Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / CallbackBehaviorAttribute.cs / 1 / CallbackBehaviorAttribute.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel { using System.ServiceModel.Administration; using System.ServiceModel.Channels; using System.ServiceModel.Dispatcher; using System.ServiceModel.Description; using System.ServiceModel.Configuration; using System.Runtime.Serialization; using System.Collections.ObjectModel; using System.Collections.Generic; using System.Threading; using System.Transactions; using System.Runtime.CompilerServices; [AttributeUsage(ServiceModelAttributeTargets.CallbackBehavior)] public sealed class CallbackBehaviorAttribute : Attribute, IEndpointBehavior { ConcurrencyMode concurrencyMode = ConcurrencyMode.Single; bool includeExceptionDetailInFaults = false; bool validateMustUnderstand = true; bool ignoreExtensionDataObject = DataContractSerializerDefaults.IgnoreExtensionDataObject; int maxItemsInObjectGraph = DataContractSerializerDefaults.MaxItemsInObjectGraph; bool automaticSessionShutdown = true; bool useSynchronizationContext = true; internal static IsolationLevel DefaultIsolationLevel = IsolationLevel.Unspecified; IsolationLevel transactionIsolationLevel = DefaultIsolationLevel; bool isolationLevelSet = false; TimeSpan transactionTimeout = TimeSpan.Zero; string transactionTimeoutString; bool transactionTimeoutSet = false; public bool AutomaticSessionShutdown { get { return this.automaticSessionShutdown; } set { this.automaticSessionShutdown = value; } } public IsolationLevel TransactionIsolationLevel { get { return this.transactionIsolationLevel; } set { switch (value) { case IsolationLevel.Serializable: case IsolationLevel.RepeatableRead: case IsolationLevel.ReadCommitted: case IsolationLevel.ReadUncommitted: case IsolationLevel.Unspecified: case IsolationLevel.Chaos: case IsolationLevel.Snapshot: break; default: throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("value")); } this.transactionIsolationLevel = value; isolationLevelSet = true; } } internal bool IsolationLevelSet { get { return this.isolationLevelSet; } } public bool IncludeExceptionDetailInFaults { get { return this.includeExceptionDetailInFaults; } set { this.includeExceptionDetailInFaults = value; } } public ConcurrencyMode ConcurrencyMode { get { return this.concurrencyMode; } set { if (!ConcurrencyModeHelper.IsDefined(value)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("value")); } this.concurrencyMode = value; } } public string TransactionTimeout { get { return transactionTimeoutString; } set { if (value == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("value")); } try { TimeSpan timeout = TimeSpan.Parse(value); if (timeout < TimeSpan.Zero) { string message = SR.GetString(SR.SFxTimeoutOutOfRange0); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("value", value, message)); } this.transactionTimeout = timeout; this.transactionTimeoutString = value; this.transactionTimeoutSet = true; } catch (FormatException e) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentException(SR.GetString(SR.SFxTimeoutInvalidStringFormat), "value", e)); } catch (OverflowException) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("value")); } } } internal bool TransactionTimeoutSet { get { return this.transactionTimeoutSet; } } public bool UseSynchronizationContext { get { return this.useSynchronizationContext; } set { this.useSynchronizationContext = value; } } public bool ValidateMustUnderstand { get { return validateMustUnderstand; } set { validateMustUnderstand = value; } } public bool IgnoreExtensionDataObject { get { return ignoreExtensionDataObject; } set { ignoreExtensionDataObject = value; } } public int MaxItemsInObjectGraph { get { return maxItemsInObjectGraph; } set { maxItemsInObjectGraph = value; } } [MethodImpl(MethodImplOptions.NoInlining)] void SetIsolationLevel(ChannelDispatcher channelDispatcher) { if (channelDispatcher == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("channelDispatcher"); } channelDispatcher.TransactionIsolationLevel = this.transactionIsolationLevel; } void IEndpointBehavior.Validate(ServiceEndpoint serviceEndpoint) { } void IEndpointBehavior.AddBindingParameters(ServiceEndpoint serviceEndpoint, BindingParameterCollection parameters) { } void IEndpointBehavior.ApplyClientBehavior(ServiceEndpoint serviceEndpoint, ClientRuntime clientRuntime) { if (!serviceEndpoint.Contract.IsDuplex()) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString( SR.SFxCallbackBehaviorAttributeOnlyOnDuplex, serviceEndpoint.Contract.Name))); } DispatchRuntime dispatchRuntime = clientRuntime.DispatchRuntime; dispatchRuntime.ValidateMustUnderstand = validateMustUnderstand; dispatchRuntime.ConcurrencyMode = this.concurrencyMode; dispatchRuntime.ChannelDispatcher.IncludeExceptionDetailInFaults = this.includeExceptionDetailInFaults; dispatchRuntime.AutomaticInputSessionShutdown = this.automaticSessionShutdown; if (!this.useSynchronizationContext) { dispatchRuntime.SynchronizationContext = null; } dispatchRuntime.ChannelDispatcher.TransactionTimeout = transactionTimeout; if (isolationLevelSet) { SetIsolationLevel(dispatchRuntime.ChannelDispatcher); } DataContractSerializerServiceBehavior.ApplySerializationSettings(serviceEndpoint, this.ignoreExtensionDataObject, this.maxItemsInObjectGraph); } void IEndpointBehavior.ApplyDispatchBehavior(ServiceEndpoint serviceEndpoint, EndpointDispatcher endpointDispatcher) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException( SR.GetString(SR.SFXEndpointBehaviorUsedOnWrongSide, typeof(CallbackBehaviorAttribute).Name))); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
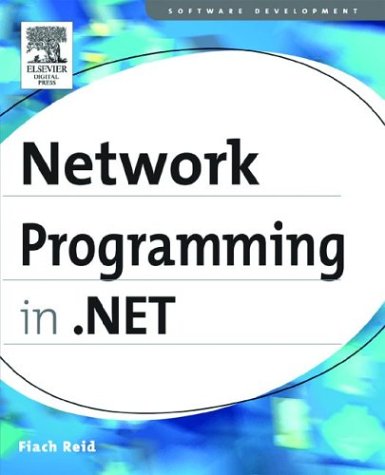
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridParentRows.cs
- DateTimeSerializationSection.cs
- MULTI_QI.cs
- DragDeltaEventArgs.cs
- CodeTypeParameterCollection.cs
- ExpressionLink.cs
- LineProperties.cs
- Encoding.cs
- KeyedHashAlgorithm.cs
- Property.cs
- EntityDataSourceContextDisposingEventArgs.cs
- UInt16Storage.cs
- SequentialOutput.cs
- XsdDateTime.cs
- Int16Converter.cs
- RestHandler.cs
- CroppedBitmap.cs
- EntityDataSourceWrapperPropertyDescriptor.cs
- ClientSettingsStore.cs
- StructuredTypeEmitter.cs
- DiscriminatorMap.cs
- RuleElement.cs
- SHA1.cs
- DataGridItemEventArgs.cs
- odbcmetadatacollectionnames.cs
- NonParentingControl.cs
- DesignerCategoryAttribute.cs
- Button.cs
- CategoryState.cs
- WindowsComboBox.cs
- RecordsAffectedEventArgs.cs
- EventPropertyMap.cs
- _LocalDataStore.cs
- ImageAnimator.cs
- SmiMetaData.cs
- DataKey.cs
- FieldAccessException.cs
- SqlConnectionPoolGroupProviderInfo.cs
- HttpInputStream.cs
- BookmarkInfo.cs
- TextReader.cs
- CodeTypeDelegate.cs
- GlobalProxySelection.cs
- BlobPersonalizationState.cs
- TreeViewCancelEvent.cs
- StorageEndPropertyMapping.cs
- DataSpaceManager.cs
- DBDataPermissionAttribute.cs
- FixedDocumentPaginator.cs
- Zone.cs
- RealProxy.cs
- sitestring.cs
- DataGridViewHitTestInfo.cs
- Binding.cs
- ColorContext.cs
- Utilities.cs
- InfoCardService.cs
- AuthorizationSection.cs
- LoadRetryConstantStrategy.cs
- RandomNumberGenerator.cs
- Point3DKeyFrameCollection.cs
- DesignerTransactionCloseEvent.cs
- BitmapFrameEncode.cs
- KeyedPriorityQueue.cs
- XmlSchemaSubstitutionGroup.cs
- ClientType.cs
- BindingContext.cs
- MenuCommandService.cs
- AutoGeneratedField.cs
- httpserverutility.cs
- EtwTrace.cs
- SubclassTypeValidator.cs
- SoapAttributeAttribute.cs
- cookieexception.cs
- CannotUnloadAppDomainException.cs
- ColumnHeaderConverter.cs
- exports.cs
- DataServiceRequestOfT.cs
- UpdateCommand.cs
- InternalConfigRoot.cs
- StackBuilderSink.cs
- MessageQueueException.cs
- _IPv4Address.cs
- FontSource.cs
- SafeFileMappingHandle.cs
- CompatibleIComparer.cs
- Msec.cs
- ProcessProtocolHandler.cs
- FixedDocumentPaginator.cs
- SkewTransform.cs
- CompilerScope.Storage.cs
- TextSimpleMarkerProperties.cs
- RC2.cs
- AsyncPostBackTrigger.cs
- DeleteHelper.cs
- KoreanCalendar.cs
- IdentityModelDictionary.cs
- CompilerGlobalScopeAttribute.cs
- ToolStripButton.cs
- NamespaceImport.cs