Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridViewRowPostPaintEventArgs.cs / 1 / DataGridViewRowPostPaintEventArgs.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.ComponentModel; using System.Diagnostics; ///public class DataGridViewRowPostPaintEventArgs : EventArgs { private DataGridView dataGridView; private Graphics graphics; private Rectangle clipBounds; private Rectangle rowBounds; private DataGridViewCellStyle inheritedRowStyle; private int rowIndex; private DataGridViewElementStates rowState; private string errorText; private bool isFirstDisplayedRow; private bool isLastVisibleRow; /// public DataGridViewRowPostPaintEventArgs(DataGridView dataGridView, Graphics graphics, Rectangle clipBounds, Rectangle rowBounds, int rowIndex, DataGridViewElementStates rowState, string errorText, DataGridViewCellStyle inheritedRowStyle, bool isFirstDisplayedRow, bool isLastVisibleRow) { if (dataGridView == null) { throw new ArgumentNullException("dataGridView"); } if (graphics == null) { throw new ArgumentNullException("graphics"); } if (inheritedRowStyle == null) { throw new ArgumentNullException("inheritedRowStyle"); } this.dataGridView = dataGridView; this.graphics = graphics; this.clipBounds = clipBounds; this.rowBounds = rowBounds; this.rowIndex = rowIndex; this.rowState = rowState; this.errorText = errorText; this.inheritedRowStyle = inheritedRowStyle; this.isFirstDisplayedRow = isFirstDisplayedRow; this.isLastVisibleRow = isLastVisibleRow; } internal DataGridViewRowPostPaintEventArgs(DataGridView dataGridView) { Debug.Assert(dataGridView != null); this.dataGridView = dataGridView; } /// public Rectangle ClipBounds { get { return this.clipBounds; } set { this.clipBounds = value; } } /// public string ErrorText { get { return this.errorText; } } /// public Graphics Graphics { get { return this.graphics; } } /// public DataGridViewCellStyle InheritedRowStyle { get { return this.inheritedRowStyle; } } /// public bool IsFirstDisplayedRow { get { return this.isFirstDisplayedRow; } } /// public bool IsLastVisibleRow { get { return this.isLastVisibleRow; } } /// public Rectangle RowBounds { get { return this.rowBounds; } } /// public int RowIndex { get { return this.rowIndex; } } /// public DataGridViewElementStates State { get { return this.rowState; } } /// public void DrawFocus(Rectangle bounds, bool cellsPaintSelectionBackground) { if (this.rowIndex < 0 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } this.dataGridView.Rows.SharedRow(rowIndex).DrawFocus(this.graphics, this.clipBounds, bounds, this.rowIndex, this.rowState, this.inheritedRowStyle, cellsPaintSelectionBackground); } /// public void PaintCells(Rectangle clipBounds, DataGridViewPaintParts paintParts) { if (this.rowIndex < 0 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } this.dataGridView.Rows.SharedRow(rowIndex).PaintCells(this.graphics, clipBounds, this.rowBounds, this.rowIndex, this.rowState, this.isFirstDisplayedRow, this.isLastVisibleRow, paintParts); } /// public void PaintCellsBackground(Rectangle clipBounds, bool cellsPaintSelectionBackground) { if (this.rowIndex < 0 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } DataGridViewPaintParts paintParts = DataGridViewPaintParts.Background | DataGridViewPaintParts.Border; if (cellsPaintSelectionBackground) { paintParts |= DataGridViewPaintParts.SelectionBackground; } this.dataGridView.Rows.SharedRow(rowIndex).PaintCells(this.graphics, clipBounds, this.rowBounds, this.rowIndex, this.rowState, this.isFirstDisplayedRow, this.isLastVisibleRow, paintParts); } /// public void PaintCellsContent(Rectangle clipBounds) { if (this.rowIndex < 0 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } this.dataGridView.Rows.SharedRow(rowIndex).PaintCells(this.graphics, clipBounds, this.rowBounds, this.rowIndex, this.rowState, this.isFirstDisplayedRow, this.isLastVisibleRow, DataGridViewPaintParts.ContentBackground | DataGridViewPaintParts.ContentForeground | DataGridViewPaintParts.ErrorIcon); } /// public void PaintHeader(bool paintSelectionBackground) { DataGridViewPaintParts paintParts = DataGridViewPaintParts.Background | DataGridViewPaintParts.Border | DataGridViewPaintParts.ContentBackground | DataGridViewPaintParts.ContentForeground | DataGridViewPaintParts.ErrorIcon; if (paintSelectionBackground) { paintParts |= DataGridViewPaintParts.SelectionBackground; } PaintHeader(paintParts); } /// public void PaintHeader(DataGridViewPaintParts paintParts) { if (this.rowIndex < 0 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } this.dataGridView.Rows.SharedRow(rowIndex).PaintHeader(this.graphics, this.clipBounds, this.rowBounds, this.rowIndex, this.rowState, this.isFirstDisplayedRow, this.isLastVisibleRow, paintParts); } internal void SetProperties(Graphics graphics, Rectangle clipBounds, Rectangle rowBounds, int rowIndex, DataGridViewElementStates rowState, string errorText, DataGridViewCellStyle inheritedRowStyle, bool isFirstDisplayedRow, bool isLastVisibleRow) { Debug.Assert(graphics != null); Debug.Assert(inheritedRowStyle != null); this.graphics = graphics; this.clipBounds = clipBounds; this.rowBounds = rowBounds; this.rowIndex = rowIndex; this.rowState = rowState; this.errorText = errorText; this.inheritedRowStyle = inheritedRowStyle; this.isFirstDisplayedRow = isFirstDisplayedRow; this.isLastVisibleRow = isLastVisibleRow; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.ComponentModel; using System.Diagnostics; ///public class DataGridViewRowPostPaintEventArgs : EventArgs { private DataGridView dataGridView; private Graphics graphics; private Rectangle clipBounds; private Rectangle rowBounds; private DataGridViewCellStyle inheritedRowStyle; private int rowIndex; private DataGridViewElementStates rowState; private string errorText; private bool isFirstDisplayedRow; private bool isLastVisibleRow; /// public DataGridViewRowPostPaintEventArgs(DataGridView dataGridView, Graphics graphics, Rectangle clipBounds, Rectangle rowBounds, int rowIndex, DataGridViewElementStates rowState, string errorText, DataGridViewCellStyle inheritedRowStyle, bool isFirstDisplayedRow, bool isLastVisibleRow) { if (dataGridView == null) { throw new ArgumentNullException("dataGridView"); } if (graphics == null) { throw new ArgumentNullException("graphics"); } if (inheritedRowStyle == null) { throw new ArgumentNullException("inheritedRowStyle"); } this.dataGridView = dataGridView; this.graphics = graphics; this.clipBounds = clipBounds; this.rowBounds = rowBounds; this.rowIndex = rowIndex; this.rowState = rowState; this.errorText = errorText; this.inheritedRowStyle = inheritedRowStyle; this.isFirstDisplayedRow = isFirstDisplayedRow; this.isLastVisibleRow = isLastVisibleRow; } internal DataGridViewRowPostPaintEventArgs(DataGridView dataGridView) { Debug.Assert(dataGridView != null); this.dataGridView = dataGridView; } /// public Rectangle ClipBounds { get { return this.clipBounds; } set { this.clipBounds = value; } } /// public string ErrorText { get { return this.errorText; } } /// public Graphics Graphics { get { return this.graphics; } } /// public DataGridViewCellStyle InheritedRowStyle { get { return this.inheritedRowStyle; } } /// public bool IsFirstDisplayedRow { get { return this.isFirstDisplayedRow; } } /// public bool IsLastVisibleRow { get { return this.isLastVisibleRow; } } /// public Rectangle RowBounds { get { return this.rowBounds; } } /// public int RowIndex { get { return this.rowIndex; } } /// public DataGridViewElementStates State { get { return this.rowState; } } /// public void DrawFocus(Rectangle bounds, bool cellsPaintSelectionBackground) { if (this.rowIndex < 0 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } this.dataGridView.Rows.SharedRow(rowIndex).DrawFocus(this.graphics, this.clipBounds, bounds, this.rowIndex, this.rowState, this.inheritedRowStyle, cellsPaintSelectionBackground); } /// public void PaintCells(Rectangle clipBounds, DataGridViewPaintParts paintParts) { if (this.rowIndex < 0 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } this.dataGridView.Rows.SharedRow(rowIndex).PaintCells(this.graphics, clipBounds, this.rowBounds, this.rowIndex, this.rowState, this.isFirstDisplayedRow, this.isLastVisibleRow, paintParts); } /// public void PaintCellsBackground(Rectangle clipBounds, bool cellsPaintSelectionBackground) { if (this.rowIndex < 0 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } DataGridViewPaintParts paintParts = DataGridViewPaintParts.Background | DataGridViewPaintParts.Border; if (cellsPaintSelectionBackground) { paintParts |= DataGridViewPaintParts.SelectionBackground; } this.dataGridView.Rows.SharedRow(rowIndex).PaintCells(this.graphics, clipBounds, this.rowBounds, this.rowIndex, this.rowState, this.isFirstDisplayedRow, this.isLastVisibleRow, paintParts); } /// public void PaintCellsContent(Rectangle clipBounds) { if (this.rowIndex < 0 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } this.dataGridView.Rows.SharedRow(rowIndex).PaintCells(this.graphics, clipBounds, this.rowBounds, this.rowIndex, this.rowState, this.isFirstDisplayedRow, this.isLastVisibleRow, DataGridViewPaintParts.ContentBackground | DataGridViewPaintParts.ContentForeground | DataGridViewPaintParts.ErrorIcon); } /// public void PaintHeader(bool paintSelectionBackground) { DataGridViewPaintParts paintParts = DataGridViewPaintParts.Background | DataGridViewPaintParts.Border | DataGridViewPaintParts.ContentBackground | DataGridViewPaintParts.ContentForeground | DataGridViewPaintParts.ErrorIcon; if (paintSelectionBackground) { paintParts |= DataGridViewPaintParts.SelectionBackground; } PaintHeader(paintParts); } /// public void PaintHeader(DataGridViewPaintParts paintParts) { if (this.rowIndex < 0 || this.rowIndex >= this.dataGridView.Rows.Count) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewElementPaintingEventArgs_RowIndexOutOfRange)); } this.dataGridView.Rows.SharedRow(rowIndex).PaintHeader(this.graphics, this.clipBounds, this.rowBounds, this.rowIndex, this.rowState, this.isFirstDisplayedRow, this.isLastVisibleRow, paintParts); } internal void SetProperties(Graphics graphics, Rectangle clipBounds, Rectangle rowBounds, int rowIndex, DataGridViewElementStates rowState, string errorText, DataGridViewCellStyle inheritedRowStyle, bool isFirstDisplayedRow, bool isLastVisibleRow) { Debug.Assert(graphics != null); Debug.Assert(inheritedRowStyle != null); this.graphics = graphics; this.clipBounds = clipBounds; this.rowBounds = rowBounds; this.rowIndex = rowIndex; this.rowState = rowState; this.errorText = errorText; this.inheritedRowStyle = inheritedRowStyle; this.isFirstDisplayedRow = isFirstDisplayedRow; this.isLastVisibleRow = isLastVisibleRow; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
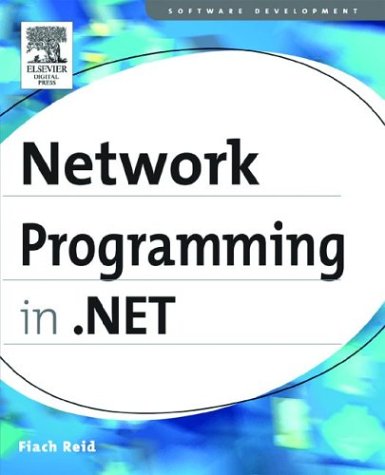
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- X509WindowsSecurityToken.cs
- WmpBitmapDecoder.cs
- CatalogPartCollection.cs
- TemplatePartAttribute.cs
- CustomPopupPlacement.cs
- RsaSecurityToken.cs
- ModulesEntry.cs
- CatalogZone.cs
- SchemaTableOptionalColumn.cs
- DnsPermission.cs
- SendKeys.cs
- TemplatePropertyEntry.cs
- MasterPageBuildProvider.cs
- FunctionUpdateCommand.cs
- GridViewCommandEventArgs.cs
- ValidationSummaryDesigner.cs
- HttpCapabilitiesBase.cs
- SpnegoTokenProvider.cs
- FormatVersion.cs
- UInt64.cs
- ModuleElement.cs
- WindowShowOrOpenTracker.cs
- SQLCharsStorage.cs
- UrlAuthFailedErrorFormatter.cs
- CounterCreationDataCollection.cs
- RuleCache.cs
- RuleInfoComparer.cs
- Icon.cs
- Identifier.cs
- CompilationRelaxations.cs
- XmlStreamNodeWriter.cs
- CanonicalFontFamilyReference.cs
- MdiWindowListItemConverter.cs
- SettingsProperty.cs
- MatrixAnimationBase.cs
- SoapParser.cs
- KnownTypesProvider.cs
- UpdateCommand.cs
- PathGeometry.cs
- ChangePasswordAutoFormat.cs
- PolyLineSegment.cs
- ActiveXContainer.cs
- InvokePattern.cs
- DbDataSourceEnumerator.cs
- ReflectEventDescriptor.cs
- AutoScrollExpandMessageFilter.cs
- ProgressBarHighlightConverter.cs
- SQLInt32Storage.cs
- LogAppendAsyncResult.cs
- ChannelSettingsElement.cs
- DynamicAttribute.cs
- HitTestWithPointDrawingContextWalker.cs
- FullTextState.cs
- ColorContextHelper.cs
- RMPermissions.cs
- DataRelation.cs
- CodeSpit.cs
- XmlObjectSerializerReadContextComplex.cs
- TdsRecordBufferSetter.cs
- SmtpCommands.cs
- ConfigurationStrings.cs
- X509WindowsSecurityToken.cs
- UserInitiatedNavigationPermission.cs
- PhysicalAddress.cs
- ClientConfigurationHost.cs
- TTSEvent.cs
- DataChangedEventManager.cs
- IndicFontClient.cs
- MouseEvent.cs
- DPCustomTypeDescriptor.cs
- ContextMenuStrip.cs
- WebServiceClientProxyGenerator.cs
- StyleBamlRecordReader.cs
- StyleTypedPropertyAttribute.cs
- ArraySet.cs
- XmlObjectSerializerReadContext.cs
- UrlPath.cs
- DataBoundControlHelper.cs
- CaseInsensitiveHashCodeProvider.cs
- Vector3DCollectionConverter.cs
- StandardToolWindows.cs
- PermissionSetTriple.cs
- PropertyBuilder.cs
- RemotingAttributes.cs
- CounterNameConverter.cs
- UnsafeNativeMethodsTablet.cs
- ObjectTypeMapping.cs
- PhonemeEventArgs.cs
- CursorConverter.cs
- SettingsSavedEventArgs.cs
- HttpApplication.cs
- XNodeValidator.cs
- WebPartDesigner.cs
- EmptyEnumerator.cs
- SystemResourceKey.cs
- XmlAttributeOverrides.cs
- Vector3DCollectionValueSerializer.cs
- SessionStateContainer.cs
- UserNamePasswordValidator.cs
- ImpersonationContext.cs