Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / Selectors / SecurityTokenRequirement.cs / 1 / SecurityTokenRequirement.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.IdentityModel.Selectors { using System.Globalization; using System.Text; using System.Collections; using System.Collections.Generic; using System.IdentityModel.Tokens; public class SecurityTokenRequirement { const string Namespace = "http://schemas.microsoft.com/ws/2006/05/identitymodel/securitytokenrequirement"; const string tokenTypeProperty = Namespace + "/TokenType"; const string keyUsageProperty = Namespace + "/KeyUsage"; const string keyTypeProperty = Namespace + "/KeyType"; const string keySizeProperty = Namespace + "/KeySize"; const string requireCryptographicTokenProperty = Namespace + "/RequireCryptographicToken"; const string peerAuthenticationMode = Namespace + "/PeerAuthenticationMode"; const bool defaultRequireCryptographicToken = false; const SecurityKeyUsage defaultKeyUsage = SecurityKeyUsage.Signature; const SecurityKeyType defaultKeyType = SecurityKeyType.SymmetricKey; const int defaultKeySize = 0; Dictionaryproperties; public SecurityTokenRequirement() { properties = new Dictionary (); this.Initialize(); } static public string TokenTypeProperty { get { return tokenTypeProperty; } } static public string KeyUsageProperty { get { return keyUsageProperty; } } static public string KeyTypeProperty { get { return keyTypeProperty; } } static public string KeySizeProperty { get { return keySizeProperty; } } static public string RequireCryptographicTokenProperty { get { return requireCryptographicTokenProperty; } } static public string PeerAuthenticationMode { get { return peerAuthenticationMode; } } public string TokenType { get { string result; return (this.TryGetProperty (TokenTypeProperty, out result)) ? result : null; } set { this.properties[TokenTypeProperty] = value; } } public bool RequireCryptographicToken { get { bool result; return (this.TryGetProperty (RequireCryptographicTokenProperty, out result)) ? result : defaultRequireCryptographicToken; } set { this.properties[RequireCryptographicTokenProperty] = (object)value; } } public SecurityKeyUsage KeyUsage { get { SecurityKeyUsage result; return (this.TryGetProperty (KeyUsageProperty, out result)) ? result : defaultKeyUsage; } set { SecurityKeyUsageHelper.Validate(value); this.properties[KeyUsageProperty] = (object)value; } } public SecurityKeyType KeyType { get { SecurityKeyType result; return (this.TryGetProperty (KeyTypeProperty, out result)) ? result : defaultKeyType; } set { SecurityKeyTypeHelper.Validate(value); this.properties[KeyTypeProperty] = (object)value; } } public int KeySize { get { int result; return (this.TryGetProperty (KeySizeProperty, out result)) ? result : defaultKeySize; } set { if (value < 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("value", SR.GetString(SR.ValueMustBeNonNegative))); } this.Properties[KeySizeProperty] = value; } } public IDictionary Properties { get { return this.properties; } } void Initialize() { this.KeyType = defaultKeyType; this.KeyUsage = defaultKeyUsage; this.RequireCryptographicToken = defaultRequireCryptographicToken; this.KeySize = defaultKeySize; } public TValue GetProperty (string propertyName) { TValue result; if (!TryGetProperty (propertyName, out result)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentException(SR.GetString(SR.SecurityTokenRequirementDoesNotContainProperty, propertyName))); } return result; } public bool TryGetProperty (string propertyName, out TValue result) { object dictionaryValue; if (!Properties.TryGetValue(propertyName, out dictionaryValue)) { result = default(TValue); return false; } if (dictionaryValue != null && !typeof(TValue).IsAssignableFrom(dictionaryValue.GetType())) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentException(SR.GetString(SR.SecurityTokenRequirementHasInvalidTypeForProperty, propertyName, dictionaryValue.GetType(), typeof(TValue)))); } result = (TValue)dictionaryValue; return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
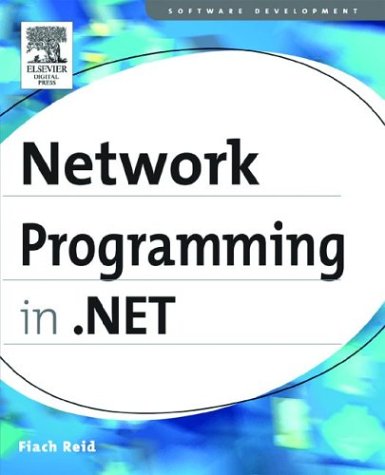
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaCollection.cs
- MyContact.cs
- Empty.cs
- KeySpline.cs
- FrameworkContentElement.cs
- ProfessionalColorTable.cs
- CommandManager.cs
- CodeDefaultValueExpression.cs
- FilteredAttributeCollection.cs
- SelectorItemAutomationPeer.cs
- UIElementAutomationPeer.cs
- COMException.cs
- EDesignUtil.cs
- VBCodeProvider.cs
- SqlDependency.cs
- AlphaSortedEnumConverter.cs
- XamlClipboardData.cs
- MethodCallExpression.cs
- EmptyEnumerator.cs
- FormatConvertedBitmap.cs
- Scripts.cs
- DataGridPagingPage.cs
- DataObjectAttribute.cs
- VisualBasicSettings.cs
- IgnoreDeviceFilterElement.cs
- SmiRecordBuffer.cs
- Intellisense.cs
- AggregationMinMaxHelpers.cs
- SQLInt32.cs
- PointCollectionValueSerializer.cs
- ProxyFragment.cs
- DefaultValueAttribute.cs
- DictionaryBase.cs
- DataListItem.cs
- PostBackOptions.cs
- XmlSchemaException.cs
- PagedControl.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- BufferAllocator.cs
- GridViewDeleteEventArgs.cs
- SafeCancelMibChangeNotify.cs
- StylusLogic.cs
- WindowsContainer.cs
- ClientRuntimeConfig.cs
- TempEnvironment.cs
- Label.cs
- QuaternionAnimation.cs
- UncommonField.cs
- QuaternionRotation3D.cs
- LinkButton.cs
- SqlConnectionFactory.cs
- DbCommandDefinition.cs
- FontEmbeddingManager.cs
- ValidationSummary.cs
- AnonymousIdentificationSection.cs
- ColumnCollection.cs
- ListQueryResults.cs
- EllipseGeometry.cs
- InstanceLockException.cs
- SqlParameter.cs
- HtmlTable.cs
- SQLInt16.cs
- ComponentCommands.cs
- SQLDouble.cs
- KeyNameIdentifierClause.cs
- FilterElement.cs
- COM2Enum.cs
- ScriptReferenceEventArgs.cs
- MappingModelBuildProvider.cs
- TableDetailsCollection.cs
- ChooseAction.cs
- PlanCompilerUtil.cs
- CodeParameterDeclarationExpressionCollection.cs
- SQLDecimal.cs
- DocumentGridContextMenu.cs
- ResXResourceSet.cs
- NameValueCollection.cs
- SqlDuplicator.cs
- TextSegment.cs
- MissingManifestResourceException.cs
- SrgsToken.cs
- TableDetailsCollection.cs
- DataGridViewRowStateChangedEventArgs.cs
- OleDbFactory.cs
- TextAction.cs
- GroupStyle.cs
- DataGridViewCellValidatingEventArgs.cs
- SqlMultiplexer.cs
- FormatSettings.cs
- HtmlHead.cs
- FlowDocumentReaderAutomationPeer.cs
- StringInfo.cs
- ObjectStateEntryOriginalDbUpdatableDataRecord.cs
- MenuCommand.cs
- DataTablePropertyDescriptor.cs
- AssemblyNameEqualityComparer.cs
- DataStreams.cs
- FrameAutomationPeer.cs
- SqlDataReader.cs
- precedingsibling.cs