Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / xsp / System / Web / Extensions / ui / ScriptReference.cs / 3 / ScriptReference.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections; using System.ComponentModel; using System.Diagnostics.CodeAnalysis; using System.Globalization; using System.Reflection; using System.Security.Permissions; using System.Web; using System.Web.Handlers; using System.Web.Resources; using System.Web.Util; using Debug = System.Diagnostics.Debug; [ AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal), AspNetHostingPermission(SecurityAction.InheritanceDemand, Level = AspNetHostingPermissionLevel.Minimal), DefaultProperty("Path"), ] public class ScriptReference : ScriptReferenceBase { // Maps Pair(resource name, assembly) to string (partial script path) private static readonly Hashtable _scriptPathCache = Hashtable.Synchronized(new Hashtable()); private string _assembly; private bool _ignoreScriptPath; private string _name; public ScriptReference() : base() {} public ScriptReference(string name, string assembly) : this() { Name = name; Assembly = assembly; } public ScriptReference(string path) : this() { Path = path; } internal ScriptReference(string name, IClientUrlResolver clientUrlResolver, Control containingControl) : this() { Debug.Assert(!String.IsNullOrEmpty(name), "The script's name must be specified."); Debug.Assert(clientUrlResolver != null && clientUrlResolver is ScriptManager, "The clientUrlResolver must be the ScriptManager."); Name = name; ClientUrlResolver = clientUrlResolver; IsStaticReference = true; ContainingControl = containingControl; } [ Category("Behavior"), DefaultValue(""), ResourceDescription("ScriptReference_Assembly") ] public string Assembly { get { return (_assembly == null) ? String.Empty : _assembly; } set { _assembly = value; } } internal ScriptMode EffectiveScriptMode { get { if (ScriptMode == ScriptMode.Auto) { // - When only Path is specified, ScriptMode.Auto is equivalent to ScriptMode.Release. // - When only Name is specified, ScriptMode.Auto is equivalent to ScriptMode.Inherit. // - When Name and Path are both specified, the Path is used instead of the Name, but // ScriptMode.Auto is still equivalent to ScriptMode.Inherit, since the assumption // is that if the Assembly contains both release and debug scripts, the Path should // contain both as well. return (String.IsNullOrEmpty(Name) ? ScriptMode.Release : ScriptMode.Inherit); } else { return ScriptMode; } } } [ Category("Behavior"), DefaultValue(false), ResourceDescription("ScriptReference_IgnoreScriptPath") ] public bool IgnoreScriptPath { get { return _ignoreScriptPath; } set { _ignoreScriptPath = value; } } [ Category("Behavior"), DefaultValue(""), ResourceDescription("ScriptReference_Name") ] public string Name { get { return (_name == null) ? String.Empty : _name; } set { _name = value; } } internal CultureInfo DetermineCulture() { if ((ResourceUICultures == null) || (ResourceUICultures.Length == 0)) { // In this case we want to determine available cultures from assembly info if available if (!String.IsNullOrEmpty(Name)) { return ScriptResourceHandler .DetermineNearestAvailableCulture(GetAssembly(), Name, CultureInfo.CurrentUICulture); } return CultureInfo.InvariantCulture; } CultureInfo currentCulture = CultureInfo.CurrentUICulture; while (!currentCulture.Equals(CultureInfo.InvariantCulture)) { string cultureName = currentCulture.ToString(); foreach (string uiCulture in ResourceUICultures) { if (String.Equals(cultureName, uiCulture.Trim(), StringComparison.OrdinalIgnoreCase)) { return currentCulture; } } currentCulture = currentCulture.Parent; } return currentCulture; } internal Assembly GetAssembly() { string assemblyName = Assembly; if (String.IsNullOrEmpty(assemblyName)) { return AssemblyCache.SystemWebExtensions; } else { // Use AssemblyCache instead of directly calling Assembly.Load(), for improved perf. // Increases requests/second by over 150% in ScriptManagerAssembly.aspx test. return AssemblyCache.Load(assemblyName); } } // Release: foo.js // Debug: foo.debug.js private static string GetDebugName(string releaseName) { // Since System.Web.Handlers.AssemblyResourceLoader treats the resource name as case-sensitive, // we must do the same when verifying the extension. // Ignore trailing whitespace. For example, "MicrosoftAjax.js " is valid (at least from // a debug/release naming perspective). if (!releaseName.EndsWith(".js", StringComparison.Ordinal)) { throw new InvalidOperationException( String.Format(CultureInfo.CurrentUICulture, AtlasWeb.ScriptReference_InvalidReleaseScriptName, releaseName)); } return ReplaceExtension(releaseName); } internal string GetPath(string releasePath, bool isDebuggingEnabled) { if (String.IsNullOrEmpty(Name)) { return GetPathWithoutName(releasePath, isDebuggingEnabled); } else { return GetPathWithName(releasePath, isDebuggingEnabled); } } // When Name is empty, there is no Name/Assembly logic involved. private string GetPathWithoutName(string releasePath, bool isDebuggingEnabled) { if (isDebuggingEnabled) { return GetDebugPath(releasePath); } else { return releasePath; } } // When Name and Path are both specified, the Path is used instead of the Name, but we // still verify that the named resource exists in the assembly. We also look inside the // assembly to determine whether to use the debug or release script. The assumption is // that if the Assembly contains only a release script, the path should contain only a // release script as well. private string GetPathWithName(string releasePath, bool isDebuggingEnabled) { if (ShouldUseDebugScript(Name, GetAssembly(), isDebuggingEnabled)) { return GetDebugPath(releasePath); } else { return releasePath; } } internal string GetResourceName(string releaseName, Assembly assembly, bool isDebuggingEnabled) { string resourceName; if (ShouldUseDebugScript(releaseName, assembly, isDebuggingEnabled)) { resourceName = GetDebugName(releaseName); } else { resourceName = releaseName; } return resourceName; } // Format: / / / // This function does not canonicalize the path in any way (i.e. remove duplicate slashes). // You must call ResolveClientUrl() on this path before rendering to the page. internal static string GetScriptPath( string resourceName, Assembly assembly, CultureInfo culture, string scriptPath) { return scriptPath + "/" + GetScriptPathCached(resourceName, assembly, culture); } // Cache partial script path, since Version.ToString() and HttpUtility.UrlEncode() are expensive. // Increases requests/second by 50% in ScriptManagerScriptPath.aspx test. private static string GetScriptPathCached(string resourceName, Assembly assembly, CultureInfo culture) { Tuple key = new Tuple(resourceName, assembly, culture); string scriptPath = (string)_scriptPathCache[key]; if (scriptPath == null) { // Need to use "new AssemblyName(assembly.FullName)" instead of "assembly.GetName()", // since Assembly.GetName() requires FileIOPermission to the path of the assembly. // In partial trust, we may not have this permission. AssemblyName assemblyName = new AssemblyName(assembly.FullName); string name = assemblyName.Name; string version = assemblyName.Version.ToString(); string fileVersion = AssemblyUtil.GetAssemblyFileVersion(assembly); if ((!culture.Equals(CultureInfo.InvariantCulture)) && resourceName.EndsWith(".js", StringComparison.OrdinalIgnoreCase)) { resourceName = resourceName.Substring(0, resourceName.Length - 3) + "." + culture.Name + ".js"; } // Assembly name, fileVersion, and resource name may contain invalid URL characters (like '#' or '/'), // so they must be url-encoded. scriptPath = String.Join("/", new string[] { HttpUtility.UrlEncode(name), version, HttpUtility.UrlEncode(fileVersion), HttpUtility.UrlEncode(resourceName) }); _scriptPathCache[key] = scriptPath; } return scriptPath; } [SuppressMessage("Microsoft.Design", "CA1055", Justification = "Consistent with other URL properties in ASP.NET.")] protected internal override string GetUrl(ScriptManager scriptManager, bool zip) { bool hasName = !String.IsNullOrEmpty(Name); bool hasAssembly = !String.IsNullOrEmpty(Assembly); bool hasPath = !String.IsNullOrEmpty(Path); if (!hasName && !hasPath) { throw new InvalidOperationException(AtlasWeb.ScriptReference_NameAndPathCannotBeEmpty); } if (hasAssembly && !hasName) { throw new InvalidOperationException(AtlasWeb.ScriptReference_AssemblyRequiresName); } // If Path+Name are specified, Path overrides Name. if (hasPath) { return GetUrlFromPath(scriptManager); } else { Debug.Assert(hasName); return GetUrlFromName(scriptManager, scriptManager.Control, zip); } } private string GetUrlFromName(ScriptManager scriptManager, IControl scriptManagerControl, bool zip) { string releaseName = Name; Assembly assembly = GetAssembly(); // GetResourceName() throws exception if the resource name does not exist in the assembly string resourceName = GetResourceName(releaseName, assembly, IsDebuggingEnabled(scriptManager)); string url; CultureInfo culture = (scriptManager.EnableScriptLocalization ? DetermineCulture() : CultureInfo.InvariantCulture); if (IgnoreScriptPath || String.IsNullOrEmpty(scriptManager.ScriptPath)) { url = ScriptResourceHandler .GetScriptResourceUrl(assembly, resourceName, culture, zip, NotifyScriptLoaded); } else { string path = GetScriptPath(resourceName, assembly, culture, scriptManager.ScriptPath); // Always want to resolve ScriptPath urls against the ScriptManager itself, // regardless of whether the ScriptReference was declared on the ScriptManager // or a ScriptManagerProxy. url = scriptManagerControl.ResolveClientUrl(path); } return url; } private string GetUrlFromPath(ScriptManager scriptManager) { string releasePath = Path; string path = GetPath(releasePath, IsDebuggingEnabled(scriptManager)); // Once the path has been determined, add localization if necessary if (scriptManager.EnableScriptLocalization) { CultureInfo culture = DetermineCulture(); if (!culture.Equals(CultureInfo.InvariantCulture)) { path = (path.Substring(0, path.Length - 2) + culture.ToString() + ".js"); } } string resolvedUrl = ClientUrlResolver.ResolveClientUrl(path); return resolvedUrl; } private bool IsDebuggingEnabled(ScriptManager scriptManager) { // Deployment mode retail overrides all values of ScriptReference.ScriptMode. if (scriptManager.DeploymentSectionRetail) { return false; } switch (EffectiveScriptMode) { case ScriptMode.Inherit: return scriptManager.IsDebuggingEnabled; case ScriptMode.Debug: return true; case ScriptMode.Release: return false; default: Debug.Fail("Invalid value for ScriptReference.EffectiveScriptMode"); return false; } } protected internal override bool IsFromSystemWebExtensions() { return (GetAssembly() == AssemblyCache.SystemWebExtensions); } internal bool ShouldUseDebugScript(string releaseName, Assembly assembly, bool isDebuggingEnabled) { bool useDebugScript; string debugName = null; if (isDebuggingEnabled) { debugName = GetDebugName(releaseName); // If an assembly contains a release script but not a corresponding debug script, and we // need to register the debug script, we normally throw an exception. However, we automatically // use the release script if ScriptReference.ScriptMode is Auto. This improves the developer // experience when ScriptMode is Auto, yet still gives the developer full control with the // other ScriptModes. if (ScriptMode == ScriptMode.Auto && !WebResourceUtil.AssemblyContainsWebResource(assembly, debugName)) { useDebugScript = false; } else { useDebugScript = true; } } else { useDebugScript = false; } // Verify that assembly contains required web resources. Always check for release // script before debug script. WebResourceUtil.VerifyAssemblyContainsReleaseWebResource(assembly, releaseName); if (useDebugScript) { Debug.Assert(debugName != null); WebResourceUtil.VerifyAssemblyContainsDebugWebResource(assembly, debugName); } return useDebugScript; } // Improves the UI in the VS collection editor, by displaying the Name or Path (if available), or // the short type name. [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public override string ToString() { if (!String.IsNullOrEmpty(Name)) { return Name; } else if (!String.IsNullOrEmpty(Path)) { return Path; } else { return GetType().Name; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections; using System.ComponentModel; using System.Diagnostics.CodeAnalysis; using System.Globalization; using System.Reflection; using System.Security.Permissions; using System.Web; using System.Web.Handlers; using System.Web.Resources; using System.Web.Util; using Debug = System.Diagnostics.Debug; [ AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal), AspNetHostingPermission(SecurityAction.InheritanceDemand, Level = AspNetHostingPermissionLevel.Minimal), DefaultProperty("Path"), ] public class ScriptReference : ScriptReferenceBase { // Maps Pair(resource name, assembly) to string (partial script path) private static readonly Hashtable _scriptPathCache = Hashtable.Synchronized(new Hashtable()); private string _assembly; private bool _ignoreScriptPath; private string _name; public ScriptReference() : base() {} public ScriptReference(string name, string assembly) : this() { Name = name; Assembly = assembly; } public ScriptReference(string path) : this() { Path = path; } internal ScriptReference(string name, IClientUrlResolver clientUrlResolver, Control containingControl) : this() { Debug.Assert(!String.IsNullOrEmpty(name), "The script's name must be specified."); Debug.Assert(clientUrlResolver != null && clientUrlResolver is ScriptManager, "The clientUrlResolver must be the ScriptManager."); Name = name; ClientUrlResolver = clientUrlResolver; IsStaticReference = true; ContainingControl = containingControl; } [ Category("Behavior"), DefaultValue(""), ResourceDescription("ScriptReference_Assembly") ] public string Assembly { get { return (_assembly == null) ? String.Empty : _assembly; } set { _assembly = value; } } internal ScriptMode EffectiveScriptMode { get { if (ScriptMode == ScriptMode.Auto) { // - When only Path is specified, ScriptMode.Auto is equivalent to ScriptMode.Release. // - When only Name is specified, ScriptMode.Auto is equivalent to ScriptMode.Inherit. // - When Name and Path are both specified, the Path is used instead of the Name, but // ScriptMode.Auto is still equivalent to ScriptMode.Inherit, since the assumption // is that if the Assembly contains both release and debug scripts, the Path should // contain both as well. return (String.IsNullOrEmpty(Name) ? ScriptMode.Release : ScriptMode.Inherit); } else { return ScriptMode; } } } [ Category("Behavior"), DefaultValue(false), ResourceDescription("ScriptReference_IgnoreScriptPath") ] public bool IgnoreScriptPath { get { return _ignoreScriptPath; } set { _ignoreScriptPath = value; } } [ Category("Behavior"), DefaultValue(""), ResourceDescription("ScriptReference_Name") ] public string Name { get { return (_name == null) ? String.Empty : _name; } set { _name = value; } } internal CultureInfo DetermineCulture() { if ((ResourceUICultures == null) || (ResourceUICultures.Length == 0)) { // In this case we want to determine available cultures from assembly info if available if (!String.IsNullOrEmpty(Name)) { return ScriptResourceHandler .DetermineNearestAvailableCulture(GetAssembly(), Name, CultureInfo.CurrentUICulture); } return CultureInfo.InvariantCulture; } CultureInfo currentCulture = CultureInfo.CurrentUICulture; while (!currentCulture.Equals(CultureInfo.InvariantCulture)) { string cultureName = currentCulture.ToString(); foreach (string uiCulture in ResourceUICultures) { if (String.Equals(cultureName, uiCulture.Trim(), StringComparison.OrdinalIgnoreCase)) { return currentCulture; } } currentCulture = currentCulture.Parent; } return currentCulture; } internal Assembly GetAssembly() { string assemblyName = Assembly; if (String.IsNullOrEmpty(assemblyName)) { return AssemblyCache.SystemWebExtensions; } else { // Use AssemblyCache instead of directly calling Assembly.Load(), for improved perf. // Increases requests/second by over 150% in ScriptManagerAssembly.aspx test. return AssemblyCache.Load(assemblyName); } } // Release: foo.js // Debug: foo.debug.js private static string GetDebugName(string releaseName) { // Since System.Web.Handlers.AssemblyResourceLoader treats the resource name as case-sensitive, // we must do the same when verifying the extension. // Ignore trailing whitespace. For example, "MicrosoftAjax.js " is valid (at least from // a debug/release naming perspective). if (!releaseName.EndsWith(".js", StringComparison.Ordinal)) { throw new InvalidOperationException( String.Format(CultureInfo.CurrentUICulture, AtlasWeb.ScriptReference_InvalidReleaseScriptName, releaseName)); } return ReplaceExtension(releaseName); } internal string GetPath(string releasePath, bool isDebuggingEnabled) { if (String.IsNullOrEmpty(Name)) { return GetPathWithoutName(releasePath, isDebuggingEnabled); } else { return GetPathWithName(releasePath, isDebuggingEnabled); } } // When Name is empty, there is no Name/Assembly logic involved. private string GetPathWithoutName(string releasePath, bool isDebuggingEnabled) { if (isDebuggingEnabled) { return GetDebugPath(releasePath); } else { return releasePath; } } // When Name and Path are both specified, the Path is used instead of the Name, but we // still verify that the named resource exists in the assembly. We also look inside the // assembly to determine whether to use the debug or release script. The assumption is // that if the Assembly contains only a release script, the path should contain only a // release script as well. private string GetPathWithName(string releasePath, bool isDebuggingEnabled) { if (ShouldUseDebugScript(Name, GetAssembly(), isDebuggingEnabled)) { return GetDebugPath(releasePath); } else { return releasePath; } } internal string GetResourceName(string releaseName, Assembly assembly, bool isDebuggingEnabled) { string resourceName; if (ShouldUseDebugScript(releaseName, assembly, isDebuggingEnabled)) { resourceName = GetDebugName(releaseName); } else { resourceName = releaseName; } return resourceName; } // Format: / / / // This function does not canonicalize the path in any way (i.e. remove duplicate slashes). // You must call ResolveClientUrl() on this path before rendering to the page. internal static string GetScriptPath( string resourceName, Assembly assembly, CultureInfo culture, string scriptPath) { return scriptPath + "/" + GetScriptPathCached(resourceName, assembly, culture); } // Cache partial script path, since Version.ToString() and HttpUtility.UrlEncode() are expensive. // Increases requests/second by 50% in ScriptManagerScriptPath.aspx test. private static string GetScriptPathCached(string resourceName, Assembly assembly, CultureInfo culture) { Tuple key = new Tuple(resourceName, assembly, culture); string scriptPath = (string)_scriptPathCache[key]; if (scriptPath == null) { // Need to use "new AssemblyName(assembly.FullName)" instead of "assembly.GetName()", // since Assembly.GetName() requires FileIOPermission to the path of the assembly. // In partial trust, we may not have this permission. AssemblyName assemblyName = new AssemblyName(assembly.FullName); string name = assemblyName.Name; string version = assemblyName.Version.ToString(); string fileVersion = AssemblyUtil.GetAssemblyFileVersion(assembly); if ((!culture.Equals(CultureInfo.InvariantCulture)) && resourceName.EndsWith(".js", StringComparison.OrdinalIgnoreCase)) { resourceName = resourceName.Substring(0, resourceName.Length - 3) + "." + culture.Name + ".js"; } // Assembly name, fileVersion, and resource name may contain invalid URL characters (like '#' or '/'), // so they must be url-encoded. scriptPath = String.Join("/", new string[] { HttpUtility.UrlEncode(name), version, HttpUtility.UrlEncode(fileVersion), HttpUtility.UrlEncode(resourceName) }); _scriptPathCache[key] = scriptPath; } return scriptPath; } [SuppressMessage("Microsoft.Design", "CA1055", Justification = "Consistent with other URL properties in ASP.NET.")] protected internal override string GetUrl(ScriptManager scriptManager, bool zip) { bool hasName = !String.IsNullOrEmpty(Name); bool hasAssembly = !String.IsNullOrEmpty(Assembly); bool hasPath = !String.IsNullOrEmpty(Path); if (!hasName && !hasPath) { throw new InvalidOperationException(AtlasWeb.ScriptReference_NameAndPathCannotBeEmpty); } if (hasAssembly && !hasName) { throw new InvalidOperationException(AtlasWeb.ScriptReference_AssemblyRequiresName); } // If Path+Name are specified, Path overrides Name. if (hasPath) { return GetUrlFromPath(scriptManager); } else { Debug.Assert(hasName); return GetUrlFromName(scriptManager, scriptManager.Control, zip); } } private string GetUrlFromName(ScriptManager scriptManager, IControl scriptManagerControl, bool zip) { string releaseName = Name; Assembly assembly = GetAssembly(); // GetResourceName() throws exception if the resource name does not exist in the assembly string resourceName = GetResourceName(releaseName, assembly, IsDebuggingEnabled(scriptManager)); string url; CultureInfo culture = (scriptManager.EnableScriptLocalization ? DetermineCulture() : CultureInfo.InvariantCulture); if (IgnoreScriptPath || String.IsNullOrEmpty(scriptManager.ScriptPath)) { url = ScriptResourceHandler .GetScriptResourceUrl(assembly, resourceName, culture, zip, NotifyScriptLoaded); } else { string path = GetScriptPath(resourceName, assembly, culture, scriptManager.ScriptPath); // Always want to resolve ScriptPath urls against the ScriptManager itself, // regardless of whether the ScriptReference was declared on the ScriptManager // or a ScriptManagerProxy. url = scriptManagerControl.ResolveClientUrl(path); } return url; } private string GetUrlFromPath(ScriptManager scriptManager) { string releasePath = Path; string path = GetPath(releasePath, IsDebuggingEnabled(scriptManager)); // Once the path has been determined, add localization if necessary if (scriptManager.EnableScriptLocalization) { CultureInfo culture = DetermineCulture(); if (!culture.Equals(CultureInfo.InvariantCulture)) { path = (path.Substring(0, path.Length - 2) + culture.ToString() + ".js"); } } string resolvedUrl = ClientUrlResolver.ResolveClientUrl(path); return resolvedUrl; } private bool IsDebuggingEnabled(ScriptManager scriptManager) { // Deployment mode retail overrides all values of ScriptReference.ScriptMode. if (scriptManager.DeploymentSectionRetail) { return false; } switch (EffectiveScriptMode) { case ScriptMode.Inherit: return scriptManager.IsDebuggingEnabled; case ScriptMode.Debug: return true; case ScriptMode.Release: return false; default: Debug.Fail("Invalid value for ScriptReference.EffectiveScriptMode"); return false; } } protected internal override bool IsFromSystemWebExtensions() { return (GetAssembly() == AssemblyCache.SystemWebExtensions); } internal bool ShouldUseDebugScript(string releaseName, Assembly assembly, bool isDebuggingEnabled) { bool useDebugScript; string debugName = null; if (isDebuggingEnabled) { debugName = GetDebugName(releaseName); // If an assembly contains a release script but not a corresponding debug script, and we // need to register the debug script, we normally throw an exception. However, we automatically // use the release script if ScriptReference.ScriptMode is Auto. This improves the developer // experience when ScriptMode is Auto, yet still gives the developer full control with the // other ScriptModes. if (ScriptMode == ScriptMode.Auto && !WebResourceUtil.AssemblyContainsWebResource(assembly, debugName)) { useDebugScript = false; } else { useDebugScript = true; } } else { useDebugScript = false; } // Verify that assembly contains required web resources. Always check for release // script before debug script. WebResourceUtil.VerifyAssemblyContainsReleaseWebResource(assembly, releaseName); if (useDebugScript) { Debug.Assert(debugName != null); WebResourceUtil.VerifyAssemblyContainsDebugWebResource(assembly, debugName); } return useDebugScript; } // Improves the UI in the VS collection editor, by displaying the Name or Path (if available), or // the short type name. [SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase")] public override string ToString() { if (!String.IsNullOrEmpty(Name)) { return Name; } else if (!String.IsNullOrEmpty(Path)) { return Path; } else { return GetType().Name; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
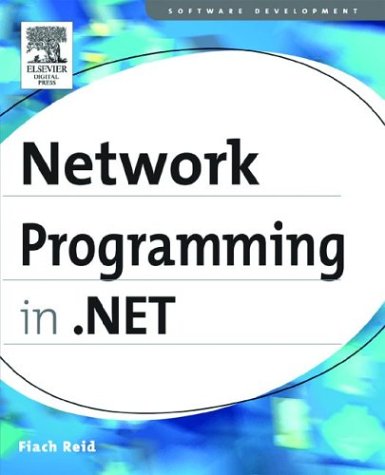
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AnonymousIdentificationSection.cs
- ProfileBuildProvider.cs
- CommandManager.cs
- XmlSerializerNamespaces.cs
- HtmlTextBoxAdapter.cs
- Dump.cs
- LocalizableAttribute.cs
- TypedTableBaseExtensions.cs
- AutomationProperties.cs
- DbModificationCommandTree.cs
- AuthStoreRoleProvider.cs
- ProfileProvider.cs
- DataControlExtensions.cs
- FlowDocumentView.cs
- DataRowChangeEvent.cs
- DataSourceDesigner.cs
- URIFormatException.cs
- IsolatedStorageSecurityState.cs
- XmlSchemaSimpleTypeRestriction.cs
- FileDialogCustomPlace.cs
- AutomationElementCollection.cs
- PointLight.cs
- CompilerCollection.cs
- FixedSOMPageConstructor.cs
- LineMetrics.cs
- HighContrastHelper.cs
- ProbeMatchesApril2005.cs
- UnknownWrapper.cs
- AnnotationResourceCollection.cs
- Expressions.cs
- SqlConnection.cs
- HttpChannelHelper.cs
- EmissiveMaterial.cs
- ExpandSegment.cs
- RealizationContext.cs
- RtType.cs
- ImpersonationContext.cs
- PeerFlooder.cs
- ValidatorCompatibilityHelper.cs
- LogicalExpr.cs
- TemplateBaseAction.cs
- Confirm.cs
- ProjectionCamera.cs
- BaseValidator.cs
- WasAdminWrapper.cs
- CompilationLock.cs
- RNGCryptoServiceProvider.cs
- AssemblyAttributesGoHere.cs
- MULTI_QI.cs
- TableAutomationPeer.cs
- TemplateAction.cs
- DiscreteKeyFrames.cs
- ShaderEffect.cs
- WsatProxy.cs
- AuthenticationModuleElementCollection.cs
- CompositionDesigner.cs
- InvariantComparer.cs
- WebControl.cs
- DispatcherProcessingDisabled.cs
- TagPrefixAttribute.cs
- EntityViewGenerationConstants.cs
- SqlDataSourceCommandEventArgs.cs
- NavigationWindowAutomationPeer.cs
- PointLight.cs
- InvokeProviderWrapper.cs
- SetStoryboardSpeedRatio.cs
- SqlMetaData.cs
- RefreshPropertiesAttribute.cs
- ProgressiveCrcCalculatingStream.cs
- TaskFileService.cs
- _AuthenticationState.cs
- BindingGraph.cs
- IconConverter.cs
- SerializerProvider.cs
- WizardForm.cs
- DataFormats.cs
- PathFigureCollection.cs
- EmptyTextWriter.cs
- DependencyPropertyKind.cs
- CodeExpressionRuleDeclaration.cs
- CallId.cs
- GenerateTemporaryTargetAssembly.cs
- WithParamAction.cs
- DetailsViewRowCollection.cs
- WindowsImpersonationContext.cs
- WasEndpointConfigContainer.cs
- _CacheStreams.cs
- IisTraceListener.cs
- EventLogPermissionAttribute.cs
- SemanticAnalyzer.cs
- HwndSourceParameters.cs
- CookieProtection.cs
- FixedTextPointer.cs
- ObjectNavigationPropertyMapping.cs
- ListViewGroup.cs
- LicenseException.cs
- MobileControlsSectionHandler.cs
- RulePatternOps.cs
- ConfigUtil.cs
- SolidColorBrush.cs