Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / MS / Internal / Data / XmlDataCollection.cs / 1 / XmlDataCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: Data collection produced by an XmlDataProvider // // Specs: http://avalon/connecteddata/M5%20Specs/IDataCollection.mht // //--------------------------------------------------------------------------- using System.Xml; using System.Collections.ObjectModel; using System.Collections.Specialized; using System.Windows.Data; using System.Windows.Markup; namespace MS.Internal.Data { ////// Implementation of a data collection based on ArrayList, /// implementing INotifyCollectionChanged to notify listeners /// when items get added, removed or the whole list is refreshed. /// internal class XmlDataCollection : ReadOnlyObservableCollection{ //----------------------------------------------------- // // Constructors // //----------------------------------------------------- /// /// Initializes a new instance of XmlDataCollection that is empty and has the specified initial capacity. /// /// Parent Xml Data Source internal XmlDataCollection(XmlDataProvider xmlDataProvider) : base(new ObservableCollection()) { _xds = xmlDataProvider; } //------------------------------------------------------ // // Internal Properties // //----------------------------------------------------- /// /// XmlNamespaceManager property, XmlNamespaceManager used for executing XPath queries. /// internal XmlNamespaceManager XmlNamespaceManager { get { if (_nsMgr == null && _xds != null) _nsMgr = _xds.XmlNamespaceManager; return _nsMgr; } set { _nsMgr = value; } } internal XmlDataProvider ParentXmlDataProvider { get { return _xds; } } //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ // return true if the counts are different or the identity of the nodes have changed internal bool CollectionHasChanged(XmlNodeList nodes) { int count = this.Count; if (count != nodes.Count) return true; for (int i = 0; i < count; ++i) { if (this[i] != nodes[i]) return true; } return false; } // Update the collection using new query results internal void SynchronizeCollection(XmlNodeList nodes) { if (nodes == null) { Items.Clear(); return; } int i = 0, j; while (i < this.Count && i < nodes.Count) { if (this[i] != nodes[i]) { // starting after current node, see if the old node is still in the new list. for (j = i + 1; j < nodes.Count; ++j) { if (this[i] == nodes[j]) { break; } } if (j < nodes.Count) { // the node from existing collection is found at [j] in the new collection; // this means the node(s) [i ~ j-1] in new collection should be inserted. while (i < j) { Items.Insert(i, nodes[i]); ++i; } ++i; // advance to next node } else { // the node from existing collection is no longer in // the new collection, delete it. Items.RemoveAt(i); // do not advance to the next node } } else { // nodes are the same; advance to the next node. ++i; } } // Remove any extra nodes left over in the old collection while (i < this.Count) { Items.RemoveAt(i); } // Add any extra new nodes from the new collection while (i < nodes.Count) { Items.Insert(i, nodes[i]); ++i; } } private XmlDataProvider _xds; private XmlNamespaceManager _nsMgr; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: Data collection produced by an XmlDataProvider // // Specs: http://avalon/connecteddata/M5%20Specs/IDataCollection.mht // //--------------------------------------------------------------------------- using System.Xml; using System.Collections.ObjectModel; using System.Collections.Specialized; using System.Windows.Data; using System.Windows.Markup; namespace MS.Internal.Data { ////// Implementation of a data collection based on ArrayList, /// implementing INotifyCollectionChanged to notify listeners /// when items get added, removed or the whole list is refreshed. /// internal class XmlDataCollection : ReadOnlyObservableCollection{ //----------------------------------------------------- // // Constructors // //----------------------------------------------------- /// /// Initializes a new instance of XmlDataCollection that is empty and has the specified initial capacity. /// /// Parent Xml Data Source internal XmlDataCollection(XmlDataProvider xmlDataProvider) : base(new ObservableCollection()) { _xds = xmlDataProvider; } //------------------------------------------------------ // // Internal Properties // //----------------------------------------------------- /// /// XmlNamespaceManager property, XmlNamespaceManager used for executing XPath queries. /// internal XmlNamespaceManager XmlNamespaceManager { get { if (_nsMgr == null && _xds != null) _nsMgr = _xds.XmlNamespaceManager; return _nsMgr; } set { _nsMgr = value; } } internal XmlDataProvider ParentXmlDataProvider { get { return _xds; } } //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ // return true if the counts are different or the identity of the nodes have changed internal bool CollectionHasChanged(XmlNodeList nodes) { int count = this.Count; if (count != nodes.Count) return true; for (int i = 0; i < count; ++i) { if (this[i] != nodes[i]) return true; } return false; } // Update the collection using new query results internal void SynchronizeCollection(XmlNodeList nodes) { if (nodes == null) { Items.Clear(); return; } int i = 0, j; while (i < this.Count && i < nodes.Count) { if (this[i] != nodes[i]) { // starting after current node, see if the old node is still in the new list. for (j = i + 1; j < nodes.Count; ++j) { if (this[i] == nodes[j]) { break; } } if (j < nodes.Count) { // the node from existing collection is found at [j] in the new collection; // this means the node(s) [i ~ j-1] in new collection should be inserted. while (i < j) { Items.Insert(i, nodes[i]); ++i; } ++i; // advance to next node } else { // the node from existing collection is no longer in // the new collection, delete it. Items.RemoveAt(i); // do not advance to the next node } } else { // nodes are the same; advance to the next node. ++i; } } // Remove any extra nodes left over in the old collection while (i < this.Count) { Items.RemoveAt(i); } // Add any extra new nodes from the new collection while (i < nodes.Count) { Items.Insert(i, nodes[i]); ++i; } } private XmlDataProvider _xds; private XmlNamespaceManager _nsMgr; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
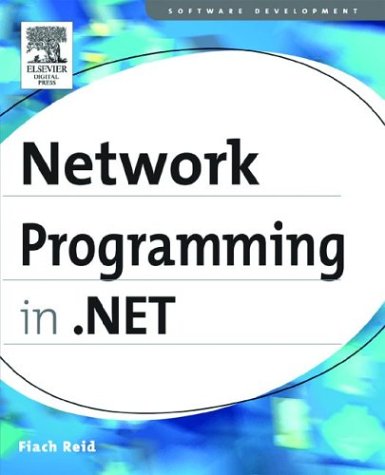
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataAdapter.cs
- DataGridRowEventArgs.cs
- FormDocumentDesigner.cs
- GroupBoxAutomationPeer.cs
- CornerRadius.cs
- SingleAnimationUsingKeyFrames.cs
- SqlUnionizer.cs
- AddingNewEventArgs.cs
- XappLauncher.cs
- XmlSerializerOperationBehavior.cs
- glyphs.cs
- TextParagraphProperties.cs
- BridgeDataRecord.cs
- DoubleLinkListEnumerator.cs
- SqlDataSourceWizardForm.cs
- SynchronizedInputPattern.cs
- MulticastNotSupportedException.cs
- ColorMap.cs
- RoutedEventArgs.cs
- ListBindingConverter.cs
- ISAPIRuntime.cs
- PhysicalFontFamily.cs
- KeyGesture.cs
- UnsafeNativeMethods.cs
- DocumentOrderQuery.cs
- XamlToRtfParser.cs
- TextEffect.cs
- SetIndexBinder.cs
- ServicePoint.cs
- GuidTagList.cs
- WSSecurityOneDotZeroReceiveSecurityHeader.cs
- SqlCharStream.cs
- Matrix.cs
- ButtonDesigner.cs
- RelationshipDetailsCollection.cs
- TransactionsSectionGroup.cs
- ScrollChrome.cs
- PreviewKeyDownEventArgs.cs
- CornerRadius.cs
- WebPartZoneDesigner.cs
- HttpStreamFormatter.cs
- SHA384Managed.cs
- HttpModulesSection.cs
- DataGridViewHeaderCell.cs
- DSASignatureFormatter.cs
- ByteConverter.cs
- isolationinterop.cs
- Cursor.cs
- RowToParametersTransformer.cs
- Recipient.cs
- DetailsViewDeleteEventArgs.cs
- EventSchemaTraceListener.cs
- StructuredProperty.cs
- CodePrimitiveExpression.cs
- UpdatePanelControlTrigger.cs
- HtmlMeta.cs
- SafeBitVector32.cs
- WarningException.cs
- TrustLevel.cs
- SubMenuStyleCollection.cs
- GCHandleCookieTable.cs
- Missing.cs
- PngBitmapEncoder.cs
- ThreadNeutralSemaphore.cs
- ThemeableAttribute.cs
- ZipIOExtraFieldElement.cs
- EndpointIdentityExtension.cs
- DCSafeHandle.cs
- SerializationInfo.cs
- SemanticKeyElement.cs
- HttpStreamFormatter.cs
- EventDescriptorCollection.cs
- OperationSelectorBehavior.cs
- TcpChannelHelper.cs
- formatter.cs
- _NestedMultipleAsyncResult.cs
- XmlValueConverter.cs
- DocumentXmlWriter.cs
- sqlpipe.cs
- SqlConnectionPoolProviderInfo.cs
- Html32TextWriter.cs
- HybridDictionary.cs
- PolygonHotSpot.cs
- WebZoneDesigner.cs
- XpsFixedDocumentReaderWriter.cs
- SafeLocalMemHandle.cs
- SendMailErrorEventArgs.cs
- UrlMappingCollection.cs
- WindowsSlider.cs
- XmlElementCollection.cs
- ValueUnavailableException.cs
- ImageIndexConverter.cs
- SoapSchemaExporter.cs
- XamlToRtfParser.cs
- TimeSpan.cs
- IncrementalReadDecoders.cs
- PixelShader.cs
- OperatingSystem.cs
- XPathNodeList.cs
- UnsafeNativeMethodsMilCoreApi.cs