Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / Generated / GradientBrush.cs / 1 / GradientBrush.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media { abstract partial class GradientBrush : Brush { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new GradientBrush Clone() { return (GradientBrush)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new GradientBrush CloneCurrentValue() { return (GradientBrush)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void ColorInterpolationModePropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { GradientBrush target = ((GradientBrush) d); target.PropertyChanged(ColorInterpolationModeProperty); } private static void MappingModePropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { GradientBrush target = ((GradientBrush) d); target.PropertyChanged(MappingModeProperty); } private static void SpreadMethodPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { GradientBrush target = ((GradientBrush) d); target.PropertyChanged(SpreadMethodProperty); } private static void GradientStopsPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { GradientBrush target = ((GradientBrush) d); target.PropertyChanged(GradientStopsProperty); } #region Public Properties ////// ColorInterpolationMode - ColorInterpolationMode. Default value is ColorInterpolationMode.SRgbLinearInterpolation. /// public ColorInterpolationMode ColorInterpolationMode { get { return (ColorInterpolationMode) GetValue(ColorInterpolationModeProperty); } set { SetValueInternal(ColorInterpolationModeProperty, value); } } ////// MappingMode - BrushMappingMode. Default value is BrushMappingMode.RelativeToBoundingBox. /// public BrushMappingMode MappingMode { get { return (BrushMappingMode) GetValue(MappingModeProperty); } set { SetValueInternal(MappingModeProperty, value); } } ////// SpreadMethod - GradientSpreadMethod. Default value is GradientSpreadMethod.Pad. /// public GradientSpreadMethod SpreadMethod { get { return (GradientSpreadMethod) GetValue(SpreadMethodProperty); } set { SetValueInternal(SpreadMethodProperty, value); } } ////// GradientStops - GradientStopCollection. Default value is new FreezableDefaultValueFactory(GradientStopCollection.Empty). /// public GradientStopCollection GradientStops { get { return (GradientStopCollection) GetValue(GradientStopsProperty); } set { SetValueInternal(GradientStopsProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties // // This property finds the correct initial size for the _effectiveValues store on the // current DependencyObject as a performance optimization // // This includes: // GradientStops // internal override int EffectiveValuesInitialSize { get { return 1; } } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the GradientBrush.ColorInterpolationMode property. /// public static readonly DependencyProperty ColorInterpolationModeProperty; ////// The DependencyProperty for the GradientBrush.MappingMode property. /// public static readonly DependencyProperty MappingModeProperty; ////// The DependencyProperty for the GradientBrush.SpreadMethod property. /// public static readonly DependencyProperty SpreadMethodProperty; ////// The DependencyProperty for the GradientBrush.GradientStops property. /// public static readonly DependencyProperty GradientStopsProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal const ColorInterpolationMode c_ColorInterpolationMode = ColorInterpolationMode.SRgbLinearInterpolation; internal const BrushMappingMode c_MappingMode = BrushMappingMode.RelativeToBoundingBox; internal const GradientSpreadMethod c_SpreadMethod = GradientSpreadMethod.Pad; internal static GradientStopCollection s_GradientStops = GradientStopCollection.Empty; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static GradientBrush() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // Debug.Assert(s_GradientStops == null || s_GradientStops.IsFrozen, "Detected context bound default value GradientBrush.s_GradientStops (See OS Bug #947272)."); // Initializations Type typeofThis = typeof(GradientBrush); ColorInterpolationModeProperty = RegisterProperty("ColorInterpolationMode", typeof(ColorInterpolationMode), typeofThis, ColorInterpolationMode.SRgbLinearInterpolation, new PropertyChangedCallback(ColorInterpolationModePropertyChanged), new ValidateValueCallback(System.Windows.Media.ValidateEnums.IsColorInterpolationModeValid), /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); MappingModeProperty = RegisterProperty("MappingMode", typeof(BrushMappingMode), typeofThis, BrushMappingMode.RelativeToBoundingBox, new PropertyChangedCallback(MappingModePropertyChanged), new ValidateValueCallback(System.Windows.Media.ValidateEnums.IsBrushMappingModeValid), /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); SpreadMethodProperty = RegisterProperty("SpreadMethod", typeof(GradientSpreadMethod), typeofThis, GradientSpreadMethod.Pad, new PropertyChangedCallback(SpreadMethodPropertyChanged), new ValidateValueCallback(System.Windows.Media.ValidateEnums.IsGradientSpreadMethodValid), /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); GradientStopsProperty = RegisterProperty("GradientStops", typeof(GradientStopCollection), typeofThis, new FreezableDefaultValueFactory(GradientStopCollection.Empty), new PropertyChangedCallback(GradientStopsPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media { abstract partial class GradientBrush : Brush { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new GradientBrush Clone() { return (GradientBrush)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new GradientBrush CloneCurrentValue() { return (GradientBrush)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- private static void ColorInterpolationModePropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { GradientBrush target = ((GradientBrush) d); target.PropertyChanged(ColorInterpolationModeProperty); } private static void MappingModePropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { GradientBrush target = ((GradientBrush) d); target.PropertyChanged(MappingModeProperty); } private static void SpreadMethodPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { GradientBrush target = ((GradientBrush) d); target.PropertyChanged(SpreadMethodProperty); } private static void GradientStopsPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { GradientBrush target = ((GradientBrush) d); target.PropertyChanged(GradientStopsProperty); } #region Public Properties ////// ColorInterpolationMode - ColorInterpolationMode. Default value is ColorInterpolationMode.SRgbLinearInterpolation. /// public ColorInterpolationMode ColorInterpolationMode { get { return (ColorInterpolationMode) GetValue(ColorInterpolationModeProperty); } set { SetValueInternal(ColorInterpolationModeProperty, value); } } ////// MappingMode - BrushMappingMode. Default value is BrushMappingMode.RelativeToBoundingBox. /// public BrushMappingMode MappingMode { get { return (BrushMappingMode) GetValue(MappingModeProperty); } set { SetValueInternal(MappingModeProperty, value); } } ////// SpreadMethod - GradientSpreadMethod. Default value is GradientSpreadMethod.Pad. /// public GradientSpreadMethod SpreadMethod { get { return (GradientSpreadMethod) GetValue(SpreadMethodProperty); } set { SetValueInternal(SpreadMethodProperty, value); } } ////// GradientStops - GradientStopCollection. Default value is new FreezableDefaultValueFactory(GradientStopCollection.Empty). /// public GradientStopCollection GradientStops { get { return (GradientStopCollection) GetValue(GradientStopsProperty); } set { SetValueInternal(GradientStopsProperty, value); } } #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties // // This property finds the correct initial size for the _effectiveValues store on the // current DependencyObject as a performance optimization // // This includes: // GradientStops // internal override int EffectiveValuesInitialSize { get { return 1; } } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the GradientBrush.ColorInterpolationMode property. /// public static readonly DependencyProperty ColorInterpolationModeProperty; ////// The DependencyProperty for the GradientBrush.MappingMode property. /// public static readonly DependencyProperty MappingModeProperty; ////// The DependencyProperty for the GradientBrush.SpreadMethod property. /// public static readonly DependencyProperty SpreadMethodProperty; ////// The DependencyProperty for the GradientBrush.GradientStops property. /// public static readonly DependencyProperty GradientStopsProperty; #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields internal const ColorInterpolationMode c_ColorInterpolationMode = ColorInterpolationMode.SRgbLinearInterpolation; internal const BrushMappingMode c_MappingMode = BrushMappingMode.RelativeToBoundingBox; internal const GradientSpreadMethod c_SpreadMethod = GradientSpreadMethod.Pad; internal static GradientStopCollection s_GradientStops = GradientStopCollection.Empty; #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- static GradientBrush() { // We check our static default fields which are of type Freezable // to make sure that they are not mutable, otherwise we will throw // if these get touched by more than one thread in the lifetime // of your app. (Windows OS Bug #947272) // Debug.Assert(s_GradientStops == null || s_GradientStops.IsFrozen, "Detected context bound default value GradientBrush.s_GradientStops (See OS Bug #947272)."); // Initializations Type typeofThis = typeof(GradientBrush); ColorInterpolationModeProperty = RegisterProperty("ColorInterpolationMode", typeof(ColorInterpolationMode), typeofThis, ColorInterpolationMode.SRgbLinearInterpolation, new PropertyChangedCallback(ColorInterpolationModePropertyChanged), new ValidateValueCallback(System.Windows.Media.ValidateEnums.IsColorInterpolationModeValid), /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); MappingModeProperty = RegisterProperty("MappingMode", typeof(BrushMappingMode), typeofThis, BrushMappingMode.RelativeToBoundingBox, new PropertyChangedCallback(MappingModePropertyChanged), new ValidateValueCallback(System.Windows.Media.ValidateEnums.IsBrushMappingModeValid), /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); SpreadMethodProperty = RegisterProperty("SpreadMethod", typeof(GradientSpreadMethod), typeofThis, GradientSpreadMethod.Pad, new PropertyChangedCallback(SpreadMethodPropertyChanged), new ValidateValueCallback(System.Windows.Media.ValidateEnums.IsGradientSpreadMethodValid), /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); GradientStopsProperty = RegisterProperty("GradientStops", typeof(GradientStopCollection), typeofThis, new FreezableDefaultValueFactory(GradientStopCollection.Empty), new PropertyChangedCallback(GradientStopsPropertyChanged), null, /* isIndependentlyAnimated = */ false, /* coerceValueCallback */ null); } #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
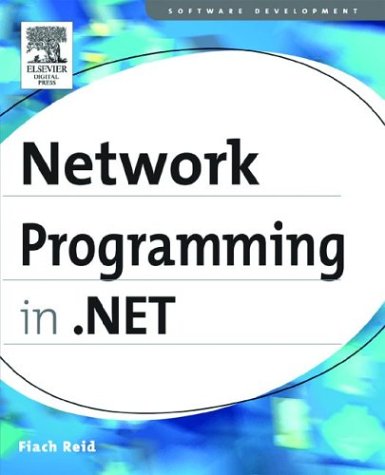
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EventLogInformation.cs
- ObjectStorage.cs
- ScriptReferenceEventArgs.cs
- HtmlControl.cs
- DbgUtil.cs
- SaveWorkflowAsyncResult.cs
- CommentAction.cs
- DirectoryObjectSecurity.cs
- SqlInternalConnection.cs
- FromReply.cs
- SortFieldComparer.cs
- SizeAnimationClockResource.cs
- PermissionSetTriple.cs
- LineBreakRecord.cs
- CachedFontFace.cs
- RightsDocument.cs
- DriveInfo.cs
- WaitHandle.cs
- ToolboxControl.cs
- BitmapMetadataBlob.cs
- CryptoProvider.cs
- LocatorGroup.cs
- Baml2006ReaderContext.cs
- TextTrailingWordEllipsis.cs
- PermissionSetTriple.cs
- FontNamesConverter.cs
- Preprocessor.cs
- BitmapEncoder.cs
- PreservationFileReader.cs
- CodeStatement.cs
- StyleHelper.cs
- RoleGroupCollection.cs
- MSAANativeProvider.cs
- SqlParameter.cs
- CachedFontFace.cs
- _NestedSingleAsyncResult.cs
- SplineKeyFrames.cs
- _WinHttpWebProxyDataBuilder.cs
- ObjectItemCachedAssemblyLoader.cs
- EditBehavior.cs
- AppDomainFactory.cs
- DbProviderServices.cs
- MetadataItemSerializer.cs
- List.cs
- DefaultValueTypeConverter.cs
- StringDictionaryCodeDomSerializer.cs
- LiteralTextContainerControlBuilder.cs
- DataGridToolTip.cs
- ControllableStoryboardAction.cs
- ISFTagAndGuidCache.cs
- ListBase.cs
- EnumType.cs
- CompileXomlTask.cs
- StatementContext.cs
- NativeMethods.cs
- BaseTreeIterator.cs
- ClientConfigurationHost.cs
- DataGridViewCellMouseEventArgs.cs
- StylusPointPropertyUnit.cs
- ReturnType.cs
- BitmapEffect.cs
- LinqDataSourceInsertEventArgs.cs
- DataGridViewRowPostPaintEventArgs.cs
- NativeRightsManagementAPIsStructures.cs
- BatchParser.cs
- IResourceProvider.cs
- WindowsGraphics2.cs
- Grid.cs
- PeerCredentialElement.cs
- XmlSchemaSimpleType.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- JavaScriptObjectDeserializer.cs
- ToolStripDropDownButton.cs
- ProtocolState.cs
- MimeMapping.cs
- ExpandableObjectConverter.cs
- assemblycache.cs
- JavaScriptString.cs
- SqlDeflator.cs
- TableLayoutPanelCellPosition.cs
- ContextMenuService.cs
- TextContainer.cs
- ValidatedControlConverter.cs
- TargetPerspective.cs
- EntityTransaction.cs
- UserControlParser.cs
- DeviceFilterEditorDialog.cs
- XmlWellformedWriter.cs
- XmlLoader.cs
- AppDomainGrammarProxy.cs
- FlowchartDesigner.Helpers.cs
- FormattedText.cs
- AuthenticationService.cs
- XNameConverter.cs
- GacUtil.cs
- CultureTable.cs
- DeviceSpecific.cs
- HtmlMeta.cs
- SamlConstants.cs
- ReturnValue.cs