Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / CompMod / System / ComponentModel / Design / DesignerActionMethodItem.cs / 1 / DesignerActionMethodItem.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel.Design { using System; using System.ComponentModel; using System.Design; using System.Reflection; ////// /// [to be provided] /// public class DesignerActionMethodItem : DesignerActionItem { private string memberName; private bool includeAsDesignerVerb; private DesignerActionList actionList; private MethodInfo methodInfo; private IComponent relatedComponent; ////// /// [to be provvided] /// public DesignerActionMethodItem(DesignerActionList actionList, string memberName, string displayName, string category, string description, bool includeAsDesignerVerb) : base( displayName, category, description) { this.actionList = actionList; this.memberName = memberName; this.includeAsDesignerVerb = includeAsDesignerVerb; } ////// /// [to be provvided] /// public DesignerActionMethodItem(DesignerActionList actionList, string memberName, string displayName) : this(actionList, memberName, displayName, null, null, false) { } ////// /// [to be provvided] /// public DesignerActionMethodItem(DesignerActionList actionList, string memberName, string displayName, bool includeAsDesignerVerb) : this(actionList, memberName, displayName, null, null, includeAsDesignerVerb) { } ////// /// [to be provvided] /// public DesignerActionMethodItem(DesignerActionList actionList, string memberName, string displayName, string category) : this(actionList, memberName, displayName, category, null, false) { } ////// /// [to be provvided] /// public DesignerActionMethodItem(DesignerActionList actionList, string memberName, string displayName, string category, bool includeAsDesignerVerb) : this(actionList, memberName, displayName, category, null, includeAsDesignerVerb) { } ////// /// [to be provvided] /// public DesignerActionMethodItem(DesignerActionList actionList, string memberName, string displayName, string category, string description) : this(actionList, memberName, displayName, category, description, false) { } internal DesignerActionMethodItem() { } ////// /// [to be provvided] /// public virtual string MemberName { get { return memberName; } } ////// /// [to be provvided] /// public IComponent RelatedComponent { get { return relatedComponent; } set { relatedComponent = value; } } ////// /// [to be provvided] /// public virtual bool IncludeAsDesignerVerb { get { return includeAsDesignerVerb; } } // this is only use for verbs so that a designer action method item can // be converted to a verb. Verbs use an EventHandler to call their invoke // so we need a way to translate the EventHandler Invoke into ou own Invoke internal void Invoke(object sender, EventArgs args) { Invoke(); } public virtual void Invoke() { if (methodInfo == null) { // we look public AND private or protected methods methodInfo = actionList.GetType().GetMethod(memberName, BindingFlags.Default | BindingFlags.Instance | BindingFlags.Public | BindingFlags.NonPublic); } if (methodInfo != null) { methodInfo.Invoke(actionList, null); } else { throw new InvalidOperationException(SR.GetString(SR.DesignerActionPanel_CouldNotFindMethod, MemberName)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
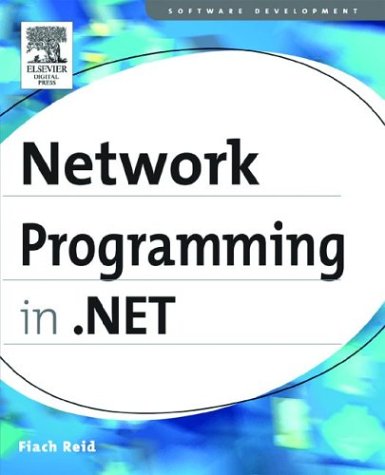
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpTransportManager.cs
- PlainXmlDeserializer.cs
- MemberProjectedSlot.cs
- XmlSchemaDatatype.cs
- OleStrCAMarshaler.cs
- SiteMap.cs
- BlockExpression.cs
- TokenBasedSet.cs
- HandledEventArgs.cs
- KeyNotFoundException.cs
- ArraySortHelper.cs
- BindingExpressionBase.cs
- SqlInternalConnectionSmi.cs
- X509WindowsSecurityToken.cs
- Font.cs
- PrivilegedConfigurationManager.cs
- SourceFileInfo.cs
- DynamicExpression.cs
- WebRequestModuleElement.cs
- SqlDataSourceParameterParser.cs
- ResolveNameEventArgs.cs
- QuaternionRotation3D.cs
- UnknownWrapper.cs
- TemplateControlBuildProvider.cs
- UnauthorizedAccessException.cs
- ListenerSessionConnection.cs
- BaseCAMarshaler.cs
- UnmanagedMarshal.cs
- MappingSource.cs
- RawStylusSystemGestureInputReport.cs
- XappLauncher.cs
- Int32RectConverter.cs
- CommandDevice.cs
- CompiledELinqQueryState.cs
- DataGridViewCellMouseEventArgs.cs
- DataBoundLiteralControl.cs
- RuntimeArgumentHandle.cs
- cookie.cs
- Module.cs
- ArglessEventHandlerProxy.cs
- TextEditorMouse.cs
- TraceUtils.cs
- PointLightBase.cs
- ComponentDispatcherThread.cs
- SdlChannelSink.cs
- SQLMembershipProvider.cs
- ProjectionPath.cs
- UnsupportedPolicyOptionsException.cs
- SQLStringStorage.cs
- FileRecordSequence.cs
- HttpCachePolicy.cs
- PageContentAsyncResult.cs
- Stylus.cs
- NativeMethodsCLR.cs
- ConsumerConnectionPoint.cs
- ReadingWritingEntityEventArgs.cs
- JsonStringDataContract.cs
- ListDictionary.cs
- SqlDataSourceCommandEventArgs.cs
- IDispatchConstantAttribute.cs
- WsdlContractConversionContext.cs
- TcpConnectionPoolSettingsElement.cs
- HttpListenerRequestUriBuilder.cs
- NativeMethods.cs
- AppDomainAttributes.cs
- TransformCryptoHandle.cs
- FtpWebRequest.cs
- DropDownButton.cs
- OleDbDataAdapter.cs
- SolidBrush.cs
- RandomNumberGenerator.cs
- HandoffBehavior.cs
- XslException.cs
- iisPickupDirectory.cs
- BamlWriter.cs
- BookmarkEventArgs.cs
- FixedSOMElement.cs
- DesignerActionListCollection.cs
- SymbolDocumentInfo.cs
- QueryContinueDragEvent.cs
- AutomationIdentifierGuids.cs
- AuthenticateEventArgs.cs
- ResponseBodyWriter.cs
- SymmetricAlgorithm.cs
- RangeBase.cs
- BindToObject.cs
- log.cs
- HScrollProperties.cs
- BitSet.cs
- ResourcesChangeInfo.cs
- CounterSample.cs
- ParsedAttributeCollection.cs
- ObjectDataSource.cs
- MenuEventArgs.cs
- EmptyEnumerator.cs
- ParallelTimeline.cs
- _AutoWebProxyScriptWrapper.cs
- Rules.cs
- LineInfo.cs
- MessageSecurityVersion.cs