Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Controls / GroupItem.cs / 1 / GroupItem.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: GroupItem object - root of the UI subtree generated for a CollectionViewGroup // // Specs: http://avalon/connecteddata/M5%20General%20Docs/Data%20Styling.mht // //--------------------------------------------------------------------------- using System; using System.Collections; namespace System.Windows.Controls { ////// A GroupItem appears as the root of the visual subtree generated for a CollectionViewGroup. /// public class GroupItem : ContentControl { static GroupItem() { DefaultStyleKeyProperty.OverrideMetadata(typeof(GroupItem), new FrameworkPropertyMetadata(typeof(GroupItem))); _dType = DependencyObjectType.FromSystemTypeInternal(typeof(GroupItem)); // GroupItems should not be focusable by default FocusableProperty.OverrideMetadata(typeof(GroupItem), new FrameworkPropertyMetadata(false)); } ////// Creates AutomationPeer ( protected override System.Windows.Automation.Peers.AutomationPeer OnCreateAutomationPeer() { return new System.Windows.Automation.Peers.GroupItemAutomationPeer(this); } ///) /// /// Gives a string representation of this object. /// ///internal override string GetPlainText() { System.Windows.Data.CollectionViewGroup cvg = Content as System.Windows.Data.CollectionViewGroup; if (cvg != null && cvg.Name != null) { return cvg.Name.ToString(); } return base.GetPlainText(); } //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- internal ItemContainerGenerator Generator { get { return _generator; } set { _generator = value; } } //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- internal void PrepareItemContainer(object item) { if (Generator == null) return; // user-declared GroupItem - ignore (bug 108423) ItemContainerGenerator generator = Generator.Parent; GroupStyle groupStyle = generator.GroupStyle; // apply the container style Style style = groupStyle.ContainerStyle; // no ContainerStyle set, try ContainerStyleSelector if (style == null) { if (groupStyle.ContainerStyleSelector != null) { style = groupStyle.ContainerStyleSelector.SelectStyle(item, this); } } // apply the style, if found if (style != null) { // verify style is appropriate before applying it if (!style.TargetType.IsInstanceOfType(this)) throw new InvalidOperationException(SR.Get(SRID.StyleForWrongType, style.TargetType.Name, this.GetType().Name)); this.Style = style; this.WriteInternalFlag2(InternalFlags2.IsStyleSetFromGenerator, true); } // forward the header template information if (!HasNonDefaultValue(ContentProperty)) this.Content = item; if (!HasNonDefaultValue(ContentTemplateProperty)) this.ContentTemplate = groupStyle.HeaderTemplate; if (!HasNonDefaultValue(ContentTemplateSelectorProperty)) this.ContentTemplateSelector = groupStyle.HeaderTemplateSelector; if (!HasNonDefaultValue(ContentStringFormatProperty)) this.ContentStringFormat = groupStyle.HeaderStringFormat; } internal void ClearContainerForItem(object item) { if (Generator == null) return; // user-declared GroupItem - ignore (bug 108423) ItemContainerGenerator generator = Generator.Parent; GroupStyle groupStyle = generator.GroupStyle; if (Object.Equals(this.Content, item)) ClearValue(ContentProperty); if (this.ContentTemplate == groupStyle.HeaderTemplate) ClearValue(ContentTemplateProperty); if (this.ContentTemplateSelector == groupStyle.HeaderTemplateSelector) ClearValue(ContentTemplateSelectorProperty); if (this.ContentStringFormat == groupStyle.HeaderStringFormat) ClearValue(ContentStringFormatProperty); Generator.Release(); } //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ ItemContainerGenerator _generator; #region DTypeThemeStyleKey // Returns the DependencyObjectType for the registered ThemeStyleKey's default // value. Controls will override this method to return approriate types. internal override DependencyObjectType DTypeThemeStyleKey { get { return _dType; } } private static DependencyObjectType _dType; #endregion DTypeThemeStyleKey } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: GroupItem object - root of the UI subtree generated for a CollectionViewGroup // // Specs: http://avalon/connecteddata/M5%20General%20Docs/Data%20Styling.mht // //--------------------------------------------------------------------------- using System; using System.Collections; namespace System.Windows.Controls { ////// A GroupItem appears as the root of the visual subtree generated for a CollectionViewGroup. /// public class GroupItem : ContentControl { static GroupItem() { DefaultStyleKeyProperty.OverrideMetadata(typeof(GroupItem), new FrameworkPropertyMetadata(typeof(GroupItem))); _dType = DependencyObjectType.FromSystemTypeInternal(typeof(GroupItem)); // GroupItems should not be focusable by default FocusableProperty.OverrideMetadata(typeof(GroupItem), new FrameworkPropertyMetadata(false)); } ////// Creates AutomationPeer ( protected override System.Windows.Automation.Peers.AutomationPeer OnCreateAutomationPeer() { return new System.Windows.Automation.Peers.GroupItemAutomationPeer(this); } ///) /// /// Gives a string representation of this object. /// ///internal override string GetPlainText() { System.Windows.Data.CollectionViewGroup cvg = Content as System.Windows.Data.CollectionViewGroup; if (cvg != null && cvg.Name != null) { return cvg.Name.ToString(); } return base.GetPlainText(); } //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- internal ItemContainerGenerator Generator { get { return _generator; } set { _generator = value; } } //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- internal void PrepareItemContainer(object item) { if (Generator == null) return; // user-declared GroupItem - ignore (bug 108423) ItemContainerGenerator generator = Generator.Parent; GroupStyle groupStyle = generator.GroupStyle; // apply the container style Style style = groupStyle.ContainerStyle; // no ContainerStyle set, try ContainerStyleSelector if (style == null) { if (groupStyle.ContainerStyleSelector != null) { style = groupStyle.ContainerStyleSelector.SelectStyle(item, this); } } // apply the style, if found if (style != null) { // verify style is appropriate before applying it if (!style.TargetType.IsInstanceOfType(this)) throw new InvalidOperationException(SR.Get(SRID.StyleForWrongType, style.TargetType.Name, this.GetType().Name)); this.Style = style; this.WriteInternalFlag2(InternalFlags2.IsStyleSetFromGenerator, true); } // forward the header template information if (!HasNonDefaultValue(ContentProperty)) this.Content = item; if (!HasNonDefaultValue(ContentTemplateProperty)) this.ContentTemplate = groupStyle.HeaderTemplate; if (!HasNonDefaultValue(ContentTemplateSelectorProperty)) this.ContentTemplateSelector = groupStyle.HeaderTemplateSelector; if (!HasNonDefaultValue(ContentStringFormatProperty)) this.ContentStringFormat = groupStyle.HeaderStringFormat; } internal void ClearContainerForItem(object item) { if (Generator == null) return; // user-declared GroupItem - ignore (bug 108423) ItemContainerGenerator generator = Generator.Parent; GroupStyle groupStyle = generator.GroupStyle; if (Object.Equals(this.Content, item)) ClearValue(ContentProperty); if (this.ContentTemplate == groupStyle.HeaderTemplate) ClearValue(ContentTemplateProperty); if (this.ContentTemplateSelector == groupStyle.HeaderTemplateSelector) ClearValue(ContentTemplateSelectorProperty); if (this.ContentStringFormat == groupStyle.HeaderStringFormat) ClearValue(ContentStringFormatProperty); Generator.Release(); } //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ ItemContainerGenerator _generator; #region DTypeThemeStyleKey // Returns the DependencyObjectType for the registered ThemeStyleKey's default // value. Controls will override this method to return approriate types. internal override DependencyObjectType DTypeThemeStyleKey { get { return _dType; } } private static DependencyObjectType _dType; #endregion DTypeThemeStyleKey } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
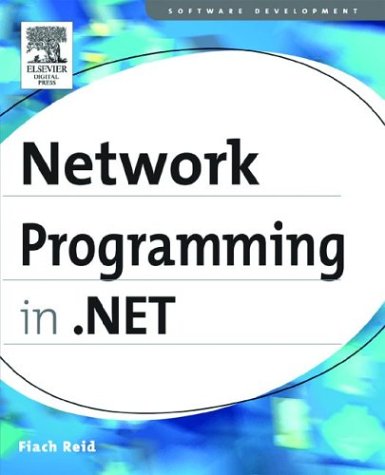
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MobileCapabilities.cs
- Parsers.cs
- DataTrigger.cs
- ApplicationManager.cs
- ScrollBarAutomationPeer.cs
- DynamicScriptObject.cs
- AssemblyBuilder.cs
- PlatformCulture.cs
- Byte.cs
- RtfToken.cs
- GeneralTransform3DTo2DTo3D.cs
- VScrollBar.cs
- _AutoWebProxyScriptWrapper.cs
- FileLogRecordEnumerator.cs
- EditorReuseAttribute.cs
- FilteredAttributeCollection.cs
- SynchronizingStream.cs
- PlatformNotSupportedException.cs
- Crc32.cs
- GridPattern.cs
- SqlInternalConnectionTds.cs
- Thread.cs
- ItemList.cs
- XmlC14NWriter.cs
- DataGridViewRowHeaderCell.cs
- NameValueConfigurationCollection.cs
- GridToolTip.cs
- FontUnit.cs
- DocumentXPathNavigator.cs
- SortAction.cs
- BindValidator.cs
- Serializer.cs
- FixedSOMContainer.cs
- ExtensionQuery.cs
- WindowPattern.cs
- DashStyles.cs
- ChangePassword.cs
- MbpInfo.cs
- ConnectionStringsExpressionBuilder.cs
- BufferedWebEventProvider.cs
- XmlObjectSerializerContext.cs
- QueryPageSettingsEventArgs.cs
- MessageQueueTransaction.cs
- ToolStripContentPanelDesigner.cs
- XmlSchemaComplexType.cs
- StringAttributeCollection.cs
- SQLDateTimeStorage.cs
- QueryCacheManager.cs
- IPHostEntry.cs
- WebControlAdapter.cs
- MenuDesigner.cs
- TraceContextEventArgs.cs
- HandleScope.cs
- IndentTextWriter.cs
- WindowsNonControl.cs
- SamlAuthorizationDecisionClaimResource.cs
- XmlSchemaCompilationSettings.cs
- ScriptResourceMapping.cs
- SynchronizedChannelCollection.cs
- TableSectionStyle.cs
- SamlConstants.cs
- MeasureData.cs
- SqlNode.cs
- QueryPageSettingsEventArgs.cs
- MultiAsyncResult.cs
- CompressedStack.cs
- XmlnsDictionary.cs
- IfAction.cs
- DelegatedStream.cs
- HandlerBase.cs
- SystemGatewayIPAddressInformation.cs
- XmlSchemaAppInfo.cs
- MetadataStore.cs
- SoapExtensionTypeElement.cs
- TextAdaptor.cs
- ReferenceEqualityComparer.cs
- TimerElapsedEvenArgs.cs
- TdsParserSessionPool.cs
- BaseContextMenu.cs
- CorrelationTokenTypeConvertor.cs
- ColorContextHelper.cs
- Utilities.cs
- ErrorFormatter.cs
- ProtocolsConfigurationHandler.cs
- FrugalList.cs
- SponsorHelper.cs
- WpfPayload.cs
- ZipIOCentralDirectoryFileHeader.cs
- GridItemCollection.cs
- DataTableReaderListener.cs
- BamlLocalizabilityResolver.cs
- ProcessProtocolHandler.cs
- CriticalExceptions.cs
- VectorAnimationUsingKeyFrames.cs
- SqlConnectionManager.cs
- ArrayListCollectionBase.cs
- WsdlBuildProvider.cs
- CompensatableSequenceActivity.cs
- ConnectionStringsExpressionBuilder.cs
- LogManagementAsyncResult.cs