Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / Security / WindowsAuthenticationModule.cs / 3 / WindowsAuthenticationModule.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * WindowsAuthenticationModule class * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web.Security { using System.Web; using System.Web.Configuration; using System.Security.Principal; using System.Security.Permissions; using System.Globalization; using System.Web.Management; using System.Web.Util; using System.Web.Hosting; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class WindowsAuthenticationModule : IHttpModule { private WindowsAuthenticationEventHandler _eventHandler; private static bool _fAuthChecked; private static bool _fAuthRequired; private static WindowsIdentity _anonymousIdentity; private static WindowsPrincipal _anonymousPrincipal; ////// Allows ASP.NET applications to use Windows/IIS authentication. /// ////// [SecurityPermission(SecurityAction.Demand, Unrestricted=true)] public WindowsAuthenticationModule() { } ////// Initializes a new instance of the ////// class. /// /// This is a global.asax event that must be /// named WindowsAuthenticate_OnAuthenticate event. It's used primarily to attach a /// custom IPrincipal object to the context. /// public event WindowsAuthenticationEventHandler Authenticate { add { _eventHandler += value; } remove { _eventHandler -= value; } } ////// public void Dispose() { } ///[To be supplied.] ////// public void Init(HttpApplication app) { app.AuthenticateRequest += new EventHandler(this.OnEnter); } //////////////////////////////////////////////////////////// // OnAuthenticate: Custom Authentication modules can override // this method to create a custom IPrincipal object from // a WindowsIdentity ///[To be supplied.] ////// Calls the /// WindowsAuthentication_OnAuthenticate handler if one exists. /// void OnAuthenticate(WindowsAuthenticationEventArgs e) { //////////////////////////////////////////////////////////// // If there are event handlers, invoke the handlers if (_eventHandler != null) _eventHandler(this, e); if (e.Context.User == null) { if (e.User != null) e.Context.User = e.User; else if (e.Identity == _anonymousIdentity) e.Context.SetPrincipalNoDemand(_anonymousPrincipal, false /*needToSetNativePrincipal*/); else e.Context.SetPrincipalNoDemand(new WindowsPrincipal(e.Identity), false /*needToSetNativePrincipal*/); } } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// // Methods for internal implementation ////// /// void OnEnter(Object source, EventArgs eventArgs) { if (!IsEnabled) return; HttpApplication app = (HttpApplication)source; HttpContext context = app.Context;; WindowsIdentity identity = null; ////////////////////////////////////////////////////////////////// // Step 2: Create a Windows Identity from the credentials from IIS if (HttpRuntime.UseIntegratedPipeline) { // The native WindowsAuthenticationModule sets the user principal in IIS7WorkerRequest.SynchronizeVariables. // The managed WindowsAuthenticationModule provides backward compatibility by rasing the OnAuthenticate event. WindowsPrincipal user = context.User as WindowsPrincipal; if (user != null) { // identity will be null if this is not a WindowsIdentity identity = user.Identity as WindowsIdentity; // clear Context.User for backward compatibility (it will be set in OnAuthenticate) context.SetPrincipalNoDemand(null, false /*needToSetNativePrincipal*/); } } else { String strLogonUser = context.WorkerRequest.GetServerVariable("LOGON_USER"); String strAuthType = context.WorkerRequest.GetServerVariable("AUTH_TYPE"); if (strLogonUser == null) { strLogonUser = String.Empty; } if (strAuthType == null) { strAuthType = String.Empty; } if (strLogonUser.Length == 0 && (strAuthType.Length == 0 || StringUtil.EqualsIgnoreCase(strAuthType, "basic"))) { //////////////////////////////////////////////////////// // Step 2a: Use the anonymous identity identity = _anonymousIdentity; } else { identity = new WindowsIdentity( context.WorkerRequest.GetUserToken(), strAuthType, WindowsAccountType.Normal, true); } } /////////////////////////////////////////////////////////////////////////////////// // Step 3: Call OnAuthenticate to create IPrincipal for this request. if (identity != null) { OnAuthenticate( new WindowsAuthenticationEventArgs(identity, context) ); } } internal static IPrincipal AnonymousPrincipal { get { return _anonymousPrincipal; } } internal static bool IsEnabled { get { if (!_fAuthChecked) { AuthenticationSection settings = RuntimeConfig.GetAppConfig().Authentication; settings.ValidateAuthenticationMode(); _fAuthRequired = (settings.Mode == AuthenticationMode.Windows); if (_fAuthRequired) { _anonymousIdentity = WindowsIdentity.GetAnonymous(); _anonymousPrincipal = new WindowsPrincipal(_anonymousIdentity); } _fAuthChecked = true; } return _fAuthRequired; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * WindowsAuthenticationModule class * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web.Security { using System.Web; using System.Web.Configuration; using System.Security.Principal; using System.Security.Permissions; using System.Globalization; using System.Web.Management; using System.Web.Util; using System.Web.Hosting; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class WindowsAuthenticationModule : IHttpModule { private WindowsAuthenticationEventHandler _eventHandler; private static bool _fAuthChecked; private static bool _fAuthRequired; private static WindowsIdentity _anonymousIdentity; private static WindowsPrincipal _anonymousPrincipal; ////// Allows ASP.NET applications to use Windows/IIS authentication. /// ////// [SecurityPermission(SecurityAction.Demand, Unrestricted=true)] public WindowsAuthenticationModule() { } ////// Initializes a new instance of the ////// class. /// /// This is a global.asax event that must be /// named WindowsAuthenticate_OnAuthenticate event. It's used primarily to attach a /// custom IPrincipal object to the context. /// public event WindowsAuthenticationEventHandler Authenticate { add { _eventHandler += value; } remove { _eventHandler -= value; } } ////// public void Dispose() { } ///[To be supplied.] ////// public void Init(HttpApplication app) { app.AuthenticateRequest += new EventHandler(this.OnEnter); } //////////////////////////////////////////////////////////// // OnAuthenticate: Custom Authentication modules can override // this method to create a custom IPrincipal object from // a WindowsIdentity ///[To be supplied.] ////// Calls the /// WindowsAuthentication_OnAuthenticate handler if one exists. /// void OnAuthenticate(WindowsAuthenticationEventArgs e) { //////////////////////////////////////////////////////////// // If there are event handlers, invoke the handlers if (_eventHandler != null) _eventHandler(this, e); if (e.Context.User == null) { if (e.User != null) e.Context.User = e.User; else if (e.Identity == _anonymousIdentity) e.Context.SetPrincipalNoDemand(_anonymousPrincipal, false /*needToSetNativePrincipal*/); else e.Context.SetPrincipalNoDemand(new WindowsPrincipal(e.Identity), false /*needToSetNativePrincipal*/); } } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// // Methods for internal implementation ////// /// void OnEnter(Object source, EventArgs eventArgs) { if (!IsEnabled) return; HttpApplication app = (HttpApplication)source; HttpContext context = app.Context;; WindowsIdentity identity = null; ////////////////////////////////////////////////////////////////// // Step 2: Create a Windows Identity from the credentials from IIS if (HttpRuntime.UseIntegratedPipeline) { // The native WindowsAuthenticationModule sets the user principal in IIS7WorkerRequest.SynchronizeVariables. // The managed WindowsAuthenticationModule provides backward compatibility by rasing the OnAuthenticate event. WindowsPrincipal user = context.User as WindowsPrincipal; if (user != null) { // identity will be null if this is not a WindowsIdentity identity = user.Identity as WindowsIdentity; // clear Context.User for backward compatibility (it will be set in OnAuthenticate) context.SetPrincipalNoDemand(null, false /*needToSetNativePrincipal*/); } } else { String strLogonUser = context.WorkerRequest.GetServerVariable("LOGON_USER"); String strAuthType = context.WorkerRequest.GetServerVariable("AUTH_TYPE"); if (strLogonUser == null) { strLogonUser = String.Empty; } if (strAuthType == null) { strAuthType = String.Empty; } if (strLogonUser.Length == 0 && (strAuthType.Length == 0 || StringUtil.EqualsIgnoreCase(strAuthType, "basic"))) { //////////////////////////////////////////////////////// // Step 2a: Use the anonymous identity identity = _anonymousIdentity; } else { identity = new WindowsIdentity( context.WorkerRequest.GetUserToken(), strAuthType, WindowsAccountType.Normal, true); } } /////////////////////////////////////////////////////////////////////////////////// // Step 3: Call OnAuthenticate to create IPrincipal for this request. if (identity != null) { OnAuthenticate( new WindowsAuthenticationEventArgs(identity, context) ); } } internal static IPrincipal AnonymousPrincipal { get { return _anonymousPrincipal; } } internal static bool IsEnabled { get { if (!_fAuthChecked) { AuthenticationSection settings = RuntimeConfig.GetAppConfig().Authentication; settings.ValidateAuthenticationMode(); _fAuthRequired = (settings.Mode == AuthenticationMode.Windows); if (_fAuthRequired) { _anonymousIdentity = WindowsIdentity.GetAnonymous(); _anonymousPrincipal = new WindowsPrincipal(_anonymousIdentity); } _fAuthChecked = true; } return _fAuthRequired; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
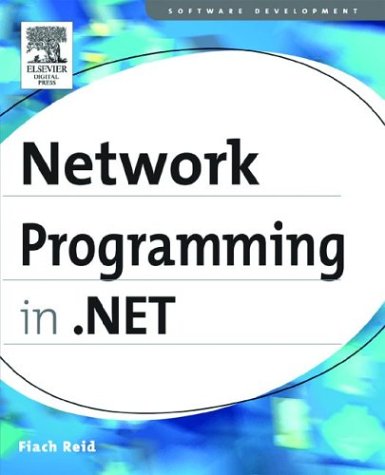
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ComponentEditorPage.cs
- CacheSection.cs
- SurrogateChar.cs
- PaintEvent.cs
- ModelFunctionTypeElement.cs
- PerformanceCounterPermissionEntry.cs
- SiteMap.cs
- WeakEventTable.cs
- ToolboxItemAttribute.cs
- EpmContentSerializerBase.cs
- EntityProviderServices.cs
- TableTextElementCollectionInternal.cs
- PenContext.cs
- EnumType.cs
- FixedStringLookup.cs
- DesignerCalendarAdapter.cs
- DataViewListener.cs
- Compiler.cs
- _NetworkingPerfCounters.cs
- QilName.cs
- safemediahandle.cs
- DataGridViewCheckBoxCell.cs
- ExecutionContext.cs
- QilStrConcatenator.cs
- XmlIgnoreAttribute.cs
- SecurityContextCookieSerializer.cs
- TextSerializer.cs
- UIElementIsland.cs
- PageStatePersister.cs
- LinqDataSourceUpdateEventArgs.cs
- CardSpaceShim.cs
- TagMapCollection.cs
- InvokeDelegate.cs
- CodeSnippetExpression.cs
- EtwTrackingBehavior.cs
- SecurityTokenRequirement.cs
- AssociationTypeEmitter.cs
- EntryPointNotFoundException.cs
- AuthenticationManager.cs
- ConstructorExpr.cs
- ErrorFormatter.cs
- MimeTextImporter.cs
- ConditionalAttribute.cs
- ChunkedMemoryStream.cs
- DBConnection.cs
- BufferBuilder.cs
- HttpVersion.cs
- HttpModulesSection.cs
- WindowsFont.cs
- LeftCellWrapper.cs
- MonthCalendar.cs
- TableCellAutomationPeer.cs
- CalendarTable.cs
- DataBinding.cs
- LogReservationCollection.cs
- ModelTreeEnumerator.cs
- DoubleLink.cs
- OutOfProcStateClientManager.cs
- ButtonColumn.cs
- ApplicationTrust.cs
- AtomMaterializerLog.cs
- SafeHandles.cs
- ToolStripGripRenderEventArgs.cs
- BamlStream.cs
- SoapFault.cs
- CorrelationTokenInvalidatedHandler.cs
- MenuEventArgs.cs
- OledbConnectionStringbuilder.cs
- BaseParagraph.cs
- Color.cs
- RawStylusActions.cs
- SessionPageStateSection.cs
- FixedSOMPage.cs
- TextParaLineResult.cs
- CustomValidator.cs
- SystemIPAddressInformation.cs
- TextureBrush.cs
- UrlAuthFailureHandler.cs
- RawStylusInput.cs
- ArrayMergeHelper.cs
- IConvertible.cs
- ArgumentOutOfRangeException.cs
- dbdatarecord.cs
- ProfileBuildProvider.cs
- CqlLexer.cs
- StaticResourceExtension.cs
- CacheSection.cs
- FamilyCollection.cs
- WrapperSecurityCommunicationObject.cs
- SspiSecurityTokenParameters.cs
- MatrixStack.cs
- ImportedPolicyConversionContext.cs
- safex509handles.cs
- GlyphElement.cs
- VisualTreeUtils.cs
- DesignerExtenders.cs
- HttpCachePolicy.cs
- WebPartEditorCancelVerb.cs
- PersonalizationStateInfoCollection.cs
- RenderData.cs