Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / ColorContextHelper.cs / 1 / ColorContextHelper.cs
//------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2001, 2002, 2003 // // File: ColorContextHelper.cs //----------------------------------------------------------------------------- using System; using System.IO; using System.Runtime.InteropServices; using System.Windows.Media; using System.Windows.Media.Imaging; using MS.Internal; using MS.Win32; using System.Security; using System.Security.Permissions; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Diagnostics; using System.Globalization; using Microsoft.Win32.SafeHandles; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods; namespace System.Windows.Media { #region SafeProfileHandle internal class SafeProfileHandle : SafeHandleZeroOrMinusOneIsInvalid { ////// Use this constructor if the handle exists at construction time. /// ////// Critical: The ctor of the base class requires SecurityPermission /// [SecurityCritical] internal SafeProfileHandle() : base(true) { } ////// Use this constructor if the handle exists at construction time. /// ////// Critical: The ctor of the base class requires SecurityPermission /// This code calls SetHandle /// [SecurityCritical] internal SafeProfileHandle(IntPtr profile) : base(true) { SetHandle(profile); } ////// Critical - calls unmanaged code, not treat as safe because you must /// validate that handle is a valid color context handle. /// [SecurityCritical] protected override bool ReleaseHandle() { if (!UnsafeNativeMethods.Mscms.CloseColorProfile(handle)) { HRESULT.Check(Marshal.GetHRForLastWin32Error()); } return true; } } #endregion #region ColorContextHelper ////// Class to call into MSCMS color context APIs /// internal class ColorContextHelper { /// Constructor internal ColorContextHelper() { } /// Opens a color profile ////// SecurityCritical: Calls unmanaged code, accepts InPtr/unverified data. /// [SecurityCritical] internal void OpenColorProfile(IntPtr pProfile) { // No need to get rid of the old handle as it will get GC'ed _profileHandle = UnsafeNativeMethods.Mscms.OpenColorProfile( pProfile, NativeMethods.PROFILE_READ, // DesiredAccess NativeMethods.FILE_SHARE_READ, // ShareMode NativeMethods.OPEN_EXISTING // CreationMode ); if (_profileHandle == null || _profileHandle.IsInvalid) { HRESULT.Check(Marshal.GetHRForLastWin32Error()); } } /// Retrieves the profile header ////// SecurityCritical: Calls unmanaged code, accepts InPtr/unverified data. /// [SecurityCritical] internal void GetColorProfileHeader(IntPtr pHeader) { if (_profileHandle == null || _profileHandle.IsInvalid) { throw new InvalidOperationException(SR.Get(SRID.Image_ColorContextInvalid)); } HRESULT.Check(UnsafeNativeMethods.Mscms.GetColorProfileHeader(_profileHandle, pHeader)); } /// Retrieves the color profile from handle ////// SecurityCritical: Calls unmanaged code, accepts InPtr/unverified data. /// [SecurityCritical] internal void GetColorProfileFromHandle(IntPtr pBuffer, IntPtr pdwSize) { if (_profileHandle == null || _profileHandle.IsInvalid) { throw new InvalidOperationException(SR.Get(SRID.Image_ColorContextInvalid)); } HRESULT.Check(UnsafeNativeMethods.Mscms.GetColorProfileFromHandle(_profileHandle, pBuffer, pdwSize)); } ////// Critical - Accesses critical resource _profileHandle /// TreatAsSafe - No inputs and just checks if SafeHandle is valid or not. /// internal bool IsInvalid { [SecurityCritical, SecurityTreatAsSafe] get { return (_profileHandle == null || _profileHandle.IsInvalid); } } ////// ProfileHandle /// ////// SecurityCritical: This comes out of an elevation needs to be critical and tracked. /// internal SafeProfileHandle ProfileHandle { [SecurityCritical] get { return _profileHandle; } } #region Data members ////// SecurityCritical: This comes out of an elevation needs to be critical and tracked. /// [SecurityCritical] SafeProfileHandle _profileHandle; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2001, 2002, 2003 // // File: ColorContextHelper.cs //----------------------------------------------------------------------------- using System; using System.IO; using System.Runtime.InteropServices; using System.Windows.Media; using System.Windows.Media.Imaging; using MS.Internal; using MS.Win32; using System.Security; using System.Security.Permissions; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Diagnostics; using System.Globalization; using Microsoft.Win32.SafeHandles; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods; namespace System.Windows.Media { #region SafeProfileHandle internal class SafeProfileHandle : SafeHandleZeroOrMinusOneIsInvalid { ////// Use this constructor if the handle exists at construction time. /// ////// Critical: The ctor of the base class requires SecurityPermission /// [SecurityCritical] internal SafeProfileHandle() : base(true) { } ////// Use this constructor if the handle exists at construction time. /// ////// Critical: The ctor of the base class requires SecurityPermission /// This code calls SetHandle /// [SecurityCritical] internal SafeProfileHandle(IntPtr profile) : base(true) { SetHandle(profile); } ////// Critical - calls unmanaged code, not treat as safe because you must /// validate that handle is a valid color context handle. /// [SecurityCritical] protected override bool ReleaseHandle() { if (!UnsafeNativeMethods.Mscms.CloseColorProfile(handle)) { HRESULT.Check(Marshal.GetHRForLastWin32Error()); } return true; } } #endregion #region ColorContextHelper ////// Class to call into MSCMS color context APIs /// internal class ColorContextHelper { /// Constructor internal ColorContextHelper() { } /// Opens a color profile ////// SecurityCritical: Calls unmanaged code, accepts InPtr/unverified data. /// [SecurityCritical] internal void OpenColorProfile(IntPtr pProfile) { // No need to get rid of the old handle as it will get GC'ed _profileHandle = UnsafeNativeMethods.Mscms.OpenColorProfile( pProfile, NativeMethods.PROFILE_READ, // DesiredAccess NativeMethods.FILE_SHARE_READ, // ShareMode NativeMethods.OPEN_EXISTING // CreationMode ); if (_profileHandle == null || _profileHandle.IsInvalid) { HRESULT.Check(Marshal.GetHRForLastWin32Error()); } } /// Retrieves the profile header ////// SecurityCritical: Calls unmanaged code, accepts InPtr/unverified data. /// [SecurityCritical] internal void GetColorProfileHeader(IntPtr pHeader) { if (_profileHandle == null || _profileHandle.IsInvalid) { throw new InvalidOperationException(SR.Get(SRID.Image_ColorContextInvalid)); } HRESULT.Check(UnsafeNativeMethods.Mscms.GetColorProfileHeader(_profileHandle, pHeader)); } /// Retrieves the color profile from handle ////// SecurityCritical: Calls unmanaged code, accepts InPtr/unverified data. /// [SecurityCritical] internal void GetColorProfileFromHandle(IntPtr pBuffer, IntPtr pdwSize) { if (_profileHandle == null || _profileHandle.IsInvalid) { throw new InvalidOperationException(SR.Get(SRID.Image_ColorContextInvalid)); } HRESULT.Check(UnsafeNativeMethods.Mscms.GetColorProfileFromHandle(_profileHandle, pBuffer, pdwSize)); } ////// Critical - Accesses critical resource _profileHandle /// TreatAsSafe - No inputs and just checks if SafeHandle is valid or not. /// internal bool IsInvalid { [SecurityCritical, SecurityTreatAsSafe] get { return (_profileHandle == null || _profileHandle.IsInvalid); } } ////// ProfileHandle /// ////// SecurityCritical: This comes out of an elevation needs to be critical and tracked. /// internal SafeProfileHandle ProfileHandle { [SecurityCritical] get { return _profileHandle; } } #region Data members ////// SecurityCritical: This comes out of an elevation needs to be critical and tracked. /// [SecurityCritical] SafeProfileHandle _profileHandle; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
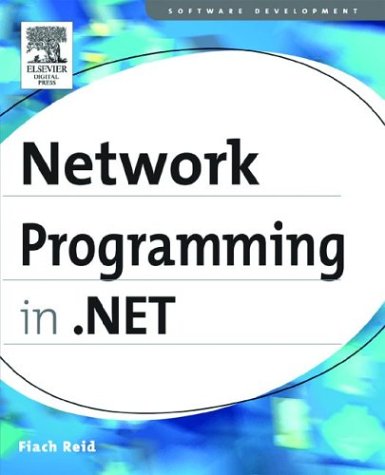
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PropertyCollection.cs
- configsystem.cs
- ExceptionWrapper.cs
- ScrollBarRenderer.cs
- WindowsFormsHelpers.cs
- NamespaceMapping.cs
- ArgIterator.cs
- MethodBody.cs
- ApplicationActivator.cs
- ExpressionBuilderContext.cs
- ChameleonKey.cs
- DataBoundControlActionList.cs
- BindingExpressionUncommonField.cs
- SimpleHandlerFactory.cs
- TextReturnReader.cs
- StringUtil.cs
- ServiceModelEnhancedConfigurationElementCollection.cs
- Roles.cs
- TypeCollectionPropertyEditor.cs
- ParagraphVisual.cs
- InternalConfigSettingsFactory.cs
- TypeInitializationException.cs
- Int32Converter.cs
- EnumerableWrapperWeakToStrong.cs
- XmlDictionaryReaderQuotas.cs
- TableCellCollection.cs
- HiddenFieldDesigner.cs
- BrowserCapabilitiesFactory.cs
- CroppedBitmap.cs
- DesignerExtenders.cs
- CompilationUtil.cs
- LongPath.cs
- SelectedGridItemChangedEvent.cs
- ElapsedEventArgs.cs
- ReadOnlyObservableCollection.cs
- ActivityCollectionMarkupSerializer.cs
- XmlReaderDelegator.cs
- CLRBindingWorker.cs
- UnsafeNativeMethods.cs
- TreeNodeBindingDepthConverter.cs
- LingerOption.cs
- SqlSupersetValidator.cs
- CommandBindingCollection.cs
- WinEventTracker.cs
- Rotation3D.cs
- State.cs
- RemotingException.cs
- ColorBlend.cs
- ZipIOLocalFileBlock.cs
- SystemTcpStatistics.cs
- _Win32.cs
- ModelProperty.cs
- Icon.cs
- ParagraphVisual.cs
- ExtensionWindowHeader.cs
- EFTableProvider.cs
- ImageFormatConverter.cs
- WrapperEqualityComparer.cs
- While.cs
- DeferredReference.cs
- XsltContext.cs
- DataViewManagerListItemTypeDescriptor.cs
- HashMembershipCondition.cs
- DataGridPageChangedEventArgs.cs
- Brush.cs
- PersonalizableTypeEntry.cs
- GradientStopCollection.cs
- InternalCache.cs
- FunctionMappingTranslator.cs
- EntityDataSourceContainerNameConverter.cs
- Expr.cs
- TextRunCache.cs
- DaylightTime.cs
- DataGridViewButtonColumn.cs
- ProviderSettings.cs
- RegistrationServices.cs
- MessageQueueException.cs
- CacheMemory.cs
- DataGridViewCellCollection.cs
- mediaeventshelper.cs
- ArgumentNullException.cs
- DbReferenceCollection.cs
- SqlUserDefinedTypeAttribute.cs
- FixedPosition.cs
- CreateUserWizardStep.cs
- ComponentResourceManager.cs
- MobileTemplatedControlDesigner.cs
- ReflectionServiceProvider.cs
- ManifestResourceInfo.cs
- MultipleViewPattern.cs
- RtfNavigator.cs
- TemplateBindingExtension.cs
- ControlBuilder.cs
- FontClient.cs
- WindowsEditBoxRange.cs
- HttpServerVarsCollection.cs
- ModuleElement.cs
- CodeIdentifiers.cs
- MsmqMessageSerializationFormat.cs
- ToolStripContextMenu.cs