Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / DataFormats.cs / 1 / DataFormats.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Text; using System.Configuration.Assemblies; using System.Diagnostics; using System; using System.ComponentModel; using System.Runtime.InteropServices; using System.Globalization; ////// /// public class DataFormats { ///Translates /// between Win Forms text-based /// ///formats and 32-bit signed integer-based /// clipboard formats. Provides methods to create new formats and add /// them to the Windows Registry. /// /// public static readonly string Text = "Text"; ///Specifies the standard ANSI text format. This ////// field is read-only. /// /// public static readonly string UnicodeText = "UnicodeText"; ///Specifies the standard Windows Unicode text format. This /// ////// field is read-only. /// /// public static readonly string Dib = "DeviceIndependentBitmap"; ///Specifies the Windows Device Independent Bitmap (DIB) /// format. This ////// field is read-only. /// /// public static readonly string Bitmap = "Bitmap"; ///Specifies a Windows bitmap format. This ///field is read-only. /// /// public static readonly string EnhancedMetafile = "EnhancedMetafile"; ///Specifies the Windows enhanced metafile format. This /// ///field is read-only. /// /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly")] // Would be a breaking change to rename this public static readonly string MetafilePict = "MetaFilePict"; ///Specifies the Windows metafile format, which Win Forms /// does not directly use. This ////// field is read-only. /// /// public static readonly string SymbolicLink = "SymbolicLink"; ///Specifies the Windows symbolic link format, which Win /// Forms does not directly use. This ////// field is read-only. /// /// public static readonly string Dif = "DataInterchangeFormat"; ///Specifies the Windows data interchange format, which Win /// Forms does not directly use. This ////// field is read-only. /// /// public static readonly string Tiff = "TaggedImageFileFormat"; ///Specifies the Tagged Image File Format (TIFF), which Win /// Forms does not directly use. This ////// field is read-only. /// /// public static readonly string OemText = "OEMText"; ///Specifies the standard Windows original equipment /// manufacturer (OEM) text format. This ///field is read-only. /// /// public static readonly string Palette = "Palette"; ///Specifies the Windows palette format. This ////// field is read-only. /// /// public static readonly string PenData = "PenData"; ///Specifies the Windows pen data format, which consists of /// pen strokes for handwriting software; Win Forms does not use this format. This /// ////// field is read-only. /// /// public static readonly string Riff = "RiffAudio"; ///Specifies the Resource Interchange File Format (RIFF) /// audio format, which Win Forms does not directly use. This ///field is read-only. /// /// public static readonly string WaveAudio = "WaveAudio"; ///Specifies the wave audio format, which Win Forms does not /// directly use. This ///field is read-only. /// /// public static readonly string FileDrop = "FileDrop"; ///Specifies the Windows file drop format, which Win Forms /// does not directly use. This ////// field is read-only. /// /// public static readonly string Locale = "Locale"; ///Specifies the Windows culture format, which Win Forms does /// not directly use. This ///field is read-only. /// /// public static readonly string Html = "HTML Format"; ///Specifies text consisting of HTML data. This /// ///field is read-only. /// /// public static readonly string Rtf = "Rich Text Format"; ///Specifies text consisting of Rich Text Format (RTF) data. This /// ///field is read-only. /// /// public static readonly string CommaSeparatedValue = "Csv"; ///Specifies a comma-separated value (CSV) format, which is a /// common interchange format used by spreadsheets. This format is not used directly /// by Win Forms. This ////// field is read-only. /// /// // I'm sure upper case "String" is a reserved word in some language that matters public static readonly string StringFormat = typeof(string).FullName; //C#r: public static readonly String CF_STRINGCLASS = typeof(String).Name; ///Specifies the Win Forms string class format, which Win /// Forms uses to store string objects. This ////// field is read-only. /// /// public static readonly string Serializable = Application.WindowsFormsVersion + "PersistentObject"; private static Format[] formatList; private static int formatCount = 0; private static object internalSyncObject = new object(); // not creatable... // private DataFormats() { } ///Specifies a format that encapsulates any type of Win Forms /// object. This ///field is read-only. /// /// public static Format GetFormat(string format) { lock(internalSyncObject) { EnsurePredefined(); // It is much faster to do a case sensitive search here. So do // the case sensitive compare first, then the expensive one. // for (int n = 0; n < formatCount; n++) { if (formatList[n].Name.Equals(format)) return formatList[n]; } for (int n = 0; n < formatCount; n++) { if (String.Equals(formatList[n].Name, format, StringComparison.OrdinalIgnoreCase)) return formatList[n]; } // need to add this format string // int formatId = SafeNativeMethods.RegisterClipboardFormat(format); if (0 == formatId) { throw new Win32Exception(Marshal.GetLastWin32Error(), SR.GetString(SR.RegisterCFFailed)); } EnsureFormatSpace(1); formatList[formatCount] = new Format(format, formatId); return formatList[formatCount++]; } } ///Gets a ///with the Windows Clipboard numeric ID and name for the specified format. /// /// public static Format GetFormat(int id) { return InternalGetFormat( null, id ); } ///Gets a ///with the Windows Clipboard numeric /// ID and name for the specified ID. /// /// Allows a the new format name to be specified if the requested format is not /// in the list /// ///private static Format InternalGetFormat(string strName, int id) { lock(internalSyncObject) { EnsurePredefined(); for (int n = 0; n < formatCount; n++) { if ((short)formatList[n].Id == id) return formatList[n]; } StringBuilder s = new StringBuilder(128); // This can happen if windows adds a standard format that we don't know about, // so we should play it safe. // if (0 == SafeNativeMethods.GetClipboardFormatName(id, s, s.Capacity)) { s.Length = 0; if (strName == null) { s.Append( "Format" ).Append( id ); } else { s.Append( strName ); } } EnsureFormatSpace(1); formatList[formatCount] = new Format(s.ToString(), id); return formatList[formatCount++]; } } /// /// /// ensures that we have enough room in our format list /// ///private static void EnsureFormatSpace(int size) { if (null == formatList || formatList.Length <= formatCount + size) { int newSize = formatCount + 20; Format[] newList = new Format[newSize]; for (int n = 0; n < formatCount; n++) { newList[n] = formatList[n]; } formatList = newList; } } /// /// /// ensures that the Win32 predefined formats are setup in our format list. This /// is called anytime we need to search the list /// ///private static void EnsurePredefined() { if (0 == formatCount) { /* not used int standardText; // We must handle text differently for Win 95 and NT. We should put // UnicodeText on the clipboard for NT, and Text for Win 95. // So, we figure it out here theh first time someone asks for format info // if (1 == Marshal.SystemDefaultCharSize) { standardText = NativeMethods.CF_TEXT; } else { standardText = NativeMethods.CF_UNICODETEXT; } */ formatList = new Format [] { // Text name Win32 format ID Data stored as a Win32 handle? new Format(UnicodeText, NativeMethods.CF_UNICODETEXT), new Format(Text, NativeMethods.CF_TEXT), new Format(Bitmap, NativeMethods.CF_BITMAP), new Format(MetafilePict, NativeMethods.CF_METAFILEPICT), new Format(EnhancedMetafile, NativeMethods.CF_ENHMETAFILE), new Format(Dif, NativeMethods.CF_DIF), new Format(Tiff, NativeMethods.CF_TIFF), new Format(OemText, NativeMethods.CF_OEMTEXT), new Format(Dib, NativeMethods.CF_DIB), new Format(Palette, NativeMethods.CF_PALETTE), new Format(PenData, NativeMethods.CF_PENDATA), new Format(Riff, NativeMethods.CF_RIFF), new Format(WaveAudio, NativeMethods.CF_WAVE), new Format(SymbolicLink, NativeMethods.CF_SYLK), new Format(FileDrop, NativeMethods.CF_HDROP), new Format(Locale, NativeMethods.CF_LOCALE) }; formatCount = formatList.Length; } } /// /// /// public class Format { readonly string name; readonly int id; ///Represents a format type. ////// /// public string Name { get { return name; } } ////// Specifies the /// name of this format. This field is read-only. /// /// ////// /// public int Id { get { return id; } } ////// Specifies the ID /// number for this format. This field is read-only. /// ////// /// public Format(string name, int id) { this.name = name; this.id = id; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.Initializes a new instance of the ///class and specifies whether a /// /// handle is expected with this format.
Link Menu
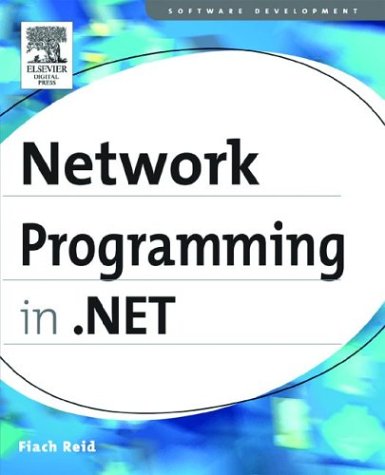
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FileDialogCustomPlace.cs
- ConnectionsZone.cs
- WebEventTraceProvider.cs
- CodeTypeDeclarationCollection.cs
- AlignmentYValidation.cs
- XXXInfos.cs
- NTAccount.cs
- mactripleDES.cs
- StylusDownEventArgs.cs
- WeakHashtable.cs
- HtmlInputPassword.cs
- FolderLevelBuildProviderCollection.cs
- HwndKeyboardInputProvider.cs
- ParagraphVisual.cs
- StringInfo.cs
- GPRECT.cs
- AsnEncodedData.cs
- ValueChangedEventManager.cs
- FirstMatchCodeGroup.cs
- TreeViewEvent.cs
- Control.cs
- TypeToken.cs
- DbMetaDataCollectionNames.cs
- XmlWrappingReader.cs
- CommandBinding.cs
- DynamicRendererThreadManager.cs
- EventProviderClassic.cs
- ObjectKeyFrameCollection.cs
- TypeToStringValueConverter.cs
- ToolStripDropTargetManager.cs
- ApplicationDirectory.cs
- LineServicesRun.cs
- WhereQueryOperator.cs
- CreateUserErrorEventArgs.cs
- TimeSpanValidatorAttribute.cs
- ScrollEventArgs.cs
- StickyNote.cs
- ExtendedTransformFactory.cs
- DataPagerFieldItem.cs
- DataSourceGroupCollection.cs
- DataTableNameHandler.cs
- WindowsAuthenticationEventArgs.cs
- TdsValueSetter.cs
- ScriptManagerProxy.cs
- DiscriminatorMap.cs
- UpDownBase.cs
- InterleavedZipPartStream.cs
- UpDownBaseDesigner.cs
- RadioButtonBaseAdapter.cs
- VariantWrapper.cs
- SubpageParaClient.cs
- ExternalException.cs
- CollectionsUtil.cs
- TypeConverter.cs
- ListViewVirtualItemsSelectionRangeChangedEvent.cs
- TypeElement.cs
- ExpressionDumper.cs
- UICuesEvent.cs
- HwndSourceKeyboardInputSite.cs
- log.cs
- PlaceHolder.cs
- DataContractAttribute.cs
- MultiSelectRootGridEntry.cs
- RegexReplacement.cs
- IPCCacheManager.cs
- InputMethod.cs
- EncoderExceptionFallback.cs
- LineGeometry.cs
- StyleReferenceConverter.cs
- TrackingStringDictionary.cs
- SHA1.cs
- SystemIPGlobalProperties.cs
- MapPathBasedVirtualPathProvider.cs
- Stack.cs
- DocumentViewer.cs
- WebRequestModulesSection.cs
- TreeNodeCollection.cs
- DictionaryEntry.cs
- Span.cs
- CommandValueSerializer.cs
- XmlWrappingReader.cs
- HttpClientCertificate.cs
- FileUpload.cs
- UpdatePanelControlTrigger.cs
- EntityDataSourceSelectedEventArgs.cs
- AutoCompleteStringCollection.cs
- XmlWriterTraceListener.cs
- XpsS0ValidatingLoader.cs
- ConsoleCancelEventArgs.cs
- SystemDropShadowChrome.cs
- HostedHttpContext.cs
- AsyncOperation.cs
- FormsIdentity.cs
- FrameworkTextComposition.cs
- OleDbDataReader.cs
- GridSplitter.cs
- XmlEncoding.cs
- InternalControlCollection.cs
- DataGridTextBoxColumn.cs
- ReadOnlyDictionary.cs