Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / TransactionFlowProperty.cs / 1 / TransactionFlowProperty.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Channels { using System; using System.Collections.Generic; using System.ServiceModel; using System.ServiceModel.Security; using System.Transactions; using System.Runtime.Remoting.Messaging; using System.ServiceModel.Transactions; using System.ServiceModel.Diagnostics; sealed public class TransactionMessageProperty { TransactionInfo flowedTransactionInfo; Transaction flowedTransaction; const string PropertyName = "TransactionMessageProperty"; private TransactionMessageProperty() { } public Transaction Transaction { get { if(this.flowedTransaction == null && this.flowedTransactionInfo != null) { try { this.flowedTransaction = this.flowedTransactionInfo.UnmarshalTransaction(); } catch(TransactionException e) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(e); } } return this.flowedTransaction; } } static internal TransactionMessageProperty TryGet(Message message) { if (message.Properties.ContainsKey(PropertyName)) return message.Properties[PropertyName] as TransactionMessageProperty; else return null; } static internal Transaction TryGetTransaction(Message message) { if (!message.Properties.ContainsKey(PropertyName)) return null; return ((TransactionMessageProperty)message.Properties[PropertyName]).Transaction; } static TransactionMessageProperty GetPropertyAndThrowIfAlreadySet(Message message) { if (message.Properties.ContainsKey(PropertyName)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new FaultException(SR.GetString(SR.SFxTryAddMultipleTransactionsOnMessage))); } return new TransactionMessageProperty(); } static public void Set(Transaction transaction, Message message) { TransactionMessageProperty property = GetPropertyAndThrowIfAlreadySet(message); property.flowedTransaction = transaction; message.Properties.Add(PropertyName, property); } static internal void Set(TransactionInfo transactionInfo, Message message) { TransactionMessageProperty property = GetPropertyAndThrowIfAlreadySet(message); property.flowedTransactionInfo = transactionInfo; message.Properties.Add(PropertyName, property); } } class TransactionFlowProperty { Transaction flowedTransaction; ListissuedTokens; const string PropertyName = "TransactionFlowProperty"; private TransactionFlowProperty() { } internal ICollection IssuedTokens { get { if (this.issuedTokens == null) { this.issuedTokens = new List (); } return this.issuedTokens; } } internal Transaction Transaction { get {return this.flowedTransaction;} } static internal TransactionFlowProperty Ensure(Message message) { if (message.Properties.ContainsKey(PropertyName)) return (TransactionFlowProperty)message.Properties[PropertyName]; TransactionFlowProperty property = new TransactionFlowProperty(); message.Properties.Add(PropertyName, property); return property; } static internal TransactionFlowProperty TryGet(Message message) { if (message.Properties.ContainsKey(PropertyName)) return message.Properties[PropertyName] as TransactionFlowProperty; else return null; } static internal ICollection TryGetIssuedTokens(Message message) { TransactionFlowProperty property = TransactionFlowProperty.TryGet(message); if (property == null) return null; // use this when reading only, consistently return null if no tokens. if (property.issuedTokens == null || property.issuedTokens.Count == 0) return null; return property.issuedTokens; } static internal Transaction TryGetTransaction(Message message) { if (!message.Properties.ContainsKey(PropertyName)) return null; return ((TransactionFlowProperty)message.Properties[PropertyName]).Transaction; } static TransactionFlowProperty GetPropertyAndThrowIfAlreadySet(Message message) { TransactionFlowProperty property = TryGet(message); if (property != null) { if(property.flowedTransaction != null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new FaultException(SR.GetString(SR.SFxTryAddMultipleTransactionsOnMessage))); } } else { property = new TransactionFlowProperty(); } return property; } static internal void Set(Transaction transaction, Message message) { TransactionFlowProperty property = GetPropertyAndThrowIfAlreadySet(message); property.flowedTransaction = transaction; message.Properties.Add(PropertyName, property); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
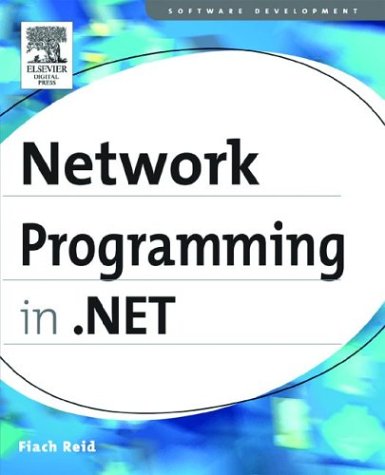
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UriTemplatePathSegment.cs
- PhysicalAddress.cs
- SmtpFailedRecipientException.cs
- CultureInfo.cs
- ApplyTemplatesAction.cs
- JumpPath.cs
- SqlDelegatedTransaction.cs
- UserControl.cs
- EntityDataSourceDesignerHelper.cs
- DurationConverter.cs
- CryptoApi.cs
- CacheHelper.cs
- HMAC.cs
- TypeGenericEnumerableViewSchema.cs
- ListItemParagraph.cs
- MenuItemAutomationPeer.cs
- __Filters.cs
- SeekStoryboard.cs
- ClosableStream.cs
- Literal.cs
- DropShadowEffect.cs
- AttributeCollection.cs
- RawAppCommandInputReport.cs
- DbParameterHelper.cs
- DataObjectPastingEventArgs.cs
- ProxyElement.cs
- SoapRpcMethodAttribute.cs
- XmlValidatingReaderImpl.cs
- StatusBar.cs
- XmlILModule.cs
- StreamGeometry.cs
- HwndKeyboardInputProvider.cs
- DispatcherSynchronizationContext.cs
- EditorPart.cs
- EditorPartChrome.cs
- RenderTargetBitmap.cs
- SystemKeyConverter.cs
- NativeObjectSecurity.cs
- PageThemeCodeDomTreeGenerator.cs
- PropertyOverridesTypeEditor.cs
- BinaryWriter.cs
- DataServiceRequestException.cs
- EncoderExceptionFallback.cs
- MimeWriter.cs
- SmiTypedGetterSetter.cs
- LifetimeServices.cs
- XmlWellformedWriterHelpers.cs
- SqlDataAdapter.cs
- ServiceModelConfigurationElementCollection.cs
- FieldBuilder.cs
- DbDataRecord.cs
- SendKeys.cs
- DoubleAnimationUsingKeyFrames.cs
- InstalledFontCollection.cs
- ContentPosition.cs
- Sequence.cs
- Function.cs
- AbstractDataSvcMapFileLoader.cs
- TextRangeAdaptor.cs
- InvokeAction.cs
- StackSpiller.Generated.cs
- DataTableNewRowEvent.cs
- ExpressionBuilder.cs
- TextTreeTextBlock.cs
- SessionStateContainer.cs
- ByteStream.cs
- FieldAccessException.cs
- SmtpException.cs
- CompletionProxy.cs
- GridPatternIdentifiers.cs
- ChtmlTextWriter.cs
- DocumentPageView.cs
- MenuRendererClassic.cs
- PasswordTextContainer.cs
- CriticalFinalizerObject.cs
- CompoundFileStreamReference.cs
- WindowHideOrCloseTracker.cs
- MethodBody.cs
- HttpApplicationFactory.cs
- PersonalizationDictionary.cs
- SoapIgnoreAttribute.cs
- UdpDuplexChannel.cs
- MultiAsyncResult.cs
- ConsoleTraceListener.cs
- Accessible.cs
- AllMembershipCondition.cs
- FixedPosition.cs
- HtmlWindowCollection.cs
- PropertiesTab.cs
- ScriptMethodAttribute.cs
- TraceData.cs
- XmlCharType.cs
- ElementInit.cs
- ErasingStroke.cs
- WorkflowOwnerAsyncResult.cs
- ObservableCollection.cs
- XmlQualifiedNameTest.cs
- InheritanceContextChangedEventManager.cs
- DeflateStream.cs
- VariableAction.cs