Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Base / MS / Internal / IO / Packaging / CompoundFile / CompoundFileStreamReference.cs / 1 / CompoundFileStreamReference.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Definition of the CompoundFileStreamReference class. // // History: // 03/27/2002: BruceMac: Initial implementation. // 05/20/2003: RogerCh: Ported to WCP tree. // 08/11/2003: LGolding: Fix Bug 864168 (some of BruceMac's bug fixes were lost // in port to WCP tree). // //----------------------------------------------------------------------------- using System; using System.IO; using System.Text; // for StringBuilder using System.Windows; namespace MS.Internal.IO.Packaging.CompoundFile { ////// Logical reference to a container stream /// ////// Use this class to represent a logical reference to a container stream, /// internal class CompoundFileStreamReference : CompoundFileReference, IComparable { //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- ////// Full path from the root to this stream /// public override string FullName { get { return _fullName; } } //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Constructors ////// Use when you know the full path /// /// whack-delimited name including at least the stream name and optionally including preceding storage names public CompoundFileStreamReference(string fullName) { SetFullName(fullName); } ////// Full featured constructor /// /// optional string describing the storage name - may be null if stream exists in the root storage /// stream name ///streamName cannot be null or empty public CompoundFileStreamReference(string storageName, string streamName) { ContainerUtilities.CheckStringAgainstNullAndEmpty( streamName, "streamName" ); if ((storageName == null) || (storageName.Length == 0)) _fullName = streamName; else { // preallocate space for the stream we are building StringBuilder sb = new StringBuilder(storageName, storageName.Length + 1 + streamName.Length); sb.Append(ContainerUtilities.PathSeparator); sb.Append(streamName); _fullName = sb.ToString(); } } #endregion #region Operators ///Compare for equality /// the CompoundFileReference to compare to public override bool Equals(object o) { if (o == null) return false; // Standard behavior. // support subclassing - our subclasses can call us and do any additive work themselves if (o.GetType() != this.GetType()) return false; CompoundFileStreamReference r = (CompoundFileStreamReference)o; return (String.CompareOrdinal(_fullName.ToUpperInvariant(), r._fullName.ToUpperInvariant()) == 0); } ///Returns an integer suitable for including this object in a hash table public override int GetHashCode() { return _fullName.GetHashCode(); } #endregion #region IComparable ////// Compares two CompoundFileReferences /// /// CompoundFileReference to compare to this one ///Supports the IComparable interface ///Less than zero if this instance is less than the given reference, zero if they are equal /// and greater than zero if this instance is greater than the given reference. Not case sensitive. int IComparable.CompareTo(object o) { if (o == null) return 1; // Standard behavior. // different type? if (o.GetType() != GetType()) throw new ArgumentException( SR.Get(SRID.CanNotCompareDiffTypes)); CompoundFileStreamReference r = (CompoundFileStreamReference)o; return String.CompareOrdinal(_fullName.ToUpperInvariant(), r._fullName.ToUpperInvariant()); } #endregion //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ ////// Initialize _fullName /// ///this should only be called from constructors as references are immutable /// string to parse ///if leading or trailing path delimiter private void SetFullName(string fullName) { ContainerUtilities.CheckStringAgainstNullAndEmpty(fullName, "fullName"); // fail on leading path separator to match functionality across the board // Although we need to do ToUpperInvariant before we do string comparison, in this case // it is not necessary since PathSeparatorAsString is a path symbol if (fullName.StartsWith(ContainerUtilities.PathSeparatorAsString, StringComparison.Ordinal)) throw new ArgumentException( SR.Get(SRID.DelimiterLeading), "fullName"); _fullName = fullName; string[] strings = ContainerUtilities.ConvertBackSlashPathToStringArrayPath(fullName); if (strings.Length == 0) throw new ArgumentException( SR.Get(SRID.CompoundFilePathNullEmpty), "fullName"); } //----------------------------------------------------- // // Private members // //------------------------------------------------------ // this can never be null - use String.Empty private String _fullName; // whack-path } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Definition of the CompoundFileStreamReference class. // // History: // 03/27/2002: BruceMac: Initial implementation. // 05/20/2003: RogerCh: Ported to WCP tree. // 08/11/2003: LGolding: Fix Bug 864168 (some of BruceMac's bug fixes were lost // in port to WCP tree). // //----------------------------------------------------------------------------- using System; using System.IO; using System.Text; // for StringBuilder using System.Windows; namespace MS.Internal.IO.Packaging.CompoundFile { ////// Logical reference to a container stream /// ////// Use this class to represent a logical reference to a container stream, /// internal class CompoundFileStreamReference : CompoundFileReference, IComparable { //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- ////// Full path from the root to this stream /// public override string FullName { get { return _fullName; } } //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Constructors ////// Use when you know the full path /// /// whack-delimited name including at least the stream name and optionally including preceding storage names public CompoundFileStreamReference(string fullName) { SetFullName(fullName); } ////// Full featured constructor /// /// optional string describing the storage name - may be null if stream exists in the root storage /// stream name ///streamName cannot be null or empty public CompoundFileStreamReference(string storageName, string streamName) { ContainerUtilities.CheckStringAgainstNullAndEmpty( streamName, "streamName" ); if ((storageName == null) || (storageName.Length == 0)) _fullName = streamName; else { // preallocate space for the stream we are building StringBuilder sb = new StringBuilder(storageName, storageName.Length + 1 + streamName.Length); sb.Append(ContainerUtilities.PathSeparator); sb.Append(streamName); _fullName = sb.ToString(); } } #endregion #region Operators ///Compare for equality /// the CompoundFileReference to compare to public override bool Equals(object o) { if (o == null) return false; // Standard behavior. // support subclassing - our subclasses can call us and do any additive work themselves if (o.GetType() != this.GetType()) return false; CompoundFileStreamReference r = (CompoundFileStreamReference)o; return (String.CompareOrdinal(_fullName.ToUpperInvariant(), r._fullName.ToUpperInvariant()) == 0); } ///Returns an integer suitable for including this object in a hash table public override int GetHashCode() { return _fullName.GetHashCode(); } #endregion #region IComparable ////// Compares two CompoundFileReferences /// /// CompoundFileReference to compare to this one ///Supports the IComparable interface ///Less than zero if this instance is less than the given reference, zero if they are equal /// and greater than zero if this instance is greater than the given reference. Not case sensitive. int IComparable.CompareTo(object o) { if (o == null) return 1; // Standard behavior. // different type? if (o.GetType() != GetType()) throw new ArgumentException( SR.Get(SRID.CanNotCompareDiffTypes)); CompoundFileStreamReference r = (CompoundFileStreamReference)o; return String.CompareOrdinal(_fullName.ToUpperInvariant(), r._fullName.ToUpperInvariant()); } #endregion //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ ////// Initialize _fullName /// ///this should only be called from constructors as references are immutable /// string to parse ///if leading or trailing path delimiter private void SetFullName(string fullName) { ContainerUtilities.CheckStringAgainstNullAndEmpty(fullName, "fullName"); // fail on leading path separator to match functionality across the board // Although we need to do ToUpperInvariant before we do string comparison, in this case // it is not necessary since PathSeparatorAsString is a path symbol if (fullName.StartsWith(ContainerUtilities.PathSeparatorAsString, StringComparison.Ordinal)) throw new ArgumentException( SR.Get(SRID.DelimiterLeading), "fullName"); _fullName = fullName; string[] strings = ContainerUtilities.ConvertBackSlashPathToStringArrayPath(fullName); if (strings.Length == 0) throw new ArgumentException( SR.Get(SRID.CompoundFilePathNullEmpty), "fullName"); } //----------------------------------------------------- // // Private members // //------------------------------------------------------ // this can never be null - use String.Empty private String _fullName; // whack-path } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
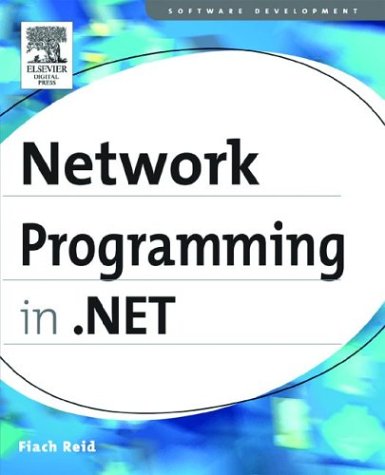
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridTemplateColumn.cs
- Grid.cs
- DesignSurfaceManager.cs
- EraserBehavior.cs
- DataGridViewColumnDividerDoubleClickEventArgs.cs
- DatePicker.cs
- GeneralTransform3D.cs
- InternalResources.cs
- IsolatedStorageFileStream.cs
- ByteFacetDescriptionElement.cs
- PersonalizationStateQuery.cs
- WindowsGraphicsWrapper.cs
- XmlSchemaChoice.cs
- SiteMapProvider.cs
- DataException.cs
- TickBar.cs
- __ComObject.cs
- StorageFunctionMapping.cs
- GcHandle.cs
- CommonDialog.cs
- PromptStyle.cs
- BamlReader.cs
- OutOfProcStateClientManager.cs
- Help.cs
- XmlQualifiedNameTest.cs
- EpmTargetTree.cs
- SqlGenericUtil.cs
- LabelLiteral.cs
- SpecialNameAttribute.cs
- Crc32Helper.cs
- FacetDescriptionElement.cs
- DataGridViewAutoSizeModeEventArgs.cs
- Bitmap.cs
- PriorityRange.cs
- TypeDescriptionProviderAttribute.cs
- Encoding.cs
- IpcPort.cs
- OleCmdHelper.cs
- SystemKeyConverter.cs
- ImpersonateTokenRef.cs
- SpellerStatusTable.cs
- ServicePointManagerElement.cs
- TreeViewEvent.cs
- ToolStripLocationCancelEventArgs.cs
- NameHandler.cs
- WebHttpSecurityModeHelper.cs
- CodeGroup.cs
- SystemIdentity.cs
- OdbcUtils.cs
- MethodRental.cs
- ValueTable.cs
- COM2PropertyBuilderUITypeEditor.cs
- IndentedTextWriter.cs
- DataSourceHelper.cs
- BinaryUtilClasses.cs
- ViewStateException.cs
- InstanceKeyNotReadyException.cs
- ViewManager.cs
- TextPattern.cs
- TypedMessageConverter.cs
- BitmapEffect.cs
- TextProperties.cs
- ToolStripDropDownClosedEventArgs.cs
- SerializationStore.cs
- UnionCodeGroup.cs
- VScrollBar.cs
- RootBrowserWindow.cs
- OrthographicCamera.cs
- EventEntry.cs
- LayoutTable.cs
- _SslSessionsCache.cs
- FlowchartSizeFeature.cs
- CompositeDuplexBindingElementImporter.cs
- SemaphoreFullException.cs
- WmlCalendarAdapter.cs
- BindableTemplateBuilder.cs
- PortCache.cs
- DrawingAttributes.cs
- MembershipPasswordException.cs
- querybuilder.cs
- WebSysDescriptionAttribute.cs
- PropertyDescriptorCollection.cs
- TranslateTransform3D.cs
- AssemblyFilter.cs
- ControlBuilderAttribute.cs
- SrgsRulesCollection.cs
- Line.cs
- DocumentAutomationPeer.cs
- ServiceOperationInvoker.cs
- Selection.cs
- LocationFactory.cs
- HostExecutionContextManager.cs
- LazyTextWriterCreator.cs
- DrawingGroup.cs
- WizardStepCollectionEditor.cs
- PrimitiveType.cs
- CodePageEncoding.cs
- Splitter.cs
- OleDbError.cs
- ApplicationActivator.cs