Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Input / Command / CommandValueSerializer.cs / 1 / CommandValueSerializer.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2005 // // File: CommandValueSerializer.cs // // Contents: ValueSerializer for the ICommand interface // // Created: 04/28/2005 [....] // //----------------------------------------------------------------------- namespace System.Windows.Input { using System; using System.Collections.Generic; using System.Text; using System.Windows.Markup; using System.Windows.Documents; // EditingCommands using System.Reflection; internal class CommandValueSerializer : ValueSerializer { public override bool CanConvertToString(object value, IValueSerializerContext context) { if (context == null || context.GetValueSerializerFor(typeof(Type)) == null) return false; // Can only convert routed commands RoutedCommand command = value as RoutedCommand; if (command == null || command.OwnerType == null) { return false; } if (CommandConverter.IsKnownType(command.OwnerType)) { return true; } else { string localName = command.Name + "Command"; Type ownerType = command.OwnerType; string typeName = ownerType.Name; // Get them from Properties PropertyInfo propertyInfo = ownerType.GetProperty(localName, BindingFlags.Public | BindingFlags.Static); if (propertyInfo != null) return true; // Get them from Fields (ScrollViewer.PageDownCommand is a static readonly field FieldInfo fieldInfo = ownerType.GetField(localName, BindingFlags.Static | BindingFlags.Public); if (fieldInfo != null) return true; } return false; } public override bool CanConvertFromString(string value, IValueSerializerContext context) { return true; } public override string ConvertToString(object value, IValueSerializerContext context) { if (value != null) { RoutedCommand command = value as RoutedCommand; if (null != command && null != command.OwnerType) { // Known Commands, so write shorter version if (CommandConverter.IsKnownType(command.OwnerType)) { return command.Name; } else { ValueSerializer typeSerializer = null; if (context == null) { throw new InvalidOperationException(SR.Get(SRID.ValueSerializerContextUnavailable, this.GetType().Name )); } // Get the ValueSerializer for the System.Type type typeSerializer = context.GetValueSerializerFor(typeof(Type)); if (typeSerializer == null) { throw new InvalidOperationException(SR.Get(SRID.TypeValueSerializerUnavailable, this.GetType().Name )); } return typeSerializer.ConvertToString(command.OwnerType, context) + "." + command.Name + "Command"; } } } else return string.Empty; throw GetConvertToException(value, typeof(string)); } public override IEnumerableTypeReferences(object value, IValueSerializerContext context) { if (value != null) { RoutedCommand command = value as RoutedCommand; if (command != null) { if (command.OwnerType != null && !CommandConverter.IsKnownType(command.OwnerType)) { return new Type[] { command.OwnerType }; } } } return base.TypeReferences(value, context); } public override object ConvertFromString(string value, IValueSerializerContext context) { if (value != null) { if (value != String.Empty) { Type declaringType = null; String commandName; // Check for "ns:Class.Command" syntax. int dotIndex = value.IndexOf('.'); if (dotIndex >= 0) { // We have "ns:Class.Command" syntax. // Find the type name in the form of "ns:Class". string typeName = value.Substring(0, dotIndex); if (context == null) { throw new InvalidOperationException(SR.Get(SRID.ValueSerializerContextUnavailable, this.GetType().Name )); } // Get the ValueSerializer for the System.Type type ValueSerializer typeSerializer = context.GetValueSerializerFor(typeof(Type)); if (typeSerializer == null) { throw new InvalidOperationException(SR.Get(SRID.TypeValueSerializerUnavailable, this.GetType().Name )); } // Use the TypeValueSerializer to parse the "ns:Class" into a System.Type. declaringType = typeSerializer.ConvertFromString(typeName, context) as Type; // Strip out the "Command" part of "ns:Class.Command". commandName = value.Substring(dotIndex + 1).Trim(); } else { // Assume the known commands commandName = value.Trim(); } // Find the command given the declaring type & name (this is shared with CommandConverter) ICommand command = CommandConverter.ConvertFromHelper( declaringType, commandName ); if (command != null) { return command; } } else { return null; // String.Empty <==> null , (for roundtrip cases where Command property values are null) } } return base.ConvertFromString(value, context); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2005 // // File: CommandValueSerializer.cs // // Contents: ValueSerializer for the ICommand interface // // Created: 04/28/2005 [....] // //----------------------------------------------------------------------- namespace System.Windows.Input { using System; using System.Collections.Generic; using System.Text; using System.Windows.Markup; using System.Windows.Documents; // EditingCommands using System.Reflection; internal class CommandValueSerializer : ValueSerializer { public override bool CanConvertToString(object value, IValueSerializerContext context) { if (context == null || context.GetValueSerializerFor(typeof(Type)) == null) return false; // Can only convert routed commands RoutedCommand command = value as RoutedCommand; if (command == null || command.OwnerType == null) { return false; } if (CommandConverter.IsKnownType(command.OwnerType)) { return true; } else { string localName = command.Name + "Command"; Type ownerType = command.OwnerType; string typeName = ownerType.Name; // Get them from Properties PropertyInfo propertyInfo = ownerType.GetProperty(localName, BindingFlags.Public | BindingFlags.Static); if (propertyInfo != null) return true; // Get them from Fields (ScrollViewer.PageDownCommand is a static readonly field FieldInfo fieldInfo = ownerType.GetField(localName, BindingFlags.Static | BindingFlags.Public); if (fieldInfo != null) return true; } return false; } public override bool CanConvertFromString(string value, IValueSerializerContext context) { return true; } public override string ConvertToString(object value, IValueSerializerContext context) { if (value != null) { RoutedCommand command = value as RoutedCommand; if (null != command && null != command.OwnerType) { // Known Commands, so write shorter version if (CommandConverter.IsKnownType(command.OwnerType)) { return command.Name; } else { ValueSerializer typeSerializer = null; if (context == null) { throw new InvalidOperationException(SR.Get(SRID.ValueSerializerContextUnavailable, this.GetType().Name )); } // Get the ValueSerializer for the System.Type type typeSerializer = context.GetValueSerializerFor(typeof(Type)); if (typeSerializer == null) { throw new InvalidOperationException(SR.Get(SRID.TypeValueSerializerUnavailable, this.GetType().Name )); } return typeSerializer.ConvertToString(command.OwnerType, context) + "." + command.Name + "Command"; } } } else return string.Empty; throw GetConvertToException(value, typeof(string)); } public override IEnumerable TypeReferences(object value, IValueSerializerContext context) { if (value != null) { RoutedCommand command = value as RoutedCommand; if (command != null) { if (command.OwnerType != null && !CommandConverter.IsKnownType(command.OwnerType)) { return new Type[] { command.OwnerType }; } } } return base.TypeReferences(value, context); } public override object ConvertFromString(string value, IValueSerializerContext context) { if (value != null) { if (value != String.Empty) { Type declaringType = null; String commandName; // Check for "ns:Class.Command" syntax. int dotIndex = value.IndexOf('.'); if (dotIndex >= 0) { // We have "ns:Class.Command" syntax. // Find the type name in the form of "ns:Class". string typeName = value.Substring(0, dotIndex); if (context == null) { throw new InvalidOperationException(SR.Get(SRID.ValueSerializerContextUnavailable, this.GetType().Name )); } // Get the ValueSerializer for the System.Type type ValueSerializer typeSerializer = context.GetValueSerializerFor(typeof(Type)); if (typeSerializer == null) { throw new InvalidOperationException(SR.Get(SRID.TypeValueSerializerUnavailable, this.GetType().Name )); } // Use the TypeValueSerializer to parse the "ns:Class" into a System.Type. declaringType = typeSerializer.ConvertFromString(typeName, context) as Type; // Strip out the "Command" part of "ns:Class.Command". commandName = value.Substring(dotIndex + 1).Trim(); } else { // Assume the known commands commandName = value.Trim(); } // Find the command given the declaring type & name (this is shared with CommandConverter) ICommand command = CommandConverter.ConvertFromHelper( declaringType, commandName ); if (command != null) { return command; } } else { return null; // String.Empty <==> null , (for roundtrip cases where Command property values are null) } } return base.ConvertFromString(value, context); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
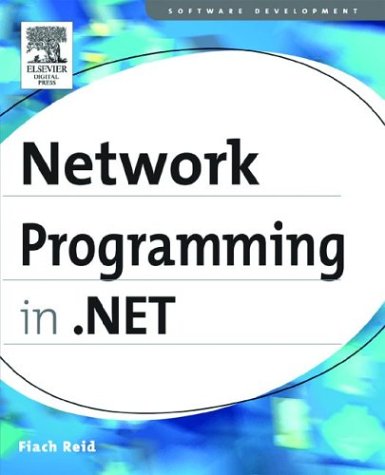
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NavigationHelper.cs
- XamlPointCollectionSerializer.cs
- DynamicPropertyReader.cs
- SmiSettersStream.cs
- TCPListener.cs
- RegexCharClass.cs
- WindowsGraphicsCacheManager.cs
- HandlerBase.cs
- BrowserCapabilitiesFactoryBase.cs
- HwndStylusInputProvider.cs
- IDReferencePropertyAttribute.cs
- BitSet.cs
- DescriptionAttribute.cs
- DBSchemaTable.cs
- PaperSource.cs
- EdmComplexPropertyAttribute.cs
- ResizeGrip.cs
- ToolStripDropDownClosedEventArgs.cs
- ContractInferenceHelper.cs
- SqlUtils.cs
- RegexStringValidator.cs
- TypeGeneratedEventArgs.cs
- HwndKeyboardInputProvider.cs
- GridViewUpdatedEventArgs.cs
- LOSFormatter.cs
- PKCS1MaskGenerationMethod.cs
- TypeSource.cs
- DesignerTransactionCloseEvent.cs
- AssemblyInfo.cs
- DefaultCompensation.cs
- PtsPage.cs
- ApplicationManager.cs
- KeyNotFoundException.cs
- BitmapCodecInfo.cs
- LinqDataSource.cs
- EmbeddedObject.cs
- ToolStripSplitButton.cs
- ObjectStateManagerMetadata.cs
- TempEnvironment.cs
- WorkflowMarkupSerializer.cs
- Light.cs
- IssuedSecurityTokenProvider.cs
- ForceCopyBuildProvider.cs
- TableRowGroup.cs
- PrivateFontCollection.cs
- FamilyTypeface.cs
- DbDataSourceEnumerator.cs
- DataPagerFieldItem.cs
- CompositeTypefaceMetrics.cs
- MD5CryptoServiceProvider.cs
- AuthorizationSection.cs
- DetailsView.cs
- NumericUpDownAccelerationCollection.cs
- WebBrowsableAttribute.cs
- PasswordRecoveryAutoFormat.cs
- PointAnimation.cs
- MultidimensionalArrayItemReference.cs
- DocumentReferenceCollection.cs
- XmlHierarchicalEnumerable.cs
- InputMethod.cs
- PropertyEntry.cs
- CallbackBehaviorAttribute.cs
- QilUnary.cs
- HtmlFormParameterReader.cs
- WebPartManagerDesigner.cs
- XmlDigitalSignatureProcessor.cs
- _AcceptOverlappedAsyncResult.cs
- CardSpacePolicyElement.cs
- COSERVERINFO.cs
- XmlDocumentSerializer.cs
- SerializationStore.cs
- XmlSchemaDatatype.cs
- FontStyles.cs
- XmlSchemaExternal.cs
- CommaDelimitedStringAttributeCollectionConverter.cs
- XamlToRtfParser.cs
- Relationship.cs
- DataListCommandEventArgs.cs
- CorrelationTokenInvalidatedHandler.cs
- WebPartConnectVerb.cs
- PolyQuadraticBezierSegment.cs
- unsafenativemethodsother.cs
- WorkflowMessageEventArgs.cs
- FakeModelPropertyImpl.cs
- EncodingNLS.cs
- ContentPropertyAttribute.cs
- SystemResources.cs
- MexHttpBindingElement.cs
- KeyToListMap.cs
- FixedPageAutomationPeer.cs
- NamespaceList.cs
- ObjRef.cs
- SafePointer.cs
- UseAttributeSetsAction.cs
- MemoryMappedViewAccessor.cs
- RoutedUICommand.cs
- ParallelDesigner.cs
- FrameworkPropertyMetadata.cs
- XmlSchemaObjectCollection.cs
- DataGridViewRowContextMenuStripNeededEventArgs.cs