Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / HttpCacheParams.cs / 2 / HttpCacheParams.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Cache Vary class. Wraps Vary header * * Copyright (c) 1998 Microsoft Corporation */ namespace System.Web { using System.Text; using System.Runtime.InteropServices; using System.Web.Util; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class HttpCacheVaryByParams { HttpDictionary _parameters; int _ignoreParams; bool _isModified; bool _paramsStar; internal HttpCacheVaryByParams() { Reset(); } internal void Reset() { _isModified = false; _paramsStar = false; _parameters = null; _ignoreParams = -1; } /* * Reset based on the cached vary headers. */ internal void ResetFromParams(String[] parameters) { int i, n; Reset(); if (parameters != null) { _isModified = true; if (parameters[0].Length == 0) { Debug.Assert(parameters.Length == 1, "parameters.Length == 1"); IgnoreParams = true; } else if (parameters[0].Equals("*")) { Debug.Assert(parameters.Length == 1, "parameters.Length == 1"); _paramsStar = true; } else { _parameters = new HttpDictionary(); for (i = 0, n = parameters.Length; i < n; i++) { _parameters.SetValue(parameters[i], parameters[i]); } } } } internal bool IsModified() { return _isModified; } internal bool AcceptsParams() { return _ignoreParams == 1 || _paramsStar || _parameters != null; } internal String[] GetParams() { String[] s = null; Object item; int i, j, c, n; if (_ignoreParams == 1) { s = new String[1] {String.Empty}; } else if (_paramsStar) { s = new String[1] {"*"}; } else if (_parameters != null) { n = _parameters.Size; c = 0; for (i = 0; i < n; i++) { item = _parameters.GetValue(i); if (item != null) { c++; } } if (c > 0) { s = new string[c]; j = 0; for (i = 0; i < n; i++) { item = _parameters.GetValue(i); if (item != null) { s[j] = (String) item; j++; } } Debug.Assert(j == c, "j == c"); } } return s; } // // Public methods and properties // ///Indicates that a cache should contain multiple /// representations for a particular Uri. This class is an encapsulation that /// provides a rich, type-safe way to set the Vary header. ////// public bool this[String header] { get { if (header == null) { throw new ArgumentNullException("header"); } if (header.Length == 0) { return _ignoreParams == 1; } else { return _paramsStar || (_parameters != null && _parameters.GetValue(header) != null); } } set { if (header == null) { throw new ArgumentNullException("header"); } if (header.Length == 0) { IgnoreParams = value; } /* * Since adding a Vary parameter is more restrictive, we don't * want components to be able to set a Vary parameter to false * if another component has set it to true. */ else if (value) { _isModified = true; _ignoreParams = 0; if (header.Equals("*")) { _paramsStar = true; _parameters = null; } else { // set value to header if true or null if false if (!_paramsStar) { if (_parameters == null) { _parameters = new HttpDictionary(); } _parameters.SetValue(header, header); } } } } } public bool IgnoreParams { get { return _ignoreParams == 1; } set { // Don't ignore if params have been added if (_paramsStar || _parameters != null) { return; } if (_ignoreParams == -1 || _ignoreParams == 1) { _ignoreParams = value ? 1 : 0; _isModified = true; } } } internal bool IsVaryByStar { get { return _paramsStar; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //Default property. /// Indexed property indicating that a cache should (or should not) vary according /// to a custom header. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Cache Vary class. Wraps Vary header * * Copyright (c) 1998 Microsoft Corporation */ namespace System.Web { using System.Text; using System.Runtime.InteropServices; using System.Web.Util; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class HttpCacheVaryByParams { HttpDictionary _parameters; int _ignoreParams; bool _isModified; bool _paramsStar; internal HttpCacheVaryByParams() { Reset(); } internal void Reset() { _isModified = false; _paramsStar = false; _parameters = null; _ignoreParams = -1; } /* * Reset based on the cached vary headers. */ internal void ResetFromParams(String[] parameters) { int i, n; Reset(); if (parameters != null) { _isModified = true; if (parameters[0].Length == 0) { Debug.Assert(parameters.Length == 1, "parameters.Length == 1"); IgnoreParams = true; } else if (parameters[0].Equals("*")) { Debug.Assert(parameters.Length == 1, "parameters.Length == 1"); _paramsStar = true; } else { _parameters = new HttpDictionary(); for (i = 0, n = parameters.Length; i < n; i++) { _parameters.SetValue(parameters[i], parameters[i]); } } } } internal bool IsModified() { return _isModified; } internal bool AcceptsParams() { return _ignoreParams == 1 || _paramsStar || _parameters != null; } internal String[] GetParams() { String[] s = null; Object item; int i, j, c, n; if (_ignoreParams == 1) { s = new String[1] {String.Empty}; } else if (_paramsStar) { s = new String[1] {"*"}; } else if (_parameters != null) { n = _parameters.Size; c = 0; for (i = 0; i < n; i++) { item = _parameters.GetValue(i); if (item != null) { c++; } } if (c > 0) { s = new string[c]; j = 0; for (i = 0; i < n; i++) { item = _parameters.GetValue(i); if (item != null) { s[j] = (String) item; j++; } } Debug.Assert(j == c, "j == c"); } } return s; } // // Public methods and properties // ///Indicates that a cache should contain multiple /// representations for a particular Uri. This class is an encapsulation that /// provides a rich, type-safe way to set the Vary header. ////// public bool this[String header] { get { if (header == null) { throw new ArgumentNullException("header"); } if (header.Length == 0) { return _ignoreParams == 1; } else { return _paramsStar || (_parameters != null && _parameters.GetValue(header) != null); } } set { if (header == null) { throw new ArgumentNullException("header"); } if (header.Length == 0) { IgnoreParams = value; } /* * Since adding a Vary parameter is more restrictive, we don't * want components to be able to set a Vary parameter to false * if another component has set it to true. */ else if (value) { _isModified = true; _ignoreParams = 0; if (header.Equals("*")) { _paramsStar = true; _parameters = null; } else { // set value to header if true or null if false if (!_paramsStar) { if (_parameters == null) { _parameters = new HttpDictionary(); } _parameters.SetValue(header, header); } } } } } public bool IgnoreParams { get { return _ignoreParams == 1; } set { // Don't ignore if params have been added if (_paramsStar || _parameters != null) { return; } if (_ignoreParams == -1 || _ignoreParams == 1) { _ignoreParams = value ? 1 : 0; _isModified = true; } } } internal bool IsVaryByStar { get { return _paramsStar; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.Default property. /// Indexed property indicating that a cache should (or should not) vary according /// to a custom header. ///
Link Menu
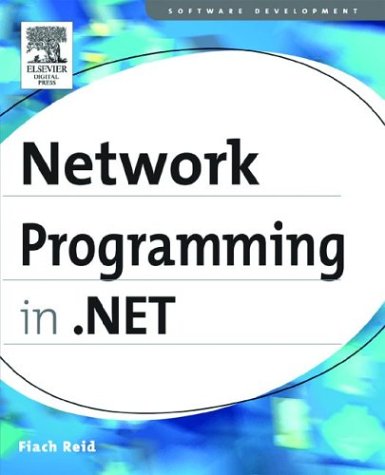
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProxySimple.cs
- TaskHelper.cs
- ObjectQuery_EntitySqlExtensions.cs
- AttachedAnnotation.cs
- XmlArrayAttribute.cs
- SqlTrackingService.cs
- PartManifestEntry.cs
- RuleConditionDialog.cs
- PopupEventArgs.cs
- SimpleBitVector32.cs
- PageVisual.cs
- TransmissionStrategy.cs
- SelectionItemProviderWrapper.cs
- CompilationUnit.cs
- StrokeFIndices.cs
- StoreConnection.cs
- FormsAuthenticationEventArgs.cs
- ObjectListCommandCollection.cs
- OleAutBinder.cs
- UnsafeCollabNativeMethods.cs
- WindowsContainer.cs
- PageTrueTypeFont.cs
- CheckBoxStandardAdapter.cs
- CommandField.cs
- AsymmetricSecurityProtocol.cs
- StatusBarPanel.cs
- NCryptSafeHandles.cs
- Exception.cs
- TagNameToTypeMapper.cs
- ObjectDataSourceView.cs
- EncryptedPackageFilter.cs
- TreeViewImageKeyConverter.cs
- ViewLoader.cs
- MetadataCollection.cs
- Size3D.cs
- CacheEntry.cs
- StopStoryboard.cs
- SchemaInfo.cs
- JsonClassDataContract.cs
- StorageRoot.cs
- WizardStepBase.cs
- streamingZipPartStream.cs
- ScriptResourceAttribute.cs
- TreeBuilderXamlTranslator.cs
- KeyTime.cs
- CodeComment.cs
- BitmapEffect.cs
- PhysicalOps.cs
- CommandLibraryHelper.cs
- OdbcEnvironment.cs
- DataGridGeneralPage.cs
- EntityTypeBase.cs
- SeparatorAutomationPeer.cs
- _Semaphore.cs
- StringBuilder.cs
- Crypto.cs
- FontSource.cs
- EdmComplexPropertyAttribute.cs
- FormView.cs
- ImageBrush.cs
- PropertiesTab.cs
- HtmlListAdapter.cs
- CheckBoxStandardAdapter.cs
- SimpleExpression.cs
- ErrorCodes.cs
- AuthStoreRoleProvider.cs
- ForEachAction.cs
- StrokeSerializer.cs
- EventLogger.cs
- CanonicalFormWriter.cs
- coordinatorfactory.cs
- ListViewDeletedEventArgs.cs
- IpcChannel.cs
- Int32CollectionValueSerializer.cs
- DataGridViewCellContextMenuStripNeededEventArgs.cs
- QuestionEventArgs.cs
- CombinedGeometry.cs
- ElementMarkupObject.cs
- WmlSelectionListAdapter.cs
- DataTableReaderListener.cs
- DbInsertCommandTree.cs
- TemplateControlCodeDomTreeGenerator.cs
- RangeContentEnumerator.cs
- CorrelationHandle.cs
- AggregateException.cs
- SchemaElementDecl.cs
- LongPath.cs
- Transactions.cs
- UserInitiatedNavigationPermission.cs
- AxWrapperGen.cs
- Evaluator.cs
- XmlAtomErrorReader.cs
- DataSourceCache.cs
- Sequence.cs
- BindingOperations.cs
- ScriptReferenceBase.cs
- RuntimeIdentifierPropertyAttribute.cs
- ToolStripLabel.cs
- OleDbConnectionInternal.cs
- SystemIPAddressInformation.cs