Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / XmlUtils / System / Xml / Xsl / XmlILCommand.cs / 1305376 / XmlILCommand.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //http://webdata/xml/specs/querylowlevel.xml //----------------------------------------------------------------------------- using System.Collections; using System.Diagnostics; using System.IO; using System.Xml.XPath; using System.Xml.Xsl.Runtime; using System.Runtime.Versioning; namespace System.Xml.Xsl { ////// This is the executable command generated by the XmlILGenerator. /// internal class XmlILCommand { private ExecuteDelegate delExec; private XmlQueryStaticData staticData; ////// Constructor. /// public XmlILCommand(ExecuteDelegate delExec, XmlQueryStaticData staticData) { Debug.Assert(delExec != null && staticData != null); this.delExec = delExec; this.staticData = staticData; } ////// Return execute delegate. /// public ExecuteDelegate ExecuteDelegate { get { return delExec; } } ////// Return query static data required by the runtime. /// public XmlQueryStaticData StaticData { get { return staticData; } } #if false ////// Default serialization options that will be used if the user does not supply an XmlWriter /// at execution time. /// public override XmlWriterSettings DefaultWriterSettings { get { return this.staticData.DefaultWriterSettings; } } ////// Default document as XPathNavigator. /// public override void Execute(IXPathNavigable contextDocument, XmlResolver dataSources, XsltArgumentList argumentList, XmlWriter results) { if (results == null) throw new ArgumentNullException("results"); if (contextDocument != null) Execute(contextDocument.CreateNavigator(), dataSources, argumentList, results, false); else Execute(null, dataSources, argumentList, results, false); } ////// Default document as XPathNavigator. /// public override void Execute(IXPathNavigable contextDocument, XmlResolver dataSources, XsltArgumentList argumentList, TextWriter results) { if (results == null) throw new ArgumentNullException("results"); Execute(contextDocument, dataSources, argumentList, XmlWriter.Create(results, this.staticData.DefaultWriterSettings)); } ////// Default document as XPathNavigator. /// public override void Execute(IXPathNavigable contextDocument, XmlResolver dataSources, XsltArgumentList argumentList, Stream results) { if (results == null) throw new ArgumentNullException("results"); Execute(contextDocument, dataSources, argumentList, XmlWriter.Create(results, this.staticData.DefaultWriterSettings)); } ////// Executes the query by accessing datasources via the XmlResolver and using run-time parameters /// as provided by the XsltArgumentList. The default document is mapped into the XmlResolver with the /// provided name. The results are output to the provided XmlWriter. /// public void Execute(string contextDocumentUri, XmlResolver dataSources, XsltArgumentList argumentList, XmlWriter results) { if (results == null) throw new ArgumentNullException("results"); Execute(contextDocumentUri, dataSources, argumentList, results, false); } #endif ////// Executes the query by accessing datasources via the XmlResolver and using /// run-time parameters as provided by the XsltArgumentList. The default document /// is mapped into the XmlResolver with the provided name. The results are returned /// as an IList. /// [ResourceConsumption(ResourceScope.Machine)] [ResourceExposure(ResourceScope.Machine)] public IList Evaluate(string contextDocumentUri, XmlResolver dataSources, XsltArgumentList argumentList) { XmlCachedSequenceWriter seqwrt = new XmlCachedSequenceWriter(); Execute(contextDocumentUri, dataSources, argumentList, seqwrt); return seqwrt.ResultSequence; } #if false ////// Executes the query by accessing datasources via the XmlResolver and using run-time parameters /// as provided by the XsltArgumentList. The default document is mapped into the XmlResolver with the /// provided name. The results are output to the provided XmlWriter. /// public override void Execute(XmlReader contextDocument, XmlResolver dataSources, XsltArgumentList argumentList, XmlWriter results) { if (results == null) throw new ArgumentNullException("results"); Execute(contextDocument, dataSources, argumentList, results, false); } ////// Executes the query by accessing datasources via the XmlResolver and using run-time parameters /// as provided by the XsltArgumentList. The default document is mapped into the XmlResolver with the /// provided name. The results are output to the provided TextWriter. /// public override void Execute(XmlReader contextDocument, XmlResolver dataSources, XsltArgumentList argumentList, TextWriter results) { if (results == null) throw new ArgumentNullException("results"); Execute(contextDocument, dataSources, argumentList, XmlWriter.Create(results, this.staticData.DefaultWriterSettings), true); } ////// Executes the query by accessing datasources via the XmlResolver and using run-time parameters /// as provided by the XsltArgumentList. The default document is mapped into the XmlResolver with the /// provided name. The results are output to the provided Stream. /// public override void Execute(XmlReader contextDocument, XmlResolver dataSources, XsltArgumentList argumentList, Stream results) { if (results == null) throw new ArgumentNullException("results"); Execute(contextDocument, dataSources, argumentList, XmlWriter.Create(results, this.staticData.DefaultWriterSettings), true); } ////// Executes the query by accessing datasources via the XmlResolver and using /// run-time parameters as provided by the XsltArgumentList. The default document /// is mapped into the XmlResolver with the provided name. The results are returned /// as an IList. /// public override IList Evaluate(XmlReader contextDocument, XmlResolver dataSources, XsltArgumentList argumentList) { XmlCachedSequenceWriter seqwrt = new XmlCachedSequenceWriter(); Execute(contextDocument, dataSources, argumentList, seqwrt); return seqwrt.ResultSequence; } #endif ////// Execute the dynamic assembly generated by the XmlILGenerator. /// [ResourceConsumption(ResourceScope.Machine)] [ResourceExposure(ResourceScope.Machine)] public void Execute(object defaultDocument, XmlResolver dataSources, XsltArgumentList argumentList, XmlWriter writer) { try { // Try to extract a RawWriter XmlWellFormedWriter wellFormedWriter = writer as XmlWellFormedWriter; if (wellFormedWriter != null && wellFormedWriter.RawWriter != null && wellFormedWriter.WriteState == WriteState.Start && wellFormedWriter.Settings.ConformanceLevel != ConformanceLevel.Document) { // Extracted RawWriter from WellFormedWriter Execute(defaultDocument, dataSources, argumentList, new XmlMergeSequenceWriter(wellFormedWriter.RawWriter)); } else { // Wrap Writer in RawWriter Execute(defaultDocument, dataSources, argumentList, new XmlMergeSequenceWriter(new XmlRawWriterWrapper(writer))); } } finally { writer.Flush(); } } ////// Execute the dynamic assembly generated by the XmlILGenerator. /// [ResourceConsumption(ResourceScope.Machine)] [ResourceExposure(ResourceScope.Machine)] private void Execute(object defaultDocument, XmlResolver dataSources, XsltArgumentList argumentList, XmlSequenceWriter results) { Debug.Assert(results != null); // Ensure that dataSources is always non-null if (dataSources == null) dataSources = XmlNullResolver.Singleton; this.delExec(new XmlQueryRuntime(this.staticData, defaultDocument, dataSources, argumentList, results)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //http://webdata/xml/specs/querylowlevel.xml //----------------------------------------------------------------------------- using System.Collections; using System.Diagnostics; using System.IO; using System.Xml.XPath; using System.Xml.Xsl.Runtime; using System.Runtime.Versioning; namespace System.Xml.Xsl { ////// This is the executable command generated by the XmlILGenerator. /// internal class XmlILCommand { private ExecuteDelegate delExec; private XmlQueryStaticData staticData; ////// Constructor. /// public XmlILCommand(ExecuteDelegate delExec, XmlQueryStaticData staticData) { Debug.Assert(delExec != null && staticData != null); this.delExec = delExec; this.staticData = staticData; } ////// Return execute delegate. /// public ExecuteDelegate ExecuteDelegate { get { return delExec; } } ////// Return query static data required by the runtime. /// public XmlQueryStaticData StaticData { get { return staticData; } } #if false ////// Default serialization options that will be used if the user does not supply an XmlWriter /// at execution time. /// public override XmlWriterSettings DefaultWriterSettings { get { return this.staticData.DefaultWriterSettings; } } ////// Default document as XPathNavigator. /// public override void Execute(IXPathNavigable contextDocument, XmlResolver dataSources, XsltArgumentList argumentList, XmlWriter results) { if (results == null) throw new ArgumentNullException("results"); if (contextDocument != null) Execute(contextDocument.CreateNavigator(), dataSources, argumentList, results, false); else Execute(null, dataSources, argumentList, results, false); } ////// Default document as XPathNavigator. /// public override void Execute(IXPathNavigable contextDocument, XmlResolver dataSources, XsltArgumentList argumentList, TextWriter results) { if (results == null) throw new ArgumentNullException("results"); Execute(contextDocument, dataSources, argumentList, XmlWriter.Create(results, this.staticData.DefaultWriterSettings)); } ////// Default document as XPathNavigator. /// public override void Execute(IXPathNavigable contextDocument, XmlResolver dataSources, XsltArgumentList argumentList, Stream results) { if (results == null) throw new ArgumentNullException("results"); Execute(contextDocument, dataSources, argumentList, XmlWriter.Create(results, this.staticData.DefaultWriterSettings)); } ////// Executes the query by accessing datasources via the XmlResolver and using run-time parameters /// as provided by the XsltArgumentList. The default document is mapped into the XmlResolver with the /// provided name. The results are output to the provided XmlWriter. /// public void Execute(string contextDocumentUri, XmlResolver dataSources, XsltArgumentList argumentList, XmlWriter results) { if (results == null) throw new ArgumentNullException("results"); Execute(contextDocumentUri, dataSources, argumentList, results, false); } #endif ////// Executes the query by accessing datasources via the XmlResolver and using /// run-time parameters as provided by the XsltArgumentList. The default document /// is mapped into the XmlResolver with the provided name. The results are returned /// as an IList. /// [ResourceConsumption(ResourceScope.Machine)] [ResourceExposure(ResourceScope.Machine)] public IList Evaluate(string contextDocumentUri, XmlResolver dataSources, XsltArgumentList argumentList) { XmlCachedSequenceWriter seqwrt = new XmlCachedSequenceWriter(); Execute(contextDocumentUri, dataSources, argumentList, seqwrt); return seqwrt.ResultSequence; } #if false ////// Executes the query by accessing datasources via the XmlResolver and using run-time parameters /// as provided by the XsltArgumentList. The default document is mapped into the XmlResolver with the /// provided name. The results are output to the provided XmlWriter. /// public override void Execute(XmlReader contextDocument, XmlResolver dataSources, XsltArgumentList argumentList, XmlWriter results) { if (results == null) throw new ArgumentNullException("results"); Execute(contextDocument, dataSources, argumentList, results, false); } ////// Executes the query by accessing datasources via the XmlResolver and using run-time parameters /// as provided by the XsltArgumentList. The default document is mapped into the XmlResolver with the /// provided name. The results are output to the provided TextWriter. /// public override void Execute(XmlReader contextDocument, XmlResolver dataSources, XsltArgumentList argumentList, TextWriter results) { if (results == null) throw new ArgumentNullException("results"); Execute(contextDocument, dataSources, argumentList, XmlWriter.Create(results, this.staticData.DefaultWriterSettings), true); } ////// Executes the query by accessing datasources via the XmlResolver and using run-time parameters /// as provided by the XsltArgumentList. The default document is mapped into the XmlResolver with the /// provided name. The results are output to the provided Stream. /// public override void Execute(XmlReader contextDocument, XmlResolver dataSources, XsltArgumentList argumentList, Stream results) { if (results == null) throw new ArgumentNullException("results"); Execute(contextDocument, dataSources, argumentList, XmlWriter.Create(results, this.staticData.DefaultWriterSettings), true); } ////// Executes the query by accessing datasources via the XmlResolver and using /// run-time parameters as provided by the XsltArgumentList. The default document /// is mapped into the XmlResolver with the provided name. The results are returned /// as an IList. /// public override IList Evaluate(XmlReader contextDocument, XmlResolver dataSources, XsltArgumentList argumentList) { XmlCachedSequenceWriter seqwrt = new XmlCachedSequenceWriter(); Execute(contextDocument, dataSources, argumentList, seqwrt); return seqwrt.ResultSequence; } #endif ////// Execute the dynamic assembly generated by the XmlILGenerator. /// [ResourceConsumption(ResourceScope.Machine)] [ResourceExposure(ResourceScope.Machine)] public void Execute(object defaultDocument, XmlResolver dataSources, XsltArgumentList argumentList, XmlWriter writer) { try { // Try to extract a RawWriter XmlWellFormedWriter wellFormedWriter = writer as XmlWellFormedWriter; if (wellFormedWriter != null && wellFormedWriter.RawWriter != null && wellFormedWriter.WriteState == WriteState.Start && wellFormedWriter.Settings.ConformanceLevel != ConformanceLevel.Document) { // Extracted RawWriter from WellFormedWriter Execute(defaultDocument, dataSources, argumentList, new XmlMergeSequenceWriter(wellFormedWriter.RawWriter)); } else { // Wrap Writer in RawWriter Execute(defaultDocument, dataSources, argumentList, new XmlMergeSequenceWriter(new XmlRawWriterWrapper(writer))); } } finally { writer.Flush(); } } ////// Execute the dynamic assembly generated by the XmlILGenerator. /// [ResourceConsumption(ResourceScope.Machine)] [ResourceExposure(ResourceScope.Machine)] private void Execute(object defaultDocument, XmlResolver dataSources, XsltArgumentList argumentList, XmlSequenceWriter results) { Debug.Assert(results != null); // Ensure that dataSources is always non-null if (dataSources == null) dataSources = XmlNullResolver.Singleton; this.delExec(new XmlQueryRuntime(this.staticData, defaultDocument, dataSources, argumentList, results)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
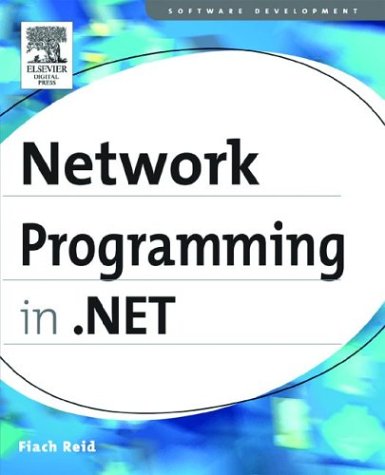
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WorkflowExecutor.cs
- WebConfigurationFileMap.cs
- ConnectionProviderAttribute.cs
- RequestQueryProcessor.cs
- ConfigXmlDocument.cs
- SqlError.cs
- ButtonColumn.cs
- MDIControlStrip.cs
- FormatException.cs
- SnapLine.cs
- DataStreams.cs
- StorageFunctionMapping.cs
- UnmanagedMemoryAccessor.cs
- HwndSourceKeyboardInputSite.cs
- ModelService.cs
- ProjectionPruner.cs
- HttpCachePolicyWrapper.cs
- SqlDataSourceParameterParser.cs
- DPAPIProtectedConfigurationProvider.cs
- ServiceBusyException.cs
- IndexedGlyphRun.cs
- SqlBulkCopyColumnMappingCollection.cs
- EntitySetDataBindingList.cs
- DbParameterCollectionHelper.cs
- WsdlExporter.cs
- ServiceDescriptionSerializer.cs
- UniformGrid.cs
- ServiceMetadataBehavior.cs
- SQLBoolean.cs
- Emitter.cs
- WebPartManager.cs
- ServiceMetadataContractBehavior.cs
- BCLDebug.cs
- Parameter.cs
- LoginUtil.cs
- DoWorkEventArgs.cs
- ResXFileRef.cs
- CustomAttribute.cs
- CodeAttributeArgumentCollection.cs
- SimpleHandlerBuildProvider.cs
- EntityDataSourceSelectedEventArgs.cs
- GrammarBuilderWildcard.cs
- NavigateUrlConverter.cs
- Enlistment.cs
- DataServiceSaveChangesEventArgs.cs
- SettingsPropertyCollection.cs
- FilterEventArgs.cs
- DataGridClipboardCellContent.cs
- QueryOpeningEnumerator.cs
- ExtenderProvidedPropertyAttribute.cs
- RectAnimation.cs
- XmlNodeChangedEventManager.cs
- ExplicitDiscriminatorMap.cs
- AnnotationDocumentPaginator.cs
- FormViewPageEventArgs.cs
- EntitySet.cs
- JoinGraph.cs
- ReadOnlyDataSource.cs
- DecimalSumAggregationOperator.cs
- EditCommandColumn.cs
- Exceptions.cs
- COM2TypeInfoProcessor.cs
- UIElementHelper.cs
- WebPartEditorOkVerb.cs
- HelpFileFileNameEditor.cs
- InternalRelationshipCollection.cs
- ListViewGroup.cs
- RegexCompilationInfo.cs
- ConnectionsZoneDesigner.cs
- HeaderCollection.cs
- CallbackWrapper.cs
- SBCSCodePageEncoding.cs
- Style.cs
- HtmlShimManager.cs
- UnknownWrapper.cs
- DependencySource.cs
- SiteIdentityPermission.cs
- Avt.cs
- DescendantBaseQuery.cs
- SerialErrors.cs
- RoutedEventValueSerializer.cs
- SponsorHelper.cs
- Rijndael.cs
- ExtensionDataObject.cs
- CodeDomSerializer.cs
- ADMembershipUser.cs
- BatchParser.cs
- Int64AnimationUsingKeyFrames.cs
- BCLDebug.cs
- DataViewListener.cs
- XmlAutoDetectWriter.cs
- ResourceReferenceKeyNotFoundException.cs
- InstanceDataCollectionCollection.cs
- MaskedTextBox.cs
- DocumentPageHost.cs
- dtdvalidator.cs
- ControlValuePropertyAttribute.cs
- AppDomainUnloadedException.cs
- CodeDOMProvider.cs
- MyContact.cs