Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / Util / DesignerForm.cs / 1 / DesignerForm.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design.Util { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Design; using System.Diagnostics; using System.Drawing; using System.Globalization; using System.Windows.Forms; using System.Windows.Forms.Design; /* */ ////// Represents a form used by a designer. /// internal abstract class DesignerForm : Form { private const int SC_CONTEXTHELP = 0xF180; private const int WM_SYSCOMMAND = 0x0112; private IServiceProvider _serviceProvider; private bool _firstActivate; #if DEBUG private bool _formInitialized; #endif ////// Creates a new DesignerForm with a given service provider. /// protected DesignerForm(IServiceProvider serviceProvider) { Debug.Assert(serviceProvider != null); _serviceProvider = serviceProvider; _firstActivate = true; } ////// The service provider for the form. /// protected internal IServiceProvider ServiceProvider { get { return _serviceProvider; } } ////// Frees up resources. /// protected override void Dispose(bool disposing) { if (disposing) { _serviceProvider = null; } base.Dispose(disposing); } protected void InitializeForm() { Font dialogFont = UIServiceHelper.GetDialogFont(ServiceProvider); if (dialogFont != null) { Font = dialogFont; } // Set RightToLeft mode based on resource file string rtlText = SR.GetString(SR.RTL); if (!String.Equals(rtlText, "RTL_False", StringComparison.Ordinal)) { RightToLeft = RightToLeft.Yes; RightToLeftLayout = true; } #pragma warning disable 618 AutoScaleBaseSize = new System.Drawing.Size(5, 14); #pragma warning restore 618 HelpButton = true; MinimizeBox = false; MaximizeBox = false; ShowIcon = false; ShowInTaskbar = false; StartPosition = System.Windows.Forms.FormStartPosition.CenterParent; #if DEBUG _formInitialized = true; #endif } ////// Gets a service of the desired type. Returns null if the service does not exist or there is no service provider. /// protected override object GetService(Type serviceType) { if (_serviceProvider != null) { return _serviceProvider.GetService(serviceType); } return null; } protected override void OnActivated(EventArgs e) { base.OnActivated(e); if (_firstActivate) { _firstActivate = false; OnInitialActivated(e); } } ////// Returns the help topic for the form. Consult with your UE contact on /// what the appropriate help topic is for your dialog. /// protected abstract string HelpTopic { get; } protected sealed override void OnHelpRequested(HelpEventArgs hevent) { ShowHelp(); hevent.Handled = true; } ////// Raised upon first activation of the form. /// /// protected virtual void OnInitialActivated(EventArgs e) { #if DEBUG Debug.Assert(_formInitialized, "All classes deriving from DesignerForm must call InitializeForm() from within InitializeComponent()."); #endif } ////// Launches the help for this form. /// private void ShowHelp() { if (ServiceProvider != null) { IHelpService helpService = (IHelpService)ServiceProvider.GetService(typeof(IHelpService)); if (helpService != null) { helpService.ShowHelpFromKeyword(HelpTopic); } } } ////// Overridden to reroute the context-help button to our own handler. /// protected override void WndProc(ref Message m) { if ((m.Msg == WM_SYSCOMMAND) && ((int)m.WParam == SC_CONTEXTHELP)) { ShowHelp(); return; } else { base.WndProc(ref m); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
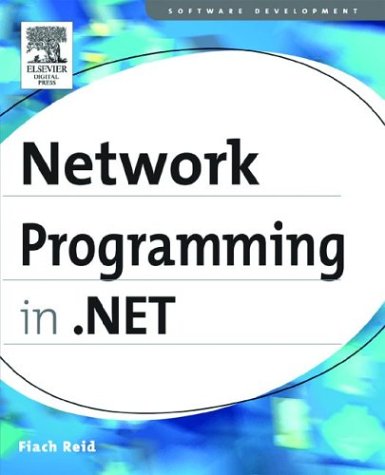
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CompositeFontFamily.cs
- DataGridViewCell.cs
- ItemDragEvent.cs
- ProjectedWrapper.cs
- WebPartCatalogAddVerb.cs
- XmlNodeChangedEventManager.cs
- FullTrustAssembliesSection.cs
- DocumentAutomationPeer.cs
- ProcessHostServerConfig.cs
- DbReferenceCollection.cs
- COM2Enum.cs
- DbConnectionPoolOptions.cs
- Popup.cs
- IsolationInterop.cs
- RandomNumberGenerator.cs
- ProjectionAnalyzer.cs
- DecimalKeyFrameCollection.cs
- SafeRegistryKey.cs
- TileBrush.cs
- OperationAbortedException.cs
- UITypeEditors.cs
- ConnectionPointGlyph.cs
- TextRangeEditTables.cs
- DataColumnChangeEvent.cs
- SystemResourceHost.cs
- Vector3DAnimation.cs
- ReturnValue.cs
- ProviderConnectionPoint.cs
- EntryWrittenEventArgs.cs
- ControlPropertyNameConverter.cs
- WebPartUserCapability.cs
- DesignerWithHeader.cs
- ExpressionBuilderCollection.cs
- BaseCollection.cs
- NullReferenceException.cs
- SymbolUsageManager.cs
- CommandBindingCollection.cs
- ModelItemDictionaryImpl.cs
- ResXResourceReader.cs
- ProcessHostServerConfig.cs
- UriWriter.cs
- TreeViewImageIndexConverter.cs
- UrlMapping.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- RadioButton.cs
- GB18030Encoding.cs
- ActivitySurrogate.cs
- TextTreeInsertUndoUnit.cs
- PeerNameRecord.cs
- EncoderParameter.cs
- FontSourceCollection.cs
- GestureRecognitionResult.cs
- SmtpNegotiateAuthenticationModule.cs
- Canvas.cs
- SoapSchemaImporter.cs
- SoapExtensionTypeElement.cs
- Double.cs
- ResolveResponseInfo.cs
- FunctionQuery.cs
- FrameworkPropertyMetadata.cs
- TypeBuilderInstantiation.cs
- GroupStyle.cs
- SettingsPropertyCollection.cs
- DbBuffer.cs
- MimeFormatExtensions.cs
- WindowsStatic.cs
- Empty.cs
- HScrollProperties.cs
- DataListItemCollection.cs
- ColumnHeaderConverter.cs
- ColorPalette.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- Latin1Encoding.cs
- WhileDesigner.xaml.cs
- TextParentUndoUnit.cs
- WebServiceEnumData.cs
- TextServicesContext.cs
- CollectionViewSource.cs
- TemplateFactory.cs
- AtlasWeb.Designer.cs
- DockPanel.cs
- NegotiateStream.cs
- SizeChangedEventArgs.cs
- UserInitiatedRoutedEventPermissionAttribute.cs
- BrowserTree.cs
- XmlName.cs
- PageAction.cs
- ParameterCollection.cs
- RegexCode.cs
- SqlNamer.cs
- XmlObjectSerializerWriteContextComplexJson.cs
- StatusInfoItem.cs
- ColorDialog.cs
- CreateUserWizardDesigner.cs
- Dictionary.cs
- HostingPreferredMapPath.cs
- XmlSchemaExporter.cs
- TagPrefixAttribute.cs
- OleDbCommandBuilder.cs
- CodeParameterDeclarationExpression.cs