Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / HtmlControls / HtmlControl.cs / 2 / HtmlControl.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HtmlControl.cs * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Web.UI.HtmlControls { using System; using System.Globalization; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.IO; using System.Web.Util; using System.Web.UI; using AttributeCollection = System.Web.UI.AttributeCollection; using System.Security.Permissions; /* * An abstract base class representing an intrinsic Html tag that * is not represented by both a begin and end tag, for example * INPUT or IMG. */ ////// [ Designer("System.Web.UI.Design.HtmlIntrinsicControlDesigner, " + AssemblyRef.SystemDesign), ToolboxItem(false) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] abstract public class HtmlControl : Control, IAttributeAccessor { internal string _tagName; private AttributeCollection _attributes; ////// The ////// class defines the methods, properties, and events /// common to all HTML Server controls in the Web Forms page framework. /// /// protected HtmlControl() : this("span") { } ////// protected HtmlControl(string tag) { _tagName = tag; } /* * Access to collection of Attributes. */ ////// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public AttributeCollection Attributes { get { if (_attributes == null) _attributes = new AttributeCollection(ViewState); return _attributes; } } /* * Access to collection of styles. */ ////// Gets all attribute name/value pairs expressed on a /// server control tag within a selected ASP.NET page. /// ////// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public CssStyleCollection Style { get { return Attributes.CssStyle; } } /* * Property to get name of tag. */ ////// Gets all /// cascading style sheet (CSS) properties that /// are applied /// to a specified HTML Server control in an .aspx /// file. /// ////// [ WebCategory("Appearance"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public virtual string TagName { get { return _tagName;} } /* * Disabled property. */ ////// Gets the element name of a tag that contains a runat=server /// attribute/value pair. /// ////// [ WebCategory("Behavior"), DefaultValue(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), TypeConverter(typeof(MinimizableAttributeTypeConverter)) ] public bool Disabled { get { string s = Attributes["disabled"]; return((s != null) ? (s.Equals("disabled")) : false); } set { if (value) Attributes["disabled"] = "disabled"; else Attributes["disabled"] = null; } } ////// Gets or sets /// a value indicating whether Disabled attribute is included when a server /// control is rendered. /// ////// ///protected override bool ViewStateIgnoresCase { get { return true; } } /// /// protected override ControlCollection CreateControlCollection() { return new EmptyControlCollection(this); } /* * Render the control into the given writer. */ ///[To be supplied.] ////// /// protected internal override void Render(HtmlTextWriter writer) { RenderBeginTag(writer); } /* * Render only the attributes, attr1=value1 attr2=value2 ... */ ////// /// protected virtual void RenderAttributes(HtmlTextWriter writer) { if (ID != null) writer.WriteAttribute("id", ClientID); Attributes.Render(writer); } /* * Render the begin tag and its attributes, <TAGNAME attr1=value1 attr2=value2>. */ ////// /// protected virtual void RenderBeginTag(HtmlTextWriter writer) { writer.WriteBeginTag(TagName); RenderAttributes(writer); writer.Write(HtmlTextWriter.TagRightChar); } /* * HtmlControls support generic access to Attributes. */ ////// /// string IAttributeAccessor.GetAttribute(string name) { return GetAttribute(name); } ////// /// protected virtual string GetAttribute(string name) { return Attributes[name]; } /* * HtmlControls support generic access to Attributes. */ ////// /// void IAttributeAccessor.SetAttribute(string name, string value) { SetAttribute(name, value); } ////// /// protected virtual void SetAttribute(string name, string value) { Attributes[name] = value; } internal void PreProcessRelativeReferenceAttribute(HtmlTextWriter writer, string attribName) { string url = Attributes[attribName]; // Don't do anything if it's not specified if (String.IsNullOrEmpty(url)) return; try { url = ResolveClientUrl(url); } catch (Exception e) { throw new HttpException(SR.GetString(SR.Property_Had_Malformed_Url, attribName, e.Message)); } writer.WriteAttribute(attribName, url); Attributes.Remove(attribName); } internal static string MapStringAttributeToString(string s) { // If it's an empty string, change it to null if (s != null && s.Length == 0) return null; // Otherwise, just return the input return s; } internal static string MapIntegerAttributeToString(int n) { // If it's -1, change it to null if (n == -1) return null; // Otherwise, convert the integer to a string return n.ToString(NumberFormatInfo.InvariantInfo); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
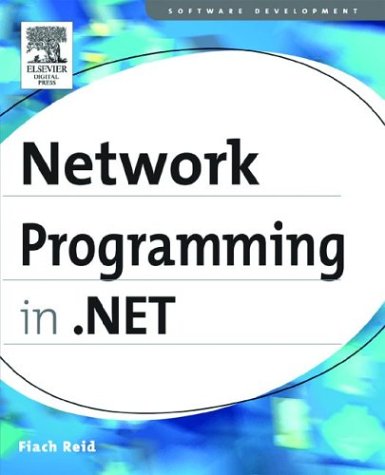
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RequestSecurityTokenResponse.cs
- DataKey.cs
- RetriableClipboard.cs
- DownloadProgressEventArgs.cs
- RectAnimationClockResource.cs
- NavigatorInput.cs
- SiteMap.cs
- ValidateNames.cs
- AdvancedBindingEditor.cs
- ColorConverter.cs
- Registry.cs
- InvokePatternIdentifiers.cs
- LoggedException.cs
- WindowsPrincipal.cs
- HttpHandlersSection.cs
- SpeakInfo.cs
- FileLevelControlBuilderAttribute.cs
- Brush.cs
- TablePattern.cs
- DesigntimeLicenseContextSerializer.cs
- OwnerDrawPropertyBag.cs
- ProcessHost.cs
- securitycriticaldataClass.cs
- Byte.cs
- DataGridViewRowContextMenuStripNeededEventArgs.cs
- ProxyElement.cs
- UdpDiscoveryEndpointElement.cs
- MimeAnyImporter.cs
- ToolStripItemClickedEventArgs.cs
- ThemeInfoAttribute.cs
- QuinticEase.cs
- TransportContext.cs
- GridViewRowCollection.cs
- Rect3D.cs
- DataMemberConverter.cs
- BasicKeyConstraint.cs
- CssClassPropertyAttribute.cs
- StreamReader.cs
- ConversionValidationRule.cs
- WarningException.cs
- ToolStripItem.cs
- XmlWriterTraceListener.cs
- cookiecontainer.cs
- BufferAllocator.cs
- Italic.cs
- HttpPostedFile.cs
- ValidationError.cs
- InputManager.cs
- TextureBrush.cs
- DataGridViewCellPaintingEventArgs.cs
- RadioButtonBaseAdapter.cs
- NavigationFailedEventArgs.cs
- GuidConverter.cs
- DSASignatureDeformatter.cs
- UnsignedPublishLicense.cs
- TextTreeRootNode.cs
- UidPropertyAttribute.cs
- XmlQueryContext.cs
- EasingFunctionBase.cs
- QueueProcessor.cs
- HelpKeywordAttribute.cs
- HandlerWithFactory.cs
- ArraySegment.cs
- PersistencePipeline.cs
- MenuAdapter.cs
- ClientConvert.cs
- StrokeDescriptor.cs
- XmlSchemas.cs
- MarkupCompiler.cs
- WorkflowMessageEventArgs.cs
- StrokeCollection.cs
- MetadataCollection.cs
- TextEmbeddedObject.cs
- MouseEvent.cs
- ClientTargetSection.cs
- Rijndael.cs
- GroupItemAutomationPeer.cs
- PasswordRecoveryDesigner.cs
- XmlEncodedRawTextWriter.cs
- NodeFunctions.cs
- loginstatus.cs
- WindowsComboBox.cs
- Rotation3DAnimationBase.cs
- HwndSubclass.cs
- SchemaTableOptionalColumn.cs
- XmlDataProvider.cs
- SizeValueSerializer.cs
- SharedStatics.cs
- ChannelEndpointElement.cs
- WebAdminConfigurationHelper.cs
- ObjectListShowCommandsEventArgs.cs
- MouseActionValueSerializer.cs
- HandleExceptionArgs.cs
- SystemWebSectionGroup.cs
- COM2ICategorizePropertiesHandler.cs
- MinMaxParagraphWidth.cs
- CompositeFontParser.cs
- RangeContentEnumerator.cs
- TemplatePagerField.cs
- EntityDataSourceDataSelection.cs