Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / Configuration / BuildProvider.cs / 1 / BuildProvider.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.Globalization; using System.IO; using System.Text; using System.Web.Compilation; using System.Reflection; using System.Web.Hosting; using System.Web.UI; using System.CodeDom.Compiler; using System.Web.Util; using System.ComponentModel; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class BuildProvider : ConfigurationElement { private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propExtension = new ConfigurationProperty("extension", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired | ConfigurationPropertyOptions.IsKey); private static readonly ConfigurationProperty _propType = new ConfigurationProperty("type", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired); private Type _type; // AppliesTo value from the BuildProviderAppliesToAttribute private BuildProviderAppliesTo _appliesToInternal; static BuildProvider() { _properties = new ConfigurationPropertyCollection(); _properties.Add(_propExtension); _properties.Add(_propType); } public BuildProvider(String extension, String type) : this() { Extension = extension; Type = type; } internal BuildProvider() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } // this override is required because AppliesTo may be in any order in the // property string but it still and the default equals operator would consider // them different depending on order in the persisted string. public override bool Equals(object provider) { BuildProvider o = provider as BuildProvider; return (o != null && StringUtil.EqualsIgnoreCase(Extension, o.Extension) && Type == o.Type); } public override int GetHashCode() { return HashCodeCombiner.CombineHashCodes(Extension.ToLower(CultureInfo.InvariantCulture).GetHashCode(), Type.GetHashCode()); } [ConfigurationProperty("extension", IsRequired = true, IsKey = true, DefaultValue = "")] [StringValidator(MinLength = 1)] public string Extension { get { return (string)base[_propExtension]; } set { base[_propExtension] = value; } } [ConfigurationProperty("type", IsRequired = true, DefaultValue = "")] [StringValidator(MinLength = 1)] public string Type { get { return (string)base[_propType]; } set { base[_propType] = value; } } internal Type TypeInternal { get { if (_type == null) { lock (this) { if (_type == null) { _type = CompilationUtil.LoadTypeWithChecks(Type, typeof(System.Web.Compilation.BuildProvider), null, this, "type"); } } } return _type; } } internal BuildProviderAppliesTo AppliesToInternal { get { if (_appliesToInternal != 0) return _appliesToInternal; // Check whether the control builder's class exposes an AppliesTo attribute object[] attrs = TypeInternal.GetCustomAttributes( typeof(BuildProviderAppliesToAttribute), /*inherit*/ true); if ((attrs != null) && (attrs.Length > 0)) { Debug.Assert(attrs[0] is BuildProviderAppliesToAttribute); _appliesToInternal = ((BuildProviderAppliesToAttribute)attrs[0]).AppliesTo; } else { // Default to applying to All _appliesToInternal = BuildProviderAppliesTo.All; } return _appliesToInternal; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.Globalization; using System.IO; using System.Text; using System.Web.Compilation; using System.Reflection; using System.Web.Hosting; using System.Web.UI; using System.CodeDom.Compiler; using System.Web.Util; using System.ComponentModel; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class BuildProvider : ConfigurationElement { private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propExtension = new ConfigurationProperty("extension", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired | ConfigurationPropertyOptions.IsKey); private static readonly ConfigurationProperty _propType = new ConfigurationProperty("type", typeof(string), null, null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.IsRequired); private Type _type; // AppliesTo value from the BuildProviderAppliesToAttribute private BuildProviderAppliesTo _appliesToInternal; static BuildProvider() { _properties = new ConfigurationPropertyCollection(); _properties.Add(_propExtension); _properties.Add(_propType); } public BuildProvider(String extension, String type) : this() { Extension = extension; Type = type; } internal BuildProvider() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } // this override is required because AppliesTo may be in any order in the // property string but it still and the default equals operator would consider // them different depending on order in the persisted string. public override bool Equals(object provider) { BuildProvider o = provider as BuildProvider; return (o != null && StringUtil.EqualsIgnoreCase(Extension, o.Extension) && Type == o.Type); } public override int GetHashCode() { return HashCodeCombiner.CombineHashCodes(Extension.ToLower(CultureInfo.InvariantCulture).GetHashCode(), Type.GetHashCode()); } [ConfigurationProperty("extension", IsRequired = true, IsKey = true, DefaultValue = "")] [StringValidator(MinLength = 1)] public string Extension { get { return (string)base[_propExtension]; } set { base[_propExtension] = value; } } [ConfigurationProperty("type", IsRequired = true, DefaultValue = "")] [StringValidator(MinLength = 1)] public string Type { get { return (string)base[_propType]; } set { base[_propType] = value; } } internal Type TypeInternal { get { if (_type == null) { lock (this) { if (_type == null) { _type = CompilationUtil.LoadTypeWithChecks(Type, typeof(System.Web.Compilation.BuildProvider), null, this, "type"); } } } return _type; } } internal BuildProviderAppliesTo AppliesToInternal { get { if (_appliesToInternal != 0) return _appliesToInternal; // Check whether the control builder's class exposes an AppliesTo attribute object[] attrs = TypeInternal.GetCustomAttributes( typeof(BuildProviderAppliesToAttribute), /*inherit*/ true); if ((attrs != null) && (attrs.Length > 0)) { Debug.Assert(attrs[0] is BuildProviderAppliesToAttribute); _appliesToInternal = ((BuildProviderAppliesToAttribute)attrs[0]).AppliesTo; } else { // Default to applying to All _appliesToInternal = BuildProviderAppliesTo.All; } return _appliesToInternal; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
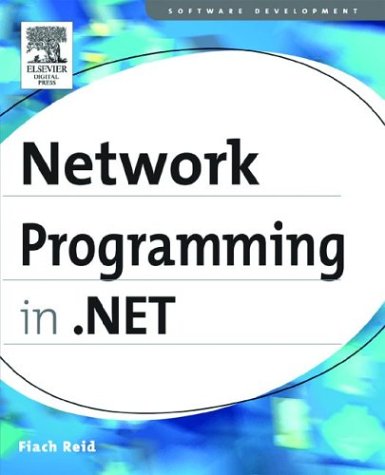
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DefaultTextStoreTextComposition.cs
- WebPartPersonalization.cs
- TableParagraph.cs
- IMembershipProvider.cs
- DSASignatureDeformatter.cs
- TCPListener.cs
- TrackPoint.cs
- DataGridColumnCollection.cs
- RouteParameter.cs
- HiddenField.cs
- Hex.cs
- DictionaryChange.cs
- Matrix.cs
- ColorAnimationBase.cs
- UserMapPath.cs
- PreviewPrintController.cs
- Point3DAnimationBase.cs
- Pkcs7Recipient.cs
- ContainerActivationHelper.cs
- BitmapCache.cs
- PropertyEmitterBase.cs
- ModuleElement.cs
- ConnectionPool.cs
- ParserStreamGeometryContext.cs
- GridEntryCollection.cs
- KeyedHashAlgorithm.cs
- TdsParserSessionPool.cs
- XPathAncestorIterator.cs
- DesignOnlyAttribute.cs
- DrawingDrawingContext.cs
- WebPartDisplayModeCancelEventArgs.cs
- CookieHandler.cs
- ControlParameter.cs
- KerberosSecurityTokenProvider.cs
- DataGridLinkButton.cs
- XsltLibrary.cs
- ParallelDesigner.cs
- TemplateXamlTreeBuilder.cs
- DayRenderEvent.cs
- IdentityHolder.cs
- Native.cs
- ContentOperations.cs
- PageParserFilter.cs
- DataGridGeneralPage.cs
- GridItemPattern.cs
- NamedElement.cs
- PageTheme.cs
- RepeatInfo.cs
- EntityTypeEmitter.cs
- ParameterElement.cs
- BitVector32.cs
- OdbcDataReader.cs
- ObjectDataSourceStatusEventArgs.cs
- XmlAttributeHolder.cs
- PaperSource.cs
- MailSettingsSection.cs
- ProfileSettings.cs
- CachingParameterInspector.cs
- DashStyle.cs
- WorkflowHostingResponseContext.cs
- ReadOnlyTernaryTree.cs
- ISSmlParser.cs
- NetTcpBindingCollectionElement.cs
- BeginStoryboard.cs
- EntityContainerEmitter.cs
- ControlPaint.cs
- DynamicArgumentDialog.cs
- FastEncoder.cs
- GeneralTransform3DTo2D.cs
- ImageMap.cs
- ColumnWidthChangedEvent.cs
- ActivityBuilderHelper.cs
- ConfigXmlWhitespace.cs
- XmlAttribute.cs
- ScrollContentPresenter.cs
- HandlerWithFactory.cs
- CellParagraph.cs
- StateDesigner.Helpers.cs
- UInt32Storage.cs
- InternalException.cs
- TcpConnectionPoolSettings.cs
- SmtpNegotiateAuthenticationModule.cs
- AssociationSet.cs
- DecimalAnimationUsingKeyFrames.cs
- OLEDB_Enum.cs
- AbsoluteQuery.cs
- ReaderContextStackData.cs
- DataSetMappper.cs
- DataGridViewRowPostPaintEventArgs.cs
- RuntimeConfig.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- DelegateSerializationHolder.cs
- ServiceBehaviorElementCollection.cs
- XmlILStorageConverter.cs
- CodeIdentifiers.cs
- SortedList.cs
- ErrorFormatterPage.cs
- Task.cs
- PackageDocument.cs
- XmlCodeExporter.cs