Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Markup / XmlnsCache.cs / 1305600 / XmlnsCache.cs
//---------------------------------------------------------------------------- // // File: XmlnsCache.cs // // Description: // Handles local caching operations on the XmlnsCache file used for parsing // // // History: // 5/10/05: peterost Created // // Copyright (C) 2005 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Reflection; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using System.Text; using MS.Internal; using MS.Utility; using Microsoft.Win32; using System.Globalization; #if PBTCOMPILER using MS.Internal.PresentationBuildTasks; #else using MS.Win32.Compile; using MS.Internal.PresentationFramework; using MS.Internal.Utility; // AssemblyCacheEnum #endif // Since we disable PreSharp warnings in this file, we first need to disable warnings about unknown message numbers and unknown pragmas. #pragma warning disable 1634, 1691 #if PBTCOMPILER namespace MS.Internal.Markup #else namespace System.Windows.Markup #endif { internal class XmlnsCache { #if PBTCOMPILER static XmlnsCache() { // if the value of any assembly key entry in _assemblyHasCacheInfo is // false - don't look for xmlnsdefinitionAttribute in that assembly. // if it is true or there is no entry then we need to reflect for this // custom attribute. _assemblyHasCacheInfo["WINDOWSBASE"] = true; _assemblyHasCacheInfo["SYSTEM"] = false; _assemblyHasCacheInfo["SYSTEM.DATA"] = false; _assemblyHasCacheInfo["SYSTEM.XML"] = false; _assemblyHasCacheInfo["UIAUTOMATIONPROVIDER"] = false; _assemblyHasCacheInfo["UIAUTOMATIONTYPES"] = false; _assemblyHasCacheInfo["PRESENTATIONCORE"] = true; _assemblyHasCacheInfo["PRESENTATIONFRAMEWORK"] = true; } // Create a new instance of the namespace / assembly cache. // Use only the assemblies that are contained in the passed table, // where keys are assembly names, and values are paths. internal XmlnsCache(HybridDictionary assemblyPathTable) { InitializeWithReferencedAssemblies(assemblyPathTable); } private void InitializeWithReferencedAssemblies(HybridDictionary assemblyPathTable) { _compatTable = new Dictionary(); _compatTableReverse = new Dictionary (); _cacheTable = new HybridDictionary(); _assemblyPathTable = assemblyPathTable; AddReferencedAssemblies(); } // Table where key is assembly name and value is the file path where it should be found private HybridDictionary _assemblyPathTable = null; // Table where key is assembly name and value indicates if assembly contains any XmlnsDefinitionAttributes private static Hashtable _assemblyHasCacheInfo = new Hashtable(8); // Reflect on any assemblies that can be found in the passed table and look for // XmlnsDefinitionAttributes. Add these to the cache table. private void AddReferencedAssemblies() { if (_assemblyPathTable == null || _assemblyPathTable.Count == 0) { return; } List interestingAssemblies = new List (); // Load all the assemblies into a list. foreach(string assemblyName in _assemblyPathTable.Keys) { bool hasCacheInfo = true; Assembly assy; if (_assemblyHasCacheInfo[assemblyName] != null) { hasCacheInfo = (bool)_assemblyHasCacheInfo[assemblyName]; } if (!hasCacheInfo) { continue; } assy = ReflectionHelper.GetAlreadyReflectionOnlyLoadedAssembly(assemblyName); if (assy == null) { string assemblyFullPath = _assemblyPathTable[assemblyName] as string; // // The assembly path set from Compiler must be a full file path, which includes // directory, file name and extension. // Don't need to try different file extensions here. // if (!String.IsNullOrEmpty(assemblyFullPath) && File.Exists(assemblyFullPath)) { assy = ReflectionHelper.LoadAssembly(assemblyName, assemblyFullPath); } } if (assy != null) { interestingAssemblies.Add(assy); } } Assembly[] asmList = interestingAssemblies.ToArray(); LoadClrnsToAssemblyNameMappingCache(asmList); ProcessXmlnsCompatibleWithAttributes(asmList); } #else internal XmlnsCache() { _compatTable = new Dictionary (); _compatTableReverse = new Dictionary (); _cacheTable = new HybridDictionary(); _uriToAssemblyNameTable = new HybridDictionary(); } #endif #if PBTCOMPILER // In the COmpiler everything is taken care of up front (all the // assemblies are listed in the PROJ file). So the cache will be // fully populated and ready. internal List GetMappingArray(string xmlns) { return _cacheTable[xmlns] as List ; } #else // At runtime the cache is lazily populated as XmlNs URI's are // encounted in the BAML. If the cache line is loaded return what // you find, other wise go load the cache. internal List GetMappingArray(string xmlns) { List clrNsMapping =null; lock(this) { clrNsMapping = _cacheTable[xmlns] as List ; if (clrNsMapping == null) { if (_uriToAssemblyNameTable[xmlns] != null) { // // if the xmlns maps to a list of assembly names which are saved in the baml records, // try to get the mapping from those assemblies. // string[] asmNameList = _uriToAssemblyNameTable[xmlns] as string[]; Assembly[] asmList = new Assembly[asmNameList.Length]; for (int i = 0; i < asmNameList.Length; i++) { asmList[i] = ReflectionHelper.LoadAssembly(asmNameList[i], null); } _cacheTable[xmlns] = GetClrnsToAssemblyNameMappingList(asmList, xmlns); } else { // // The xmlns uri doesn't map to a list of assemblies, this might be for // regular xaml loading. // // Get the xmlns - clrns mapping from the currently loaded assemblies. // Assembly[] asmList = AppDomain.CurrentDomain.GetAssemblies(); _cacheTable[xmlns] = GetClrnsToAssemblyNameMappingList(asmList, xmlns); ProcessXmlnsCompatibleWithAttributes(asmList); } clrNsMapping = _cacheTable[xmlns] as List ; } } return clrNsMapping; } internal void SetUriToAssemblyNameMapping(string namespaceUri, string [] asmNameList) { _uriToAssemblyNameTable[namespaceUri] = asmNameList; } #endif // Return the new compatible namespace, given an old namespace internal string GetNewXmlnamespace(string oldXmlnamespace) { string newXmlNamespace; if (_compatTable.TryGetValue(oldXmlnamespace, out newXmlNamespace)) { return newXmlNamespace; } return null; } #if PBTCOMPILER private CustomAttributeData[] GetAttributes(Assembly asm, string fullClrName) { IList allAttributes = CustomAttributeData.GetCustomAttributes(asm); List foundAttributes = new List (); for(int i=0; i constructorArguments = data.ConstructorArguments; for (int i = 0; i constructorArguments = data.ConstructorArguments; for (int i=0; i pairList; // For each assembly, enmerate all the XmlnsDefinition attributes. for(int asmIdx=0; asmIdx (); } pairList = _cacheTable[xmlns] as List ; pairList.Add(new ClrNamespaceAssemblyPair(clrns, assemblyName)); } } } #else // Get a list of (clrNs, asmName) pairs for a given XmlNs from a // given list of Assemblies. This returns a list that the caller // Will add to the _cacheTable. At runtime the needed assemblies // appear with various XmlNs namespaces one at a time as we read the BAML private List GetClrnsToAssemblyNameMappingList( Assembly[] asmList, string xmlnsRequested ) { List pairList = new List (); // For each assembly, enmerate all the XmlnsDefinition attributes. for(int asmIdx=0; asmIdx _compatTable = null; // Table where new namespaces are the key, and old namespaces are the value private Dictionary _compatTableReverse = null; #if !PBTCOMPILER private HybridDictionary _uriToAssemblyNameTable = null; #endif } // Structure used to associate a Clr namespace with the assembly that contains it. internal struct ClrNamespaceAssemblyPair { // constructor internal ClrNamespaceAssemblyPair(string clrNamespace,string assemblyName) { _clrNamespace = clrNamespace; _assemblyName = assemblyName; #if PBTCOMPILER _localAssembly = false; #endif } // AssemblyName specified in the using Data internal string AssemblyName { get { return _assemblyName; } } // ClrNamespace portion of the using syntax internal string ClrNamespace { get { return _clrNamespace; } } #if PBTCOMPILER internal bool LocalAssembly { get { return _localAssembly; } set { _localAssembly = value; } } #endif #if PBTCOMPILER bool _localAssembly; #endif string _assemblyName; string _clrNamespace; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: XmlnsCache.cs // // Description: // Handles local caching operations on the XmlnsCache file used for parsing // // // History: // 5/10/05: peterost Created // // Copyright (C) 2005 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Reflection; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using System.Text; using MS.Internal; using MS.Utility; using Microsoft.Win32; using System.Globalization; #if PBTCOMPILER using MS.Internal.PresentationBuildTasks; #else using MS.Win32.Compile; using MS.Internal.PresentationFramework; using MS.Internal.Utility; // AssemblyCacheEnum #endif // Since we disable PreSharp warnings in this file, we first need to disable warnings about unknown message numbers and unknown pragmas. #pragma warning disable 1634, 1691 #if PBTCOMPILER namespace MS.Internal.Markup #else namespace System.Windows.Markup #endif { internal class XmlnsCache { #if PBTCOMPILER static XmlnsCache() { // if the value of any assembly key entry in _assemblyHasCacheInfo is // false - don't look for xmlnsdefinitionAttribute in that assembly. // if it is true or there is no entry then we need to reflect for this // custom attribute. _assemblyHasCacheInfo["WINDOWSBASE"] = true; _assemblyHasCacheInfo["SYSTEM"] = false; _assemblyHasCacheInfo["SYSTEM.DATA"] = false; _assemblyHasCacheInfo["SYSTEM.XML"] = false; _assemblyHasCacheInfo["UIAUTOMATIONPROVIDER"] = false; _assemblyHasCacheInfo["UIAUTOMATIONTYPES"] = false; _assemblyHasCacheInfo["PRESENTATIONCORE"] = true; _assemblyHasCacheInfo["PRESENTATIONFRAMEWORK"] = true; } // Create a new instance of the namespace / assembly cache. // Use only the assemblies that are contained in the passed table, // where keys are assembly names, and values are paths. internal XmlnsCache(HybridDictionary assemblyPathTable) { InitializeWithReferencedAssemblies(assemblyPathTable); } private void InitializeWithReferencedAssemblies(HybridDictionary assemblyPathTable) { _compatTable = new Dictionary (); _compatTableReverse = new Dictionary (); _cacheTable = new HybridDictionary(); _assemblyPathTable = assemblyPathTable; AddReferencedAssemblies(); } // Table where key is assembly name and value is the file path where it should be found private HybridDictionary _assemblyPathTable = null; // Table where key is assembly name and value indicates if assembly contains any XmlnsDefinitionAttributes private static Hashtable _assemblyHasCacheInfo = new Hashtable(8); // Reflect on any assemblies that can be found in the passed table and look for // XmlnsDefinitionAttributes. Add these to the cache table. private void AddReferencedAssemblies() { if (_assemblyPathTable == null || _assemblyPathTable.Count == 0) { return; } List interestingAssemblies = new List (); // Load all the assemblies into a list. foreach(string assemblyName in _assemblyPathTable.Keys) { bool hasCacheInfo = true; Assembly assy; if (_assemblyHasCacheInfo[assemblyName] != null) { hasCacheInfo = (bool)_assemblyHasCacheInfo[assemblyName]; } if (!hasCacheInfo) { continue; } assy = ReflectionHelper.GetAlreadyReflectionOnlyLoadedAssembly(assemblyName); if (assy == null) { string assemblyFullPath = _assemblyPathTable[assemblyName] as string; // // The assembly path set from Compiler must be a full file path, which includes // directory, file name and extension. // Don't need to try different file extensions here. // if (!String.IsNullOrEmpty(assemblyFullPath) && File.Exists(assemblyFullPath)) { assy = ReflectionHelper.LoadAssembly(assemblyName, assemblyFullPath); } } if (assy != null) { interestingAssemblies.Add(assy); } } Assembly[] asmList = interestingAssemblies.ToArray(); LoadClrnsToAssemblyNameMappingCache(asmList); ProcessXmlnsCompatibleWithAttributes(asmList); } #else internal XmlnsCache() { _compatTable = new Dictionary (); _compatTableReverse = new Dictionary (); _cacheTable = new HybridDictionary(); _uriToAssemblyNameTable = new HybridDictionary(); } #endif #if PBTCOMPILER // In the COmpiler everything is taken care of up front (all the // assemblies are listed in the PROJ file). So the cache will be // fully populated and ready. internal List GetMappingArray(string xmlns) { return _cacheTable[xmlns] as List ; } #else // At runtime the cache is lazily populated as XmlNs URI's are // encounted in the BAML. If the cache line is loaded return what // you find, other wise go load the cache. internal List GetMappingArray(string xmlns) { List clrNsMapping =null; lock(this) { clrNsMapping = _cacheTable[xmlns] as List ; if (clrNsMapping == null) { if (_uriToAssemblyNameTable[xmlns] != null) { // // if the xmlns maps to a list of assembly names which are saved in the baml records, // try to get the mapping from those assemblies. // string[] asmNameList = _uriToAssemblyNameTable[xmlns] as string[]; Assembly[] asmList = new Assembly[asmNameList.Length]; for (int i = 0; i < asmNameList.Length; i++) { asmList[i] = ReflectionHelper.LoadAssembly(asmNameList[i], null); } _cacheTable[xmlns] = GetClrnsToAssemblyNameMappingList(asmList, xmlns); } else { // // The xmlns uri doesn't map to a list of assemblies, this might be for // regular xaml loading. // // Get the xmlns - clrns mapping from the currently loaded assemblies. // Assembly[] asmList = AppDomain.CurrentDomain.GetAssemblies(); _cacheTable[xmlns] = GetClrnsToAssemblyNameMappingList(asmList, xmlns); ProcessXmlnsCompatibleWithAttributes(asmList); } clrNsMapping = _cacheTable[xmlns] as List ; } } return clrNsMapping; } internal void SetUriToAssemblyNameMapping(string namespaceUri, string [] asmNameList) { _uriToAssemblyNameTable[namespaceUri] = asmNameList; } #endif // Return the new compatible namespace, given an old namespace internal string GetNewXmlnamespace(string oldXmlnamespace) { string newXmlNamespace; if (_compatTable.TryGetValue(oldXmlnamespace, out newXmlNamespace)) { return newXmlNamespace; } return null; } #if PBTCOMPILER private CustomAttributeData[] GetAttributes(Assembly asm, string fullClrName) { IList allAttributes = CustomAttributeData.GetCustomAttributes(asm); List foundAttributes = new List (); for(int i=0; i constructorArguments = data.ConstructorArguments; for (int i = 0; i constructorArguments = data.ConstructorArguments; for (int i=0; i pairList; // For each assembly, enmerate all the XmlnsDefinition attributes. for(int asmIdx=0; asmIdx (); } pairList = _cacheTable[xmlns] as List ; pairList.Add(new ClrNamespaceAssemblyPair(clrns, assemblyName)); } } } #else // Get a list of (clrNs, asmName) pairs for a given XmlNs from a // given list of Assemblies. This returns a list that the caller // Will add to the _cacheTable. At runtime the needed assemblies // appear with various XmlNs namespaces one at a time as we read the BAML private List GetClrnsToAssemblyNameMappingList( Assembly[] asmList, string xmlnsRequested ) { List pairList = new List (); // For each assembly, enmerate all the XmlnsDefinition attributes. for(int asmIdx=0; asmIdx _compatTable = null; // Table where new namespaces are the key, and old namespaces are the value private Dictionary _compatTableReverse = null; #if !PBTCOMPILER private HybridDictionary _uriToAssemblyNameTable = null; #endif } // Structure used to associate a Clr namespace with the assembly that contains it. internal struct ClrNamespaceAssemblyPair { // constructor internal ClrNamespaceAssemblyPair(string clrNamespace,string assemblyName) { _clrNamespace = clrNamespace; _assemblyName = assemblyName; #if PBTCOMPILER _localAssembly = false; #endif } // AssemblyName specified in the using Data internal string AssemblyName { get { return _assemblyName; } } // ClrNamespace portion of the using syntax internal string ClrNamespace { get { return _clrNamespace; } } #if PBTCOMPILER internal bool LocalAssembly { get { return _localAssembly; } set { _localAssembly = value; } } #endif #if PBTCOMPILER bool _localAssembly; #endif string _assemblyName; string _clrNamespace; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
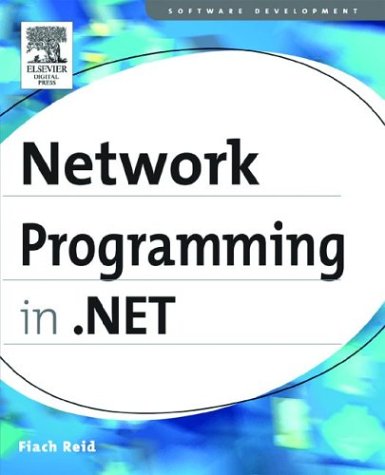
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MenuBindingsEditorForm.cs
- BindingRestrictions.cs
- OutgoingWebResponseContext.cs
- StateWorkerRequest.cs
- KoreanLunisolarCalendar.cs
- TemplateControlParser.cs
- CompilationRelaxations.cs
- DataGridColumnCollection.cs
- TextTreeDeleteContentUndoUnit.cs
- BitmapScalingModeValidation.cs
- CodeTypeConstructor.cs
- ValidationService.cs
- AssertValidation.cs
- SqlDependency.cs
- AssemblyAttributes.cs
- RequestResponse.cs
- DebugControllerThread.cs
- BamlBinaryReader.cs
- FramingEncoders.cs
- InfoCardClaim.cs
- DataSourceHelper.cs
- GrammarBuilderWildcard.cs
- XmlNamespaceMapping.cs
- CollectionView.cs
- Font.cs
- DefaultPrintController.cs
- ShaderEffect.cs
- Enlistment.cs
- ContainerVisual.cs
- UpdatePanelTrigger.cs
- StretchValidation.cs
- CryptoStream.cs
- UserControl.cs
- StringArrayConverter.cs
- ResourcePermissionBase.cs
- CollectionType.cs
- RoutedEventConverter.cs
- EdmToObjectNamespaceMap.cs
- SqlSelectStatement.cs
- PersistenceTypeAttribute.cs
- LinearQuaternionKeyFrame.cs
- UriParserTemplates.cs
- SmtpNetworkElement.cs
- DbBuffer.cs
- UIElementHelper.cs
- NeutralResourcesLanguageAttribute.cs
- SchemaNames.cs
- VirtualizedCellInfoCollection.cs
- SelectionManager.cs
- TextChange.cs
- ResourceDescriptionAttribute.cs
- BufferedStream.cs
- Activity.cs
- DictionaryTraceRecord.cs
- ClientBuildManager.cs
- WebPartCancelEventArgs.cs
- JsonSerializer.cs
- IntersectQueryOperator.cs
- VirtualizingPanel.cs
- StaticExtension.cs
- LightweightCodeGenerator.cs
- MessageContractMemberAttribute.cs
- TraceShell.cs
- PointLightBase.cs
- TransformerTypeCollection.cs
- RuntimeConfigLKG.cs
- GridEntryCollection.cs
- ArgumentOutOfRangeException.cs
- XsdDateTime.cs
- RadioButtonBaseAdapter.cs
- Context.cs
- Parser.cs
- ObjectParameterCollection.cs
- MD5.cs
- GlyphInfoList.cs
- TransformGroup.cs
- WorkflowServiceHostFactory.cs
- PixelFormatConverter.cs
- ObjectDataSource.cs
- shaper.cs
- XmlSchemaProviderAttribute.cs
- HtmlProps.cs
- WebEvents.cs
- MarkupCompilePass1.cs
- ImpersonationContext.cs
- SafeSystemMetrics.cs
- DbConnectionClosed.cs
- util.cs
- RegularExpressionValidator.cs
- httpapplicationstate.cs
- CompensableActivity.cs
- SingleAnimation.cs
- FrameworkElementFactoryMarkupObject.cs
- DataBindEngine.cs
- Matrix.cs
- AppDomainFactory.cs
- PerformanceCounterPermissionAttribute.cs
- TypedTableBase.cs
- JsonSerializer.cs
- QueryOperationResponseOfT.cs