Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / MS / Internal / Text / LineProperties.cs / 1 / LineProperties.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: LineProperties.cs // // Description: Text line properties provider. // // History: // 04/25/2003 : grzegorz - moving from Avalon branch. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using System.Windows.Controls; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Media.TextFormatting; using MS.Internal.Documents; using MS.Internal.PtsHost; // TextParagraph namespace MS.Internal.Text { // --------------------------------------------------------------------- // Text line properties provider. // --------------------------------------------------------------------- internal class LineProperties : TextParagraphProperties { // ------------------------------------------------------------------ // // TextParagraphProperties Implementation // // ----------------------------------------------------------------- #region TextParagraphProperties Implementation ////// This property specifies whether the primary text advance /// direction shall be left-to-right, right-to-left, or top-to-bottom. /// public override FlowDirection FlowDirection { get { return _flowDirection; } } ////// This property describes how inline content of a block is aligned. /// public override TextAlignment TextAlignment { get { return IgnoreTextAlignment ? TextAlignment.Left : _textAlignment; } } ////// Paragraph's line height /// ////// TextFormatter does not do appropriate line height handling, so /// report always 0 as line height. /// Line height is handled by TextFormatter host. /// public override double LineHeight { get { if (LineStackingStrategy == LineStackingStrategy.BlockLineHeight && !Double.IsNaN(_lineHeight)) { return _lineHeight; } return 0.0; } } ////// Indicates the first line of the paragraph. /// public override bool FirstLineInParagraph { get { return false; } } ////// Paragraph's default run properties /// public override TextRunProperties DefaultTextRunProperties { get { return _defaultTextProperties; } } ////// Text decorations specified at the paragraph level. /// public override TextDecorationCollection TextDecorations { get { return _defaultTextProperties.TextDecorations; } } ////// This property controls whether or not text wraps when it reaches the flow edge /// of its containing block box /// public override TextWrapping TextWrapping { get { return _textWrapping; } } ////// This property specifies marker characteristics of the first line in paragraph /// public override TextMarkerProperties TextMarkerProperties { get { return _markerProperties; } } ////// Line indentation /// ////// Line indentation. Line indent by default is always 0. /// Use FirstLineProperties class to return real value of this property. /// public override double Indent { get { return 0.0; } } #endregion TextParagraphProperties Implementation ////// Constructor. /// internal LineProperties( DependencyObject element, DependencyObject contentHost, TextProperties defaultTextProperties, MarkerProperties markerProperties) : this(element, contentHost, defaultTextProperties, markerProperties, (TextAlignment)element.GetValue(Block.TextAlignmentProperty)) { } ////// Constructor. /// internal LineProperties( DependencyObject element, DependencyObject contentHost, TextProperties defaultTextProperties, MarkerProperties markerProperties, TextAlignment textAlignment) { _defaultTextProperties = defaultTextProperties; _markerProperties = (markerProperties != null) ? markerProperties.GetTextMarkerProperties(this) : null; _flowDirection = (FlowDirection)element.GetValue(Block.FlowDirectionProperty); _textAlignment = textAlignment; _lineHeight = (double)element.GetValue(Block.LineHeightProperty); _textIndent = (double)element.GetValue(Paragraph.TextIndentProperty); _lineStackingStrategy = (LineStackingStrategy)element.GetValue(Block.LineStackingStrategyProperty); _textWrapping = TextWrapping.Wrap; _textTrimming = TextTrimming.None; if (contentHost is TextBlock || contentHost is ITextBoxViewHost) { // NB: we intentially don't try to find the "PropertyOwner" of // a FlowDocument here. TextWrapping has a hard-coded // value SetValue'd when a FlowDocument is hosted by a TextBoxBase. _textWrapping = (TextWrapping)contentHost.GetValue(TextBlock.TextWrappingProperty); _textTrimming = (TextTrimming)contentHost.GetValue(TextBlock.TextTrimmingProperty); } else if (contentHost is FlowDocument) { _textWrapping = ((FlowDocument)contentHost).TextWrapping; } } ////// Calculate line advance for TextParagraphs - this method has special casing in case the LineHeight property on the Paragraph element /// needs to be respected /// /// /// TextParagraph that owns the line /// /// /// Dcp of the line /// /// /// Calculated height of the line /// internal double CalcLineAdvanceForTextParagraph(TextParagraph textParagraph, int dcp, double lineAdvance) { if (!DoubleUtil.IsNaN(_lineHeight)) { switch (LineStackingStrategy) { case LineStackingStrategy.BlockLineHeight: lineAdvance = _lineHeight; break; case LineStackingStrategy.MaxHeight: default: if (dcp == 0 && textParagraph.HasFiguresOrFloaters() && ((textParagraph.GetLastDcpAttachedObjectBeforeLine(0) + textParagraph.ParagraphStartCharacterPosition) == textParagraph.ParagraphEndCharacterPosition)) { // The Paragraph element contains only figures and floaters and has LineHeight set. In this case LineHeight // should be respected lineAdvance = _lineHeight; } else { lineAdvance = Math.Max(lineAdvance, _lineHeight); } break; // case LineStackingStrategy.InlineLineHeight: // // Inline uses the height of the line just processed. // break; // case LineStackingStrategy.GridHeight: // lineAdvance = (((TextDpi.ToTextDpi(lineAdvance) - 1) / TextDpi.ToTextDpi(_lineHeight)) + 1) * _lineHeight; // break; //} } } return lineAdvance; } ////// Calculate line advance from actual line height and the line stacking strategy. /// internal double CalcLineAdvance(double lineAdvance) { // We support MaxHeight and BlockLineHeight stacking strategies if (!DoubleUtil.IsNaN(_lineHeight)) { switch (LineStackingStrategy) { case LineStackingStrategy.BlockLineHeight: lineAdvance = _lineHeight; break; case LineStackingStrategy.MaxHeight: default: lineAdvance = Math.Max(lineAdvance, _lineHeight); break; // case LineStackingStrategy.InlineLineHeight: // // Inline uses the height of the line just processed. // break; // case LineStackingStrategy.GridHeight: // lineAdvance = (((TextDpi.ToTextDpi(lineAdvance) - 1) / TextDpi.ToTextDpi(_lineHeight)) + 1) * _lineHeight; // break; //} } } return lineAdvance; } ////// Raw TextAlignment, without considering IgnoreTextAlignment. /// internal TextAlignment TextAlignmentInternal { get { return _textAlignment; } } ////// Ignore text alignment? /// internal bool IgnoreTextAlignment { get { return _ignoreTextAlignment; } set { _ignoreTextAlignment = value; } } ////////// Line stacking strategy. ///// internal LineStackingStrategy LineStackingStrategy { get { return _lineStackingStrategy; } } ////// Text trimming. /// internal TextTrimming TextTrimming { get { return _textTrimming; } } ////// Does it have first line specific properties? /// internal bool HasFirstLineProperties { get { return (_markerProperties != null || !DoubleUtil.IsZero(_textIndent)); } } ////// Local cache for first line properties. /// internal TextParagraphProperties FirstLineProps { get { if (_firstLineProperties == null) { _firstLineProperties = new FirstLineProperties(this); } return _firstLineProperties; } } // ------------------------------------------------------------------ // Local cache for line properties with paragraph ellipsis. // ------------------------------------------------------------------ ////// Local cache for line properties with paragraph ellipsis. /// internal TextParagraphProperties GetParaEllipsisLineProps(bool firstLine) { return new ParaEllipsisLineProperties(firstLine ? FirstLineProps : this); } private TextRunProperties _defaultTextProperties; // Line's default text properties. private TextMarkerProperties _markerProperties; // Marker properties private FirstLineProperties _firstLineProperties; // Local cache for first line properties. private bool _ignoreTextAlignment; // Ignore horizontal alignment? private FlowDirection _flowDirection; private TextAlignment _textAlignment; private TextWrapping _textWrapping; private TextTrimming _textTrimming; private double _lineHeight; private double _textIndent; private LineStackingStrategy _lineStackingStrategy; // --------------------------------------------------------------------- // First text line properties provider. // ---------------------------------------------------------------------- private sealed class FirstLineProperties : TextParagraphProperties { // ----------------------------------------------------------------- // // TextParagraphProperties Implementation // // ----------------------------------------------------------------- #region TextParagraphProperties Implementation // ----------------------------------------------------------------- // Text flow direction (text advance + block advance direction). // ------------------------------------------------------------------ public override FlowDirection FlowDirection { get { return _lp.FlowDirection; } } // ----------------------------------------------------------------- // Alignment of the line's content. // ------------------------------------------------------------------ public override TextAlignment TextAlignment { get { return _lp.TextAlignment; } } // ------------------------------------------------------------------ // Line's height. // ----------------------------------------------------------------- public override double LineHeight { get { return _lp.LineHeight; } } // ------------------------------------------------------------------ // An instance of this class is always the first line in a paragraph. // ----------------------------------------------------------------- public override bool FirstLineInParagraph { get { return true; } } // ----------------------------------------------------------------- // Line's default text properties. // ----------------------------------------------------------------- public override TextRunProperties DefaultTextRunProperties { get { return _lp.DefaultTextRunProperties; } } // ------------------------------------------------------------------ // Line's text decorations (in addition to any run-level text decorations). // ----------------------------------------------------------------- public override TextDecorationCollection TextDecorations { get { return _lp.TextDecorations; } } // ------------------------------------------------------------------ // Text wrap control. // ------------------------------------------------------------------ public override TextWrapping TextWrapping { get { return _lp.TextWrapping; } } // ----------------------------------------------------------------- // Marker characteristics of the first line in paragraph. // ------------------------------------------------------------------ public override TextMarkerProperties TextMarkerProperties { get { return _lp.TextMarkerProperties; } } // ----------------------------------------------------------------- // Line indentation. // ----------------------------------------------------------------- public override double Indent { get { return _lp._textIndent; } } #endregion TextParagraphProperties Implementation // ----------------------------------------------------------------- // Constructor. // ------------------------------------------------------------------ internal FirstLineProperties(LineProperties lp) { _lp = lp; // properly set the local copy of hyphenator accordingly. Hyphenator = lp.Hyphenator; } // ----------------------------------------------------------------- // LineProperties object reference. // ------------------------------------------------------------------ private LineProperties _lp; } // ---------------------------------------------------------------------- // Line properties provider for line with paragraph ellipsis. // --------------------------------------------------------------------- private sealed class ParaEllipsisLineProperties : TextParagraphProperties { // ------------------------------------------------------------------ // // TextParagraphProperties Implementation // // ----------------------------------------------------------------- #region TextParagraphProperties Implementation // ----------------------------------------------------------------- // Text flow direction (text advance + block advance direction). // ----------------------------------------------------------------- public override FlowDirection FlowDirection { get { return _lp.FlowDirection; } } // ------------------------------------------------------------------ // Alignment of the line's content. // ----------------------------------------------------------------- public override TextAlignment TextAlignment { get { return _lp.TextAlignment; } } // ------------------------------------------------------------------ // Line's height. // ------------------------------------------------------------------ public override double LineHeight { get { return _lp.LineHeight; } } // ----------------------------------------------------------------- // First line in paragraph option. // ------------------------------------------------------------------ public override bool FirstLineInParagraph { get { return _lp.FirstLineInParagraph; } } // ----------------------------------------------------------------- // Always collapsible option. // ----------------------------------------------------------------- public override bool AlwaysCollapsible { get { return _lp.AlwaysCollapsible; } } // ----------------------------------------------------------------- // Line's default text properties. // ------------------------------------------------------------------ public override TextRunProperties DefaultTextRunProperties { get { return _lp.DefaultTextRunProperties; } } // ----------------------------------------------------------------- // Line's text decorations (in addition to any run-level text decorations). // ------------------------------------------------------------------ public override TextDecorationCollection TextDecorations { get { return _lp.TextDecorations; } } // ------------------------------------------------------------------ // Text wrap control. // If paragraph ellipsis are enabled, force this line not to wrap. // ----------------------------------------------------------------- public override TextWrapping TextWrapping { get { return TextWrapping.NoWrap; } } // ------------------------------------------------------------------ // Marker characteristics of the first line in paragraph. // ----------------------------------------------------------------- public override TextMarkerProperties TextMarkerProperties { get { return _lp.TextMarkerProperties; } } // ----------------------------------------------------------------- // Line indentation. // ----------------------------------------------------------------- public override double Indent { get { return _lp.Indent; } } #endregion TextParagraphProperties Implementation // ------------------------------------------------------------------ // Constructor. // ----------------------------------------------------------------- internal ParaEllipsisLineProperties(TextParagraphProperties lp) { _lp = lp; } // ------------------------------------------------------------------ // LineProperties object reference. // ------------------------------------------------------------------ private TextParagraphProperties _lp; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: LineProperties.cs // // Description: Text line properties provider. // // History: // 04/25/2003 : grzegorz - moving from Avalon branch. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using System.Windows.Controls; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Media.TextFormatting; using MS.Internal.Documents; using MS.Internal.PtsHost; // TextParagraph namespace MS.Internal.Text { // --------------------------------------------------------------------- // Text line properties provider. // --------------------------------------------------------------------- internal class LineProperties : TextParagraphProperties { // ------------------------------------------------------------------ // // TextParagraphProperties Implementation // // ----------------------------------------------------------------- #region TextParagraphProperties Implementation ////// This property specifies whether the primary text advance /// direction shall be left-to-right, right-to-left, or top-to-bottom. /// public override FlowDirection FlowDirection { get { return _flowDirection; } } ////// This property describes how inline content of a block is aligned. /// public override TextAlignment TextAlignment { get { return IgnoreTextAlignment ? TextAlignment.Left : _textAlignment; } } ////// Paragraph's line height /// ////// TextFormatter does not do appropriate line height handling, so /// report always 0 as line height. /// Line height is handled by TextFormatter host. /// public override double LineHeight { get { if (LineStackingStrategy == LineStackingStrategy.BlockLineHeight && !Double.IsNaN(_lineHeight)) { return _lineHeight; } return 0.0; } } ////// Indicates the first line of the paragraph. /// public override bool FirstLineInParagraph { get { return false; } } ////// Paragraph's default run properties /// public override TextRunProperties DefaultTextRunProperties { get { return _defaultTextProperties; } } ////// Text decorations specified at the paragraph level. /// public override TextDecorationCollection TextDecorations { get { return _defaultTextProperties.TextDecorations; } } ////// This property controls whether or not text wraps when it reaches the flow edge /// of its containing block box /// public override TextWrapping TextWrapping { get { return _textWrapping; } } ////// This property specifies marker characteristics of the first line in paragraph /// public override TextMarkerProperties TextMarkerProperties { get { return _markerProperties; } } ////// Line indentation /// ////// Line indentation. Line indent by default is always 0. /// Use FirstLineProperties class to return real value of this property. /// public override double Indent { get { return 0.0; } } #endregion TextParagraphProperties Implementation ////// Constructor. /// internal LineProperties( DependencyObject element, DependencyObject contentHost, TextProperties defaultTextProperties, MarkerProperties markerProperties) : this(element, contentHost, defaultTextProperties, markerProperties, (TextAlignment)element.GetValue(Block.TextAlignmentProperty)) { } ////// Constructor. /// internal LineProperties( DependencyObject element, DependencyObject contentHost, TextProperties defaultTextProperties, MarkerProperties markerProperties, TextAlignment textAlignment) { _defaultTextProperties = defaultTextProperties; _markerProperties = (markerProperties != null) ? markerProperties.GetTextMarkerProperties(this) : null; _flowDirection = (FlowDirection)element.GetValue(Block.FlowDirectionProperty); _textAlignment = textAlignment; _lineHeight = (double)element.GetValue(Block.LineHeightProperty); _textIndent = (double)element.GetValue(Paragraph.TextIndentProperty); _lineStackingStrategy = (LineStackingStrategy)element.GetValue(Block.LineStackingStrategyProperty); _textWrapping = TextWrapping.Wrap; _textTrimming = TextTrimming.None; if (contentHost is TextBlock || contentHost is ITextBoxViewHost) { // NB: we intentially don't try to find the "PropertyOwner" of // a FlowDocument here. TextWrapping has a hard-coded // value SetValue'd when a FlowDocument is hosted by a TextBoxBase. _textWrapping = (TextWrapping)contentHost.GetValue(TextBlock.TextWrappingProperty); _textTrimming = (TextTrimming)contentHost.GetValue(TextBlock.TextTrimmingProperty); } else if (contentHost is FlowDocument) { _textWrapping = ((FlowDocument)contentHost).TextWrapping; } } ////// Calculate line advance for TextParagraphs - this method has special casing in case the LineHeight property on the Paragraph element /// needs to be respected /// /// /// TextParagraph that owns the line /// /// /// Dcp of the line /// /// /// Calculated height of the line /// internal double CalcLineAdvanceForTextParagraph(TextParagraph textParagraph, int dcp, double lineAdvance) { if (!DoubleUtil.IsNaN(_lineHeight)) { switch (LineStackingStrategy) { case LineStackingStrategy.BlockLineHeight: lineAdvance = _lineHeight; break; case LineStackingStrategy.MaxHeight: default: if (dcp == 0 && textParagraph.HasFiguresOrFloaters() && ((textParagraph.GetLastDcpAttachedObjectBeforeLine(0) + textParagraph.ParagraphStartCharacterPosition) == textParagraph.ParagraphEndCharacterPosition)) { // The Paragraph element contains only figures and floaters and has LineHeight set. In this case LineHeight // should be respected lineAdvance = _lineHeight; } else { lineAdvance = Math.Max(lineAdvance, _lineHeight); } break; // case LineStackingStrategy.InlineLineHeight: // // Inline uses the height of the line just processed. // break; // case LineStackingStrategy.GridHeight: // lineAdvance = (((TextDpi.ToTextDpi(lineAdvance) - 1) / TextDpi.ToTextDpi(_lineHeight)) + 1) * _lineHeight; // break; //} } } return lineAdvance; } ////// Calculate line advance from actual line height and the line stacking strategy. /// internal double CalcLineAdvance(double lineAdvance) { // We support MaxHeight and BlockLineHeight stacking strategies if (!DoubleUtil.IsNaN(_lineHeight)) { switch (LineStackingStrategy) { case LineStackingStrategy.BlockLineHeight: lineAdvance = _lineHeight; break; case LineStackingStrategy.MaxHeight: default: lineAdvance = Math.Max(lineAdvance, _lineHeight); break; // case LineStackingStrategy.InlineLineHeight: // // Inline uses the height of the line just processed. // break; // case LineStackingStrategy.GridHeight: // lineAdvance = (((TextDpi.ToTextDpi(lineAdvance) - 1) / TextDpi.ToTextDpi(_lineHeight)) + 1) * _lineHeight; // break; //} } } return lineAdvance; } ////// Raw TextAlignment, without considering IgnoreTextAlignment. /// internal TextAlignment TextAlignmentInternal { get { return _textAlignment; } } ////// Ignore text alignment? /// internal bool IgnoreTextAlignment { get { return _ignoreTextAlignment; } set { _ignoreTextAlignment = value; } } ////////// Line stacking strategy. ///// internal LineStackingStrategy LineStackingStrategy { get { return _lineStackingStrategy; } } ////// Text trimming. /// internal TextTrimming TextTrimming { get { return _textTrimming; } } ////// Does it have first line specific properties? /// internal bool HasFirstLineProperties { get { return (_markerProperties != null || !DoubleUtil.IsZero(_textIndent)); } } ////// Local cache for first line properties. /// internal TextParagraphProperties FirstLineProps { get { if (_firstLineProperties == null) { _firstLineProperties = new FirstLineProperties(this); } return _firstLineProperties; } } // ------------------------------------------------------------------ // Local cache for line properties with paragraph ellipsis. // ------------------------------------------------------------------ ////// Local cache for line properties with paragraph ellipsis. /// internal TextParagraphProperties GetParaEllipsisLineProps(bool firstLine) { return new ParaEllipsisLineProperties(firstLine ? FirstLineProps : this); } private TextRunProperties _defaultTextProperties; // Line's default text properties. private TextMarkerProperties _markerProperties; // Marker properties private FirstLineProperties _firstLineProperties; // Local cache for first line properties. private bool _ignoreTextAlignment; // Ignore horizontal alignment? private FlowDirection _flowDirection; private TextAlignment _textAlignment; private TextWrapping _textWrapping; private TextTrimming _textTrimming; private double _lineHeight; private double _textIndent; private LineStackingStrategy _lineStackingStrategy; // --------------------------------------------------------------------- // First text line properties provider. // ---------------------------------------------------------------------- private sealed class FirstLineProperties : TextParagraphProperties { // ----------------------------------------------------------------- // // TextParagraphProperties Implementation // // ----------------------------------------------------------------- #region TextParagraphProperties Implementation // ----------------------------------------------------------------- // Text flow direction (text advance + block advance direction). // ------------------------------------------------------------------ public override FlowDirection FlowDirection { get { return _lp.FlowDirection; } } // ----------------------------------------------------------------- // Alignment of the line's content. // ------------------------------------------------------------------ public override TextAlignment TextAlignment { get { return _lp.TextAlignment; } } // ------------------------------------------------------------------ // Line's height. // ----------------------------------------------------------------- public override double LineHeight { get { return _lp.LineHeight; } } // ------------------------------------------------------------------ // An instance of this class is always the first line in a paragraph. // ----------------------------------------------------------------- public override bool FirstLineInParagraph { get { return true; } } // ----------------------------------------------------------------- // Line's default text properties. // ----------------------------------------------------------------- public override TextRunProperties DefaultTextRunProperties { get { return _lp.DefaultTextRunProperties; } } // ------------------------------------------------------------------ // Line's text decorations (in addition to any run-level text decorations). // ----------------------------------------------------------------- public override TextDecorationCollection TextDecorations { get { return _lp.TextDecorations; } } // ------------------------------------------------------------------ // Text wrap control. // ------------------------------------------------------------------ public override TextWrapping TextWrapping { get { return _lp.TextWrapping; } } // ----------------------------------------------------------------- // Marker characteristics of the first line in paragraph. // ------------------------------------------------------------------ public override TextMarkerProperties TextMarkerProperties { get { return _lp.TextMarkerProperties; } } // ----------------------------------------------------------------- // Line indentation. // ----------------------------------------------------------------- public override double Indent { get { return _lp._textIndent; } } #endregion TextParagraphProperties Implementation // ----------------------------------------------------------------- // Constructor. // ------------------------------------------------------------------ internal FirstLineProperties(LineProperties lp) { _lp = lp; // properly set the local copy of hyphenator accordingly. Hyphenator = lp.Hyphenator; } // ----------------------------------------------------------------- // LineProperties object reference. // ------------------------------------------------------------------ private LineProperties _lp; } // ---------------------------------------------------------------------- // Line properties provider for line with paragraph ellipsis. // --------------------------------------------------------------------- private sealed class ParaEllipsisLineProperties : TextParagraphProperties { // ------------------------------------------------------------------ // // TextParagraphProperties Implementation // // ----------------------------------------------------------------- #region TextParagraphProperties Implementation // ----------------------------------------------------------------- // Text flow direction (text advance + block advance direction). // ----------------------------------------------------------------- public override FlowDirection FlowDirection { get { return _lp.FlowDirection; } } // ------------------------------------------------------------------ // Alignment of the line's content. // ----------------------------------------------------------------- public override TextAlignment TextAlignment { get { return _lp.TextAlignment; } } // ------------------------------------------------------------------ // Line's height. // ------------------------------------------------------------------ public override double LineHeight { get { return _lp.LineHeight; } } // ----------------------------------------------------------------- // First line in paragraph option. // ------------------------------------------------------------------ public override bool FirstLineInParagraph { get { return _lp.FirstLineInParagraph; } } // ----------------------------------------------------------------- // Always collapsible option. // ----------------------------------------------------------------- public override bool AlwaysCollapsible { get { return _lp.AlwaysCollapsible; } } // ----------------------------------------------------------------- // Line's default text properties. // ------------------------------------------------------------------ public override TextRunProperties DefaultTextRunProperties { get { return _lp.DefaultTextRunProperties; } } // ----------------------------------------------------------------- // Line's text decorations (in addition to any run-level text decorations). // ------------------------------------------------------------------ public override TextDecorationCollection TextDecorations { get { return _lp.TextDecorations; } } // ------------------------------------------------------------------ // Text wrap control. // If paragraph ellipsis are enabled, force this line not to wrap. // ----------------------------------------------------------------- public override TextWrapping TextWrapping { get { return TextWrapping.NoWrap; } } // ------------------------------------------------------------------ // Marker characteristics of the first line in paragraph. // ----------------------------------------------------------------- public override TextMarkerProperties TextMarkerProperties { get { return _lp.TextMarkerProperties; } } // ----------------------------------------------------------------- // Line indentation. // ----------------------------------------------------------------- public override double Indent { get { return _lp.Indent; } } #endregion TextParagraphProperties Implementation // ------------------------------------------------------------------ // Constructor. // ----------------------------------------------------------------- internal ParaEllipsisLineProperties(TextParagraphProperties lp) { _lp = lp; } // ------------------------------------------------------------------ // LineProperties object reference. // ------------------------------------------------------------------ private TextParagraphProperties _lp; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
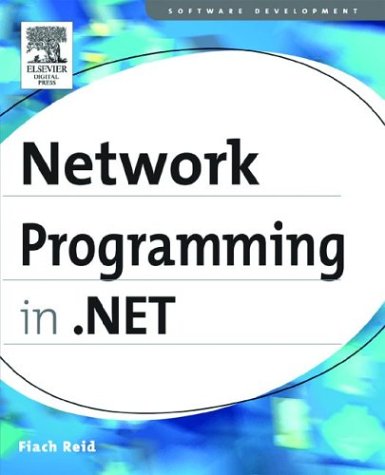
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartDescription.cs
- ObjectAnimationUsingKeyFrames.cs
- FlowPanelDesigner.cs
- PipeException.cs
- WinEventTracker.cs
- InputLanguageCollection.cs
- Rotation3DAnimation.cs
- SHA1Cng.cs
- DrawingDrawingContext.cs
- PasswordTextContainer.cs
- WebExceptionStatus.cs
- OleDbRowUpdatingEvent.cs
- Visual.cs
- StringAttributeCollection.cs
- BridgeDataReader.cs
- CodeThrowExceptionStatement.cs
- StackSpiller.cs
- SHA256Managed.cs
- InputLanguageProfileNotifySink.cs
- OutputCacheModule.cs
- TableRowsCollectionEditor.cs
- DataSvcMapFile.cs
- IdleTimeoutMonitor.cs
- _ProxyRegBlob.cs
- TransactionBridgeSection.cs
- XmlLinkedNode.cs
- ElementUtil.cs
- Keyboard.cs
- RuleDefinitions.cs
- ConfigDefinitionUpdates.cs
- CompiledAction.cs
- ToolStripLocationCancelEventArgs.cs
- CharEntityEncoderFallback.cs
- DoWorkEventArgs.cs
- PageFunction.cs
- FormClosedEvent.cs
- PointLightBase.cs
- DataSourceViewSchemaConverter.cs
- Operand.cs
- glyphs.cs
- MarkupExtensionParser.cs
- LateBoundBitmapDecoder.cs
- EmbeddedMailObject.cs
- Equal.cs
- InvalidateEvent.cs
- MetadataUtilsSmi.cs
- CalendarModeChangedEventArgs.cs
- DataView.cs
- ListCollectionView.cs
- ClientSettingsSection.cs
- SecUtil.cs
- ProfileEventArgs.cs
- RemotingAttributes.cs
- ObjectStorage.cs
- TextBoxAutoCompleteSourceConverter.cs
- SqlTrackingQuery.cs
- DesignerObjectListAdapter.cs
- DataListItem.cs
- QilFunction.cs
- ConfigurationException.cs
- XmlSchemaInclude.cs
- KeyedHashAlgorithm.cs
- ViewManager.cs
- TimeSpanSecondsConverter.cs
- NavigationCommands.cs
- Codec.cs
- XmlNamedNodeMap.cs
- SmiSettersStream.cs
- SQLInt16Storage.cs
- LinqToSqlWrapper.cs
- AppearanceEditorPart.cs
- Matrix3D.cs
- DataRowComparer.cs
- DataTemplateKey.cs
- InkPresenter.cs
- EdmError.cs
- CommonDialog.cs
- OleDbSchemaGuid.cs
- HierarchicalDataBoundControlAdapter.cs
- ParameterExpression.cs
- MD5CryptoServiceProvider.cs
- TargetPerspective.cs
- ObjectView.cs
- Pair.cs
- NetSectionGroup.cs
- XmlSchemaAppInfo.cs
- FixedPageAutomationPeer.cs
- PackageRelationship.cs
- PolicyStatement.cs
- LogStream.cs
- FontUnit.cs
- DocumentPageViewAutomationPeer.cs
- HtmlWindow.cs
- TextPattern.cs
- initElementDictionary.cs
- EnumValAlphaComparer.cs
- FileEnumerator.cs
- CommonGetThemePartSize.cs
- SoapTypeAttribute.cs
- ELinqQueryState.cs