProperty.cs
- AssociationTypeEmitter.cs
- ScalarType.cs
- TypeBuilder.cs
- StrongNameUtility.cs
- WebPartEditorCancelVerb.cs
- WindowsListViewScroll.cs
- StringUtil.cs
- PropertyEmitter.cs
- DataFormat.cs
- PhysicalAddress.cs
- OracleParameter.cs
- ObjectCloneHelper.cs
- UnmanagedHandle.cs
- uribuilder.cs
- DataGridTableCollection.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- VectorAnimationBase.cs
- WebContext.cs
- CombinedTcpChannel.cs
- Span.cs
- PtsPage.cs
- SafeMILHandleMemoryPressure.cs
- xdrvalidator.cs
- StringCollectionEditor.cs
- DoubleStorage.cs
- CqlParser.cs
- GeometryCollection.cs
- Missing.cs
- ScriptingProfileServiceSection.cs
- LowerCaseStringConverter.cs
- CultureData.cs
- DrawingBrush.cs
- StaticTextPointer.cs
- DataGridViewTextBoxCell.cs
- PositiveTimeSpanValidatorAttribute.cs
- GifBitmapDecoder.cs
- XmlWrappingReader.cs
- SafePointer.cs
- SqlTriggerAttribute.cs
- ViewGenerator.cs
- IdentityReference.cs
- BuildManager.cs
- Avt.cs
- DbProviderFactory.cs
- SecurityKeyEntropyMode.cs
- StylusOverProperty.cs
- StoreContentChangedEventArgs.cs
- PersonalizableTypeEntry.cs
- DivideByZeroException.cs
- HTTPNotFoundHandler.cs
- LayoutSettings.cs
- DataTablePropertyDescriptor.cs
- IsolatedStorageFileStream.cs
- WindowsScrollBar.cs
- MdiWindowListItemConverter.cs
- RuntimeConfig.cs
- Matrix3DStack.cs
- WindowsFormsSynchronizationContext.cs
- StorageModelBuildProvider.cs
- StreamGeometry.cs
- FlowLayoutSettings.cs
- ItemCheckedEvent.cs
- ClientScriptManager.cs
- WebConvert.cs
- WindowAutomationPeer.cs
- SystemThemeKey.cs
- DynamicRendererThreadManager.cs
- NameValueCollection.cs
- WindowsFormsHelpers.cs
- CollectionsUtil.cs
- BitmapMetadataEnumerator.cs
- CellLabel.cs
- OutOfProcStateClientManager.cs
- IdleTimeoutMonitor.cs
- HuffModule.cs
- WrappedReader.cs
- FrameworkContextData.cs
- QueryableDataSourceHelper.cs
- BinaryObjectReader.cs
- EventHandlersStore.cs