Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / DurationConverter.cs / 1 / DurationConverter.cs
//------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2004 // // File: DurationConverter.cs //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Security; namespace System.Windows { ////// Provides a type converter to convert Duration to and from other representations. /// public class DurationConverter : TypeConverter { ////// CanConvertFrom - Returns whether or not this class can convert from a given type /// ///public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } /// /// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible ///public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if ( destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } else { return false; } } /// /// ConvertFrom /// ///public override object ConvertFrom( ITypeDescriptorContext td, CultureInfo cultureInfo, object value) { string stringValue = value as string; // Override the converter for sentinel values if (stringValue != null) { stringValue = stringValue.Trim(); if (stringValue == "Automatic") { return Duration.Automatic; } else if (stringValue == "Forever") { return Duration.Forever; } } TimeSpan duration = TimeSpan.Zero; if(_timeSpanConverter == null) { _timeSpanConverter = new TimeSpanConverter(); } duration = (TimeSpan)_timeSpanConverter.ConvertFrom(td, cultureInfo, value); return new Duration(duration); } /// /// TypeConverter method implementation. /// /// ITypeDescriptorContext /// current culture (see CLR specs) /// value to convert from /// Type to convert to ///converted value ////// /// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for Duration, not an arbitrary class /// [SecurityCritical] public override object ConvertTo( ITypeDescriptorContext context, CultureInfo cultureInfo, object value, Type destinationType) { if (destinationType != null && value is Duration) { Duration durationValue = (Duration)value; if (destinationType == typeof(InstanceDescriptor)) { MemberInfo mi; if (durationValue.HasTimeSpan) { mi = typeof(Duration).GetConstructor(new Type[] { typeof(TimeSpan) }); return new InstanceDescriptor(mi, new object[] { durationValue.TimeSpan }); } else if (durationValue == Duration.Forever) { mi = typeof(Duration).GetProperty("Forever"); return new InstanceDescriptor(mi, null); } else { Debug.Assert(durationValue == Duration.Automatic); // Only other legal duration type mi = typeof(Duration).GetProperty("Automatic"); return new InstanceDescriptor(mi, null); } } else if (destinationType == typeof(string)) { return durationValue.ToString(); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, cultureInfo, value, destinationType); } private static TimeSpanConverter _timeSpanConverter; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2004 // // File: DurationConverter.cs //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Security; namespace System.Windows { ////// Provides a type converter to convert Duration to and from other representations. /// public class DurationConverter : TypeConverter { ////// CanConvertFrom - Returns whether or not this class can convert from a given type /// ///public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } /// /// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible ///public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if ( destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } else { return false; } } /// /// ConvertFrom /// ///public override object ConvertFrom( ITypeDescriptorContext td, CultureInfo cultureInfo, object value) { string stringValue = value as string; // Override the converter for sentinel values if (stringValue != null) { stringValue = stringValue.Trim(); if (stringValue == "Automatic") { return Duration.Automatic; } else if (stringValue == "Forever") { return Duration.Forever; } } TimeSpan duration = TimeSpan.Zero; if(_timeSpanConverter == null) { _timeSpanConverter = new TimeSpanConverter(); } duration = (TimeSpan)_timeSpanConverter.ConvertFrom(td, cultureInfo, value); return new Duration(duration); } /// /// TypeConverter method implementation. /// /// ITypeDescriptorContext /// current culture (see CLR specs) /// value to convert from /// Type to convert to ///converted value ////// /// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for Duration, not an arbitrary class /// [SecurityCritical] public override object ConvertTo( ITypeDescriptorContext context, CultureInfo cultureInfo, object value, Type destinationType) { if (destinationType != null && value is Duration) { Duration durationValue = (Duration)value; if (destinationType == typeof(InstanceDescriptor)) { MemberInfo mi; if (durationValue.HasTimeSpan) { mi = typeof(Duration).GetConstructor(new Type[] { typeof(TimeSpan) }); return new InstanceDescriptor(mi, new object[] { durationValue.TimeSpan }); } else if (durationValue == Duration.Forever) { mi = typeof(Duration).GetProperty("Forever"); return new InstanceDescriptor(mi, null); } else { Debug.Assert(durationValue == Duration.Automatic); // Only other legal duration type mi = typeof(Duration).GetProperty("Automatic"); return new InstanceDescriptor(mi, null); } } else if (destinationType == typeof(string)) { return durationValue.ToString(); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, cultureInfo, value, destinationType); } private static TimeSpanConverter _timeSpanConverter; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
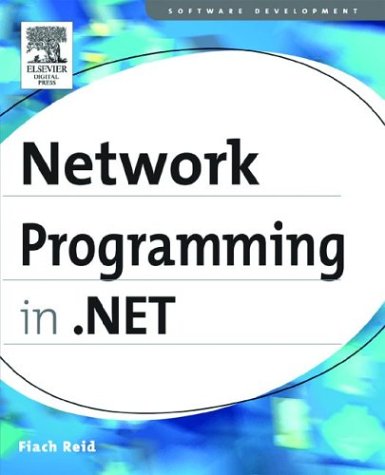
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EventBookmark.cs
- CleanUpVirtualizedItemEventArgs.cs
- _ListenerRequestStream.cs
- BaseTemplateParser.cs
- RecordsAffectedEventArgs.cs
- ErrorInfoXmlDocument.cs
- SHA384Managed.cs
- SchemaImporterExtension.cs
- ManifestResourceInfo.cs
- ValueSerializerAttribute.cs
- EntityTemplateFactory.cs
- TextEffect.cs
- ZipIOLocalFileBlock.cs
- X509ChainPolicy.cs
- SettingsAttributes.cs
- ChameleonKey.cs
- MemberPathMap.cs
- XamlStream.cs
- Margins.cs
- DetailsViewPagerRow.cs
- ManagedWndProcTracker.cs
- ObjectRef.cs
- SqlClientMetaDataCollectionNames.cs
- ZipPackage.cs
- _CookieModule.cs
- SessionStateSection.cs
- IItemContainerGenerator.cs
- VisualTarget.cs
- ExpandedWrapper.cs
- SqlInternalConnectionTds.cs
- Switch.cs
- WebRequestModuleElementCollection.cs
- XamlPathDataSerializer.cs
- XmlComment.cs
- StreamUpgradeAcceptor.cs
- DataSourceCacheDurationConverter.cs
- Point3DCollectionConverter.cs
- HttpCookiesSection.cs
- StructuralCache.cs
- ProfileProvider.cs
- Parameter.cs
- PreviewControlDesigner.cs
- XmlNodeChangedEventArgs.cs
- QueryLifecycle.cs
- DirectionalLight.cs
- TaskbarItemInfo.cs
- ObjectItemConventionAssemblyLoader.cs
- IriParsingElement.cs
- DllHostedComPlusServiceHost.cs
- ChoiceConverter.cs
- DataGridColumnStyleMappingNameEditor.cs
- IconConverter.cs
- GridItem.cs
- SafeArchiveContext.cs
- GrammarBuilder.cs
- LocalFileSettingsProvider.cs
- NumberFormatter.cs
- SerialStream.cs
- SiteMapNodeCollection.cs
- CommonObjectSecurity.cs
- PersonalizationStateInfo.cs
- EntityDataSourceValidationException.cs
- PassportPrincipal.cs
- UIElement3D.cs
- PrivateFontCollection.cs
- SplayTreeNode.cs
- Keywords.cs
- MailAddressCollection.cs
- DesignerActionListCollection.cs
- WindowsListView.cs
- ClientSettingsSection.cs
- ItemsControlAutomationPeer.cs
- ArgumentException.cs
- IisTraceListener.cs
- DataGridViewCellEventArgs.cs
- DataGridViewCellCancelEventArgs.cs
- RepeatBehavior.cs
- HttpModulesSection.cs
- TraceProvider.cs
- RelationshipWrapper.cs
- PanelStyle.cs
- ErrorProvider.cs
- TypeConverterValueSerializer.cs
- RadialGradientBrush.cs
- BufferedReadStream.cs
- QualifiedCellIdBoolean.cs
- EventEntry.cs
- HttpAsyncResult.cs
- MessageEventSubscriptionService.cs
- IERequestCache.cs
- Operand.cs
- HttpCachePolicyElement.cs
- WmfPlaceableFileHeader.cs
- DataReceivedEventArgs.cs
- TextBoxBase.cs
- IIS7WorkerRequest.cs
- MailMessageEventArgs.cs
- SqlDataSourceAdvancedOptionsForm.cs
- QilVisitor.cs
- UnsafeNativeMethods.cs