Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / CompMod / System / ComponentModel / Design / Serialization / StatementContext.cs / 1 / StatementContext.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel.Design.Serialization { using System; using System.CodeDom; using System.Collections; using System.Collections.Generic; ////// /// This object can be placed on the context stack to provide a place for statements /// to be serialized into. Normally, statements are serialized into whatever statement /// collection that is on the context stack. You can modify this behavior by creating /// a statement context and calling Populate with a collection of objects whose statements /// you would like stored in the statement table. As each object is serialized in /// SerializeToExpression it will have its contents placed in the statement table. /// saved in a table within the context. If you push this object on the stack it is your /// responsibility to integrate the statements added to it into your own collection of statements. /// public sealed class StatementContext { private ObjectStatementCollection _statements; ////// /// This is a table of statements that is offered by the statement context. /// public ObjectStatementCollection StatementCollection { get { if (_statements == null) { _statements = new ObjectStatementCollection(); } return _statements; } } } ////// /// This is a table of statements that is offered by the statement context. /// public sealed class ObjectStatementCollection : IEnumerable { private List_table; private int _version; /// /// Only creatable by the StatementContext. /// internal ObjectStatementCollection() { } ////// Adds an owner to the table. Statements can be null, in which case it /// will be demand created when fished out of the table. This will throw /// if there is already a valid collection for the owner. /// private void AddOwner(object statementOwner, CodeStatementCollection statements) { if (_table == null) { _table = new List(); } else { for (int idx = 0; idx < _table.Count; idx++) { if (object.ReferenceEquals(_table[idx].Owner, statementOwner)) { if (_table[idx].Statements != null) { throw new InvalidOperationException(); } else { if (statements != null) { _table[idx] = new TableEntry(statementOwner, statements); } return; } } } } _table.Add(new TableEntry(statementOwner, statements)); _version++; } /// /// /// Indexer. This will return the statement collection for the given owner. /// It will return null only if the owner is not in the table. /// public CodeStatementCollection this[object statementOwner] { get { if (statementOwner == null) { throw new ArgumentNullException("statementOwner"); } if (_table != null) { for (int idx = 0; idx < _table.Count; idx++) { if (object.ReferenceEquals(_table[idx].Owner, statementOwner)) { if (_table[idx].Statements == null) { _table[idx] = new TableEntry(statementOwner, new CodeStatementCollection()); } return _table[idx].Statements; } } foreach(TableEntry e in _table) { if (object.ReferenceEquals(e.Owner, statementOwner)) { return e.Statements; } } } return null; } } ////// /// Returns true if the given statement owner is in the table. /// public bool ContainsKey(object statementOwner) { if (statementOwner == null) { throw new ArgumentNullException("statementOwner"); } if (_table != null) { return (this[statementOwner] != null); } return false; } ////// /// Returns an enumerator for this table. The keys of the enumerator are statement /// owner objects and the values are instances of CodeStatementCollection. /// public IDictionaryEnumerator GetEnumerator() { return new TableEnumerator(this); } ////// /// This method populates the statement table with a collection of statement owners. /// The creator of the statement context should do this if it wishes statement tables to be used to store /// values for certain objects. /// public void Populate(ICollection statementOwners) { if (statementOwners == null) { throw new ArgumentNullException("statementOwners"); } foreach(object o in statementOwners) { Populate(o); } } ////// /// This method populates the statement table with a collection of statement owners. /// The creator of the statement context should do this if it wishes statement tables to be used to store /// values for certain objects. /// public void Populate(object owner) { if (owner == null) { throw new ArgumentNullException("owner"); } AddOwner(owner, null); } ////// /// Returns an enumerator for this table. The value is a DictionaryEntry containing /// the statement owner and the statement collection. /// IEnumerator IEnumerable.GetEnumerator() { return GetEnumerator(); } private struct TableEntry { public object Owner; public CodeStatementCollection Statements; public TableEntry(object owner, CodeStatementCollection statements) { this.Owner = owner; this.Statements = statements; } } private struct TableEnumerator : IDictionaryEnumerator { private ObjectStatementCollection _table; private int _version; int _position; public TableEnumerator(ObjectStatementCollection table) { _table = table; _version = _table._version; _position = -1; } public object Current { get { return Entry; } } public DictionaryEntry Entry { get { if (_version != _table._version) { throw new InvalidOperationException(); } if (_position < 0 || _table._table == null || _position >= _table._table.Count) { throw new InvalidOperationException(); } if (_table._table[_position].Statements == null) { _table._table[_position] = new TableEntry(_table._table[_position].Owner, new CodeStatementCollection()); } TableEntry entry = _table._table[_position]; return new DictionaryEntry(entry.Owner, entry.Statements); } } public object Key { get { return Entry.Key; } } public object Value { get { return Entry.Value; } } public bool MoveNext() { if (_table._table != null && (_position+1) < _table._table.Count) { _position++; return true; } return false; } public void Reset() { _position = -1; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
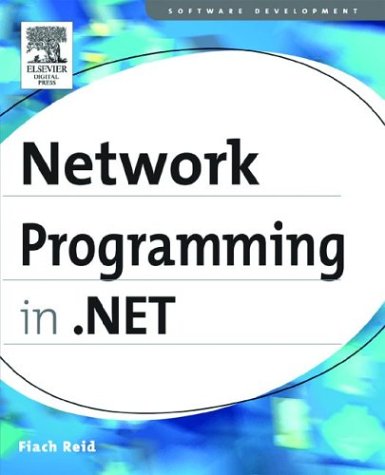
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MachineKeySection.cs
- DataStreams.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- XmlSerializerFactory.cs
- OleDbStruct.cs
- InheritanceRules.cs
- ZipIOExtraFieldPaddingElement.cs
- TabControl.cs
- Span.cs
- _LocalDataStoreMgr.cs
- TransformCryptoHandle.cs
- FixedDocument.cs
- SecurityElement.cs
- PlatformCulture.cs
- BitSet.cs
- TCPListener.cs
- DataSourceCache.cs
- CheckBoxAutomationPeer.cs
- Animatable.cs
- TreeNodeCollection.cs
- TextParagraph.cs
- Dump.cs
- NativeMethods.cs
- Array.cs
- BindingCollection.cs
- EdmComplexPropertyAttribute.cs
- MdiWindowListStrip.cs
- VirtualDirectoryMapping.cs
- ScaleTransform.cs
- HttpStaticObjectsCollectionBase.cs
- SetterBase.cs
- WebPartConnectVerb.cs
- WmlTextBoxAdapter.cs
- DataGridItemCollection.cs
- ToolStripPanelRow.cs
- EntityUtil.cs
- DataGridViewAccessibleObject.cs
- SqlDataSourceCommandEventArgs.cs
- AspCompat.cs
- GroupDescription.cs
- ToolStripItem.cs
- listitem.cs
- BindingElement.cs
- precedingquery.cs
- PointUtil.cs
- Propagator.cs
- CapiSafeHandles.cs
- MDIControlStrip.cs
- DesignerObject.cs
- BuildDependencySet.cs
- Axis.cs
- OptimisticConcurrencyException.cs
- DictionaryMarkupSerializer.cs
- JournalEntry.cs
- CodeAttributeArgumentCollection.cs
- NestPullup.cs
- DataGridColumn.cs
- SafeFileMappingHandle.cs
- ConfigurationManager.cs
- ContextBase.cs
- StructuralCache.cs
- Figure.cs
- InvalidPipelineStoreException.cs
- WebPartMinimizeVerb.cs
- DllHostedComPlusServiceHost.cs
- CommonXSendMessage.cs
- AggregationMinMaxHelpers.cs
- TextDecoration.cs
- DbException.cs
- FullTextState.cs
- NavigationWindowAutomationPeer.cs
- FontInfo.cs
- XmlWrappingWriter.cs
- NominalTypeEliminator.cs
- WorkflowRuntimeElement.cs
- Util.cs
- MimeTypeMapper.cs
- AnnouncementService.cs
- HandledEventArgs.cs
- DataGridViewColumnStateChangedEventArgs.cs
- CookieParameter.cs
- FamilyCollection.cs
- contentDescriptor.cs
- ObjectTag.cs
- SinglePageViewer.cs
- MsmqHostedTransportManager.cs
- ToolStripDropDownDesigner.cs
- _BaseOverlappedAsyncResult.cs
- controlskin.cs
- WebControl.cs
- GeometryHitTestParameters.cs
- ZoneLinkButton.cs
- DigitalSignatureProvider.cs
- LambdaExpression.cs
- ListBase.cs
- ActivityDesignerResources.cs
- ObjectDataProvider.cs
- AnimationClockResource.cs
- NetworkStream.cs
- Visual3DCollection.cs