Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / WebControls / HyperLinkColumn.cs / 1305376 / HyperLinkColumn.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Globalization; using System.Web; using System.Web.UI; using System.Web.Util; ////// public class HyperLinkColumn : DataGridColumn { private PropertyDescriptor textFieldDesc; private PropertyDescriptor urlFieldDesc; ///Creates a column within the ///containing hyperlinks that /// navigate to specified URLs. /// public HyperLinkColumn() { } ///Initializes a new instance of the ///class. /// [ WebCategory("Data"), DefaultValue(""), WebSysDescription(SR.HyperLinkColumn_DataNavigateUrlField) ] public virtual string DataNavigateUrlField { get { object o = ViewState["DataNavigateUrlField"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["DataNavigateUrlField"] = value; OnColumnChanged(); } } ///Gets or sets the field in the DataSource that provides the URL of the page to navigate to. ////// [ WebCategory("Data"), DefaultValue(""), DescriptionAttribute("The formatting applied to the value bound to the NavigateUrl property.") ] public virtual string DataNavigateUrlFormatString { get { object o = ViewState["DataNavigateUrlFormatString"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["DataNavigateUrlFormatString"] = value; OnColumnChanged(); } } ///Gets or sets the formatting applied to the ////// property. /// [ WebCategory("Data"), DefaultValue(""), WebSysDescription(SR.HyperLinkColumn_DataTextField) ] public virtual string DataTextField { get { object o = ViewState["DataTextField"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["DataTextField"] = value; OnColumnChanged(); } } ///Gets or sets the field in the DataSource that will be used as the source of /// data for the ///property. /// [ WebCategory("Data"), DefaultValue(""), DescriptionAttribute("The formatting applied to the value bound to the Text property.") ] public virtual string DataTextFormatString { get { object o = ViewState["DataTextFormatString"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["DataTextFormatString"] = value; OnColumnChanged(); } } ///Gets or sets the formatting applied to the ////// property. /// [ WebCategory("Behavior"), DefaultValue(""), UrlProperty(), WebSysDescription(SR.HyperLinkColumn_NavigateUrl) ] public virtual string NavigateUrl { get { object o = ViewState["NavigateUrl"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["NavigateUrl"] = value; OnColumnChanged(); } } ///Gets or sets the URL to navigate to when the hyperlink is clicked. ////// [ WebCategory("Behavior"), DefaultValue(""), TypeConverter(typeof(TargetConverter)), WebSysDescription(SR.HyperLink_Target) ] public virtual string Target { get { object o = ViewState["Target"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["Target"] = value; OnColumnChanged(); } } ///Gets or sets the window or target frame that is /// used to display the contents resulting from the hyperlink. ////// [ Localizable(true), WebCategory("Appearance"), DefaultValue(""), WebSysDescription(SR.HyperLinkColumn_Text) ] public virtual string Text { get { object o = ViewState["Text"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["Text"] = value; OnColumnChanged(); } } ///Gets or sets the text to display for the hyperlink. ////// protected virtual string FormatDataNavigateUrlValue(object dataUrlValue) { string formattedUrlValue = String.Empty; if (!DataBinder.IsNull(dataUrlValue)) { string formatting = DataNavigateUrlFormatString; if (formatting.Length == 0) { formattedUrlValue = dataUrlValue.ToString(); } else { formattedUrlValue = String.Format(CultureInfo.CurrentCulture, formatting, dataUrlValue); } } return formattedUrlValue; } ////// protected virtual string FormatDataTextValue(object dataTextValue) { string formattedTextValue = String.Empty; if (!DataBinder.IsNull(dataTextValue)) { string formatting = DataTextFormatString; if (formatting.Length == 0) { formattedTextValue = dataTextValue.ToString(); } else { formattedTextValue = String.Format(CultureInfo.CurrentCulture, formatting, dataTextValue); } } return formattedTextValue; } ////// public override void Initialize() { base.Initialize(); textFieldDesc = null; urlFieldDesc = null; } ////// public override void InitializeCell(TableCell cell, int columnIndex, ListItemType itemType) { base.InitializeCell(cell, columnIndex, itemType); if ((itemType != ListItemType.Header) && (itemType != ListItemType.Footer)) { HyperLink hyperLink = new HyperLink(); hyperLink.Text = Text; hyperLink.NavigateUrl = NavigateUrl; hyperLink.Target = Target; if ((DataNavigateUrlField.Length != 0) || (DataTextField.Length != 0)) { hyperLink.DataBinding += new EventHandler(this.OnDataBindColumn); } cell.Controls.Add(hyperLink); } } ////// Initializes the cell representing this column with the /// contained hyperlink. ////// private void OnDataBindColumn(object sender, EventArgs e) { Debug.Assert((DataTextField.Length != 0) || (DataNavigateUrlField.Length != 0), "Shouldn't be DataBinding without a DataTextField and DataNavigateUrlField"); HyperLink boundControl = (HyperLink)sender; DataGridItem item = (DataGridItem)boundControl.NamingContainer; object dataItem = item.DataItem; if ((textFieldDesc == null) && (urlFieldDesc == null)) { PropertyDescriptorCollection props = TypeDescriptor.GetProperties(dataItem); string fieldName; fieldName = DataTextField; if (fieldName.Length != 0) { textFieldDesc = props.Find(fieldName, true); if ((textFieldDesc == null) && !DesignMode) { throw new HttpException(SR.GetString(SR.Field_Not_Found, fieldName)); } } fieldName = DataNavigateUrlField; if (fieldName.Length != 0) { urlFieldDesc = props.Find(fieldName, true); if ((urlFieldDesc == null) && !DesignMode) { throw new HttpException(SR.GetString(SR.Field_Not_Found, fieldName)); } } } if (textFieldDesc != null) { object data = textFieldDesc.GetValue(dataItem); string dataValue = FormatDataTextValue(data); boundControl.Text = dataValue; } else if (DesignMode && (DataTextField.Length != 0)) { boundControl.Text = SR.GetString(SR.Sample_Databound_Text); } if (urlFieldDesc != null) { object data = urlFieldDesc.GetValue(dataItem); string dataValue = FormatDataNavigateUrlValue(data); boundControl.NavigateUrl = dataValue; } else if (DesignMode && (DataNavigateUrlField.Length != 0)) { boundControl.NavigateUrl = "url"; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Globalization; using System.Web; using System.Web.UI; using System.Web.Util; ////// public class HyperLinkColumn : DataGridColumn { private PropertyDescriptor textFieldDesc; private PropertyDescriptor urlFieldDesc; ///Creates a column within the ///containing hyperlinks that /// navigate to specified URLs. /// public HyperLinkColumn() { } ///Initializes a new instance of the ///class. /// [ WebCategory("Data"), DefaultValue(""), WebSysDescription(SR.HyperLinkColumn_DataNavigateUrlField) ] public virtual string DataNavigateUrlField { get { object o = ViewState["DataNavigateUrlField"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["DataNavigateUrlField"] = value; OnColumnChanged(); } } ///Gets or sets the field in the DataSource that provides the URL of the page to navigate to. ////// [ WebCategory("Data"), DefaultValue(""), DescriptionAttribute("The formatting applied to the value bound to the NavigateUrl property.") ] public virtual string DataNavigateUrlFormatString { get { object o = ViewState["DataNavigateUrlFormatString"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["DataNavigateUrlFormatString"] = value; OnColumnChanged(); } } ///Gets or sets the formatting applied to the ////// property. /// [ WebCategory("Data"), DefaultValue(""), WebSysDescription(SR.HyperLinkColumn_DataTextField) ] public virtual string DataTextField { get { object o = ViewState["DataTextField"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["DataTextField"] = value; OnColumnChanged(); } } ///Gets or sets the field in the DataSource that will be used as the source of /// data for the ///property. /// [ WebCategory("Data"), DefaultValue(""), DescriptionAttribute("The formatting applied to the value bound to the Text property.") ] public virtual string DataTextFormatString { get { object o = ViewState["DataTextFormatString"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["DataTextFormatString"] = value; OnColumnChanged(); } } ///Gets or sets the formatting applied to the ////// property. /// [ WebCategory("Behavior"), DefaultValue(""), UrlProperty(), WebSysDescription(SR.HyperLinkColumn_NavigateUrl) ] public virtual string NavigateUrl { get { object o = ViewState["NavigateUrl"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["NavigateUrl"] = value; OnColumnChanged(); } } ///Gets or sets the URL to navigate to when the hyperlink is clicked. ////// [ WebCategory("Behavior"), DefaultValue(""), TypeConverter(typeof(TargetConverter)), WebSysDescription(SR.HyperLink_Target) ] public virtual string Target { get { object o = ViewState["Target"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["Target"] = value; OnColumnChanged(); } } ///Gets or sets the window or target frame that is /// used to display the contents resulting from the hyperlink. ////// [ Localizable(true), WebCategory("Appearance"), DefaultValue(""), WebSysDescription(SR.HyperLinkColumn_Text) ] public virtual string Text { get { object o = ViewState["Text"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["Text"] = value; OnColumnChanged(); } } ///Gets or sets the text to display for the hyperlink. ////// protected virtual string FormatDataNavigateUrlValue(object dataUrlValue) { string formattedUrlValue = String.Empty; if (!DataBinder.IsNull(dataUrlValue)) { string formatting = DataNavigateUrlFormatString; if (formatting.Length == 0) { formattedUrlValue = dataUrlValue.ToString(); } else { formattedUrlValue = String.Format(CultureInfo.CurrentCulture, formatting, dataUrlValue); } } return formattedUrlValue; } ////// protected virtual string FormatDataTextValue(object dataTextValue) { string formattedTextValue = String.Empty; if (!DataBinder.IsNull(dataTextValue)) { string formatting = DataTextFormatString; if (formatting.Length == 0) { formattedTextValue = dataTextValue.ToString(); } else { formattedTextValue = String.Format(CultureInfo.CurrentCulture, formatting, dataTextValue); } } return formattedTextValue; } ////// public override void Initialize() { base.Initialize(); textFieldDesc = null; urlFieldDesc = null; } ////// public override void InitializeCell(TableCell cell, int columnIndex, ListItemType itemType) { base.InitializeCell(cell, columnIndex, itemType); if ((itemType != ListItemType.Header) && (itemType != ListItemType.Footer)) { HyperLink hyperLink = new HyperLink(); hyperLink.Text = Text; hyperLink.NavigateUrl = NavigateUrl; hyperLink.Target = Target; if ((DataNavigateUrlField.Length != 0) || (DataTextField.Length != 0)) { hyperLink.DataBinding += new EventHandler(this.OnDataBindColumn); } cell.Controls.Add(hyperLink); } } ////// Initializes the cell representing this column with the /// contained hyperlink. ////// private void OnDataBindColumn(object sender, EventArgs e) { Debug.Assert((DataTextField.Length != 0) || (DataNavigateUrlField.Length != 0), "Shouldn't be DataBinding without a DataTextField and DataNavigateUrlField"); HyperLink boundControl = (HyperLink)sender; DataGridItem item = (DataGridItem)boundControl.NamingContainer; object dataItem = item.DataItem; if ((textFieldDesc == null) && (urlFieldDesc == null)) { PropertyDescriptorCollection props = TypeDescriptor.GetProperties(dataItem); string fieldName; fieldName = DataTextField; if (fieldName.Length != 0) { textFieldDesc = props.Find(fieldName, true); if ((textFieldDesc == null) && !DesignMode) { throw new HttpException(SR.GetString(SR.Field_Not_Found, fieldName)); } } fieldName = DataNavigateUrlField; if (fieldName.Length != 0) { urlFieldDesc = props.Find(fieldName, true); if ((urlFieldDesc == null) && !DesignMode) { throw new HttpException(SR.GetString(SR.Field_Not_Found, fieldName)); } } } if (textFieldDesc != null) { object data = textFieldDesc.GetValue(dataItem); string dataValue = FormatDataTextValue(data); boundControl.Text = dataValue; } else if (DesignMode && (DataTextField.Length != 0)) { boundControl.Text = SR.GetString(SR.Sample_Databound_Text); } if (urlFieldDesc != null) { object data = urlFieldDesc.GetValue(dataItem); string dataValue = FormatDataNavigateUrlValue(data); boundControl.NavigateUrl = dataValue; } else if (DesignMode && (DataNavigateUrlField.Length != 0)) { boundControl.NavigateUrl = "url"; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
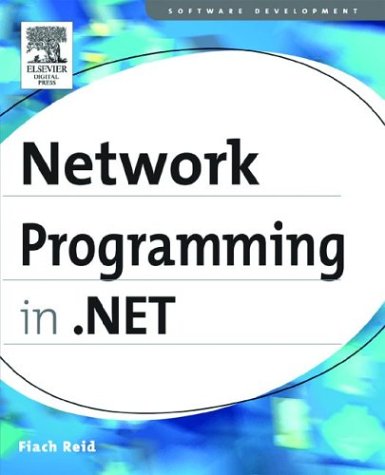
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- JournalEntry.cs
- FontClient.cs
- ContractAdapter.cs
- ExternalFile.cs
- SqlNotificationRequest.cs
- SurrogateEncoder.cs
- RightsManagementResourceHelper.cs
- FastEncoderWindow.cs
- StylusEditingBehavior.cs
- ConfigurationStrings.cs
- XPathDocument.cs
- CodeComment.cs
- ClientTarget.cs
- ChangeDirector.cs
- OutputWindow.cs
- BitmapDecoder.cs
- BoundPropertyEntry.cs
- DataGridDetailsPresenterAutomationPeer.cs
- DelimitedListTraceListener.cs
- UriExt.cs
- codemethodreferenceexpression.cs
- OperationAbortedException.cs
- DynamicControlParameter.cs
- EdmPropertyAttribute.cs
- PeerInputChannel.cs
- KeyedCollection.cs
- httpserverutility.cs
- MemberMaps.cs
- RoutedUICommand.cs
- WebEvents.cs
- TextProperties.cs
- SQLChars.cs
- CompositeDataBoundControl.cs
- PartitionResolver.cs
- MdiWindowListStrip.cs
- WebPartMenuStyle.cs
- PointIndependentAnimationStorage.cs
- TemplateGroupCollection.cs
- _ListenerAsyncResult.cs
- TreeViewEvent.cs
- QilReference.cs
- DesignerForm.cs
- FormClosingEvent.cs
- MethodResolver.cs
- RequestCache.cs
- DynamicILGenerator.cs
- BigInt.cs
- WebZone.cs
- IIS7WorkerRequest.cs
- VectorAnimation.cs
- UnmanagedMemoryStream.cs
- MetadataCollection.cs
- DispatcherTimer.cs
- DataGridRowClipboardEventArgs.cs
- HtmlTableCell.cs
- ToolbarAUtomationPeer.cs
- NamespaceCollection.cs
- ListViewGroup.cs
- DataGridSortingEventArgs.cs
- ColorContext.cs
- OleDbConnection.cs
- WebServiceResponseDesigner.cs
- DataPagerFieldCollection.cs
- PropertyFilter.cs
- CngKey.cs
- MembershipSection.cs
- WebMessageEncoderFactory.cs
- MediaTimeline.cs
- StaticResourceExtension.cs
- Setter.cs
- PresentationAppDomainManager.cs
- ReverseInheritProperty.cs
- DataObjectAttribute.cs
- SafeRightsManagementHandle.cs
- XomlCompilerHelpers.cs
- UxThemeWrapper.cs
- MachineKeySection.cs
- LinkedResourceCollection.cs
- CurrentChangingEventArgs.cs
- CommandManager.cs
- Vector3DCollectionConverter.cs
- TransformerInfo.cs
- WindowsNonControl.cs
- TextAnchor.cs
- Icon.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- CodeGen.cs
- SkipStoryboardToFill.cs
- PersonalizationAdministration.cs
- MulticastNotSupportedException.cs
- TextFormatter.cs
- MachineKeySection.cs
- SimpleNameService.cs
- HMACMD5.cs
- Int32CollectionConverter.cs
- RewritingPass.cs
- Expressions.cs
- Label.cs
- PathGradientBrush.cs
- SortDescription.cs