Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridViewCellCollection.cs / 1 / DataGridViewCellCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Diagnostics; using System.Collections; using System.Windows.Forms; using System.ComponentModel; using System.Globalization; using System.Diagnostics.CodeAnalysis; ////// /// [ ListBindable(false), SuppressMessage("Microsoft.Design", "CA1010:CollectionsShouldImplementGenericInterface") // Consider adding an IListRepresents a collection of ///objects in the /// control. implementation ] public class DataGridViewCellCollection : BaseCollection, IList { CollectionChangeEventHandler onCollectionChanged; ArrayList items = new ArrayList(); DataGridViewRow owner = null; /// /// int IList.Add(object value) { return this.Add((DataGridViewCell) value); } /// /// void IList.Clear() { this.Clear(); } /// /// bool IList.Contains(object value) { return this.items.Contains(value); } /// /// int IList.IndexOf(object value) { return this.items.IndexOf(value); } /// /// void IList.Insert(int index, object value) { this.Insert(index, (DataGridViewCell) value); } /// /// void IList.Remove(object value) { this.Remove((DataGridViewCell) value); } /// /// void IList.RemoveAt(int index) { this.RemoveAt(index); } /// /// bool IList.IsFixedSize { get {return false;} } /// /// bool IList.IsReadOnly { get {return false;} } /// /// object IList.this[int index] { get { return this[index]; } set { this[index] = (DataGridViewCell) value; } } /// /// void ICollection.CopyTo(Array array, int index) { this.items.CopyTo(array, index); } /// /// int ICollection.Count { get {return this.items.Count;} } /// /// bool ICollection.IsSynchronized { get {return false;} } /// /// object ICollection.SyncRoot { get {return this;} } /// /// IEnumerator IEnumerable.GetEnumerator() { return this.items.GetEnumerator(); } /// public DataGridViewCellCollection(DataGridViewRow dataGridViewRow) { Debug.Assert(dataGridViewRow != null); this.owner = dataGridViewRow; } /// /// /// protected override ArrayList List { get { return this.items; } } ///[To be supplied.] ////// /// Retrieves the DataGridViewCell with the specified index. /// public DataGridViewCell this[int index] { get { return (DataGridViewCell) this.items[index]; } set { DataGridViewCell dataGridViewCell = value; if (dataGridViewCell == null) { throw new ArgumentNullException("value"); } if (dataGridViewCell.DataGridView != null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_CellAlreadyBelongsToDataGridView)); } if (dataGridViewCell.OwningRow != null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_CellAlreadyBelongsToDataGridViewRow)); } if (this.owner.DataGridView != null) { this.owner.DataGridView.OnReplacingCell(this.owner, index); } DataGridViewCell oldDataGridViewCell = (DataGridViewCell) this.items[index]; this.items[index] = dataGridViewCell; dataGridViewCell.OwningRowInternal = this.owner; dataGridViewCell.StateInternal = oldDataGridViewCell.State; if (this.owner.DataGridView != null) { dataGridViewCell.DataGridViewInternal = this.owner.DataGridView; dataGridViewCell.OwningColumnInternal = this.owner.DataGridView.Columns[index]; this.owner.DataGridView.OnReplacedCell(this.owner, index); } oldDataGridViewCell.DataGridViewInternal = null; oldDataGridViewCell.OwningRowInternal = null; oldDataGridViewCell.OwningColumnInternal = null; if (oldDataGridViewCell.ReadOnly) { oldDataGridViewCell.ReadOnlyInternal = false; } if (oldDataGridViewCell.Selected) { oldDataGridViewCell.SelectedInternal = false; } } } ////// /// Retrieves the DataGridViewCell with the specified column name. /// public DataGridViewCell this[string columnName] { get { DataGridViewColumn dataGridViewColumn = null; if (this.owner.DataGridView != null) { dataGridViewColumn = this.owner.DataGridView.Columns[columnName]; } if (dataGridViewColumn == null) { throw new ArgumentException(SR.GetString(SR.DataGridViewColumnCollection_ColumnNotFound, columnName), "columnName"); } return (DataGridViewCell) this.items[dataGridViewColumn.Index]; } set { DataGridViewColumn dataGridViewColumn = null; if (this.owner.DataGridView != null) { dataGridViewColumn = this.owner.DataGridView.Columns[columnName]; } if (dataGridViewColumn == null) { throw new ArgumentException(SR.GetString(SR.DataGridViewColumnCollection_ColumnNotFound, columnName), "columnName"); } this[dataGridViewColumn.Index] = value; } } ////// /// public event CollectionChangeEventHandler CollectionChanged { add { this.onCollectionChanged += value; } remove { this.onCollectionChanged -= value; } } ///[To be supplied.] ////// /// public virtual int Add(DataGridViewCell dataGridViewCell) { if (this.owner.DataGridView != null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_OwningRowAlreadyBelongsToDataGridView)); } if (dataGridViewCell.OwningRow != null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_CellAlreadyBelongsToDataGridViewRow)); } Debug.Assert(!dataGridViewCell.ReadOnly); return AddInternal(dataGridViewCell); } internal int AddInternal(DataGridViewCell dataGridViewCell) { Debug.Assert(!dataGridViewCell.Selected); int index = this.items.Add(dataGridViewCell); dataGridViewCell.OwningRowInternal = this.owner; DataGridView dataGridView = this.owner.DataGridView; if (dataGridView != null && dataGridView.Columns.Count > index) { dataGridViewCell.OwningColumnInternal = dataGridView.Columns[index]; } OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Add, dataGridViewCell)); return index; } ///Adds a ///to this collection. /// /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public virtual void AddRange(params DataGridViewCell[] dataGridViewCells) { if (dataGridViewCells == null) { throw new ArgumentNullException("dataGridViewCells"); } if (this.owner.DataGridView != null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_OwningRowAlreadyBelongsToDataGridView)); } foreach (DataGridViewCell dataGridViewCell in dataGridViewCells) { if (dataGridViewCell == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_AtLeastOneCellIsNull)); } if (dataGridViewCell.OwningRow != null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_CellAlreadyBelongsToDataGridViewRow)); } } // Make sure no two cells are identical int cellCount = dataGridViewCells.Length; for (int cell1 = 0; cell1 < cellCount - 1; cell1++) { for (int cell2 = cell1 + 1; cell2 < cellCount; cell2++) { if (dataGridViewCells[cell1] == dataGridViewCells[cell2]) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_CannotAddIdenticalCells)); } } } this.items.AddRange(dataGridViewCells); foreach (DataGridViewCell dataGridViewCell in dataGridViewCells) { dataGridViewCell.OwningRowInternal = this.owner; Debug.Assert(!dataGridViewCell.ReadOnly); Debug.Assert(!dataGridViewCell.Selected); } OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Refresh, null)); } ///[To be supplied.] ////// /// public virtual void Clear() { if (this.owner.DataGridView != null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_OwningRowAlreadyBelongsToDataGridView)); } foreach (DataGridViewCell dataGridViewCell in this.items) { dataGridViewCell.OwningRowInternal = null; } this.items.Clear(); OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Refresh, null)); } ///[To be supplied.] ///public void CopyTo(DataGridViewCell[] array, int index) { this.items.CopyTo(array, index); } /// /// /// Checks to see if a DataGridViewCell is contained in this collection. /// public virtual bool Contains(DataGridViewCell dataGridViewCell) { int index = this.items.IndexOf(dataGridViewCell); return index != -1; } ///public int IndexOf(DataGridViewCell dataGridViewCell) { return this.items.IndexOf(dataGridViewCell); } /// public virtual void Insert(int index, DataGridViewCell dataGridViewCell) { if (this.owner.DataGridView != null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_OwningRowAlreadyBelongsToDataGridView)); } if (dataGridViewCell.OwningRow != null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_CellAlreadyBelongsToDataGridViewRow)); } Debug.Assert(!dataGridViewCell.ReadOnly); Debug.Assert(!dataGridViewCell.Selected); this.items.Insert(index, dataGridViewCell); dataGridViewCell.OwningRowInternal = this.owner; OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Add, dataGridViewCell)); } internal void InsertInternal(int index, DataGridViewCell dataGridViewCell) { Debug.Assert(!dataGridViewCell.Selected); this.items.Insert(index, dataGridViewCell); dataGridViewCell.OwningRowInternal = this.owner; DataGridView dataGridView = this.owner.DataGridView; if (dataGridView != null && dataGridView.Columns.Count > index) { dataGridViewCell.OwningColumnInternal = dataGridView.Columns[index]; } OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Add, dataGridViewCell)); } /// /// /// protected void OnCollectionChanged(CollectionChangeEventArgs e) { if (this.onCollectionChanged != null) { this.onCollectionChanged(this, e); } } ///[To be supplied.] ////// /// public virtual void Remove(DataGridViewCell cell) { if (this.owner.DataGridView != null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_OwningRowAlreadyBelongsToDataGridView)); } int cellIndex = -1; int itemsCount = this.items.Count; for (int i = 0; i < itemsCount; ++i) { if (this.items[i] == cell) { cellIndex = i; break; } } if (cellIndex == -1) { throw new ArgumentException(SR.GetString(SR.DataGridViewCellCollection_CellNotFound)); } else { RemoveAt(cellIndex); } } ///[To be supplied.] ////// /// public virtual void RemoveAt(int index) { if (this.owner.DataGridView != null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_OwningRowAlreadyBelongsToDataGridView)); } RemoveAtInternal(index); } internal void RemoveAtInternal(int index) { DataGridViewCell dataGridViewCell = (DataGridViewCell) this.items[index]; this.items.RemoveAt(index); dataGridViewCell.DataGridViewInternal = null; dataGridViewCell.OwningRowInternal = null; if (dataGridViewCell.ReadOnly) { dataGridViewCell.ReadOnlyInternal = false; } if (dataGridViewCell.Selected) { dataGridViewCell.SelectedInternal = false; } OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Remove, dataGridViewCell)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Diagnostics; using System.Collections; using System.Windows.Forms; using System.ComponentModel; using System.Globalization; using System.Diagnostics.CodeAnalysis; ////// /// [ ListBindable(false), SuppressMessage("Microsoft.Design", "CA1010:CollectionsShouldImplementGenericInterface") // Consider adding an IListRepresents a collection of ///objects in the /// control. implementation ] public class DataGridViewCellCollection : BaseCollection, IList { CollectionChangeEventHandler onCollectionChanged; ArrayList items = new ArrayList(); DataGridViewRow owner = null; /// /// int IList.Add(object value) { return this.Add((DataGridViewCell) value); } /// /// void IList.Clear() { this.Clear(); } /// /// bool IList.Contains(object value) { return this.items.Contains(value); } /// /// int IList.IndexOf(object value) { return this.items.IndexOf(value); } /// /// void IList.Insert(int index, object value) { this.Insert(index, (DataGridViewCell) value); } /// /// void IList.Remove(object value) { this.Remove((DataGridViewCell) value); } /// /// void IList.RemoveAt(int index) { this.RemoveAt(index); } /// /// bool IList.IsFixedSize { get {return false;} } /// /// bool IList.IsReadOnly { get {return false;} } /// /// object IList.this[int index] { get { return this[index]; } set { this[index] = (DataGridViewCell) value; } } /// /// void ICollection.CopyTo(Array array, int index) { this.items.CopyTo(array, index); } /// /// int ICollection.Count { get {return this.items.Count;} } /// /// bool ICollection.IsSynchronized { get {return false;} } /// /// object ICollection.SyncRoot { get {return this;} } /// /// IEnumerator IEnumerable.GetEnumerator() { return this.items.GetEnumerator(); } /// public DataGridViewCellCollection(DataGridViewRow dataGridViewRow) { Debug.Assert(dataGridViewRow != null); this.owner = dataGridViewRow; } /// /// /// protected override ArrayList List { get { return this.items; } } ///[To be supplied.] ////// /// Retrieves the DataGridViewCell with the specified index. /// public DataGridViewCell this[int index] { get { return (DataGridViewCell) this.items[index]; } set { DataGridViewCell dataGridViewCell = value; if (dataGridViewCell == null) { throw new ArgumentNullException("value"); } if (dataGridViewCell.DataGridView != null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_CellAlreadyBelongsToDataGridView)); } if (dataGridViewCell.OwningRow != null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_CellAlreadyBelongsToDataGridViewRow)); } if (this.owner.DataGridView != null) { this.owner.DataGridView.OnReplacingCell(this.owner, index); } DataGridViewCell oldDataGridViewCell = (DataGridViewCell) this.items[index]; this.items[index] = dataGridViewCell; dataGridViewCell.OwningRowInternal = this.owner; dataGridViewCell.StateInternal = oldDataGridViewCell.State; if (this.owner.DataGridView != null) { dataGridViewCell.DataGridViewInternal = this.owner.DataGridView; dataGridViewCell.OwningColumnInternal = this.owner.DataGridView.Columns[index]; this.owner.DataGridView.OnReplacedCell(this.owner, index); } oldDataGridViewCell.DataGridViewInternal = null; oldDataGridViewCell.OwningRowInternal = null; oldDataGridViewCell.OwningColumnInternal = null; if (oldDataGridViewCell.ReadOnly) { oldDataGridViewCell.ReadOnlyInternal = false; } if (oldDataGridViewCell.Selected) { oldDataGridViewCell.SelectedInternal = false; } } } ////// /// Retrieves the DataGridViewCell with the specified column name. /// public DataGridViewCell this[string columnName] { get { DataGridViewColumn dataGridViewColumn = null; if (this.owner.DataGridView != null) { dataGridViewColumn = this.owner.DataGridView.Columns[columnName]; } if (dataGridViewColumn == null) { throw new ArgumentException(SR.GetString(SR.DataGridViewColumnCollection_ColumnNotFound, columnName), "columnName"); } return (DataGridViewCell) this.items[dataGridViewColumn.Index]; } set { DataGridViewColumn dataGridViewColumn = null; if (this.owner.DataGridView != null) { dataGridViewColumn = this.owner.DataGridView.Columns[columnName]; } if (dataGridViewColumn == null) { throw new ArgumentException(SR.GetString(SR.DataGridViewColumnCollection_ColumnNotFound, columnName), "columnName"); } this[dataGridViewColumn.Index] = value; } } ////// /// public event CollectionChangeEventHandler CollectionChanged { add { this.onCollectionChanged += value; } remove { this.onCollectionChanged -= value; } } ///[To be supplied.] ////// /// public virtual int Add(DataGridViewCell dataGridViewCell) { if (this.owner.DataGridView != null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_OwningRowAlreadyBelongsToDataGridView)); } if (dataGridViewCell.OwningRow != null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_CellAlreadyBelongsToDataGridViewRow)); } Debug.Assert(!dataGridViewCell.ReadOnly); return AddInternal(dataGridViewCell); } internal int AddInternal(DataGridViewCell dataGridViewCell) { Debug.Assert(!dataGridViewCell.Selected); int index = this.items.Add(dataGridViewCell); dataGridViewCell.OwningRowInternal = this.owner; DataGridView dataGridView = this.owner.DataGridView; if (dataGridView != null && dataGridView.Columns.Count > index) { dataGridViewCell.OwningColumnInternal = dataGridView.Columns[index]; } OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Add, dataGridViewCell)); return index; } ///Adds a ///to this collection. /// /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden)] public virtual void AddRange(params DataGridViewCell[] dataGridViewCells) { if (dataGridViewCells == null) { throw new ArgumentNullException("dataGridViewCells"); } if (this.owner.DataGridView != null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_OwningRowAlreadyBelongsToDataGridView)); } foreach (DataGridViewCell dataGridViewCell in dataGridViewCells) { if (dataGridViewCell == null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_AtLeastOneCellIsNull)); } if (dataGridViewCell.OwningRow != null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_CellAlreadyBelongsToDataGridViewRow)); } } // Make sure no two cells are identical int cellCount = dataGridViewCells.Length; for (int cell1 = 0; cell1 < cellCount - 1; cell1++) { for (int cell2 = cell1 + 1; cell2 < cellCount; cell2++) { if (dataGridViewCells[cell1] == dataGridViewCells[cell2]) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_CannotAddIdenticalCells)); } } } this.items.AddRange(dataGridViewCells); foreach (DataGridViewCell dataGridViewCell in dataGridViewCells) { dataGridViewCell.OwningRowInternal = this.owner; Debug.Assert(!dataGridViewCell.ReadOnly); Debug.Assert(!dataGridViewCell.Selected); } OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Refresh, null)); } ///[To be supplied.] ////// /// public virtual void Clear() { if (this.owner.DataGridView != null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_OwningRowAlreadyBelongsToDataGridView)); } foreach (DataGridViewCell dataGridViewCell in this.items) { dataGridViewCell.OwningRowInternal = null; } this.items.Clear(); OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Refresh, null)); } ///[To be supplied.] ///public void CopyTo(DataGridViewCell[] array, int index) { this.items.CopyTo(array, index); } /// /// /// Checks to see if a DataGridViewCell is contained in this collection. /// public virtual bool Contains(DataGridViewCell dataGridViewCell) { int index = this.items.IndexOf(dataGridViewCell); return index != -1; } ///public int IndexOf(DataGridViewCell dataGridViewCell) { return this.items.IndexOf(dataGridViewCell); } /// public virtual void Insert(int index, DataGridViewCell dataGridViewCell) { if (this.owner.DataGridView != null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_OwningRowAlreadyBelongsToDataGridView)); } if (dataGridViewCell.OwningRow != null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_CellAlreadyBelongsToDataGridViewRow)); } Debug.Assert(!dataGridViewCell.ReadOnly); Debug.Assert(!dataGridViewCell.Selected); this.items.Insert(index, dataGridViewCell); dataGridViewCell.OwningRowInternal = this.owner; OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Add, dataGridViewCell)); } internal void InsertInternal(int index, DataGridViewCell dataGridViewCell) { Debug.Assert(!dataGridViewCell.Selected); this.items.Insert(index, dataGridViewCell); dataGridViewCell.OwningRowInternal = this.owner; DataGridView dataGridView = this.owner.DataGridView; if (dataGridView != null && dataGridView.Columns.Count > index) { dataGridViewCell.OwningColumnInternal = dataGridView.Columns[index]; } OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Add, dataGridViewCell)); } /// /// /// protected void OnCollectionChanged(CollectionChangeEventArgs e) { if (this.onCollectionChanged != null) { this.onCollectionChanged(this, e); } } ///[To be supplied.] ////// /// public virtual void Remove(DataGridViewCell cell) { if (this.owner.DataGridView != null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_OwningRowAlreadyBelongsToDataGridView)); } int cellIndex = -1; int itemsCount = this.items.Count; for (int i = 0; i < itemsCount; ++i) { if (this.items[i] == cell) { cellIndex = i; break; } } if (cellIndex == -1) { throw new ArgumentException(SR.GetString(SR.DataGridViewCellCollection_CellNotFound)); } else { RemoveAt(cellIndex); } } ///[To be supplied.] ////// /// public virtual void RemoveAt(int index) { if (this.owner.DataGridView != null) { throw new InvalidOperationException(SR.GetString(SR.DataGridViewCellCollection_OwningRowAlreadyBelongsToDataGridView)); } RemoveAtInternal(index); } internal void RemoveAtInternal(int index) { DataGridViewCell dataGridViewCell = (DataGridViewCell) this.items[index]; this.items.RemoveAt(index); dataGridViewCell.DataGridViewInternal = null; dataGridViewCell.OwningRowInternal = null; if (dataGridViewCell.ReadOnly) { dataGridViewCell.ReadOnlyInternal = false; } if (dataGridViewCell.Selected) { dataGridViewCell.SelectedInternal = false; } OnCollectionChanged(new CollectionChangeEventArgs(CollectionChangeAction.Remove, dataGridViewCell)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
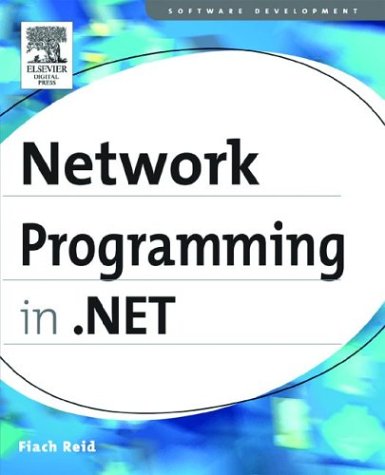
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartEditorCancelVerb.cs
- JoinTreeSlot.cs
- ReliabilityContractAttribute.cs
- EventListenerClientSide.cs
- ObjectTypeMapping.cs
- Int32KeyFrameCollection.cs
- AssemblyCollection.cs
- EntityCommandDefinition.cs
- Delegate.cs
- SecurityMessageProperty.cs
- ValidatingReaderNodeData.cs
- COM2PropertyDescriptor.cs
- _NegotiateClient.cs
- OutputCache.cs
- Point3DCollectionConverter.cs
- PageTextBox.cs
- FileDataSourceCache.cs
- relpropertyhelper.cs
- HtmlMeta.cs
- RSAOAEPKeyExchangeFormatter.cs
- SQLMembershipProvider.cs
- FixedSOMContainer.cs
- Int32AnimationBase.cs
- documentsequencetextcontainer.cs
- CompilationLock.cs
- AbstractExpressions.cs
- OTFRasterizer.cs
- ObjectDataSourceStatusEventArgs.cs
- IImplicitResourceProvider.cs
- WorkflowInstanceExtensionProvider.cs
- PassportAuthenticationModule.cs
- ComplexTypeEmitter.cs
- Vector.cs
- WorkflowRuntimeServicesBehavior.cs
- AttachedAnnotationChangedEventArgs.cs
- InvalidStoreProtectionKeyException.cs
- ThreadPool.cs
- Grant.cs
- SqlServices.cs
- NullReferenceException.cs
- MembershipValidatePasswordEventArgs.cs
- FileEnumerator.cs
- PointAnimation.cs
- InternalBase.cs
- ClientSettingsStore.cs
- DefaultValueTypeConverter.cs
- MultiAsyncResult.cs
- FileSecurity.cs
- RuntimeResourceSet.cs
- ResponseStream.cs
- SmiEventSink_Default.cs
- LinearGradientBrush.cs
- ObjectListCommandEventArgs.cs
- SkipStoryboardToFill.cs
- MailWebEventProvider.cs
- PlacementWorkspace.cs
- WebBrowserHelper.cs
- Directory.cs
- DataComponentGenerator.cs
- Property.cs
- Parser.cs
- ConfigurationErrorsException.cs
- GlyphCache.cs
- GcHandle.cs
- BamlLocalizabilityResolver.cs
- InternalBase.cs
- LongValidator.cs
- Currency.cs
- DropShadowBitmapEffect.cs
- TimeManager.cs
- DispatcherTimer.cs
- MouseBinding.cs
- CodeMemberMethod.cs
- XmlSchemaException.cs
- DeviceSpecificChoiceCollection.cs
- BitmapEffectDrawingContent.cs
- ArgumentOutOfRangeException.cs
- CodeDomDesignerLoader.cs
- WebConfigurationHostFileChange.cs
- CultureInfo.cs
- MissingSatelliteAssemblyException.cs
- GenericAuthenticationEventArgs.cs
- BasePattern.cs
- OleDbConnection.cs
- CompressedStack.cs
- NoneExcludedImageIndexConverter.cs
- DefaultBindingPropertyAttribute.cs
- DataControlFieldsEditor.cs
- ValueUnavailableException.cs
- Registry.cs
- XmlBuffer.cs
- SizeConverter.cs
- TextView.cs
- AdapterDictionary.cs
- DataRowCollection.cs
- MultiSelectRootGridEntry.cs
- FixedSOMGroup.cs
- StructureChangedEventArgs.cs
- Style.cs
- XmlExpressionDumper.cs