Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Extensions / Compilation / WCFModel / AbstractDataSvcMapFileLoader.cs / 1305376 / AbstractDataSvcMapFileLoader.cs
//------------------------------------------------------------------------------ //// Copyright (C) Microsoft Corporation. All Rights Reserved. // //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Globalization; using System.IO; using System.Text; using System.Xml; using System.Xml.Schema; #if WEB_EXTENSIONS_CODE using System.Web.Resources; #else using Microsoft.VSDesigner.Resources.Microsoft.VSDesigner; #endif #if WEB_EXTENSIONS_CODE namespace System.Web.Compilation.WCFModel #else namespace Microsoft.VSDesigner.WCFModel #endif { ////// Helper class that wraps the creation of the appropriate XML serializer /// for the svcmap class. /// /// The SvcMapFileLoader is responsible for serializing/deserializing all the referenced /// metadata and extension files. /// ///#if WEB_EXTENSIONS_CODE internal abstract class AbstractDataSvcMapFileLoader #else [CLSCompliant(true)] public abstract class AbstractDataSvcMapFileLoader #endif { /// /// Cached XML serializer for SvcMapFile objects /// protected internal System.Xml.Serialization.XmlSerializer serializer; ////// Schema for the svcmap file /// private static XmlSchemaSet m_ServiceMapSchemaSet; ////// Load map file /// /// /// The name of the file that we are currently loading. This value is only used for /// error reporting. /// ///Deserialized DataSvcMapFile ///public virtual DataSvcMapFile LoadMapFile(string fileName) { if (fileName == null) throw new ArgumentNullException("fileName"); using (System.IO.TextReader mapFileReader = this.GetMapFileReader()) { List proxyGenerationErrors = new List (); XmlReaderSettings readerSettings = new XmlReaderSettings(); readerSettings.Schemas = ServiceMapSchemaSet; readerSettings.ValidationType = ValidationType.Schema; readerSettings.ValidationFlags = XmlSchemaValidationFlags.ReportValidationWarnings; ValidationEventHandler handler = delegate(object sender, System.Xml.Schema.ValidationEventArgs e) { bool isError = (e.Severity == XmlSeverityType.Error); proxyGenerationErrors.Add(new ProxyGenerationError(ProxyGenerationError.GeneratorState.LoadMetadata, fileName, e.Exception, !isError)); if (isError) { throw e.Exception; } }; readerSettings.ValidationEventHandler += handler; DataSvcMapFile svcMapFile = null; try { XmlReader xReader = XmlReader.Create(mapFileReader, readerSettings, (string)null); try { svcMapFile = (DataSvcMapFile)Serializer.Deserialize(xReader); } catch (System.InvalidOperationException ex) { System.Xml.XmlException xmlException = ex.InnerException as System.Xml.XmlException; if (xmlException != null) { // the innerException contains detail error message throw xmlException; } System.Xml.Schema.XmlSchemaException schemaException = ex.InnerException as System.Xml.Schema.XmlSchemaException; if (schemaException != null) { if (schemaException.LineNumber > 0) { // append line/position to the message throw new System.Xml.Schema.XmlSchemaException( String.Format(CultureInfo.CurrentCulture, WCFModelStrings.ReferenceGroup_AppendLinePosition, schemaException.Message, schemaException.LineNumber, schemaException.LinePosition), schemaException, schemaException.LineNumber, schemaException.LinePosition); } throw schemaException; } throw; } // basic check to prevent loading an invalid svcmap file ValidateSvcMapFile(svcMapFile); foreach (MetadataFile metadataFile in svcMapFile.MetadataList) { metadataFile.IsExistingFile = true; LoadMetadataFile(metadataFile); } foreach (ExtensionFile extensionFile in svcMapFile.Extensions) { extensionFile.IsExistingFile = true; LoadExtensionFile(extensionFile); } } finally { readerSettings.ValidationEventHandler -= handler; } if (svcMapFile != null) { svcMapFile.SetLoadErrors(proxyGenerationErrors); } return svcMapFile; } } /// /// Load metadata file from file system /// /// ///public virtual void LoadMetadataFile(MetadataFile metadataFile) { try { // clean up metadataFile.CleanUpContent(); metadataFile.LoadContent(this.ReadMetadataFile(metadataFile.FileName)); } catch (Exception ex) { metadataFile.ErrorInLoading = ex; } } /// /// Load extension file /// /// ///public virtual void LoadExtensionFile(ExtensionFile extensionFile) { try { // clean up extensionFile.CleanUpContent(); // load extensionFile.ContentBuffer = ReadExtensionFile(extensionFile.FileName); } catch (System.Exception ex) { extensionFile.ErrorInLoading = ex; } } /// /// Get access to a text reader that gets access to the svcmap byte stream /// ///protected abstract System.IO.TextReader GetMapFileReader(); /// /// Get access to a byte array that contain the contents of the given extension /// file /// /// /// Name of the extension file. Could be a path relative to the svcmap file location /// or the name of an item in a metadata storage. /// ///protected abstract byte[] ReadExtensionFile(string name); /// /// Get access to a byte array that contain the contents of the given metadata /// file /// /// /// Name of the metadata file. Could be a path relative to the svcmap file location /// or the name of an item in a metadata storage. /// ///protected abstract byte[] ReadMetadataFile(string name); /// /// Do basic check after the svcmap file is loaded /// /// ////// private void ValidateSvcMapFile(DataSvcMapFile svcMapFile) { // check duplicated file names Dictionary externalFileList = new Dictionary (StringComparer.OrdinalIgnoreCase); foreach (MetadataFile metadataFile in svcMapFile.MetadataList) { if (metadataFile.FileName != null) { if (externalFileList.ContainsKey(metadataFile.FileName)) { throw new FormatException(String.Format(CultureInfo.CurrentCulture, WCFModelStrings.ReferenceGroup_TwoExternalFilesWithSameName, metadataFile.FileName)); } else { externalFileList.Add(metadataFile.FileName, metadataFile); } } } foreach (ExtensionFile extensionFile in svcMapFile.Extensions) { if (extensionFile.FileName != null) { if (externalFileList.ContainsKey(extensionFile.FileName)) { throw new FormatException(String.Format(CultureInfo.CurrentCulture, WCFModelStrings.ReferenceGroup_TwoExternalFilesWithSameName, extensionFile.FileName)); } else { externalFileList.Add(extensionFile.FileName, extensionFile); } } } } /// /// Demand-create an XmlSerializer for the SvcMap file... /// protected virtual System.Xml.Serialization.XmlSerializer Serializer { get { if (serializer == null) { serializer = new System.Xml.Serialization.XmlSerializer(typeof(DataSvcMapFile), SvcMapFile.NamespaceUri); } return serializer; } } ////////// event handler to collect validation error messages ///// ///// ///// ///////private void OnValidationError(object sender, System.Xml.Schema.ValidationEventArgs e) //{ // bool isError = (e.Severity == XmlSeverityType.Error); // if (m_ProxyGenerationErrors != null) // { // m_ProxyGenerationErrors.Add(new ProxyGenerationError(ProxyGenerationError.GeneratorState.LoadMetadata, fileName, e.Exception, !isError)); // } // if (isError) // { // throw e.Exception; // } //} /// /// The schema of the service map file /// ////// private static XmlSchemaSet ServiceMapSchemaSet { get { if (m_ServiceMapSchemaSet == null) { XmlSchema serviceMapSchema; System.IO.Stream fileStream = typeof(AbstractDataSvcMapFileLoader).Assembly.GetManifestResourceStream(typeof(AbstractDataSvcMapFileLoader), @"Schema.DataServiceMapSchema.xsd"); System.Diagnostics.Debug.Assert(fileStream != null, "Couldn't find ServiceMapSchema.xsd resource"); serviceMapSchema = XmlSchema.Read(fileStream, null); m_ServiceMapSchemaSet = new XmlSchemaSet(); m_ServiceMapSchemaSet.Add(serviceMapSchema); } return m_ServiceMapSchemaSet; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
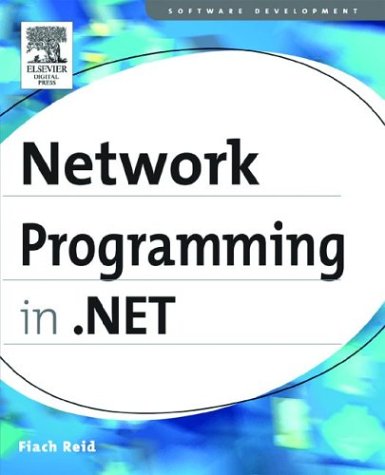
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PrinterResolution.cs
- RsaKeyIdentifierClause.cs
- DataGridViewSelectedCellCollection.cs
- RSAPKCS1KeyExchangeFormatter.cs
- ThaiBuddhistCalendar.cs
- FloaterParaClient.cs
- Claim.cs
- AppSettingsReader.cs
- FullTrustAssemblyCollection.cs
- SystemParameters.cs
- AssemblyUtil.cs
- log.cs
- WebExceptionStatus.cs
- DbExpressionVisitor_TResultType.cs
- InvalidContentTypeException.cs
- CodeCatchClause.cs
- OperationAbortedException.cs
- ListenerConfig.cs
- OleDbConnection.cs
- ThreadAbortException.cs
- DropShadowBitmapEffect.cs
- Int32RectValueSerializer.cs
- SubpageParagraph.cs
- MultipartContentParser.cs
- PropagatorResult.cs
- XPathNavigator.cs
- FormatSettings.cs
- Constants.cs
- ExportOptions.cs
- Panel.cs
- ActiveDesignSurfaceEvent.cs
- WindowManager.cs
- PenThreadPool.cs
- LocalizationComments.cs
- AssemblyAssociatedContentFileAttribute.cs
- GZipDecoder.cs
- ManipulationBoundaryFeedbackEventArgs.cs
- DateTime.cs
- ServiceDiscoveryBehavior.cs
- HttpListenerException.cs
- OleDbPermission.cs
- CustomAttributeFormatException.cs
- DataGridViewColumn.cs
- MenuItemStyleCollection.cs
- ServiceDefaults.cs
- CodeGenHelper.cs
- MessageFault.cs
- UserControlCodeDomTreeGenerator.cs
- BuiltInExpr.cs
- FontNameEditor.cs
- WMIInterop.cs
- EventBookmark.cs
- _RequestCacheProtocol.cs
- IdentityElement.cs
- DerivedKeyCachingSecurityTokenSerializer.cs
- DataGridViewCellCancelEventArgs.cs
- BreadCrumbTextConverter.cs
- AnimationClockResource.cs
- UserControlBuildProvider.cs
- KeyToListMap.cs
- AuthorizationPolicyTypeElementCollection.cs
- WebPartPersonalization.cs
- Vector3D.cs
- XmlCustomFormatter.cs
- SqlBooleanizer.cs
- AdRotator.cs
- PropertyEmitter.cs
- FieldInfo.cs
- XsdDateTime.cs
- ClientSettingsProvider.cs
- InputLanguageSource.cs
- ByteStorage.cs
- Html32TextWriter.cs
- Viewport3DAutomationPeer.cs
- CodeSpit.cs
- DateTimeParse.cs
- PersonalizationStateInfoCollection.cs
- WSSecurityPolicy11.cs
- DesignerEditorPartChrome.cs
- ProjectedSlot.cs
- SerialStream.cs
- ImageAnimator.cs
- LoginDesigner.cs
- EventMappingSettingsCollection.cs
- AcceleratedTokenAuthenticator.cs
- DataBindingHandlerAttribute.cs
- CacheOutputQuery.cs
- HtmlHead.cs
- MyContact.cs
- ProjectionCamera.cs
- ArrayWithOffset.cs
- DataBinding.cs
- MessageSecurityOverMsmqElement.cs
- OdbcStatementHandle.cs
- dbenumerator.cs
- Column.cs
- Line.cs
- SoapReflectionImporter.cs
- DesignerForm.cs
- IisTraceListener.cs