Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Animation / Generated / CharAnimationBase.cs / 1 / CharAnimationBase.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- // Allow use of presharp: #pragma warning suppress#pragma warning disable 1634, 1691 using MS.Internal; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; using MS.Internal.PresentationCore; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Animation { /// /// /// public abstract class CharAnimationBase : AnimationTimeline { #region Constructors ////// Creates a new CharAnimationBase. /// protected CharAnimationBase() : base() { } #endregion #region Freezable ////// Creates a copy of this CharAnimationBase /// ///The copy public new CharAnimationBase Clone() { return (CharAnimationBase)base.Clone(); } #endregion #region IAnimation ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public override sealed object GetCurrentValue(object defaultOriginValue, object defaultDestinationValue, AnimationClock animationClock) { // Verify that object arguments are non-null since we are a value type if (defaultOriginValue == null) { throw new ArgumentNullException("defaultOriginValue"); } if (defaultDestinationValue == null) { throw new ArgumentNullException("defaultDestinationValue"); } return GetCurrentValue((Char)defaultOriginValue, (Char)defaultDestinationValue, animationClock); } ////// Returns the type of the target property /// public override sealed Type TargetPropertyType { get { ReadPreamble(); return typeof(Char); } } #endregion #region Methods ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public Char GetCurrentValue(Char defaultOriginValue, Char defaultDestinationValue, AnimationClock animationClock) { ReadPreamble(); if (animationClock == null) { throw new ArgumentNullException("animationClock"); } // We check for null above but presharp doesn't notice so we suppress the // warning here. #pragma warning suppress 6506 if (animationClock.CurrentState == ClockState.Stopped) { return defaultDestinationValue; } /* if (!AnimatedTypeHelpers.IsValidAnimationValueChar(defaultDestinationValue)) { throw new ArgumentException( SR.Get( SRID.Animation_InvalidBaseValue, defaultDestinationValue, defaultDestinationValue.GetType(), GetType()), "defaultDestinationValue"); } */ return GetCurrentValueCore(defaultOriginValue, defaultDestinationValue, animationClock); } ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// protected abstract Char GetCurrentValueCore(Char defaultOriginValue, Char defaultDestinationValue, AnimationClock animationClock); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- // Allow use of presharp: #pragma warning suppress#pragma warning disable 1634, 1691 using MS.Internal; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; using MS.Internal.PresentationCore; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Animation { /// /// /// public abstract class CharAnimationBase : AnimationTimeline { #region Constructors ////// Creates a new CharAnimationBase. /// protected CharAnimationBase() : base() { } #endregion #region Freezable ////// Creates a copy of this CharAnimationBase /// ///The copy public new CharAnimationBase Clone() { return (CharAnimationBase)base.Clone(); } #endregion #region IAnimation ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public override sealed object GetCurrentValue(object defaultOriginValue, object defaultDestinationValue, AnimationClock animationClock) { // Verify that object arguments are non-null since we are a value type if (defaultOriginValue == null) { throw new ArgumentNullException("defaultOriginValue"); } if (defaultDestinationValue == null) { throw new ArgumentNullException("defaultDestinationValue"); } return GetCurrentValue((Char)defaultOriginValue, (Char)defaultDestinationValue, animationClock); } ////// Returns the type of the target property /// public override sealed Type TargetPropertyType { get { ReadPreamble(); return typeof(Char); } } #endregion #region Methods ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public Char GetCurrentValue(Char defaultOriginValue, Char defaultDestinationValue, AnimationClock animationClock) { ReadPreamble(); if (animationClock == null) { throw new ArgumentNullException("animationClock"); } // We check for null above but presharp doesn't notice so we suppress the // warning here. #pragma warning suppress 6506 if (animationClock.CurrentState == ClockState.Stopped) { return defaultDestinationValue; } /* if (!AnimatedTypeHelpers.IsValidAnimationValueChar(defaultDestinationValue)) { throw new ArgumentException( SR.Get( SRID.Animation_InvalidBaseValue, defaultDestinationValue, defaultDestinationValue.GetType(), GetType()), "defaultDestinationValue"); } */ return GetCurrentValueCore(defaultOriginValue, defaultDestinationValue, animationClock); } ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// protected abstract Char GetCurrentValueCore(Char defaultOriginValue, Char defaultDestinationValue, AnimationClock animationClock); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
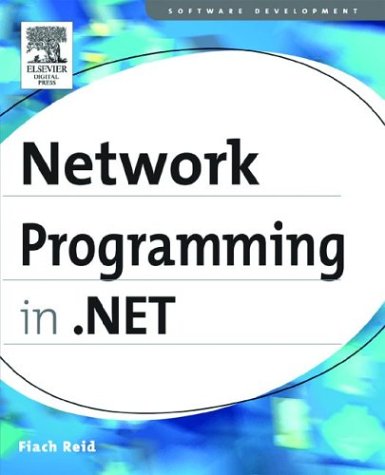
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _ProxyChain.cs
- DoubleLinkListEnumerator.cs
- ResourceAssociationSetEnd.cs
- DataControlLinkButton.cs
- Vector.cs
- PreviewKeyDownEventArgs.cs
- XXXOnTypeBuilderInstantiation.cs
- FixedPageStructure.cs
- WebServiceAttribute.cs
- EntityDataSourceDesignerHelper.cs
- TraceListener.cs
- BaseValidator.cs
- RuntimeHandles.cs
- ListItemParagraph.cs
- MdiWindowListItemConverter.cs
- MachineKey.cs
- LocationUpdates.cs
- MeasurementDCInfo.cs
- Wildcard.cs
- DesignConnectionCollection.cs
- PersistenceTask.cs
- ExpanderAutomationPeer.cs
- AvTraceFormat.cs
- RenderTargetBitmap.cs
- EditorZone.cs
- PointAnimationBase.cs
- XPathSingletonIterator.cs
- SchemaEntity.cs
- CommonProperties.cs
- DataContractJsonSerializer.cs
- GregorianCalendar.cs
- FaultDesigner.cs
- PreviewPageInfo.cs
- BatchServiceHost.cs
- StrokeCollection2.cs
- Logging.cs
- RepeaterItemEventArgs.cs
- FilteredSchemaElementLookUpTable.cs
- PhysicalFontFamily.cs
- DataGrid.cs
- ControlBuilder.cs
- ObjectRef.cs
- AliasGenerator.cs
- _TLSstream.cs
- PerfCounterSection.cs
- ControlBuilderAttribute.cs
- AnnotationService.cs
- PasswordBoxAutomationPeer.cs
- TaskHelper.cs
- SyndicationSerializer.cs
- DeviceContext.cs
- StateChangeEvent.cs
- PagerSettings.cs
- SettingsAttributes.cs
- SecurityAppliedMessage.cs
- XamlReaderHelper.cs
- EncryptedPackageFilter.cs
- ReadOnlyDataSourceView.cs
- DataGridAddNewRow.cs
- MediaSystem.cs
- CodeSubDirectory.cs
- ScriptingProfileServiceSection.cs
- SortedDictionary.cs
- PointValueSerializer.cs
- Pair.cs
- DataGridViewControlCollection.cs
- ConnectivityStatus.cs
- XmlSchemaCompilationSettings.cs
- Automation.cs
- TextDecorations.cs
- FreezableDefaultValueFactory.cs
- ProfileBuildProvider.cs
- PageFunction.cs
- SamlSecurityToken.cs
- DtrList.cs
- ColumnPropertiesGroup.cs
- FileRecordSequence.cs
- PersonalizationStateQuery.cs
- DBParameter.cs
- SplineKeyFrames.cs
- SqlRetyper.cs
- Hash.cs
- EdmEntityTypeAttribute.cs
- OdbcInfoMessageEvent.cs
- SafeNativeMethods.cs
- MenuTracker.cs
- BindingManagerDataErrorEventArgs.cs
- BuildResultCache.cs
- followingsibling.cs
- EntityCommandExecutionException.cs
- MouseEvent.cs
- AddInProcess.cs
- UrlRoutingHandler.cs
- BaseValidator.cs
- QuotedStringWriteStateInfo.cs
- DataIdProcessor.cs
- SelectingProviderEventArgs.cs
- XPathSingletonIterator.cs
- Menu.cs
- XmlSchemaElement.cs