Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / infocard / Client / System / IdentityModel / Selectors / CryptoHandle.cs / 1305376 / CryptoHandle.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // // Presharp uses the c# pragma mechanism to supress its warnings. // These are not recognised by the base compiler so we need to explictly // disable the following warnings. See http://winweb/cse/Tools/PREsharp/userguide/default.asp // for details. // #pragma warning disable 1634, 1691 // unknown message, unknown pragma namespace System.IdentityModel.Selectors { using System; using System.Runtime.InteropServices; using System.Threading; using System.Security; using System.Runtime.ConstrainedExecution; using System.Runtime.CompilerServices; using IDT=Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // For common & resources // using Microsoft.InfoCards; // // Summary: // Wraps and manages the lifetime of a native crypto handle passed back from the native InfoCard API. // internal abstract class CryptoHandle : IDisposable { bool m_isDisposed; InternalRefCountedHandle m_internalHandle; // // Summary: // Creates a new CryptoHandle. ParamType has information as to what // nativeParameters has to be marshaled into. // protected CryptoHandle( InternalRefCountedHandle nativeHandle, DateTime expiration, IntPtr nativeParameters, Type paramType ) { m_internalHandle = nativeHandle; m_internalHandle.Initialize( expiration, Marshal.PtrToStructure( nativeParameters, paramType ) ); } // // Summary: // This constructor creates a new CryptoHandle instance with the same InternalRefCountedHandle and adds // a ref count to that InternalRefCountedHandle. // protected CryptoHandle( InternalRefCountedHandle internalHandle ) { m_internalHandle = internalHandle; m_internalHandle.AddRef(); } public InternalRefCountedHandle InternalHandle { get { ThrowIfDisposed(); return m_internalHandle; } } public DateTime Expiration { get { ThrowIfDisposed(); return m_internalHandle.Expiration; } } public object Parameters { get { ThrowIfDisposed(); return m_internalHandle.Parameters; } } // // Summary: // Creates a new CryptoHandle with same InternalRefCountedCryptoHandle. // public CryptoHandle Duplicate() { ThrowIfDisposed(); return OnDuplicate(); } // // Summary: // Allows subclasses to create a duplicate of their particular class. // protected abstract CryptoHandle OnDuplicate(); protected void ThrowIfDisposed() { if ( m_isDisposed ) { throw IDT.ThrowHelperError( new ObjectDisposedException( SR.GetString( SR.ClientCryptoSessionDisposed ) ) ); } } public void Dispose() { if ( m_isDisposed ) { return; } m_internalHandle.Release(); m_internalHandle = null; m_isDisposed = true; } // // Summary: // Given a pointer to a native cryptosession this method creates the appropriate CryptoHandle type. // static internal CryptoHandle Create( InternalRefCountedHandle nativeHandle ) { CryptoHandle handle = null; bool mustRelease = false; RuntimeHelpers.PrepareConstrainedRegions(); try { nativeHandle.DangerousAddRef( ref mustRelease ); RpcInfoCardCryptoHandle hCrypto = (RpcInfoCardCryptoHandle)Marshal.PtrToStructure( nativeHandle.DangerousGetHandle(), typeof( RpcInfoCardCryptoHandle ) ); DateTime expiration = DateTime.FromFileTimeUtc( hCrypto.expiration ); switch ( hCrypto.type ) { case RpcInfoCardCryptoHandle.HandleType.Asymmetric: handle = new AsymmetricCryptoHandle( nativeHandle, expiration, hCrypto.cryptoParameters ); break; case RpcInfoCardCryptoHandle.HandleType.Symmetric: handle = new SymmetricCryptoHandle( nativeHandle, expiration, hCrypto.cryptoParameters ); break; case RpcInfoCardCryptoHandle.HandleType.Transform: handle = new TransformCryptoHandle( nativeHandle, expiration, hCrypto.cryptoParameters ); break; case RpcInfoCardCryptoHandle.HandleType.Hash: handle = new HashCryptoHandle( nativeHandle, expiration, hCrypto.cryptoParameters ); break; default: IDT.DebugAssert( false, "Invalid crypto operation type" ); throw IDT.ThrowHelperError( new InvalidOperationException( SR.GetString( SR.GeneralExceptionMessage ) ) ); } return handle; } finally { if( mustRelease ) { nativeHandle.DangerousRelease(); } } } } // // Summary: // This class manages the lifetime of a native crypto handle through ref counts. Any number of CryptoHandles // may refer to a single InternalRefCountedHandle, but once they are all disposed this object will dispose // itself as well. // internal class InternalRefCountedHandle : SafeHandle { int m_refcount = 0; DateTime m_expiration; object m_parameters = null; [DllImport( "infocardapi.dll", EntryPoint ="CloseCryptoHandle", CharSet = CharSet.Unicode, CallingConvention = CallingConvention.StdCall, ExactSpelling = true, SetLastError = true ) ] [SuppressUnmanagedCodeSecurity] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] private static extern bool CloseCryptoHandle( [In] IntPtr hKey ); private InternalRefCountedHandle() : base( IntPtr.Zero, true ) { m_refcount = 1; } public void Initialize( DateTime expiration, object parameters ) { m_expiration = expiration; m_parameters = parameters; } // // Summary: // The deserialized parameters specific to a particular type of CryptoHandle. // public object Parameters { get { ThrowIfInvalid(); return m_parameters; } } // // Summary: // The expiration of this CryptoHandle // public DateTime Expiration { get { ThrowIfInvalid(); return m_expiration; } } public void AddRef() { ThrowIfInvalid(); Interlocked.Increment( ref m_refcount ); } public void Release() { ThrowIfInvalid(); int refcount = Interlocked.Decrement( ref m_refcount ); if ( 0 == refcount ) { Dispose(); } } private void ThrowIfInvalid() { if ( IsInvalid ) { throw IDT.ThrowHelperError( new ObjectDisposedException( "InternalRefCountedHandle" ) ); } } public override bool IsInvalid { get { return ( IntPtr.Zero == base.handle ); } } protected override bool ReleaseHandle() { #pragma warning suppress 56523 return CloseCryptoHandle( base.handle ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // // Presharp uses the c# pragma mechanism to supress its warnings. // These are not recognised by the base compiler so we need to explictly // disable the following warnings. See http://winweb/cse/Tools/PREsharp/userguide/default.asp // for details. // #pragma warning disable 1634, 1691 // unknown message, unknown pragma namespace System.IdentityModel.Selectors { using System; using System.Runtime.InteropServices; using System.Threading; using System.Security; using System.Runtime.ConstrainedExecution; using System.Runtime.CompilerServices; using IDT=Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // For common & resources // using Microsoft.InfoCards; // // Summary: // Wraps and manages the lifetime of a native crypto handle passed back from the native InfoCard API. // internal abstract class CryptoHandle : IDisposable { bool m_isDisposed; InternalRefCountedHandle m_internalHandle; // // Summary: // Creates a new CryptoHandle. ParamType has information as to what // nativeParameters has to be marshaled into. // protected CryptoHandle( InternalRefCountedHandle nativeHandle, DateTime expiration, IntPtr nativeParameters, Type paramType ) { m_internalHandle = nativeHandle; m_internalHandle.Initialize( expiration, Marshal.PtrToStructure( nativeParameters, paramType ) ); } // // Summary: // This constructor creates a new CryptoHandle instance with the same InternalRefCountedHandle and adds // a ref count to that InternalRefCountedHandle. // protected CryptoHandle( InternalRefCountedHandle internalHandle ) { m_internalHandle = internalHandle; m_internalHandle.AddRef(); } public InternalRefCountedHandle InternalHandle { get { ThrowIfDisposed(); return m_internalHandle; } } public DateTime Expiration { get { ThrowIfDisposed(); return m_internalHandle.Expiration; } } public object Parameters { get { ThrowIfDisposed(); return m_internalHandle.Parameters; } } // // Summary: // Creates a new CryptoHandle with same InternalRefCountedCryptoHandle. // public CryptoHandle Duplicate() { ThrowIfDisposed(); return OnDuplicate(); } // // Summary: // Allows subclasses to create a duplicate of their particular class. // protected abstract CryptoHandle OnDuplicate(); protected void ThrowIfDisposed() { if ( m_isDisposed ) { throw IDT.ThrowHelperError( new ObjectDisposedException( SR.GetString( SR.ClientCryptoSessionDisposed ) ) ); } } public void Dispose() { if ( m_isDisposed ) { return; } m_internalHandle.Release(); m_internalHandle = null; m_isDisposed = true; } // // Summary: // Given a pointer to a native cryptosession this method creates the appropriate CryptoHandle type. // static internal CryptoHandle Create( InternalRefCountedHandle nativeHandle ) { CryptoHandle handle = null; bool mustRelease = false; RuntimeHelpers.PrepareConstrainedRegions(); try { nativeHandle.DangerousAddRef( ref mustRelease ); RpcInfoCardCryptoHandle hCrypto = (RpcInfoCardCryptoHandle)Marshal.PtrToStructure( nativeHandle.DangerousGetHandle(), typeof( RpcInfoCardCryptoHandle ) ); DateTime expiration = DateTime.FromFileTimeUtc( hCrypto.expiration ); switch ( hCrypto.type ) { case RpcInfoCardCryptoHandle.HandleType.Asymmetric: handle = new AsymmetricCryptoHandle( nativeHandle, expiration, hCrypto.cryptoParameters ); break; case RpcInfoCardCryptoHandle.HandleType.Symmetric: handle = new SymmetricCryptoHandle( nativeHandle, expiration, hCrypto.cryptoParameters ); break; case RpcInfoCardCryptoHandle.HandleType.Transform: handle = new TransformCryptoHandle( nativeHandle, expiration, hCrypto.cryptoParameters ); break; case RpcInfoCardCryptoHandle.HandleType.Hash: handle = new HashCryptoHandle( nativeHandle, expiration, hCrypto.cryptoParameters ); break; default: IDT.DebugAssert( false, "Invalid crypto operation type" ); throw IDT.ThrowHelperError( new InvalidOperationException( SR.GetString( SR.GeneralExceptionMessage ) ) ); } return handle; } finally { if( mustRelease ) { nativeHandle.DangerousRelease(); } } } } // // Summary: // This class manages the lifetime of a native crypto handle through ref counts. Any number of CryptoHandles // may refer to a single InternalRefCountedHandle, but once they are all disposed this object will dispose // itself as well. // internal class InternalRefCountedHandle : SafeHandle { int m_refcount = 0; DateTime m_expiration; object m_parameters = null; [DllImport( "infocardapi.dll", EntryPoint ="CloseCryptoHandle", CharSet = CharSet.Unicode, CallingConvention = CallingConvention.StdCall, ExactSpelling = true, SetLastError = true ) ] [SuppressUnmanagedCodeSecurity] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] private static extern bool CloseCryptoHandle( [In] IntPtr hKey ); private InternalRefCountedHandle() : base( IntPtr.Zero, true ) { m_refcount = 1; } public void Initialize( DateTime expiration, object parameters ) { m_expiration = expiration; m_parameters = parameters; } // // Summary: // The deserialized parameters specific to a particular type of CryptoHandle. // public object Parameters { get { ThrowIfInvalid(); return m_parameters; } } // // Summary: // The expiration of this CryptoHandle // public DateTime Expiration { get { ThrowIfInvalid(); return m_expiration; } } public void AddRef() { ThrowIfInvalid(); Interlocked.Increment( ref m_refcount ); } public void Release() { ThrowIfInvalid(); int refcount = Interlocked.Decrement( ref m_refcount ); if ( 0 == refcount ) { Dispose(); } } private void ThrowIfInvalid() { if ( IsInvalid ) { throw IDT.ThrowHelperError( new ObjectDisposedException( "InternalRefCountedHandle" ) ); } } public override bool IsInvalid { get { return ( IntPtr.Zero == base.handle ); } } protected override bool ReleaseHandle() { #pragma warning suppress 56523 return CloseCryptoHandle( base.handle ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
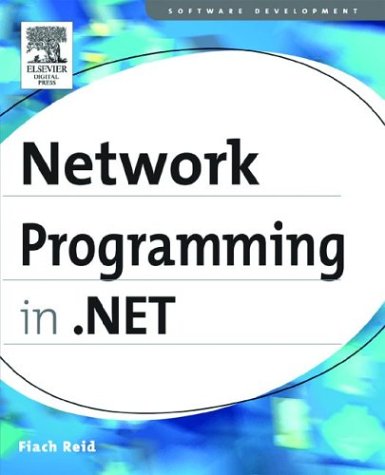
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PeerNameRecordCollection.cs
- NativeMethods.cs
- ParameterBuilder.cs
- WindowsBrush.cs
- StructuralType.cs
- XmlReflectionMember.cs
- ShapingWorkspace.cs
- UdpConstants.cs
- BitmapDownload.cs
- OleDbInfoMessageEvent.cs
- HuffModule.cs
- RijndaelManaged.cs
- DefaultPropertiesToSend.cs
- TouchFrameEventArgs.cs
- CodeCatchClause.cs
- XmlLoader.cs
- Animatable.cs
- XmlSerializableServices.cs
- XmlSchemaSubstitutionGroup.cs
- ParameterReplacerVisitor.cs
- NullableLongSumAggregationOperator.cs
- BamlLocalizabilityResolver.cs
- TransactionFlowProperty.cs
- sqlpipe.cs
- NativeMethods.cs
- LocalizabilityAttribute.cs
- CqlBlock.cs
- ZipIOExtraFieldPaddingElement.cs
- FormatSelectingMessageInspector.cs
- EventlogProvider.cs
- TextDecorationCollectionConverter.cs
- TTSEngineTypes.cs
- DataContractSerializer.cs
- NumericExpr.cs
- ConsumerConnectionPoint.cs
- EntityProxyTypeInfo.cs
- OdbcConnectionFactory.cs
- ImageListStreamer.cs
- GeometryDrawing.cs
- SecureEnvironment.cs
- SelectionEditingBehavior.cs
- PositiveTimeSpanValidatorAttribute.cs
- ImageUrlEditor.cs
- SequentialWorkflowRootDesigner.cs
- BitmapEffectState.cs
- exports.cs
- SwitchElementsCollection.cs
- IndependentlyAnimatedPropertyMetadata.cs
- TileBrush.cs
- SqlDataSourceSelectingEventArgs.cs
- MDIClient.cs
- SQLInt16.cs
- XmlAttributes.cs
- TableItemPatternIdentifiers.cs
- Brushes.cs
- UDPClient.cs
- RadioButtonRenderer.cs
- XsltFunctions.cs
- DbProviderFactoriesConfigurationHandler.cs
- TextTreeUndo.cs
- BaseCollection.cs
- Registry.cs
- XmlObjectSerializerReadContextComplex.cs
- BroadcastEventHelper.cs
- TreeNodeBindingDepthConverter.cs
- EndpointAddressMessageFilterTable.cs
- WebPartVerbCollection.cs
- ColorConvertedBitmapExtension.cs
- MailAddress.cs
- ServiceNameElement.cs
- AssemblyCollection.cs
- TransformGroup.cs
- WindowsRebar.cs
- ResolveNextArgumentWorkItem.cs
- DataGridViewCellConverter.cs
- AutomationPatternInfo.cs
- TypedReference.cs
- X509Extension.cs
- StateMachineAction.cs
- RequiredFieldValidator.cs
- CodeRemoveEventStatement.cs
- HtmlMeta.cs
- SoapElementAttribute.cs
- ReadOnlyHierarchicalDataSourceView.cs
- SmiEventSink_DeferedProcessing.cs
- CompilerCollection.cs
- DataGridAutoGeneratingColumnEventArgs.cs
- LabelTarget.cs
- AccessViolationException.cs
- TableRow.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- _TransmitFileOverlappedAsyncResult.cs
- XmlQueryRuntime.cs
- ReaderOutput.cs
- ReflectionHelper.cs
- AuthenticatedStream.cs
- ThreadPool.cs
- NullRuntimeConfig.cs
- StorageConditionPropertyMapping.cs
- Rule.cs